The yield() method in java is used to give the hint to the thread scheduler and gives the chance to other threads. The programmer gets confused about the working of the yield() method. In this post, we will see what is yield() method in java is and how to use the yield method in thread.
Here is the table content of the article will we will cover this topic.
1. What is the yield() method in java?
2. A real-life example of the yield() method
3. Why we use the yield() method?
What is the yield() method in java?
In simple terms, the meaning of ‘yield’ is “to give up” or “to surrender”. The yield() method gives the hint to the thread scheduler that the current running thread is not doing something too critical and provides a chance for other waiting threads of the same priority for their execution. The thread scheduler can ignore the hint or can take action also.
thread.yield()
When JVM executes the above line, it gives the hint to the thread scheduler that if CPU is not executing anything important and any other thread is RUNNABLE state then other threads should be run.
This method exists in the Thread class doesn’t take any parameter. It is a static and native method and doesn’t return anything. If a thread is interrupted, then it will throw InterruptedException.
- When the thread scheduler gets a call from the yield() method. The thread scheduler is free to ignore the hint, or it can pause the execution of the current thread. that it is ready to pause its execution
- When the thread scheduler gets a call from the yield() method. The thread scheduler checks if there is any thread having the same or high priority as the current thread. If any thread has the higher or same priority, then it will move the current thread to the Ready/Runnable state and give the processor to another thread. But if not, the current thread will keep executing.
A real-life example of the yield() method
Let’s say we have two threads, which are thread1, thread2, and both threads have the same priority. Thread1 uses the processor time and it is in a running state. The thread2 is in a RUNNABLE state. The completion time of thread1 is 1 hour and the completion time of thread2 is 5 minutes. So thread2 must wait for 1 hour to just finish the 5-minute job. In such types of scenarios where one thread is taking too much time to complete its execution and other important threads are waiting. Here can use the yield() method and give the chance to execute the other threads. The yield) method gives the hint to thread scheduler, The thread scheduler can take the action or it can ignore the hint.
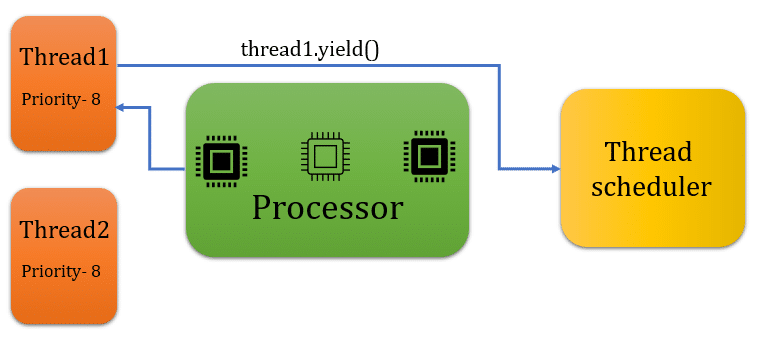
class ThreadOne extends Thread { public void run() { for(int i=1;i<=5;i++) { System.out.println("Thread one is running"); Thread.yield(); } } } class ThreadTwo extends Thread { public void run() { for(int i=1;i<=5;i++) { System.out.println("Thread two is running"); } } } class MainThread { public static void main(String args[]) { ThreadOne threadOne = new ThreadOne(); ThreadTwo threadTwo = new ThreadTwo(); threadOne.start(); threadTwo.start(); } }
Output: Thread one is running
Thread two is running
Thread two is running
Thread two is running
Thread two is running
Thread two is running
Thread one is running
Thread one is running
Thread one is running
Thread one is running
Why do we use the yield() method?
A program can have any number of threads and each thread has a different priority. The thread scheduler takes the responsibility to execute the threads. But a thread scheduler doesn’t give a guarantee to the order of execution of threads. By using of yield() method, we can give the hint to the thread scheduler, the currently running thread is not doing anything critically important. If you have any other thread that is on the priority you can assign the CPU to them. Now it totally depends upon the thread scheduler, it can ignore the hint and also make a decision to run other threads.
To give importance to the critical threads we can use the yield() method. Although we have some other methods like sleep() method that is used to pause the current thread. But the yield() method doesn’t pause the execution, it just gives the hint to the thread scheduler, now thread scheduler takes the decision whether it needs to pause or let it be.
Let’s take an example of a program and see how does yield() method works. Here we have two threads one is the main thread and another is the child thread. In the main thread the for loop is printing counting from 1 to 10, But in between the main thread calls the yield() method and wants to give chance to other threads for execution.
class ChildThread extends Thread { public void run() { for(int i=1;i<=10;i++) { System.out.println("Child Thread Running"); } } } class MainThread { public static void main(String args[]) { ChildThread childThread = new ChildThread(); childThread.start(); for(int i=1;i<=10;i++) { System.out.println("Main Thread Running"); Thread.yield(); } } }
Output: Main Thread Running
Child Thread Running
Child Thread Running
Child Thread Running
Child Thread Running
Child Thread Running
Child Thread Running
Child Thread Running
Child Thread Running
Child Thread Running
Child Thread Running
Main Thread Running
Main Thread Running
Main Thread Running
Main Thread Running
Main Thread Running
Main Thread Running
Main Thread Running
Main Thread Running
Main Thread Running
