String in Java is the most popular and frequently used data type. String concatenation is the most common and widely used operation in java. In java string concatenation is the process that is used to join two or more String and produce a new String. We can join strings in java in various ways.
In this post, we will read some ways to join string in java.
String concatenation in java means joining the strings and making a combined string. In java, we can concatenate strings in multiple ways like the concatenation operator (+), java string concat() method, StringBuffer, and StringBuilder, join() method etc
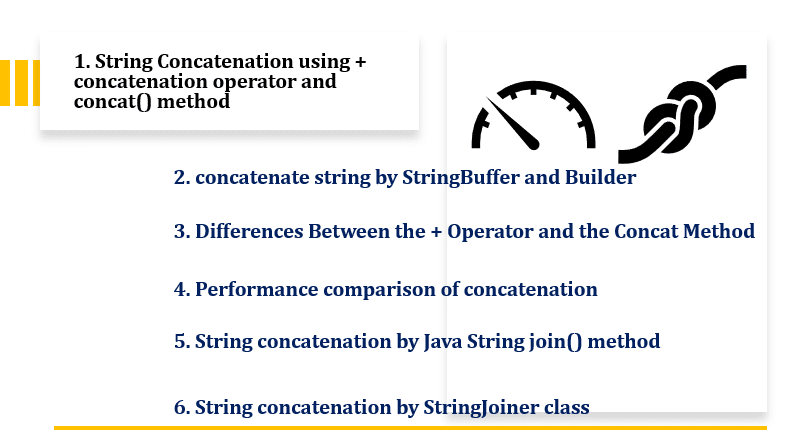
Here is the table content of the article will we will cover this topic.
1. String Concatenation using + concatenation operator
2. java string concatenation using concat() method in java
i) java string concat() method
ii) What is concat() method in java?
3. concatenate string by StringBuffer and Builder
4. Differences Between the + Operator and the Concat Method
5. Performance comparison of concatenation
6. String concatenation by Java String join() method
7. String concatenation by StringJoiner class
1. String Concatenation using + concatenation operator
By use of the + operator, we can concatenate any number of Strings. It always appends the specified string at the end of the given string. It is the most common way to concatenate the strings in Java.
String str = "Java" + "Goal" ; String s1 = "Java"; String s2 = "Goal"; String s3 = s1 + s2; String strMsg = "Java" + "Goal" + ".com";
By use of + operator, we can append the primitive types of data with String. The JVM uses the string representation of primitive data and concatenates it to a String and produces a new String.
String str = "Hello" + 5; String str = 123 + "Hi";
We can also concatenate the object with String, But whenever we use + operator to concatenate with an object, The JVM calls the toString() method of the object and get the string representation. Then both the strings are concatenated.
class Student { int rollNo; String name; } public class Data { public static void main(String[] args) { Student obj = new Student(); String str = "JavaGoal"; String s = str + obj; System.out.println(s); } }
Output: JavaGoalStudent@3b22cdd0
Here you can see the JVM invoking the toString() method of the Student class that was inherited in from the Object class. You can override the toString() method and return the string accordingly.
class Student { int rollNo = 1; String name = ".com"; @Override public String toString() { return name; } } public class Data { public static void main(String[] args) { Student obj = new Student(); String str = "JavaGoal"; String s = str + obj; System.out.println(s); } }
Output: JavaGoal.com
The concatenation operator + can be used only with an immutable string and it produces the immutable string after concatenation.
2. java string concatenation using concat() method in java
java string concat() method
The concat() method in java is present in the String class. It is another way to concatenate two strings in java. In Java string concat() method is used to concatenate multiple strings. This method appends the specified string at the end of the given string and returns the combined string. But if the length of the specified string is 0, then it returns the given String.
What is concat() method in java?
The concat() method is always used to concatenate multiple strings. We can call the concat() method by string object that we want to concatenate with the specified string in the parameter.
public String concat (String str)
str: String that is concatenated to the end of this String.
return: It returns a combined string.
class ExampleOfConcat { public static void main(String args[]) { String str1 = "Java"; String str2 = "Goal"; System.out.println("After concatenation : "+ str1.concat(str2)); String s1 = "Hi"; String s2 = "Hello"; String s3 = "Bye"; System.out.println("After concatenation : "+ s1.concat(s2).concat(s3)); } }
Output: After concatenation : JavaGoal
After concatenation : HiHelloBye
3. concatenate string by StringBuffer and Builder
We have seen we can concatenate string by use of the + operator and concatenate. We can concatenate strings by use of the StringBuffer class. Whenever we want to concatenate a large number of strings, StringBuilder and StringBuffer are good choices. Actually, it is considered a proper way to concatenate strings.
StringBuffer and StringBuilder always return the mutable string. Actually, StringBuffer provides some methods to concatenate the strings that are must faster than the + operator and concat() method.
