An abstract class in java is a class that is a non-concrete class. By means of non-concrete is something that is not complete. You must think what’s the meaning of not complete? How we can say the class is incomplete?
The answer is here if a class contains an abstract method then the class is known as non-concrete (incomplete class). In this article, we will discuss the use of abstract class in java and java abstract class example
Here is the table content of the article will we will cover this topic.
1. What is an abstract class in Java?
2. Properties of an abstract class?
3. What is the abstract method in java?
4. Rules of abstract method in java?
5. Purpose of Abstract method?
6. How abstract method works with the final keyword?
7. How abstract method works with the private keyword?
8. How abstract method works with the static keyword?
9. How abstract method works with the synchronized keyword?
10. How abstract method works with the strictfp keyword?
11. How to create an Abstract class in java?
12. Why can’t we create the object of an abstract class?
13. How to use abstract classes and abstract methods in Real life?
14. When to use the abstract class?
15. Can an abstract class be final in java?
16. Can we create an instance of an abstract class?
17. Can an abstract class have a constructor?
18. Why can an abstract class have constructors in Java?
What is an abstract class in Java?
A class that is declared with an abstract keyword is known as an abstract class. An abstract class can have abstract methods (methods without the body) and concrete/non-abstract methods (Methods with the body) also. A normal class can’t have any abstract method.
The abstract class provides the abstraction level from 1 to 100%. The level of abstraction is totally depending upon the abstract methods. If an abstract class has an abstract method and non-abstract method, then the abstraction level lies between 1 to 100. But if an abstract class contains only abstract methods then it provides 100% abstraction. We can use any number of abstract methods in the abstract class as per the use.
Properties of an abstract class
1. An abstract class is always declared with an abstract keyword. If a class is declared with an
abstract keyword then JVM considers it as an abstract class.
2. If we are declaring an abstract method in any class then it must be an abstract class otherwise it gives a compilation error. Because this is the general rule of the abstract class.
3. We can’t create an object of the abstract class. It means we can’t instantiate the abstract class.
4. It can contain abstract methods and non-abstract methods.
5. It can contain constructors and static methods.
6. It can contain final methods.
7. If you are declaring a class an abstract class, then there must be a class that extends it and
provide implementation to abstract methods in it.
8. If you inherit an abstract class in the Concrete class then you must need to provide
implementations to all the abstract methods in it. Otherwise, it gives a compilation error.
9. If you inherit an abstract class in another abstract class then it depends upon the developer whether he wants to provide the implementation in this class or later.
Before we learn about it in detail, we need to understand abstract methods.
What is the abstract method in java?
An abstract method in java and the use of abstract method in java. Many programmers have a common question if there is an abstract method in a class then? So let’s start, The abstract method is part of abstraction in java. A method declared without a body within an abstract class/interface is an abstract method. We declare the abstract method only when we require just method declaration in Parent classes and implementation will provide by child classes. We can declare an abstract method by use of the abstract keyword. This is a special type of method because it doesn’t have a body. An abstract method is declared either within the abstract class or interface. You can’t declare an abstract method in concrete class. To declare an abstract method, we have to follow the syntax:
// This is an abstract method abstract return_Type methodName();
To make an abstract method you must provide an abstract keyword at the time of declaration.
Rules of abstract method in java
- To declare the method as abstract, use the abstract keyword.
- You must place the abstract keyword before the method name while you are declaring the method.
- An abstract method does not contain a method body.
- Instead of curly braces, an abstract method will have a semicolon (;) at the end.
// This is an abstract method without implementation public abstract void calculates();
Purpose of Abstract method
Let’s discuss a java abstract method example If a programmer doesn’t want to provide an implementation of the method at the time of declaration. And he/she wants to provide the implementation in the derived class. This can be achieved by specifying the abstract keyword. Sometimes, the programmer doesn’t know what will be the implementation of the method in different scenarios. So, it will be the derived class’s responsibility to provide an implementation of abstract methods.
abstract class Data { abstract void showData(); } class NationalSchool extends Data { @Override void showData() { System.out.println("This is National School's data"); } } class IndianSchool extends Data { @Override void showData() { System.out.println("This is Indian School's data"); } } public class MainClass { public static void main(String arg[]) { Data obj = new NationalSchool(); obj.showData(); Data obj1 = new IndianSchool(); obj1.showData(); } }
Output: This is National School’s data
This is Indian School’s data
Explanation of Example: Let’s say a programmer want to print the data from different school but he doesn’t know want will be output from different schools. So, he/she declares an abstract method in an abstract class. So that each derived class can provide implements its body according to its functionality. In this example we have three classes, one is abstract class Data and two class is Concrete/non-abstract. Data is an abstract class having one abstract method showData().
