The package in java is one of the most important features of Java. If you are learning Java, you need to understand the packages in java. Here we will describe what is the package in java, the steps to create the package in java with the example, the java package naming convention, the default package in java, and user-defined packages in java.
Here is the table content of the article will we will cover this topic.
1. What is the package in Java?
2. Build-in package?
3. How the package is working internally?
4. User-defined packages in java
5. How to create a package?
i) Creating package in java (Without IDE)
ii) Creating package with IDE(Eclipse)
6. java package naming convention
7. Adding a Class in the existing package
8. Adding a class in the package while importing another package
9. Important points about the package
10. A real-life scenario in a java package example
11. Sub packages in java
12. Advantages of package
13. Access of package
What is the package in Java?
On your computer, you must see the different drives, folders, and subfolders that use to classify the various files. We can provide the accessibility of various files and folders. Like a folder, we use packages in java and manage the Java applications. The main use of the package is to organize the classes, interfaces, enum, and sub-packages.
A Package in java is a group of similar types of classes, interfaces, enumerations, and sub-interfaces. A package is like a container that can have a group of related classes. Where some of the classes are accessible and some of them are kept for internal purposes. It is used to organize the classes, and interfaces and also provide access protection and namespace management
Suppose you are creating an application that will contain 100 classes and interfaces. So, it would be better if we use the package and organize your classes into a folder structure and make it easy to locate and use them. It will help to improve re-usability.
In Java, there are many in-build packages like java.lang, java.util, java.io, and java.net. All the packages contain a number of classes, interfaces, enums, and sub-packages. All these packages are defined as a very clear and systematic packaging mechanism for categorizing and managing.
Let’s say you want to print the current date in java. Here you need to invoke the constructor of the Date() class. The Date class exists in java.util package. So we need to import that package into the class.
import java.util.Date; public class EncapsulationExample { public static void main(String args[]) { System.out.println(new Date()); } }
Output: Sun Apr 26 16:51:09 IST 2020
Packages are divided into two categories:
- Built-in packages in java
- user-defined packages in java
1. Built-in packages in java
Packages provided by JAVA are known as built-in packages. These packages contain a lot of classes, interfaces, enums, etc. That can be used by the user. There are many built-in packages. Some of the commonly used :
- java.lang: Here, Java is a top-level package, and lang is a subpackage of java. The lang package contains classes that are fundamental to the design of the Java programming language. The most important class is the Object, which is the root of the class hierarchy.
- java.util: Here, Java is a top-level package, and util is a subpackage of java. The util package contains all the utility classes, and it contains the collections framework.
- java.awt: Here, Java is a top-level package, and awt is a subpackage of java. The awt package contains classes for implementing the components for graphical user interfaces.

In the JAVA language, java is the top package in build-in packages. All sub-packages are part of the java package.
import java.util.ArrayList; public class EncapsulationExample { public static void main(String args[]) { ArrayList<String> list = new ArrayList<String>(); list.add("Ram"); list.add("Sham"); list.add("Krishan"); } }
Output: [Ram, Sham, Krishan]
How package is working internally
Package in Java is based on directory structure. In a directory structure, a directory can contain several sub-directories, folders, and files. Similarly, the package can contain sub-packages, classes, interfaces, etc.
For Example Here java.util.Date is a package. It means it consists of two directories. The Date is a class that is a sub-part of the util package, and util is a sub-package of java.
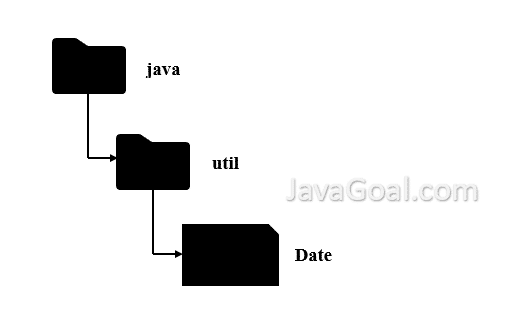
2. User-defined packages in java
A programmer can define their own package, and this package is a known user-defined package. It is a good practice to group related classes implemented by you so that a programmer can easily determine that the classes, interfaces, etc are related. Let’s see how can we create a package in java?
How to create a package in java?
The package in java that is created by the user is known as a User-defined package. It can contain user-defined classes, enums, and interfaces.
