In a recent topic, we have seen what is an immutable string in java and Why string is immutable in java? If you are a beginner, you must read the immutable string first. In this post, we will see what is a mutable string in java and how to create a mutable string.
Here is the table content of the article will we will cover this topic.
1. What is a mutable string in java?
2. How to create a mutable string in java?
3. How does mutable string work in memory?
What is a mutable string in java?
Immutable means unchanging over time or unable to be changed. Whenever we create a string object of the String class, it is by default created immutable in nature. If we change the value of the string, the JVM creates a new object.
Mutable means changing over time or that can be changed. In a mutable string, we can change the value of the string and JVM doesn’t create a new object. In a mutable string, we can change the value of the string in the same object.
To create a mutable string in java, Java has two classes StringBuffer and StringBuilder where the String class is used for the immutable string.
How to create a mutable string in java?
To create a mutable string, we can use StringBuffer and StringBuilder class. Both classes create a mutable object of string but which one we should use totally depends on the scenario.
Suppose you want to work in a multithreading environment and the string should be thread-safe then you should use the StringBuffer class. On the other hand, if you don’t want a multithreading environment then you can use StringBuilder is not.
But when you consider performance first then StringBuilder is better in terms of performance as compared to StringBuffer.
Let’s see how to create a string by StringBuffer and StringBuilder class.
public class MutableString { public static void main (String[] args) { StringBuffer str1 = new StringBuffer("JavaGoal"); StringBuilder str2 = new StringBuilder("Learning"); System.out.println("Value of str1 before change :" + str1); System.out.println("Value of str2 before change :" + str2); str1.append(".com"); str2.append(" website"); System.out.println("Value of str1 after change :" + str1); System.out.println("Value of str2 after change :" + str2); } }
Output: Value of str1 before change :JavaGoal
Value of str2 before change :Learning
Value of str1 after change :JavaGoal.com
Value of str2 after change :Learning website
In the above example, we are creating two mutable strings one is from StringBuffer and another from StringBuilder. Here we are changing the value of string after creation. We can see we do not need to assign the value to assign in the same object, unlike immutable strings.
How does mutable string work in memory?
In the above example, you have seen how to create a mutable string. Let’s see how it is working in memory.
StringBuffer str1 = new StringBuffer("JavaGoal"); StringBuilder str2 = new StringBuilder("Learning");
After execution of the above line, the JVM will create two objects in memory and return the reference to the variable.
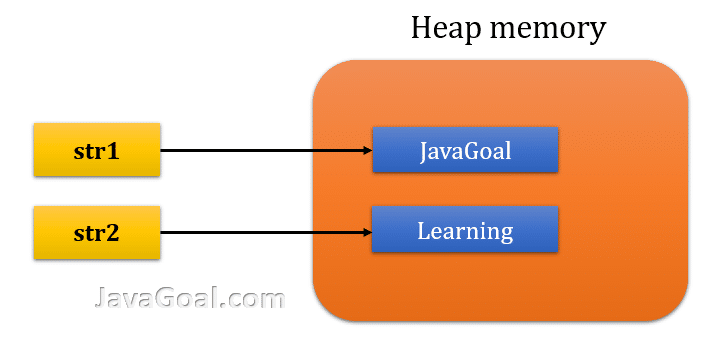
str1.append(".com"); str2.append(" website");
After execution of the above line, the JVM will change the value in existing objects. It will not create new objects.

Can we use StringBuffer and StringBuilder for passwords?