So, when a programmer extends the Data class, It is the programmer’s responsibility to provide an implementation of the showData() method in derived classes.
How abstract method works with the final keyword?
An abstract method can’t declare as final.
Reason: abstract and final terms are opposite to each other. An abstract method says you must redefine it in a derived class. A final method says you can’t redefine this method in a derived class. Let’s see how the use of abstract methods in java.
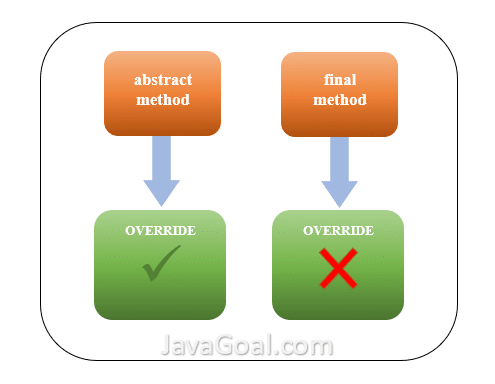
abstract class Data { abstract final void showData(); } class NationalSchool extends Data { @Override void showData() { System.out.println("This is National School's data"); } } class MainClass { public static void main(String arg[]) { Data obj = new NationalSchool(); obj.showData(); } }
Output: This will show compile time exception.
How abstract method works with the private keyword?
An abstract method can’t be private because a private method is not accessible outside the class.
Reason: An abstract method says programmers have to provide the implementation in the derived class. The programmer provides implementation only if it is accessible from outside the class. A private method is not accessible from outside the class. So, we can’t use the private keyword with abstract methods.
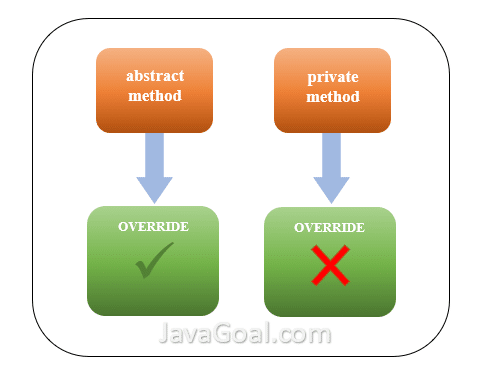
abstract class Data { abstract private void showData(); } class NationalSchool extends Data { @Override void showData() { System.out.println("This is National School's data"); } } class MainClass { public static void main(String arg[]) { Data obj = new NationalSchool(); obj.showData(); } }
Output: This will show compile time exception.
The abstract method showData() in-type Data can only set a visibility modifier, one of public or protected.
How abstract method works with the static keyword?
An abstract method can’t be static because we can’t override a static method.
Reason: If Java allows us to create an abstract static method(Method without body) and someone calls the abstract method using the class name(because the static method can access directly by class name), then What would happen? Because it will be an incomplete method.
abstract class Data { static abstract void showData(); } class NationalSchool extends Data { @Override void showData() { System.out.println("This is National School's data"); } } class MainClass { public static void main(String arg[]) { Data obj = new NationalSchool(); obj.showData(); } }
Output: This will show compile time exception.
How abstract method works with the synchronized keyword?
An abstract method can’t be synchronized because a thread gets a lock on an object when it enters the method.
Reason: A thread that enters the synchronized method must get the lock of the object (or of the class) in which the method is defined. We can’t create an abstract class so there is no object with the lock.
abstract class Data { Abstract synchronized void showData(); } class NationalSchool extends Data { @Override void showData() { System.out.println("This is National School's data"); } } class MainClass { public static void main(String arg[]) { Data obj = new NationalSchool(); obj.showData(); } }
Output: This will show compile time exception.