There are two ways to create a package. If you are working with IDE like eclipse, then you don’t need to worry about the syntax of the package. But if you are not using any IDE then you have to follow some syntax. Here we will discuss them one by one.
Creating package in java (Without IDE)
In java, the creation of the package is very simple. To create a package in java we need to use the package keyword and choose the name of the package. The declaration of the package should be in the first line of the program. After the declaration of the package, you can create a class, enum, or interface.
To create a package in java we must follow two steps. In the first step, The declaration of the package, and in the second step, the creation of the package by use of the command.
Step1: Syntax to declare a package in java.
package packageName;
Here package is a keyword and packageName is the name of the package.
package goal;
We can declare a package inside another package. Because of java support subdirectories.
package java.goal
Let’s take an example and see how we can use the package keyword in java. Here we will use the package keyword with the name of the package at the top of the Java source file.
package data; public class Calculator { public void calculate(int a, int b, char operation) { switch(operation) { case '+' : System.out.println("Addition of number : "+ (a+b)); break; case '*': System.out.println("Multiplication of number : "+ (a*b)); break; case '-': System.out.println("Subtraction of number : "+ (a-b)); break; case '/': System.out.println("Division of number : "+ (a/b)); break; } } public static void main(String arg[]) { Calculator calculator = new Calculator(); calculator.calculate(1, 2, '+'); calculator.calculate(4, 2, '*'); calculator.calculate(10, 5, '-'); calculator.calculate(4, 2, '/'); } }
Output:Addition of number : 3
Multiplication of number : 8
Subtraction of number : 5
Division of number : 2
Here I have created a Calculator class that can perform the calculation for my application. So, I have created it inside a package name data. As we already discuss, to create a package with a java source file, we need to use the package keyword with the package name. Always remember the declaration should be the first statement in the source program.
Step2: Now we have declared the package in the java source file. Now we need to compile it.
i) Save the file with java extension. Like Calculator.java
ii) Now compile the file with the command “javac Calculator.java” After the compilation of the java file a class file Calculator.class will be created. But there is no package is created yet. To create a package, we need to see the next step.
iii) Now we must create a package, and use the command
javac -d . Calculator.java
This command created a folder in a directory and place the class file in that folder. The folder name must be the same as the package name.
Creating a package with IDE(Eclipse)
If you are using any IDE like eclipse then you don’t need to worry about the command or something else. You just need to follow some steps in eclipse.
Right-click on your project and go to New > package. Now put the name of the package and click on the finish button.

OR
Right-click on your project and go to New > class. A dialog will appear that asks for the class and package names also.
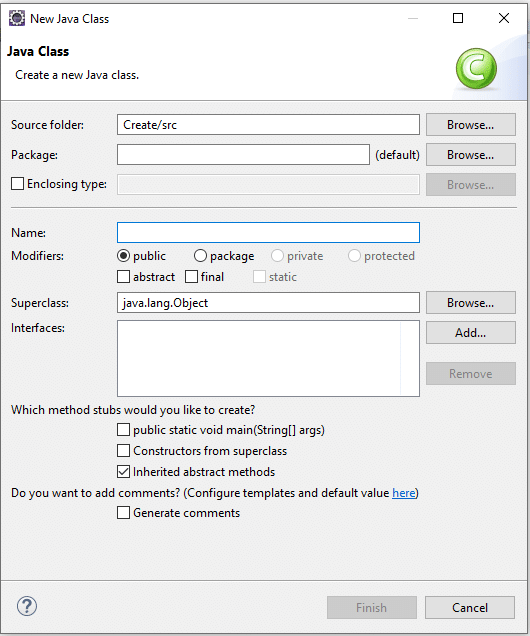
java package naming convention
1. Always define the package name in lowercase. All letters should be in lowercase.
2. The name of the package should be realistic. The name can determine by the company or organization that creates it. We can determine the package name by reversing the company domain name.
3. If a package contains multiple names then it should be separated by dots(.)
4. The package names must be groups of characters. Java does not recommend a package name with only a single character.
5. Package names and class names should reflect business concepts.
6. Package name must be unique (as a domain name). The convention is to create a package as a domain name but in reverse order. For example, com.goal.java
Adding a Class in the existing package
We can add any class to the existing package. To include a class inside an existing package, you should mention the package name with the package keyword as the first statement in the program. We can include a class in only one package at one time.
