We have read how to create and initialize the ArrayList in java. The ArrayList class provides a lot of Java ArrayList methods to operate the data of ArrayList. In this post, we will cover all the methods of ArrayList in java, and let’s see the java ArrayList methods with example.
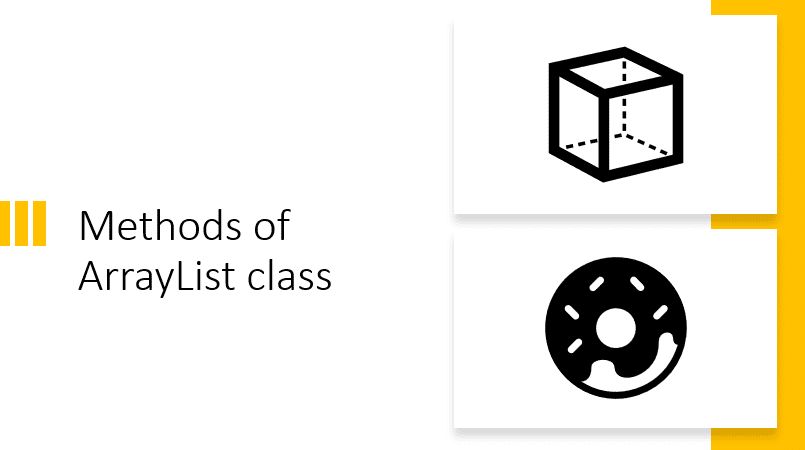
How to add elements to an ArrayList?
1. add(E e): The add(E e) method is used to add the specified element e in the ArrayList. You can read it with an example from here.
2. add(int index, E e): The add(int index, E e) method is used to add the specified element at a specific position in the ArrayList. You can read it with an example from here.
3. addAll(Collection c): The addAll(Collection c) is used to add the collection of elements to the end of the ArrayList. You can read it with an example from here.
4. addAll(int index, Collection c): The addAll(int index, Collection c) is used to add the collection of elements at specified position in the ArrayList. You can read it with an example from here.
How to remove elements from an ArrayList?
5. remove(Object o): The remove(Object o) method is used to remove the first occurrence of the element from the ArrayList. You can read it with an example from here.
6. remove(int index): The remove(int index) method is used to remove the element at the specified index in the ArrayList. You can read it with an example from here.
7. remove(Collection c): The remove(Collection c) method is used to remove all the elements of the specified collection from the ArrayList. You can read it with an example from here.
8. removeIf(Predicate filter): The removeIf(Predicate filter) method is used to remove all elements from the ArrayList based on the predicate’s condition. You can read it with an example from here.
How to access elements from an ArrayList?
9. get(int index): The get(int index) method is used to get the element at the specified index in the ArrayList. You can read it with an example from here.
10. iterator(): The iterator() method is used to return an iterator over the elements in the ArrayList. You can read it with an example from here.
How to get index of object in ArrayList java?
11. index(object o): The index(object o) method is used to get the index of the specified object. You can read it with an example from here.
12. lastIndexOf(Object o): The lastIndexOf(Object o) method is used to get the last index of the specified object. You can read it with an example from here.
How to replace element in an ArrayList?
13. set(int index, E element): The set(int index, E element) method is used to replace the element at a particular index in the ArrayList with the specified element. You can read it with an example from here.
How to Iterate an ArrayList
14. iterator(): The iterator() method is used to iterate the elements of an ArrayList. You can read it with an example from here.
15. spliterator(): The spliterator() method returns an object Spliterator. You can read it with an example from here.
16. listIterator(): The listIterator() method is used to return a list iterator over the elements in the ArrayList. You can read it with an example from here.
17. listIterator(int index): The listIterator(int index) method is used to return a list iterator over the elements in ArrayList starting at the specified position in the ArrayList. You can read it with an example from here.
How to Sort an ArrayList
18. sort(Comparator c): By use of ArrayList.sort(Comparator c), we can sort the ArrayList. It takes only one argument that is a reference of Comparator. You can read it with an example from here.
How to get subList from ArrayList
19. subList(int fromIndex, int toIndex) method: The subList() method returns a portion from the ArrayList that start from fromIndex and ends to toIndex. You can read it with an example from here.
How to Convert list to array in java
20. toArray() method: This method is used to convert ArrayList to Array. You can read it with an example from here.
21. toArray(T[] a) method : It is also used to convert the list to array but it takes parameter of array type. You can read it with an example from here.
Some other methods of ArrayList
22. ensureCapacity(int minCapacity)
The ensureCapacity(int minCapacity) method is used to increase the capacity of ArrayList. It ensures that it can hold at least the number of elements specified by the minimum capacity argument. Its return type is boolean void.
ensureCapacity(int minCapacity):
minCapacity , this method takes the desired minimum capacity as a parameter.
import java.util.ArrayList; public class ArraylistExample { public static void main(String[] args) { ArrayList<String> listOfNames = new ArrayList<String>(); listOfNames.add("JAVA"); listOfNames.add("GOAL"); listOfNames.add("WebSite"); // ensure that the ArrayList can hold upto 5000 elements using ensureCapacity() method listOfNames.ensureCapacity(500); System.out.println("ArrayList can now surely store upto 500 elements."); } }
Output: ArrayList can now surely store upto 500 elements.