How abstract method works with the strictfp keyword?
An abstract method can’t be strictfp.
Reason: Strictfp is a modifier and as you know we can change the modifier of an overridden method (method of parent class) while overriding the method in the child class. It would make no sense to make an abstract method strictfp. That is, methods do not inherit modifiers.
NOTE: No modifiers are allowed on abstract methods except public and protected. You’ll get a compile-time error if you try.
Example:
abstract class Data { Abstract strictfp void showData(); } class NationalSchool extends Data { @Override void showData() { System.out.println("This is National School's data"); } } class MainClass { public static void main(String arg[]) { Data obj = new NationalSchool(); obj.showData(); } }
Output: This will show compile time exception.
How to create an Abstract class in java?
//Declaration of the abstract class by using abstract keyword abstract class className { //abstract method because its hasn’t body abstract access_Specifier methodName(); }
abstract: It is a keyword that must be used at the time of declaration.
class_Name: You can provide class names according to your functionality.
abstract method: Each abstract class has at least one abstract method.
//Declaration of the abstract class by using abstract keyword abstract class ExampleOfAbstractClass { //This is abstract method because it hasn’t body. abstract void sound(); }
abstract class Course { public void courseDetail() { System.out.println("Course name: MCA"); System.out.println("Course Duration : 2020 - 2023"); } public abstract void studentData(); } class StudentRecord extends Course { @Override public void studentData() { Student student = new Student(1, "Ram", "MCA"); System.out.println("RollNo: "+student.getRollNo()); System.out.println("Name: "+student.getName()); System.out.println("Class Name: "+student.getClassName()); } } class HostelRecord extends Course { @Override public void studentData() { Student student = new Student(2, "Krishan", "MCA"); System.out.println("RollNo: "+student.getRollNo()); System.out.println("Name: "+student.getName()); System.out.println("Class Name: "+student.getClassName()); } } public class MainClass { public static void main(String arg[]) { StudentRecord studentRecord = new StudentRecord(); studentRecord.courseDetail(); studentRecord.studentData(); HostelRecord hostelRecord = new HostelRecord(); hostelRecord.courseDetail(); hostelRecord.studentData(); } } class Student { int rollNo; String name; String className; public Student(int rollNo, String name, String className) { this.rollNo = rollNo; this.name = name; this.className = className; } public int getRollNo() { return rollNo; } public void setRollNo(int rollNo) { this.rollNo = rollNo; } public String getName() { return name; } public void setName(String name) { this.name = name; } public String getClassName() { return className; } public void setClassName(String className) { this.className = className; } }
Output: Course name: MCA
Course Duration : 2020 – 2023
RollNo: 1
Name: Ram
Class Name: MCA
Course name: MCA
Course Duration : 2020 – 2023
RollNo: 2
Name: Krishan
Class Name: MCA
Why can’t we create the object of an abstract class?
Abstract classes are not complete because they have abstract methods and abstract methods don’t have a body. If java allows the creation of an object of the abstract class, then it will lead to two problems:
1. The first problem occurs because the abstract method has nobody. If someone calls the
abstract method using that object, then What would happen?
2. Abstract classes are only like a template, to create an object of class the class should be
concrete. because an object is a concrete.
How to use abstract classes and abstract methods in Real life?
Let’s say, I am the principal of a college and I want details of each department of the college. My college has various departments like Art, science, commerce, etc. I want to know the department name, how many teachers are in each department(mandatory information), and some additional information. So, I will create a structure that will be followed by each department. I will create an abstract class with an abstract method that will be inherited by each department. So each department must provide the implementation of the abstract method.

