We have read how does put() method work and by using the put() method we can replace the existing object of HashMap. In this post, we will see another way to replace the object of Hashmap by use of java hashmap replace.
Here is the table content of the article will we will cover this topic.
replace(K key, V value) method
replace(K key, V oldValue, V newValue) method
replace(K key, V value) method
This method is used to replace the entry for the specified key only if it is currently mapped to some value. It throws NullPointerException if the given key or value is null. Let’s see the java hashmap replace the example
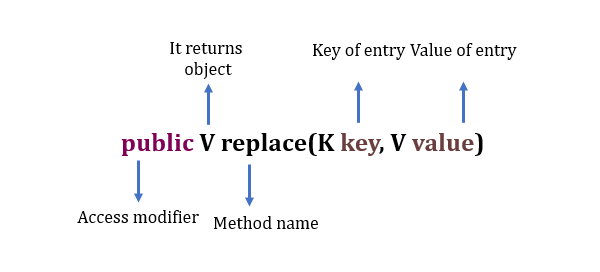
Where, Key is the key with which the specified value(V) is to be associated in HashMap.
Value is the value to be associated with the specified key(K) in HashMap.
import java.util.HashMap; public class ExampleOfHashMap { public static void main(String[] args) { HashMap<Integer, String> hashMap = new HashMap<Integer, String>(); // Adding objects in HashMap hashMap.put(1, "JavaGoal.com"); hashMap.put(2, "Learning"); hashMap.put(3, "Website"); // Before replacing entry System.out.println("Before replacing HashMap: "+ hashMap); hashMap.replace(2, "A learning"); // After replacing entry System.out.println("After replacing HashMap: "+ hashMap); } }
Output: Before replacing HashMap: {1=JavaGoal.com, 2=Learning, 3=Website}
After replacing HashMap: {1=JavaGoal.com, 2=A learning, 3=Website}
replace(K key, V oldValue, V newValue) method
This method is used to replace the entry for the specified key only if it is currently mapped to some value. If oldValue doesn’t match with the associated key, then it does not replace the value. Its return type is boolean.

Where Key is the key with which the specified value(V) is to be associated with HashMap.
oldValue is the value to be associated with the specified key(K) in HashMap.
newValue is the value that will be associate with the specified key(K) in HashMap.
import java.util.HashMap; public class ExampleOfHashMap { public static void main(String[] args) { HashMap<Integer, String> hashMap = new HashMap<Integer, String>(); // Adding objects in HashMap hashMap.put(1, "JavaGoal.com"); hashMap.put(2, "Learning"); hashMap.put(3, "Website"); // Before replacing entry System.out.println("Before replacing HashMap: "+ hashMap); hashMap.replace(2, "Learning", "A Learning"); // After replacing entry System.out.println("After replacing HashMap: "+ hashMap); } }
Output: Before replacing HashMap: {1=JavaGoal.com, 2=Learning, 3=Website}
After replacing HashMap: {1=JavaGoal.com, 2=A Learning, 3=Website}