As we know java is a high-level programming language. Our system/machine can understand only machine level language. So, the machine can’t run the program of java directly because it’s written in a high-level language. It needs to be translated into that machine language.
The java compiler translates the source code (High-level language) to byte code (Not a machine level language). Now Java Virtual Machine(JVM) translates the byte code to machine level language. So we will learn Java Virtual Machine (JVM) & JVM Architecture.
Java Virtual Machine (JVM) is a virtual machine it doesn’t physically exist. It resides in the real machine (your computer) and provides a run time environment in which Java bytecode can be executed. It can also run native language programs (Those programs which are written in other languages like C and C++) and compiled to Java bytecode.
Java Virtual Machine(JVM) specialization
By the use of JVM it possible to make java platform independent. Because the compiler has to generate byte code for JVM rather than different machine codes for each type of machine. The compiler translates the code into byte code only it is JVM responsible to convert that code to machine code. The primary function of JVM is to execute the byte code produced by the compiler.
Every operating system has a different JVM, but they produce the same output after the execution of the byte code. It means generated byte code on one operating system can run in different operating systems. The programmer can write code on one system and can run on any other system without any changes. That why Java applications are called WORA (Write Once Run Anywhere).
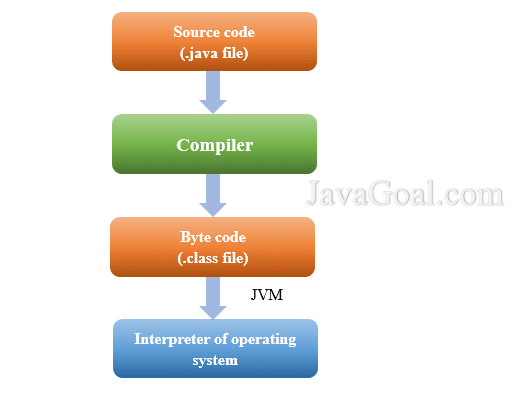
The compiler compiles .java files and creates .class files that contain the byte codes. Now JVM translates the byte code that will run by an interpreter.
Operation of JVM
classloader – load code: Classloader has three responsibility:
- Firstly, load the class files (Loading)
- Validation, preparation, and resolution(linking)
- Initialization
- Loading: Classloader is a sub-part of JVM which is used to load .class files and save the byte code in the method area. JVM stores some information in the method area for each .class file.
- Loader stores the fully qualified name of the loaded class and its parent class
- Loader stores the information of .class file is related to Class or Interface or Enum
- Method information, Variables, etc.
- Linking :
- Verification: It checks the formatted and generated by the correct compiler or not. It will throw run-time exception java.lang.VerifyError if verification is failed.
- Preparation: Initialization of the class variable are part of preparation. In this part memory allocation for default values.
- Resolution: Replace all the symbolic references from the type with direct references.
- Initialization: In this section, all the static variables and static blocks will be assigned their values. There are three built-in class loaders in Java.
- Bootstrap ClassLoader: It is the first classloader which is the superclass of Extension classloader. It loads some class files of Java:
- java.lang package classes
- java.net package classes
- java.util package classes
- java.io package classes
- java.sql package classes etc.
- Extension ClassLoader: This classloader is a middle-class loader because of it child classloader of Bootstrap and parent classloader of System classloader. It loaded the jar files located inside $JAVA_HOME/jre/lib/ext directory.
- System/Application ClassLoader: It loads the .class files from classpath. The default classpath is set current directory.
- Bootstrap ClassLoader: It is the first classloader which is the superclass of Extension classloader. It loads some class files of Java:
Java Virtual Machine (JVM) Memory
- Method/class Area: JVM has only one Method area which is part of JVM memory, and it is a shared resource. The method area contains the class level information of each .class file. Method Area stores per-class structures such as class name, immediate parent class name, methods and variables information, etc. are stored, including static variables.
- Heap: JVM has only one heap area which is part of JVM memory. Heap is the run time data area which stores all objects. It is also a shared resource.
- Stack: Stack is also part of JVM memory, and it is used for storing temporary variables. It is not a shared resource. Whenever a thread created then JVM creates one run-time stack which is called activation record/stack frame. All local variables of methods stored in their corresponding frame. After completion of the method, the run-time stack will be destroyed by JVM.
- PC Registers (Program counter register): Each thread has a separate PC register. The contains which instruction has been executed and which one is going to be executed.
- Native method stack: It stores all the native methods.
- Execution Engine: It executes the (bytecode) of the application by use of data and information present in the various memory areas. It can be classified into three parts: Interpreter: It reads the code line by line and after that, it interprets and executes. Just-In-Time Compiler (JIT): it reads the entire bytecode and changes it to native code. Garbage Collector: It destroys all the unreferenced objects.
- Native Method Interface: It provides an interface to communicate with another application written in another language like C, C++, etc
- Native Method Libraries: It is a collection of native libraries that is used by the execution engine.
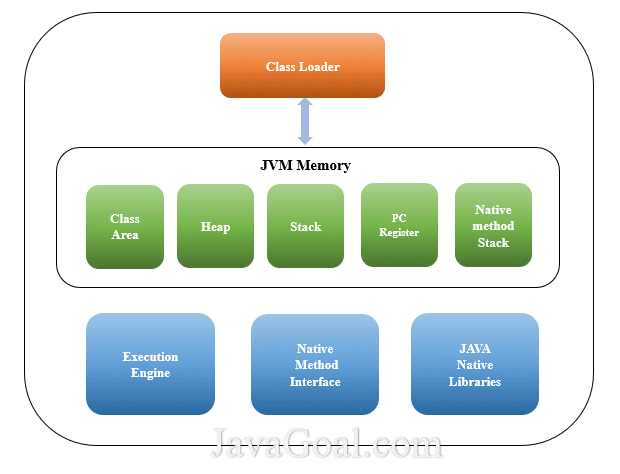