Here we have three classes one class is abstract (Department) and the other two are concrete classes(Art class and science class). These concrete classes inherit the abstract class so they must provide the implementation of abstract methods.
abstract class Department { String collegeName = "KUK"; public void collegeName() { System.out.println("Name of college = "+ collegeName); } //abstract methods public abstract void deptName(); public abstract void noOfTeachers(); } class ArtDepartment extends Department { public void showData() { System.out.println("This is Art department's data"); } @Override public void deptName() { System.out.println("Department name is Art"); } @Override public void noOfTeachers() { System.out.println("Number of teacher are 10"); } } class ScienceDepartment extends Department { public void getStudentsData() { System.out.println("This data is belongs to science students"); } @Override public void deptName() { System.out.println("Department name is Science"); } @Override public void noOfTeachers() { System.out.println("Number of teacher are 20"); } } class MainClass { public static void main(String arg[]) { // Displaying information of different departments ArtDepartment artDepartment = new ArtDepartment(); artDepartment.showData(); artDepartment.deptName(); artDepartment.noOfTeachers(); artDepartment.collegeName(); System.out.println(""); ScienceDepartment scienceDepartment = new ScienceDepartment(); scienceDepartment.getStudentsData(); scienceDepartment.deptName(); scienceDepartment.noOfTeachers(); scienceDepartment.collegeName(); } }
Output:
This is Art department’s data
Department name is Art
Number of teacher are 10
Name of college = KUK
This data is belongs to science students
Department name is Science
Number of teacher are 20
Name of college = KUK
When to use the abstract class
Every programmer knows abstract class in java is used to achieve abstraction in java. But only a few of them know when to use abstract class in java? You can’t use such things in your program if you don’t know when we should use them?
Suppose we want to maintain the data of a Company. A company has different types of employees in different departments like Software developer, IT, and Management. Each employee has different personal information and salary. So, we need to create a class Company and some subclasses that inherit the method of the Company class.
Firstly, we will discuss the problem area. After that we will find the solution with abstract class.

Let’s say we have a class Company that has two methods companyDetail() and employeeDetail(). The companyDetail() method contains information about the company that is common for all employees and employeeDetails() method contains information about employees which differs for every employee. We have three subclasses for employees that are Developer, Manager and IT. Since each employee has different personal information and salary. So, every subclass overrides the employeeDetails() method to give the details of its own implementation. Here you must have noticed, the employeeDetails() method in Company class (Parent class) is not creating any value because every child class overrides the method.
class Company { public void companyDetail() { System.out.println("Company name : Final Rope"); System.out.println("Company GST : 123xxxxxxxxxx"); } public void employeeDetail() { System.out.println("Employee name : Ram"); System.out.println("Employee ID : 101"); System.out.println("Employee Salary : XXXXX"); } } class Developer extends Company { public void employeeDetail() { System.out.println("Employee name : Sham"); System.out.println("Employee ID : 201"); System.out.println("Employee Salary : XXXXX"); } } class Manager extends Company { public void employeeDetail() { System.out.println("Employee name : Krishan"); System.out.println("Employee ID : 301"); System.out.println("Employee Salary : XXXXX"); } } class IT extends Company { public void employeeDetail() { System.out.println("Employee name : John"); System.out.println("Employee ID : 401"); System.out.println("Employee Salary : XXXXX"); } } public class MainClass { public static void main(String arg[]) { Developer developer = new Developer(); developer.companyDetail(); developer.employeeDetail(); Manager manager = new Manager(); manager.companyDetail(); manager.employeeDetail(); IT it = new IT(); it.companyDetail(); it.employeeDetail(); } }
Output: Company name : Final Rope
Company GST : 123xxxxxxxxxx
Employee name : Sham
Employee ID : 201
Employee Salary : XXXXX
Company name : Final Rope
Company GST : 123xxxxxxxxxx
Employee name : Krishan
Employee ID : 301
Employee Salary : XXXXX
Company name : Final Rope
Company GST : 123xxxxxxxxxx
Employee name : John
Employee ID : 401
Employee Salary : XXXXX
There is no point to implement the employeeDetails() method in Company class. Hence, we should make the employeeDetails() method an abstract method. By making this method abstract there is no need to give any implementation in parent class. The abstract method(employeeDetails() method) forces all the sub-classes to implement this method. If any sub-class will not provide the implementation to this abstract method, then it will show a compilation error.