Let’s take an example from an existing package. Here we will add a new class Utility.
package data; public class Utility { public static void main(String arg[]) { System.out.println("It is a utility class"); System.out.println("We are adding this class in existing package"); } }
Output: It is a utility class
We are adding this class in existing package
As you can see, I have created a Utility class in an existing package named data. This package already contains a Calculator class. When we compile the Utility class, the Utility.class will be created in the data folder.
Adding a class in a package while importing another package
You must have seen in the above example we are adding a class to the existing package. But sometimes we need to import a class from other packages and also need to create a new package. In Java, we can use the import statement to import the package and at the same time, we can declare a new package also. But the declaration statement of the package should be in the first line.
package data; import java.util.Date; public class DateProvider { public void dateProvider() { System.out.println(new Date(2, 2, 2020)); } public static void main(String arg[]) { DateProvider provider = new DateProvider(); provider.dateProvider(); } }
Output: Tue Sep 10 00:00:00 IST 1907
I first declared the package data, then imported the class Date from the package java.util. We should follow the order when we are adding a class to the package and also importing another package. Package declaration should be the first statement then the import statement would be used.
Important points about the package
1. Every class is existing in a package. If you are not declaring any package the JVM put the class in the default package. A class that exists in the default package means the current working directory. JVM put all the classes in the same directory that haven’t package names.
2. A class can import any number of packages by import statements, but it can use only one package statement.
3. The creation statement of a package should be the first statement of the program. You can’t specify any other statement above the package statement. You can define comments to the above of the package statement.
4. The name of the package must be the same as the directory under which the file is saved.
5. A public class can directly access the package. You can access public classes in another package. Here is the syntax to access the public class
packageName.className
6. There may be a situation when class name conflict may occur. Let’s say we have two packages java.util and java.sql. Both the packages have a class with the same name that is the Date class. Now suppose a class imports both these packages like this
import java.util.Date; import java.sql.Date; public class ExampleOfPackage { public static void main(String arg[]) { System.out.println("Date with time : "+ new Date(0)); System.out.println("Sql date : "+ new Date(0)); } }
It shows an error at compile time “The import java.sql.Date collides with another import statement“.
In this type of scenario, we should use the full qualified name of the class with the package. If we will not use a fully qualified name? Then the compiler will get confused about which class should be used.
import java.util.Date; public class ExampleOfPackage { public static void main(String arg[]) { System.out.println("Date with time : "+ new Date(0)); System.out.println("Sql date : "+ new java.sql. Date(0)); } }
Output: Date with time: Thu Jan 01 05:30:00 IST 1970
Sql date : 1970-01-01
A real-life scenario in a java package example
Let’s say you are creating a website for a University. A university can have multiple colleges in different districts or states.
- Let’s say the university’s name is KUK which placed in Kurukshetra city.
- Some colleges have the same name but different cities, and some have different names but the same cities.
- MLN college from Yamunanagar
- MLN college from Ambala.
- Khalsa college Ambala.
- So, if we want to create classes, interfaces, and enumerations for these colleges, we can create these separately based on cities. We can place all the related classes, interfaces, and enumerations in packages.
- If anyone programmer faces any issue with Yamunanagar college’s data, then he/she will not search the file on the whole directory. He/she will directly go through with the package name.
- Without the package, you can’t create two classes with the same name because they are treated as in the same default package. But we can create classes with the same name but in different packages.

package kuk.yamunanagar; public class MLN { public static void main(String args[]) { System.out.println("Welcome to MLN college"); } }
package kuk.ambala; public class MLN { public static void main(String args[]) { System.out.println("Welcome to MLN college"); } }
package kuk.ambala; public class Khalsa { public static void main(String args[]) { System.out.println("Welcome to Khalsa college"); } }
How the package works internally for the university:
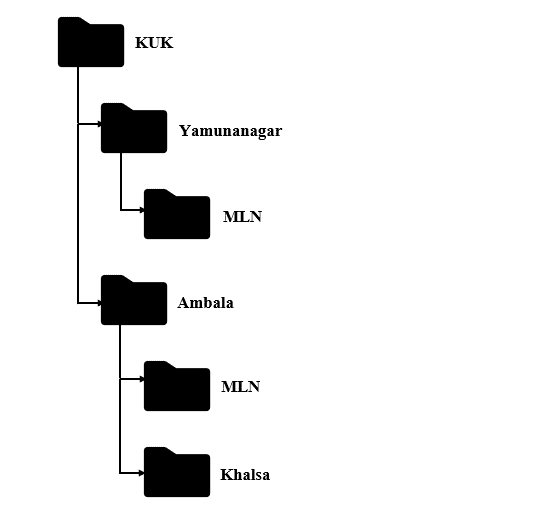
Adding a class to a Package
We can add more classes to the existing package by using package names at the top of the program and saving it in the package directory. We need a new java file to define a public class, otherwise, we can add the new class to an existing .java file and recompile it.
