A TreeMap class is part of the Collection framework. The TreeMap implements the Map interface like HashMap and LinkedHashMap. We have learned about HashMap and LinkedHashMap in java. In this post, we will see what is TreeMap in java and TreeMap internal working. Let’s see the tree map java
Here is the table content of the article will we will cover this topic.
1. What is TreeMap in Java?
2. Important points about TreeMap in java?
3. How to create a TreeMap in java?
4. TreeMap constructor?
5. Methods of TreeMap class?
6. How to add element in TreeMap by use of TreeMap put() method
i). put(K key, V value) method
ii). putAll(Map map) method
7. How to check given key exists by treemap containsKey() method
iii) containsKey(Object key) method
8. How to check given value exists by treemap containsValue() method
iv). containsValue(Object value) method
9. How to replace in Treemap by use of treemap replace() method
v). replace(K key, V value) method
vi). replace(K key, V oldValue, V newValue) method
10. How to get HeadMap and TailMap from TreeMap?
vii). headMap(K toKey) method
viii). headMap(K toKey, boolean inclusive) method
ix). tailMap(K fromKey) method
x). tailMap(K fromKey, boolean inclusive) method
What is TreeMap in Java?
The TreeMap class implements the NavigableMap interface and extends the AbstractMap Class. The NavigableMap interface extends the SortedMap interface that extends the Map interface.
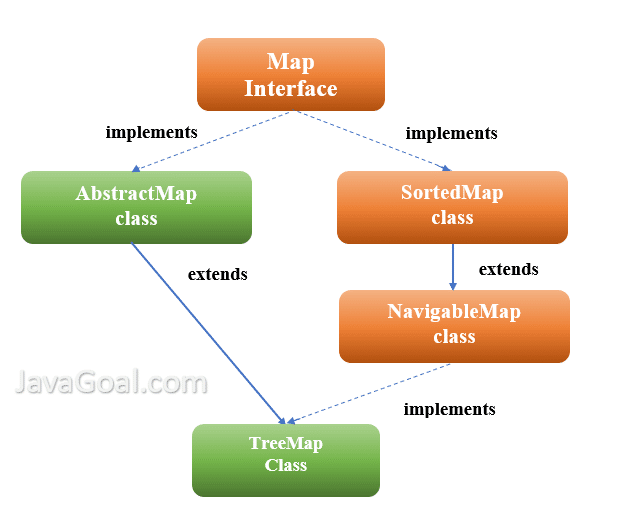
The TreeMap in java works based on key-value pairs like HashMap. But a TreeMap maintains the order of objects. By default, it sorts the objects in the natural order of its keys, and we can sort the object by a Comparator provided at map creation time. It is an efficient way of sorting and storing key-value pairs.
TreeMap<K,V> listOfNames = new TreeMap<K, V>();
Here K is the type of type data that you want to maintain in TreeMap.
V, the type of Value that you want to store in TreeMap.
Important points about TreeMap in java
1. The TreeMap class implementing the Map interface and also implementing NavigableMap and indirectly implementing the SortedMap interface. It extends the AbstractMap class.
2. The TreeMap stores each value stored with a unique key like HashMap. But HashMap is an unordered collection while TreeMap is sorted in the ascending order of its keys.
3. . Like HashMap, TreeMap doesn’t contain duplicate key but it can contain the duplicate value.
4. TreeMap doesn’t contain the null key. It throws NullPointerException if TreeSet contains a null key.
5. The TreeMap maintains the insertion order by default. We can maintain the custom order by use of Comparator.
6. TreeMap class is non-synchronized which means it is not suitable for thread-safe operations. We can make it synchronized explicitly.
7. TreeMap is the best choice when we want to store an object based on keys and also want to maintain the order.
8. It provides log(n) time cost for the containsKey, get, put and remove operations.
9. It returns the Iterator that is fail-fast in nature, so it throws ConcurrentModificationException if we do any concurrent modification.
How to create a TreeMap in java
In the above section, we have discussed what is TreeMap in java. Let’s see how to make a tree map in java. The TreeMap class provides different constructors that are used to create a TreeMap. We can create a TreeMap with natural sorted order or custom order with different types of Constructors. Let’s see all the TreeMap constructor with example
TreeMap constructor
TreeMap(): It is a default constructor of TreeMap class. It creates an empty object of the TreeMap class and maintains the natural order of its key. All keys interested in TreeMap must implement a Comparable interface. The default constructor internally assigns the null to Comparator. Let’s have a look at JDK.