abstract class Company { public void companyDetail() { System.out.println("Company name : Final Rope"); System.out.println("Company GST : 123xxxxxxxxxx"); } public abstract void employeeDetail(); } class Developer extends Company { public void employeeDetail() { System.out.println("Employee name : Sham"); System.out.println("Employee ID : 201"); System.out.println("Employee Salary : XXXXX"); } } class Manager extends Company { public void employeeDetail() { System.out.println("Employee name : Krishan"); System.out.println("Employee ID : 301"); System.out.println("Employee Salary : XXXXX"); } } class IT extends Company { public void employeeDetail() { System.out.println("Employee name : John"); System.out.println("Employee ID : 401"); System.out.println("Employee Salary : XXXXX"); } } public class MainClass { public static void main(String arg[]) { Developer developer = new Developer(); developer.companyDetail(); developer.employeeDetail(); Manager manager = new Manager(); manager.companyDetail(); manager.employeeDetail(); IT it = new IT(); it.companyDetail(); it.employeeDetail(); } }
Output: Company name : Final Rope
Company GST : 123xxxxxxxxxx
Employee name : Sham
Employee ID : 201
Employee Salary : XXXXX
Company name : Final Rope
Company GST : 123xxxxxxxxxx
Employee name : Krishan
Employee ID : 301
Employee Salary : XXXXX
Company name : Final Rope
Company GST : 123xxxxxxxxxx
Employee name : John
Employee ID : 401
Employee Salary : XXXXX
As we have seen above example in detail. Now we can predicate the scenarios and think in a better way and discuss when to use abstract class in java?
Can an abstract class be final in java?
No, An abstract class can’t be final because the final and abstract are opposite terms in JAVA.
Reason: An abstract class must be inherited by any derived class because a derived class is responsible to provide the implementation of abstract methods of an abstract class. But on another hand, if a class is a final class, then it can’t be extended(inherited). So both concepts are opposite to each other.
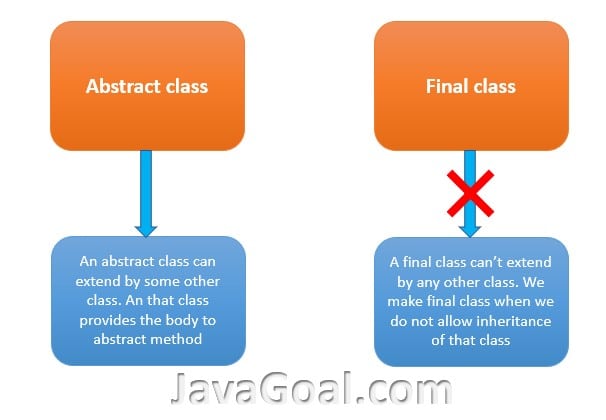
abstract final class Department { String collegeName = "KUK"; public void collegeName() { System.out.println("Name of college = "+ collegeName); } //abstract methods public abstract void deptName(); public abstract void noOfTeachers(); } class MainClass extends Department { public static void main(String arg[]) { MainClass obj = new MainClass(); } @Override public void deptName() { // TODO Auto-generated method stub } @Override public void noOfTeachers() { // TODO Auto-generated method stub } }
Output: This will show exception at compilation time.
As you can see in the above example, We are trying to use two opposite keywords (abstract and final) for a single class. In this case, the compiler shows an error compile-time, because Java doesn’t permit it.
Because if the compiler works according to the final keyword then the Department class must not be extended by any other class. But the abstract keyword is vice-versa. It means according to abstract keyword the Department must be extended by some other class. To remove this ambiguity of compiler, Java doesn’t permit it.
Can we create an instance of an abstract class?
No, we can’t create an object of it. As know abstract class is not a complete class. because an abstract class has abstract method (Methods without body). Although an abstract class has a constructor if you will try to create an object of it, It will throw compile time exception.
abstract class ExampleOfAbstractClass { public abstract void showData(); } public class MainClass { public static void main(String arg[]) { ExampleOfAbstractClass object = new ExampleOfAbstractClass(); } }
Exception in thread “main” java.lang.Error: Unresolved compilation problem: Cannot instantiate the type ExampleOfAbstractClass at create.MainClass.main(MainClass.java:13)
You have seen in the above example the compiler throws the exception at compile time. The exception message is not directly saying that you can’t create an object it. It just says “Cannot Instantiate The Type ExampleOfAbstractClass”, which means that you cannot create an object of it.
