Like other collections, we can’t directly traverse map in java. To iterate hashmap in java we can use the HashMap iterator() method and java map foreach() method. In this post, we will see how to Iterate a HashMap in Java.
As we already know HashMap stores data in form of entry and each entry is a pair of keys and value. But we can’t directly iterate the objects of HashMap, unlike HashSet. Here we have some methods that are used to get the objects from HashMap. Before moving further we should know about the keySet() method, values() method, and entrySet() method.
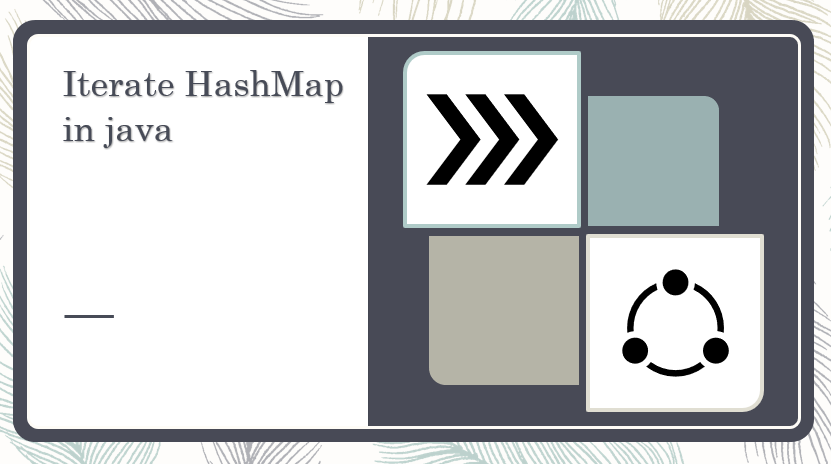
iterate map in java
We can iterate a hashmap in java by use of its keys, values, and entries. Now it will depend on the scenario which method you should use:
1. If you want to get each value based on the key then you should iterate based on keys(By keySet())
2. If you just want to use values of HashMap and you don’t care about any order then use-values. (By values)
3. If you want to key and values at the same time then use entire(By entrySet())
By use of the iterator() method iterate through hashmap
Let’s see the example, Here we will see how we can iterator by use of keySet() method.
import java.util.HashMap; import java.util.Iterator; public class HashMapExample { public static void main(String args[]) { HashMap<Integer, String> hashMap = new HashMap<Integer, String>(); hashMap.put(1, "JavaGoal.com"); hashMap.put(2, "Learning"); hashMap.put(3, "Website"); Iterator<Integer> hashMapKey = hashMap.keySet().iterator(); while(hashMapKey.hasNext()) { Integer key = hashMapKey.next(); System.out.println("Key: "+ key); System.out.println("Value from Key: "+ hashMap.get(key)); } } }
Output: Key: 1
Value from Key: JavaGoal.com
Key: 2
Value from Key: Learning
Key: 3
Value from Key: Website
Let’s see the another example, Here we will see how we can iterator by use of values() method.
import java.util.Collection; import java.util.HashMap; import java.util.Iterator; public class HashMapExample { public static void main(String args[]) { HashMap<Integer, String> hashMap = new HashMap<Integer, String>(); hashMap.put(1, "JavaGoal.com"); hashMap.put(2, "Learning"); hashMap.put(3, "Website"); Collection<String> hashMapValues = hashMap.values(); Iterator<String> values = hashMapValues.iterator(); while(values.hasNext()) { System.out.println("Value from HashMap: "+ values.next()); } } }
Output:Value from HashMap: JavaGoal.com
Value from HashMap: Learning
Value from HashMap: Website
Let’s see the another example, Here we will see how we can iterator by use of entrySet() method.
import java.util.Collection; import java.util.HashMap; import java.util.Iterator; import java.util.Map.Entry; public class HashMapExample { public static void main(String args[]) { HashMap<Integer, String> hashMap = new HashMap<Integer, String>(); hashMap.put(1, "JavaGoal.com"); hashMap.put(2, "Learning"); hashMap.put(3, "Website"); Collection<Entry<Integer, String>> allEntrySet = hashMap.entrySet(); Iterator<Entry<Integer, String>> entriesIterator = allEntrySet.iterator(); while(entriesIterator.hasNext()) { System.out.println("Entry from HashMap: "+entriesIterator.next()); } } }
Output: Entry from HashMap: 1=JavaGoal.com
Entry from HashMap: 2=Learning
Entry from HashMap: 3=Website
Java map foreach
We can transverse a HashMap in java by the use of java hashmap foreach() method. Here we will discuss the same ways to iterate the HashMap by forEach() method.
import java.util.HashMap; public class HashMapExample { public static void main(String args[]) { HashMap<Integer, String> hashMap = new HashMap<Integer, String>(); hashMap.put(1, "JavaGoal.com"); hashMap.put(2, "Learning"); hashMap.put(3, "Website"); for(Integer key : hashMap.keySet()) { System.out.println("Key: "+ key); System.out.println("Value from Key: "+ hashMap.get(key)); } } }
Output: Key: 1
Value from Key: JavaGoal.com
Key: 2
Value from Key: Learning
Key: 3
Value from Key: Website
import java.util.HashMap; public class HashMapExample { public static void main(String args[]) { HashMap<Integer, String> hashMap = new HashMap<Integer, String>(); hashMap.put(1, "JavaGoal.com"); hashMap.put(2, "Learning"); hashMap.put(3, "Website"); for(String value : hashMap.values()) { System.out.println("Value from HashMap: "+ value); } } }
Output: Value from HashMap: JavaGoal.com
Value from HashMap: Learning
Value from HashMap: Website
import java.util.HashMap; import java.util.Map.Entry; public class HashMapExample { public static void main(String args[]) { HashMap<Integer, String> hashMap = new HashMap<Integer, String>(); hashMap.put(1, "JavaGoal.com"); hashMap.put(2, "Learning"); hashMap.put(3, "Website"); for(Entry<Integer, String> entry : hashMap.entrySet()) { System.out.println("Key and Value from HashMap: "+ entry); } } }
Output: Key and Value from HashMap: 1=JavaGoal.com
Key and Value from HashMap: 2=Learning
Key and Value from HashMap: 3=Website