Garbage collection is a process of removing unused objects from heap memory. It works with a Garbage collector. There are different kinds of garbage collector available in Java that collects different area of heap memory.
In this article, we will discuss the Garbage Collector and how many types of garbage collectors are used.
Here is the table content of the article will we will cover this topic.
1. What is Garbage Collector?
2. How does Garbage Collector work?
3. How does Garbage Collector Work in Heap memory internally?
4. Ways for requesting JVM to run Garbage Collector?
5. Types of garbage collectors?
What is Garbage Collector?
Garbage Collector is a program that runs by daemon thread in the background to manage the heap memory. It takes the responsibility of the de-allocation of objects. Whenever we create an object in Java by use of the new operator, it occupies some space in heap memory. The memory remains allocated until there are references for the use of the object.
The Garbage Collector checks the objects if there are no references to an object, it is assumed to not be in use and eligible for Garbage collection. If any object is eligible for garbage collection, then the memory occupied by the object can be reclaimed.
The technique of reclaiming memory is known as Garbage Collection.
1. It is an automatic process that is handled by JVM, so the programmer doesn’t need to take responsibility for memory management.
2. The garbage collector is a daemon thread that always runs in the background and takes care of the Garbage Collection.
3. The garbage collector always invokes the finalize() method before removing an object from memory. It gives an opportunity to perform the cleanup required.
4. We can’t force the JVM to clean or remove some objects from memory. It always depends on JVM. Most probably the JVM calls Garbage Collector if there is less memory available in the Heap area or when the memory is low.
How does Garbage Collector work?
As you know JVM loads the Java program and compiles it. JVM creates the objects in the heap memory. During the execution of the program, some objects will no longer be needed. If any object is not in use, then a garbage collector comes into the role. The Garbage Collector finds the unused objects and deletes them to free up memory.
Step 1: Firstly, Garbage collection identified and marked the unreferenced objects that are ready for garbage collection.
Step 2: Before removing an object from memory the garbage collector
invokes finalize() method of that object and gives an opportunity to perform any sort of cleanup required.
Step 3: The Garbage collector deletes only those elements which are eligible for Garbage collection. Any object remains allocated in memory till its references are in use. If there are no references for an object, it is assumed to be no longer needed and it is eligible for Garbage collection.
Step 4: After deleting the object from memory, the memory can be compacted. It makes it easier to allocate memory to new objects.
Step 5: Garbage collection is Daemon thread which is always running in the background and makes a strategy to categorize all the objects by age. The reason behind this categorization is that most objects are short-lived and will be ready for garbage collection soon after creation.
How does Garbage Collector Work in Heap memory internally?
As you already know Heap memory is used to store the java objects created.
Heap is divided into three parts.

1. Young generation
The young Generation is the memory area occupied by the newly created object. It is the memory area in which an object starts its journey when it is created.
This memory area is also divided into three parts.
i) Eden space: It is the first subpart of memory where all new objects start. Whenever an instance is created. It is first stored in the Eden space.
ii.) Survivor Space (S0 and S1): Apart from Eden space there are two Survivor spaces in which objects are moved from Eden. The objects will move into this memory after one garbage collection cycle. Those objects are still alive(Which are referenced) are moved to S0. Similarly, the garbage collector performs a cycle in S0 and moves the live instances to S1. If any object is not live, then it is marked for garbage collection.
2. Old Generation(Tenured)
It is the second logical part of the heap memory. It collects objects from the young generation that are long-lived. After performing the minor GC cycle, if instances are still live then those will be promoted to the old generation. It is a major garbage collection event.
3. Permanent Generation
It contains Metadata such as classes and methods. After Permanent Generation, classes that are no longer in use.
Ways for requesting JVM to run Garbage Collector?
Garbage collector collects all the objects those are eligible for garbage collection. These objects will not destroy immediately by the garbage collector. These objects will be destroyed when JVM runs the Garbage Collector program. There is no guarantee when JVM runs the Garbage Collector.
JVM makes a request to run the Garbage Collector.