You must think about the constructor of the abstract class. If we can’t create the object of it then what is the use of a constructor in the abstract class? The constructor of the abstract class is to initialize the fields which belong to the abstract class. But this constructor is invoked from a concrete subclass as part of creating an instance of a concrete subclass in Java.
Why we can’t create an object abstract class?
If java allows creating an object of it, then it will lead to two problems:
1. The first problem occurs because the abstract method doesn’t provide the body of the method. They are just declared in the abstract class. If someone calls the abstract method using that object, then What would happen?
2. Abstract classes are only like a template, to create an object of any class, the class should be concrete because an object is concrete.
Can an abstract class have a constructor?
An abstract class can have abstract methods and we can’t create its object. You can read “Why we can’t create an object of the abstract class?” But here a question strike in mind, Can abstract class have a constructor? If yes, then why and what is the use of constructor in abstract even we can’t create the object of an abstract class. Here we will discuss it in detail.
Yes, an abstract class can have a constructor in Java. The compiler automatically adds the default constructor in every class either it is an abstract class or concrete class. You can also provide a constructor to abstract class explicitly. The constructor is always used to initiate a class or use it to create an object of the class. But we can’t instantiate it with the new() operator or any other ways.
Why can an abstract class have constructors in Java?
To support the chaining of the constructor
By default, JVM is dealing with the constructor chaining because if you are creating an object by a new keyword then JVM invokes the constructor of that class and that constructor invokes the superclass constructor. If you want to know how constructor chaining works, then read the article.
To use an abstract class in Java, we use the concrete class that extends the abstract class and provides the implementation to the abstract methods. When we use the constructor of the child class, the constructor of the parent class invoked by child class constructor either implicitly or explicitly. This is one of the reasons abstract class can have constructors in Java. Suppose if JVM doesn’t provide the default constructor to an abstract class then JVM not able to support constructor chaining.
To initialize the fields of abstract class
As you know a constructor is used to initialize the fields of the class. Suppose we want to set some common behavior for all the child classes and some behavior depends upon the subclass implementation. Then we can use the constructor of the abstractor class to initialize the fields.
Let’s suppose we have an abstract class Car that has some common properties. These common properties will be common for each subclass that extends the Car class. We can initialize these fields in the Constructor of abstract class so, when you instantiate the constructor of child classes then the parent constructor will be called, and the fields will be initialized.
abstract class Car { String model; String brand; public abstract void addFeatures(); Car() { this.model = "X5"; this.brand = "BMW"; } } class SportCar extends Car { boolean nitro; @Override public void addFeatures() { this.nitro = true; } public void showDetail() { System.out.println("Model :"+ model); System.out.println("Brand :"+ brand); System.out.println("Nitro :"+ nitro); } } public class MainClass { public static void main(String arg[]) { SportCar sportCar = new SportCar(); sportCar.addFeatures(); sportCar.showDetail(); } }
Output: Model :X5
Brand :BMW
Nitro :true
How to create an Abstract class in java? –> For this example – variables access modifier should be private right ? without declaring access modifier as private you are setting and getting the values of the variables? Is that correct? Please response
class Student
{
int rollNo;
String name;
String className;
public Student(int rollNo, String name, String className) {
this.rollNo = rollNo;
this.name = name;
this.className = className;
}
public int getRollNo() {
return rollNo;
}
public void setRollNo(int rollNo) {
this.rollNo = rollNo;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getClassName() {
return className;
}
public void setClassName(String className) {
this.className = className;
}
}
How to use abstract class and abstract methods in Real life?
in the below example – you’re trying to print the college name but in the program example, college name is not printed in the console. Can you please check this program
abstract class Department
{
String collegeName = “KUK”;
public void collegeName()
{
System.out.println(“Name of college = “+ collegeName);
}
Because collegeName() of the class Department was never called. Although this method is inherited, it should be called to print the content on the console. Hope this helps.
Yes lla, you are right. I have made the changes.
Please verify it again Megha 🙂