StringBuffer and StringBuilder classes provide the append() method to concatenate strings but all append methods of the StringBuffer class are synchronized. It also provides several overloaded append() methods to concatenate integer, char, short, etc.
class ExampleOfAppendMethod { public static void main(String args[]) { StringBuffer str1 = new StringBuffer("Hi!!!!!"); str1.append(" It's JavaGoal.com"); System.out.println(str1); StringBuffer str2 = new StringBuffer("A learning website"); String s = " For Java"; str2.append(s); System.out.println(str2); } }
Output: Hi!!!!! It’s JavaGoal.com
A learning website For Java
class ExampleOfAppendMethod { public static void main(String args[]) { StringBuilder str1 = new StringBuilder("Hi!!!!!"); str1.append(" It's JavaGoal.com"); System.out.println(str1); StringBuilder str2 = new StringBuilder("A learning website"); String s = " For Java"; str2.append(s); System.out.println(str2); } }
Output: Hi!!!!! It’s JavaGoal.com
A learning website For Java
4. Differences Between the + Operator and the Concat Method
You have seen the concatenation of String by + operator and concat() method. But there are some differences that will help to understand when it makes sense to use the + operator to concatenate, and when you should use the concat() method.
Here are some differences between the two:
- The concat() method concatenates only immutable String objects and it must be called on immutable String object and its parameter must be an immutable String object.
But the + operator can concatenate any non-string object like a Primitive, object. It converts the non-string object to a String and then appends it.
class ExampleOfString { public static void main(String args[]) { String str1 = "Java"; String str2 = "Goal"; System.out.println(str1.concat(str2)); String str3 = "Hi"; System.out.println(str3 + 123); } }
Output: JavaGoal
Hi123
- As we said the concat() method concatenates only the string objects, if an object has a null reference it will throw NullPointerException. But the + operator concatenates the null reference as a “null” string.
class ExampleOfString { public static void main(String args[]) { String str1 = "Java"; String str2 = null; System.out.println("By + operator: "+(str1+str2)); System.out.println("By concat(): "+str1.concat(str2)); } }
Output: By + operator: Javanull
Exception in thread “main” java.lang.NullPointerException at java.base/java.lang.String.concat(String.java:1937) at ExampleOfString.main(ExampleOfString.java:9)
5. Performance comparison of concatenation
public class ConcatenationPerformance { public static void main(String args[]) { final int ITERATION = 10000; // String Concatenation using + operator String stringbyOperator = ""; long startTime = System.nanoTime(); for (int i = 0; i < ITERATION; i++) { stringbyOperator = stringbyOperator + Integer.toString(i); } long duration = (System.nanoTime() - startTime) / 1000; System.out.println("Time taken to concatenate by + operator : " + duration); // Using String concat() method startTime = System.nanoTime(); for (int i = 0; i < ITERATION; i++) { stringbyOperator.concat(Integer.toString(i)); } duration = (System.nanoTime() - startTime) / 1000; System.out.println("Time taken to concatenate by concat method: " + duration); // StringBuffer example to concate String in Java StringBuffer buffer = new StringBuffer(); startTime = System.nanoTime(); for (int i = 0; i < ITERATION; i++) { buffer.append(i); } duration = (System.nanoTime() - startTime) / 1000; System.out.println("Time taken to concatenate by StringBuffer: " + duration); // StringBuilder example to concate two String in Java StringBuilder builder = new StringBuilder(); startTime = System.nanoTime(); for (int i = 0; i < ITERATION; i++) { builder.append(i); } duration = (System.nanoTime() - startTime) / 1000; System.out.println("Time taken to concatenate by StringBuilder: " + duration); } }
Output: Time taken to concatenate by + operator : 132484
Time taken to concatenate by concat method: 78762
Time taken to concatenate by StringBuffer: 1167
Time taken to concatenate by StringBuilder: 570
6. String concatenation by Java String join() method
Since java 8 we can use the join() method to concatenate the String in java. But the join method works differently than other concatenation ways. We can join an array of Strings with a common delimiter, ensuring no spaces are missed.
public class ExampleJoinMethod { public static void main(String args[]) { String[] strings = {"Java", "Goal", "website", "for", "Java", "Learning"}; String joinedString = String.join(" ", strings); System.out.println(joinedString); } }
Output: Java Goal website for Java Learning
7. String concatenation by StringJoiner class
In java 8, a new class was introduced that was StringJoiner. The StringJoiner class can concatenate different strings. The constructor takes a delimiter, with an optional prefix and suffix. We can append Strings using the well-named add method.
import java.util.StringJoiner; public class ExampleJoinMethod { public static void main(String args[]) { StringJoiner joinerString = new StringJoiner(" "); joinerString.add("Java"); joinerString.add("Goal"); joinerString.add("Website"); System.out.println(joinerString); StringJoiner joinerString2 = new StringJoiner(", "); joinerString2.add("Java"); joinerString2.add("Goal"); joinerString2.add("Website"); System.out.println(joinerString2); } }
Output: Java Goal Website
Java, Goal, Website
I really like your writing style, excellent info,
thanks for putting up :D.
Some really superb info, Gladiola I observed this.
It is not my first time to visit this web site, i am browsing this
web site dailly and get nice data from here everyday.
Fantastic site. Lots of useful information here. I’m sending it to several friends ans also sharing in delicious.
And obviously, thank you for your sweat!
I got this website from my buddy who told me concerning this web page
and now this time I am browsing this site and reading very informative content at this time.