In Java 8 Consumer interface is defined in the java.util.function package. It contains one abstract method that is known as accept() method. This interface accepts a single input argument and doesn’t return the result.
The Consumer interface is useful when an object needs to be consumed. It takes an object as input and you can perform the operation on an object without returning any result. This interface is used in a lambda expression and method reference where the user wants to perform the operation without returning the object.
@FunctionalInterface public interface Consumer<T> { // Body of Interface }
Where T, The type of input of Consumer. Basically, it is the data type for e.g: String, Integer.
void accept(T t) method: This method is used to consume and perform the operation on the object. Its return type is void.
void catch(T t);
This interface contains a few extra methods but since they all come with a default implementation, you do not have to implement these extra methods.
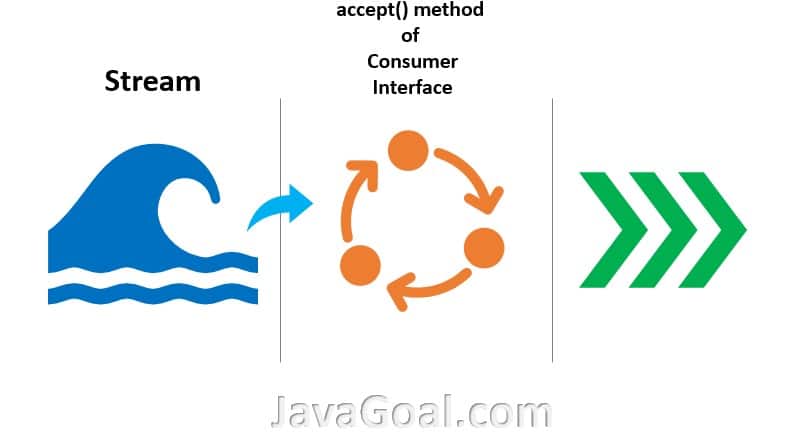
How to use Java 8 Consumer interface?
In Java by use of Consumer, you can perform the different operations on objects as per the requirement. Here we have a common example of such an operation is printing. To print any data, you just need the object and you can print it.
First of all, we will see how to use the Consumer interface without a lambda expression:
import java.util.function.Consumer; public class ExampleOfConsumerWithoutLambda { public static void main(String[] args) { String stringOne = "Hello"; Consumer<String> consumer = new Consumer<String>() { @Override public void accept(String string) { System.out.println("Accept method of Consumer = "+ string); } }; System.out.println("It accept method consumer the data "); consumer.accept(stringOne); } }
Output: It accept method consumer the data
Accept method of Consumer = Hello
Now, we will see how you can use the Consumer interface with a lambda expression:
import java.util.function.Consumer; public class ExampleOfConsumerWithLambda { public static void main(String[] args) { Consumer<String> consumer = (value) -> System.out.println("It is a consumer: "+ value); consumer.accept("Hi!!!!!"); } }
Output: It is a consumer: Hi!!!!!
Let’s discuss Consumer with user-defined class:
public class Student { int rollNo; String className; String name; public Student(int rollNo, String className, String name) { this.rollNo = rollNo; this.className = className; this.name = name; } public int getRollNo() { return rollNo; } public void setRollNo(int rollNo) { this.rollNo = rollNo; } public String getClassName() { return className; } public void setClassName(String className) { this.className = className; } public String getName() { return name; } public void setName(String name) { this.name = name; } }
import java.util.function.Consumer; public class ExampleOfConsumerForStudent { public static void main(String[] args) { // Adding data of Student Student studentOne = new Student(1, "MCA", "Ram"); Student studentTwo = new Student(2, "MSC", "Sham"); Consumer<Student> consumer = (object) -> { System.out.println(object.getName()); System.out.println(object.getRollNo()); System.out.println(object.getClassName()); }; System.out.println("First student data:"); consumer.accept(studentOne); System.out.println("Second student data:"); consumer.accept(studentTwo); } }
Output: First student data:
Ram
1
MCA
Second student data:
Sham
2
MSC
Let’s discuss Consumer with Collection using foreach:
public class Book { int bookId; String name; String authorName; public Book(int bookId, String name, String authorName) { this.bookId = bookId; this.name = name; this.authorName = authorName; } public int getBookId() { return bookId; } public void setBookId(int bookId) { this.bookId = bookId; } public String getName() { return name; } public void setName(String name) { this.name = name; } public String getAuthorName() { return authorName; } public void setAuthorName(String authorName) { this.authorName = authorName; } }
import java.util.ArrayList; import java.util.List; import java.util.function.Consumer; public class ExampleOfConsumerWithCollection { public static void main(String[] args) { Book bookOne = new Book(1, "ABC", "shiva"); Book bookTwo = new Book(5, "DEF", "RAMA"); Book bookThree = new Book(8, "GHI", "Krishna"); List<Book> books = new ArrayList<Book>(); books.add(bookOne); books.add(bookTwo); books.add(bookThree); // Creating consumer Consumer<Book> consumer = (book) -> System.out.println("Book id :"+book.getBookId()); // foreach accepts object of consumer books.stream().forEach(consumer); } }
Output: Book id :1
Book id :5
Book id :8
You see in the above example, we created a consumer and used foreach to print all data. Because forEach accepts the object of Consumer.
Advantage of Consumer:
1. By use of Consumer, you can move the operation to one place.
2. It improves code maintenance if you want to make any change in one place.
public class Book { int bookId; String name; String authorName; public Book(int bookId, String name, String authorName) { this.bookId = bookId; this.name = name; this.authorName = authorName; } public int getBookId() { return bookId; } public void setBookId(int bookId) { this.bookId = bookId; } public String getName() { return name; } public void setName(String name) { this.name = name; } public String getAuthorName() { return authorName; } public void setAuthorName(String authorName) { this.authorName = authorName; } }
import java.util.ArrayList; import java.util.List; import java.util.function.Consumer; public class ExampleOfConsumerWithCollection { public static void main(String[] args) { Book bookOne = new Book(1, "ABC", "shiva"); Book bookTwo = new Book(5, "DEF", "RAMA"); Book bookThree = new Book(8, "GHI", "Krishna"); List<Book> books = new ArrayList<Book>(); books.add(bookOne); books.add(bookTwo); books.add(bookThree); ExampleOfConsumerWithCollection obj = new ExampleOfConsumerWithCollection(); // foreach accepts object of consumer books.stream().forEach(obj.getConsumer()); } private Consumer<Book> getConsumer() { // Creating consumer Consumer<Book> consumer = (book) -> { System.out.println("Book id :"+book.getBookId()); System.out.println("Book name:"+ book.getName()); System.out.println("Auther name"); }; return consumer; } }
Output: Book id :1
Book name:ABC
Auther name
Book id :5
Book name:DEF
Auther name
Book id :8
Book name:GHI
Auther name