Sub packages in java
A package can have many classes, interfaces, enums, or sub-packages. When a package is created inside another package is known as a subpackage in java. In our JDK, there are many examples of sub-packages like java.lang here java is a package and lang is a subpackage, java.util here java is a package and util is a subpackage.
These are not imported by default. So, you have to import it explicitly. You can import any class or interface from a subpackage by use of import statements. Let’s read how to access packages.
Advantages of packages in java
1. Reuse of code
The most common advantages of packages in java are reusability. During the development, if you feel you are writing some duplicate code that is already written. You can create such things in the form of classes inside a package. So, whenever you need to perform that same task or need the same code then you can use it by importing that package.
For example: If a company has two departments one is the HR Department, and another is the IT Department. Both departments want to perform some calculations. So, we can place the logic of calculation in class that will be part of another package. In JAVA, we will create three classes in different packages.
package myCalculator; public class Calculator { public int sum(int a, int b) { return a+b; } public int sub(int a, int b) { return a-b; } public int multiple(int a, int b) { return a*b; } public int divide(int a, int b) { return a/b; } }
package hR; import myCalculator.calculator; public class HRDepartment { public static void main(String[] args) { Calculator calculator = new Calculator(); System.out.println(calculator.sum(1, 3)); System.out.println(calculator.sub(8, 6)); System.out.println(calculator.multiple(2, 3)); System.out.println(calculator.divide(9, 3)); } }
package iT; import myCalculator.calculator; public class ITDepartment { public static void main(String[] args) { Calculator calculator = new Calculator(); System.out.println(calculator.sum(1, 3)); System.out.println(calculator.sub(8, 6)); System.out.println(calculator.multiple(2, 3)); System.out.println(calculator.divide(9, 3)); } }
In the above example, Calculator is a class in myCalculator package. So that we can use the code of the class by use of import statements.
2. Categorize/Organization of project
Packages are used to organize the structure of the project that contains the number of classes, interfaces, or enumerations. A project can have any number of functionality, So it would be better if you can place the related functionality classes, interfaces, or enumerations in a package. In large projects where we have several hundreds of classes, it is always better to create packages of similar types of classes in a meaningful package name. So that you can organize your project and if someone wants to check something they can quickly access it. It improves the efficiency of the project.
Example: In the above example, HRDepartment and ITDepartment can have any number of classes, interfaces, or enumerations. So, it is an example of a well-organized project.
3. Removes naming conflicts
The package in java plays an important role to resolve the naming conflict of classes. You must be seen you can’t create two classes with the same name(without a package). But if you are creating packages then you can define two classes with the same name but in different packages. So, by use of the package, you can remove the name collision.
We can take an example from our JDK. There are two packages java.util and java.sql that contain Date class. Although the class name is the same in both packages it doesn’t create any conflict.
4. Access protection
If you don’t know about access control you should read the access modifiers in java. The access modifiers specify access control, but the package provides an important role there. The package also provides encapsulation in java. A package can have any classes and other sub-packages and a class can have data and code. Both are the container that holds the data. You can read the access right within the package and outside the package from the access modifier.
By using packages with Access modifiers, Java provides access protection.
5. Modularity
A package contains several classes, interfaces, or enums. Suppose you are working on a large project having many functionalities. But all the functionalities need some common functionality(Which is the base for all). You can create the common functionality in a package and use that package wherever it needs. The common package acts like a module that can use accordingly. By using the package, java supports the modularity concept.
6. Information Hiding
By use of packages, you can hide the information of classes. You can specify the data member as private, protected, public, and default. By use of a modifier, you can specify which class data should be hidden from the other classes.