The most popular questions in java how to sort an ArrayList in java. The sorting of ArrayList objects totally depends on Comparable or Comparator. In this post, we will see the sorting an ArrayList. To sort an ArrayList we have two ways:
Here is the table content of the article will we will cover this topic.
1. ArrayList.sort(Comparator c)
2. Collections.sort(Comparator c)
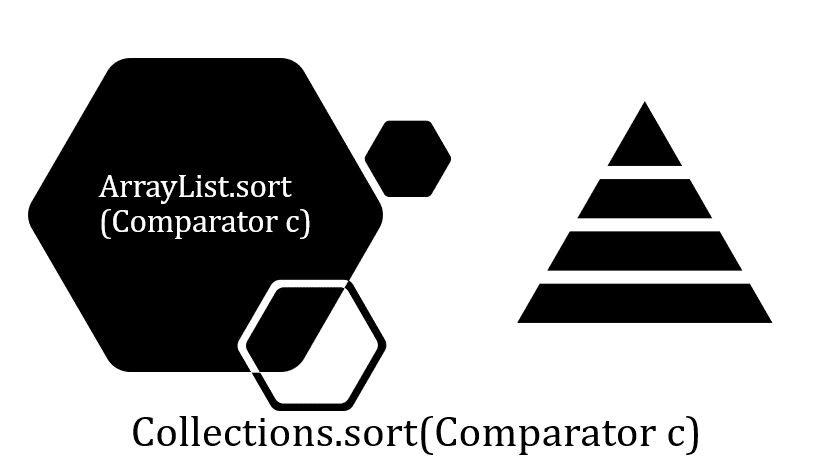
ArrayList.sort(Comparator c)
Suppose we have an ArrayList that stores some String objects. To sort the ArrayList, We need to provide the reference of the Comparator object. If you are a beginner then read the Comparator interface first. The sort(Comparator c) method, sorts the Strings alphabetically in ascending order).
public void sort(Comparator<E> c)
void, This method doesn’t return anything because it’s return type is void.
c, Object of comparator
import java.util.ArrayList; import java.util.Comparator; public class ArrayListExample { public static void main(String[] args) { ArrayList<String> listOfNames = new ArrayList<String>(); listOfNames.add("D"); listOfNames.add("A"); listOfNames.add("Z"); listOfNames.add("Y"); listOfNames.add("X"); System.out.println("Before sorting: "+ listOfNames); listOfNames.sort(new MyComparator()); System.out.println("After sorting: "+ listOfNames); } } class MyComparator implements Comparator<String> { public int compare(String string1, String string2) { return string1.compareTo(string2); } }
Output: Before sorting: [D, A, Z, Y, X]
After sorting: [A, D, X, Y, Z]
Sorting of ArrayList contains User-defined class objects
Suppose we have an ArrayList of Student’s object and we want to sort the ArrayList on based of different criteria. Let’s see java sort ArrayList of objects
import java.util.ArrayList; import java.util.Comparator; class Student { int rollNo; String name; int age; Student(int rollno,String name,int age) { this.rollNo=rollno; this.name=name; this.age=age; } } class AgeComparator implements Comparator<Student> { @Override public int compare(Student student1, Student student2) { if(student1.age==student2.age) return 0; else if(student1.age>student2.age) return 1; else return -1; } } class RollNoComparator implements Comparator<Student> { @Override public int compare(Student student1, Student student2) { if(student1.rollNo==student2.rollNo) return 0; else if(student1.rollNo>student2.rollNo) return 1; else return -1; } } public class ArrayListExample { public static void main(String args[]) { ArrayList<Student> listOfStudent = new ArrayList<Student>(); listOfStudent.add(new Student(1,"Ravi",26)); listOfStudent.add(new Student(2,"kant",27)); listOfStudent.add(new Student(3,"kamboj",20)); // It Sorts all the objects based on Age listOfStudent.sort(new AgeComparator()); System.out.println("Sorting based on Age:"); for(Student student:listOfStudent) { System.out.println("RollNo of Student = "+student.rollNo); System.out.println("Age of Student = "+student.age); System.out.println("Name of Student = "+student.name); } // It Sorts all the objects based on RollNo listOfStudent.sort(new RollNoComparator()); System.out.println("Sorting based on RollNo:"); for(Student student:listOfStudent) { System.out.println("RollNo of Student = "+student.rollNo); System.out.println("Age of Student = "+student.age); System.out.println("Name of Student = "+student.name); } } }