public TreeMap() { comparator = null; }
import java.util.TreeMap; public class TreeMapExample { public static void main(String args[]) { TreeMap<Integer, String> treeMap = new TreeMap<Integer, String>(); treeMap.put(5, "Hi"); treeMap.put(2, "JavaGoal"); treeMap.put(3, "Learning"); treeMap.put(1, "Website"); System.out.println("All object from TreeSet: "+ treeMap); } }
Output: All object from TreeSet: {1=Website, 2=JavaGoal, 3=Learning, 5=Hi}
TreeMap(Map m): It is a parameterized constructor of the TreeMap class. This constructor takes one parameter of Map type. It creates an object of TreeMap with specified Map m and maintains the natural order of its key. It copies all the objects from the specified map and store in TreeMap. It throws NullPointerException if the given map is null. This constructor internally assigns the null to Comparator and puts all objects from the specified map. Let’s have a look at JDK.
public TreeMap(Map<? extends K, ? extends V> m) { comparator = null; putAll(m); }
import java.util.Map; import java.util.TreeMap; public class TreeMapExample { public static void main(String args[]) { Map<Integer, String> map = new TreeMap<Integer, String>(); map.put(5, "Hi"); map.put(2, "JavaGoal"); map.put(3, "Learning"); map.put(1, "Website"); TreeMap<Integer, String> treeMap = new TreeMap<Integer, String>(map); System.out.println("All object from TreeSet: "+ treeMap); } }
Output: All object from TreeSet: {1=Website, 2=JavaGoal, 3=Learning, 5=Hi}
TreeMap(Comparator comp): It creates an object of TreeMap and maintains the order of objects according to the given comparator. This constructor takes an object of Comparator. Let’s take a java TreeMap example or TreeMap comparator example.
import java.util.Comparator; import java.util.TreeMap; class Student { int rollNo; String name; int age; Student(int rollno,String name,int age) { this.rollNo=rollno; this.name=name; this.age=age; } } class AgeComparator implements Comparator<Student> { @Override public int compare(Student student1, Student student2) { if(student1.age==student2.age) return 0; else if(student1.age>student2.age) return 1; else return -1; } } public class TreeMapExample { public static void main(String args[]) { TreeMap<Student, String> treeMap = new TreeMap<Student, String>(new AgeComparator()); treeMap.put(new Student(1, "John", 19), "From USA"); treeMap.put(new Student(2, "Ram", 18), "From India"); treeMap.put(new Student(3, "Krishna", 20), "From UK"); System.out.println("Sorted by Age: "); for(Student student : treeMap.keySet()) { System.out.println(student.age); System.out.println(student.name); System.out.println(student.rollNo); } } }
Output: Sorted by Age:
18
Ram
2
19
John
1
20
Krishna
3
TreeMap(SortedMap s): It is a parametrized constructor of TreeMap class that accepts a parameter of the SortedMap type. This constructor is used to convert the SortedMap object to TreeMap Object. It throws NullPointerException if given SortedMap is null.
import java.util.SortedMap; import java.util.TreeMap; public class TreeMapExample { public static void main(String args[]) { SortedMap<Integer, String> sortedMap = new TreeMap<Integer, String>(); sortedMap.put(1, "Hello"); sortedMap.put(5, "Hi"); sortedMap.put(4, "Hye"); sortedMap.put(3, "JavaGoal.com"); sortedMap.put(2, "Website"); System.out.println("All objects from SortedMap: "+ sortedMap); } }
Output: All objects from SortedMap: {1=Hello, 2=Website, 3=JavaGoal.com, 4=Hye, 5=Hi}
Methods of TreeMap class
- ceilingEntry(K key): It returns the pair of key-value having the closest greater than or equal to the specified key. It returns null if there is no such key. You can read it in detail from here.
- floorEntry(K key): It returns the pair of key-value having closest less than or equal to the specified key. It returns null if there is no such key. You can read it in detail from here.
- ceilingKey(K key): It returns the least key, greater than the specified key. It returns null if there is no such key. You can read it in detail from here.
- floorKey(K key): It returns the greatest key, less than the specified key. It returns null if there is no such key. You can read it in detail from here.
- comparator(): It returns a Comparator that used to sort the elements of TreeMap. If, it will return null if default natural sorting order is used. You can read it in detail from here.
- get(Object key): This method is used to get or fetch the value mapped by the specified key. You can read it in detail from here.
- containsKey(Object key): This method is used to check whether the specified key exists in TreeMap or not. Its returns type is boolean. You can read it in detail from here.
- containsValue(Object value): This method is used to check whether the specified Value exists in TreeMap or not. Its returns type is boolean. You can read it in detail from here.
- clear(): This method is used to remove all mapping(Pair) from the TreeMap. It doesn’t return anything, so it returns type is void. You can read it in detail from here.
- clone(): The clone() method of TreeMap class is used to return a shallow copy of the specified TreeMap.
- descendingKeySet(): It returns a NavigableSet view of the Keys in reverse order. You can read it in detail from here.