There are two ways to do it
Using the System.gc() method
To run the Garbage collector the JVM makes a call to gc() method. It is a static method gc() of System class.
class Emp { int empId; String name; Emp(int empId, String name) { this.empId = empId; this.name = name; } public int getEmpId() { return empId; } public void setEmpId(int empId) { this.empId = empId; } public String getName() { return name; } public void setName(String name) { this.name = name; } } class Example { public static void main(String arg[]) { // Here we are creating two objects Emp emp1 = new Emp(1, "Ravi"); Emp emp2 = new Emp(2, "John"); if(emp1.getEmpId() == 1) { emp2 = null; } // JVM will make a call to gc() // Because emp2 is eligible for Garbage collection. // System.gc(); } }
Using Runtime.getRuntime().gc() method
Runtime class allows the application to interface with the JVM in which the application is running. Hence by using its gc() method, we can request JVM to run the Garbage Collector.
Types of garbage collectors?
There are four types of Garbage collectors. You can choose one of them based on your application’s performance requirement. Each garbage collector has some advantages and disadvantages. You can choose one of them bypassing the choice as a JVM argument.
Before making a choice, you must understand each type of garbage collector and how you should use them based on the application. You should understand the best and the right garbage collector for application.
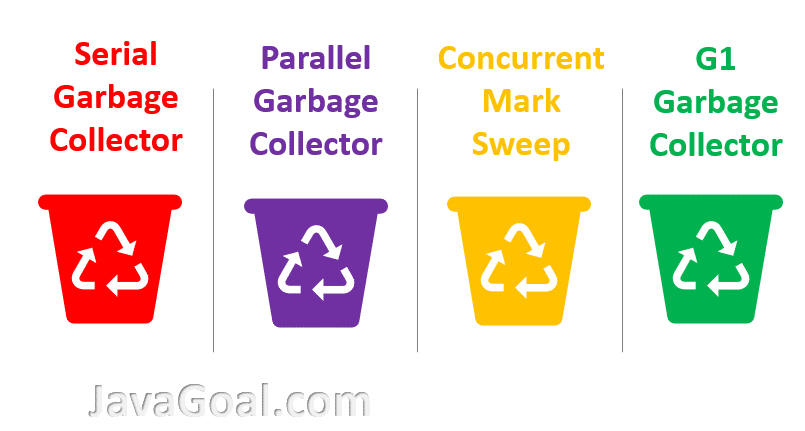
1. Serial Garbage Collector
Serial garbage is designed for a single-thread environment. This collector handles all the garbage collection events conducted serially in one thread. It holds all the threads of the application but uses just a single thread for garbage collection.
This garbage collector may not suitable for a server application. Because freezes all the application threads while doing garbage collection. It is best suited for simple command-line programs.
You can use the serial garbage collector by providing the JVM argument. Turn on the -XX:+UseSerialGC JVM argument to use the serial garbage collector.
2. Parallel/ Throughput Garbage collector
It is JVM’s default collector in JDK 8. It uses multiple threads for minor garbage collection. Unlike the serial garbage collector, it uses multiple threads to perform the operation on garbage collection. But the drawback of this is also freezing all the application threads while performing garbage collection.
It is best suited if applications can handle such pauses and try to optimize CPU overhead caused by the collector then it is a good option.
You can use the Parallel/ Throughput Garbage collector by providing the JVM argument. Turn on the -XX:+UseParallelGC JVM argument to use the Parallel/ Throughput Garbage collector.
3. CMS (Concurrent Mark Sweep) Collector
This is also referred to, Concurrent low pause Collector. There are multiple threads that are used to mark instances for eviction and then sweep the marked instances from the heap memory. CMS collector uses more CPU than the parallel garbage collector. Because it gives better performance to the application.
You can use the Concurrent Mark Sweep collector by providing the JVM argument. Turn on the XX:+USeParNewGC JVM argument to use the Concurrent Mark Sweep Collector.
4. G1 (Garbage First)
The G1 garbage collector is used for large heap memory areas. The newest garbage collector is intended as a replacement for CMS. G1 also compacts the free heap space on the go just after reclaiming the memory. It is parallel and concurrent like CMS, but it works quite differently under the hood compared to the older garbage collectors.
You can use the G1 collector by providing the JVM argument. Turn on the –XX:+UseG1GC JVM argument to use the G1 collector.