Output: Sorting based on Age:
RollNo of Student = 3
Age of Student = 20
Name of Student = kamboj
RollNo of Student = 1
Age of Student = 26
Name of Student = Ravi
RollNo of Student = 2
Age of Student = 27
Name of Student = kant
Sorting based on RollNo:
RollNo of Student = 1
Age of Student = 26
Name of Student = Ravi
RollNo of Student = 2
Age of Student = 27
Name of Student = kant
RollNo of Student = 3
Age of Student = 20
Name of Student = kamboj
Collections.sort(Comparator c)
By use of Collections.sort(Comparator c) we can sort the ArrayList. Suppose we have an ArrayList of String we can sort it by Collections.sort() method.
import java.util.ArrayList; import java.util.Collections; import java.util.Comparator; public class ArrayListExample { public static void main(String[] args) { ArrayList<String> listOfNames = new ArrayList<String>(); listOfNames.add("D"); listOfNames.add("A"); listOfNames.add("Z"); listOfNames.add("Y"); listOfNames.add("X"); System.out.println("Before sorting: "+ listOfNames); Collections.sort(listOfNames); System.out.println("After sorting: "+ listOfNames); } } class MyComparator implements Comparator<String> { public int compare(String string1, String string2) { return string1.compareTo(string2); } }
Output: Before sorting: [D, A, Z, Y, X]
After sorting: [A, D, X, Y, Z]
Sorting of ArrayList contains User-defined class objects
import java.util.ArrayList; import java.util.Collections; import java.util.Comparator; class Student { int rollNo; String name; int age; Student(int rollno,String name,int age) { this.rollNo=rollno; this.name=name; this.age=age; } } class AgeComparator implements Comparator<Student> { @Override public int compare(Student student1, Student student2) { if(student1.age==student2.age) return 0; else if(student1.age>student2.age) return 1; else return -1; } } class RollNoComparator implements Comparator<Student> { @Override public int compare(Student student1, Student student2) { if(student1.rollNo==student2.rollNo) return 0; else if(student1.rollNo>student2.rollNo) return 1; else return -1; } } public class ExampleOfComparable { public static void main(String args[]) { ArrayList<Student> listOfStudent = new ArrayList<Student>(); listOfStudent.add(new Student(1,"Ravi",26)); listOfStudent.add(new Student(2,"kant",27)); listOfStudent.add(new Student(3,"kamboj",20)); // It Sorts all the objects based on Age Collections.sort(listOfStudent, new AgeComparator()); for(Student student:listOfStudent) { System.out.println("RollNo of Student = "+student.rollNo); System.out.println("Age of Student = "+student.age); System.out.println("Name of Student = "+student.name); } // It Sorts all the objects based on RollNo Collections.sort(listOfStudent, new RollNoComparator()); for(Student student:listOfStudent) { System.out.println("RollNo of Student = "+student.rollNo); System.out.println("Age of Student = "+student.age); System.out.println("Name of Student = "+student.name); } } }
Output: RollNo of Student = 3
Age of Student = 20
Name of Student = kamboj
RollNo of Student = 1
Age of Student = 26
Name of Student = Ravi
RollNo of Student = 2
Age of Student = 27
Name of Student = kant
RollNo of Student = 1
Age of Student = 26
Name of Student = Ravi
RollNo of Student = 2
Age of Student = 27
Name of Student = kant
RollNo of Student = 3
Age of Student = 20
Name of Student = kamboj