A HashMap stores all the objects in form of entries. An entry is a pair of key and value, Each value associated with a unique key. By using java entryset() method we can get all the entries from the HashMap. As we know we can’t directly transverse on HashMap, So it is one of the ways to transverse on HashMap.
entrySet() method
This method is used to fetch the Set view of all keys and Values. It returns all the key and values in form of Set. This Set worked on backed of HashMap, if any change makes in HashMap also reflects in this Set and vice versa. It returns type is Set. Let’s see the java entryset() method with example.
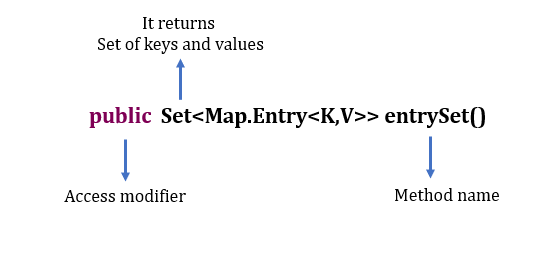
import java.util.HashMap; public class ExampleOfHashMap { public static void main(String[] args) { HashMap<Integer, String> hashMap = new HashMap<Integer, String>(); // Adding objects in HashMap hashMap.put(1, "JavaGoal.com"); hashMap.put(2, "Learning"); hashMap.put(3, "Website"); System.out.println("Get all keys and values: "+ hashMap.entrySet()); } }
Output: Get all keys and values: [1=JavaGoal.com, 2=Learning, 3=Website]
import java.util.HashMap; public class ExampleOfHashMap { public static void main(String[] args) { HashMap<String, String> hashMap = new HashMap<String, String>(); // Adding objects in HashMap hashMap.put("Hi", "JavaGoal.com"); hashMap.put("Hello", "Learning"); hashMap.put("Bye", "Website"); System.out.println("Get all keys and values: "+ hashMap.entrySet()); } }
Output: Get all keys and values: [Hi=JavaGoal.com, Hello=Learning, Bye=Website]