- descendingMap(): It returns a NavigableSet view of the Key-Value(pairs) in reverse order. You can read it in detail from here.
- firstEntry(): It returns the key-value pair having the least key(Key having lowest value). It returns the pair that associated with the least key. You can read it in detail from here.
- lastEntry(): It returns the greatest key of Sorted Map. It returns the pair that associated with the greatest key(Key having greatest value). You can read it in detail from here.
- firstKey(): It returns the least key of the Sorted Map. It returns the pair that associated with the least key(Key having the lowest value). You can read it in detail from here.
- lastKey(): It returns the greatest key of Sorted Map. It returns the pair that associated with the greatest key(Key having the highest value). You can read it in detail from here.
- keySet(): This method is used to fetch the Set view of all keys. It returns all the key set. You can read it in detail from here.
- entrySet(): This method is used to fetch the Set view of all the keys and values. It returns all the keys and values.
- headMap(K toKey): It returns the key-value pairs whose keys are strictly less than the specified key toKey. You can read it in detail from here.
- headMap(K toKey, boolean inclusive): It returns the key-value pairs whose keys are less than (or equal to if inclusive is true) toKey. You can read it in detail from here.
- tailMap(K fromKey): It returns key-value pairs of NavigableMap whose keys are greater than or equal to fromKey. You can read it in detail from here.
- tailMap(K fromKey, boolean inclusive): ): It returns key-value pairs of NavigableMap whose keys are greater than (or equal to if inclusive is true). You can read it in detail from here.
- higherEntry(K key): It returns the key-value pair whose key strictly greater than the given key, or null if there is no such key.
- lowerEntry(K key): It returns the key-value pair whose key strictly less than the given key, or null if there is no such key.
- higherKey(K key): It returns the key whose key strictly greater than the given key, or null if there is no such key. You can read it in detail from here.
- lowerKey(K key): It returns the key whose key strictly less than the given key, or null if there is no such key. You can read it in detail from here.
- navigableKeySet(): It returns a view of the keys of NavigableSet.
- values(): This method is used to get all values of LinkedHashMap. It returns Collection type.
- pollFirstEntry(): It removes and returns a key-value pair that associated with the least key in TreeMap. It returns null if the TreeMap is empty.
- pollLastEntry(): It removes and returns a key-value pair that associated with the greatest key in TreeMap. It returns null if the TreeMap is empty.
- put(K key, V value): This method is used to set the value with the corresponding key in TreeMap. If TreeMap already contained the key, then the old value is replaced by new Value. You can read it in detail from here.
- putAll(Map map): This method is used to copy all elements of the specified map in current TreeMap. It copies all the mapping(Key and value pairs) of the specified Map to current TreeMap. You can read it in detail from here.
- replace(K key, V value): This method is used to replace the entry for the specified key only if it is currently mapped to some value. You can read it in detail from here.
- replace(K key, V oldValue, V newValue): This method is used to replace the entry for the specified key only if it is currently mapped to some value. If oldValue doesn’t match with the associated key, then it does not replace the value. Its return type is boolean. You can read it in detail from here.
- subMap(K fromKey, boolean fromInclusive, K toKey, boolean toInclusive): It returns key-value pairs of NavigableMap whose keys range from fromKey to toKey.
- subMap(K fromKey, K toKey): It returns key-value pairs of NavigableMap whose keys range from fromKey, inclusive, to toKey, exclusive.
- remove() method: This method is used to remove the given element from the TreeMap.
- size() method: This method returns the number of elements in TreeMap. Its return type is int. You can read it in detail from here.
Java TreeMap example
import java.util.TreeMap; public class ExampleOfTreeMap { public static void main(String[] args) { // creating a TreeMap that can contain Integer type data and Key is String type TreeMap<Integer, String> listOfCount = new TreeMap<Integer, String>(); // Adding String data in TreeMap listOfCount.put(3, "Three"); listOfCount.put(1, "One"); listOfCount.put(2, "Two"); listOfCount.put(5, "Five"); listOfCount.put(4, "Four"); // For transverse we have to get all keys for(Integer key : listOfCount.keySet()) System.out.println("Now Count is = "+ listOfCount.get(key)); // Adding duplicate key but it doesn't add it in TreeMap listOfCount.put(8, "Three"); } }
Output: Now Count is = One
Now Count is = Two
Now Count is = Three
Now Count is = Four
Now Count is = Five
In the above example, we created a TreeMap in java that can contain Integer values with their corresponding String Keys. It means it can contain only the String values.
TreeMap<Integer, String> listOfCount = new TreeMap<Integer, String>();
The above line creates a new TreeMap in Heap memory. After that, we added some Entries in TreeMap. Each Entry contains (Key, value) pair. As you know TreeMap can contain only unique Key, so it doesn’t contain key “Three” multiple times.
listOfCount.put("Three", 8);