23. replaceAll(UnaryOperator operator) method
The replaceAll(UnaryOperator<E> operator) was introduced in Java 8. It is used to replace all the elements from the list with the specified element. It takes only one argument of UnaryOperator. This method doesn’t return anything. Its return type is void.
void replaceAll(UnaryOperator<E> operator)
Where the operator is UnaryOperator. The UnaryOperator interface is a functional interface that has a single abstract method named apply that returns a result of the same object type as the operand.
import java.util.ArrayList; public class ArraylistExample { public static void main(String[] args) { ArrayList<String> listOfNames = new ArrayList<String>(); listOfNames.add("JAVA"); listOfNames.add("GOAL"); listOfNames.add("WEBSITE"); listOfNames.replaceAll(name -> (name.toLowerCase())); for(String name : listOfNames) System.out.println("Name from list = "+ name); } }
Output: Output:Name from list = java
Name from list = goal
Name from list = website
24. retainAll(Collection c) method
The retainAll(Collection c) method returns only those elements, which are common in collection and ArrayList. It removed all the elements from ArrayList that are not present in Collection c.
boolean retainAll(Collection<?> c)
Where, c is the collection which you want to retain from the ArrayList.
return type: Its return type is boolean. It can return either true or false.
If specified collection c presents in ArrayList then it returns true after retaining otherwise it returns false.
import java.util.ArrayList; public class ArraylistExample { public static void main(String[] args) { ArrayList<Integer> listOfNames = new ArrayList<Integer>(); listOfNames.add(1); listOfNames.add(2); listOfNames.add(3); listOfNames.add(4); listOfNames.add(5); listOfNames.add(6); ArrayList<Integer> listOfNames2 = new ArrayList<Integer>(); listOfNames2.add(4); listOfNames2.add(5); listOfNames2.add(6); listOfNames.retainAll(listOfNames2); for(Integer number : listOfNames) System.out.println("Name from list = "+ number); } }
Output: Name from list = 4
Name from list = 5
Name from list = 6
25. isEmpty() method
The isEmpty() method is used to whether ArrayList is empty or not. Its return type is boolean. If the size of Arraylist is 0 then it will true otherwise false.
public boolean isEmpty()
import java.util.ArrayList; public class ArrayListExample { public static void main(String[] args) { ArrayList<Integer> listOfNumbers = new ArrayList<Integer>(); listOfNumbers.add(1); listOfNumbers.add(2); listOfNumbers.add(3); listOfNumbers.add(4); listOfNumbers.add(5); System.out.println("Is empty: "+ listOfNumbers.isEmpty()); ArrayList<Integer> listOfNumbers2 = new ArrayList<Integer>(); System.out.println("Is empty: "+ listOfNumbers2.isEmpty()); } }
Output: Is empty: false
Is empty: true
26. clear() method
The clear() method is used to remove all elements from the ArrayList. It doesn’t return anything.
import java.util.ArrayList; public class ArrayListExample { public static void main(String[] args) { ArrayList<Integer> listOfNumbers = new ArrayList<Integer>(); listOfNumbers.add(1); listOfNumbers.add(2); listOfNumbers.add(3); listOfNumbers.add(4); listOfNumbers.add(5); listOfNumbers.clear(); System.out.println("Is empty: "+ listOfNumbers.isEmpty()); } }
Output: Is empty: true
27. contains(Object o)
The contains(Object o) method is used to check the specified element is present in ArrayList or not. It returns a boolean value, If the given element exists in ArrayList, then it returns true otherwise false.
boolean contains(Object obj);
Where, Object represents the type of class in ArrayList.
obj is the element which you want to check in the ArrayList.
return type: Its return type is boolean. It can return either true or false.
import java.util.ArrayList; public class ArrayListExample { public static void main(String[] args) { ArrayList<String> listOfNames = new ArrayList<String>(); listOfNames.add("JAVA"); listOfNames.add("GOAL"); listOfNames.add("Learning"); listOfNames.add("SITE"); // Return, true because RAVI is exist System.out.println("Is 'GOAL' present: "+listOfNames.contains("GOAL")); // Return, true because RAVI KANT doesn't exist System.out.println("Is 'LEARNING' present: "+listOfNames.contains("LEARNING")); } }
Output: Is ‘GOAL’ present: true
Is ‘LEARNING’ present: false
28. trimToSize() method
This trimToSize() method is used to trim the capacity of the instance of the ArrayList to the list’s current size.
void trimToSize();
It doesn’t return anything, so its return type is void.
import java.util.ArrayList; public class ArrayListExample { public static void main(String arg[]) { ArrayList<String> listOfNames = new ArrayList<String>(20); listOfNames.add("A"); listOfNames.add("B"); listOfNames.add("C"); listOfNames.add("D"); listOfNames.trimToSize(); System.out.println(listOfNames); } }
Output: [A, B, C, D]
29. clone() method
The clone() method of ArrayList class is used to return a shallow copy of the specified ArrayList. If you are not familiar with Cloning in java please read it first
Object clone();
Its return type is Object. It returns the object of Object class.
import java.util.ArrayList; public class ArraylistExample { public static void main(String[] args) { ArrayList<String> listOfNames = new ArrayList<String>(); listOfNames.add("JAVA"); listOfNames.add("GOAL"); listOfNames.add("RAVI"); for(String name : listOfNames) System.out.println("Names from list = "+name); ArrayList<String> cloneCopy = (ArrayList<String>) listOfNames.clone(); for(String name : cloneCopy) System.out.println("Names from clones list = "+name); } }
Output: Names from list = JAVA
Names from list = GOAL
Names from list = RAVI
Names from clones list = JAVA
Names from clones list = GOAL
Names from clones list = RAVI
We have covered all the Java ArrayList methods in this post. If you have any doubt or question please comment on comment section. We will help you.