
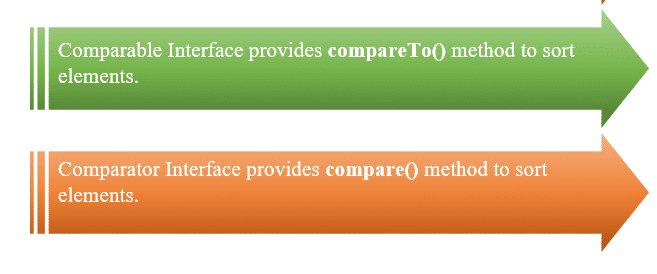
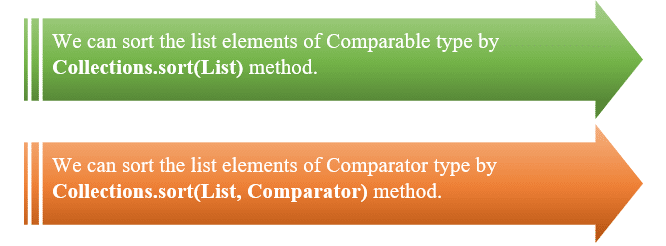
How Comparator useful over comparable
Let’s see how Comparator is useful over comparable, Let’s discuss them with different examples.
comparator vs comparable
Changes in Main Class: As you know Comparable must be implemented in your class for which you want to perform Sorting. So, you need to change your class.
public class Student implements Comparable { // Body of Class }
For more details on the example please click here.
But to use the Comparator interface you do not need to change the original class. You can create a separate class for sorting. It provides ease to programmers. For more details of the example please click here.
Single Vs Multiple Sorting: If you have only single sorting criteria then you should go with Comparable. Here the meaning of the single sort is, that you want to Sort your data only based on a single data member. Let’s say you want to sort Student data based on roll number only. For more details on the example please click here.
But if you have more than one sorting criteria then you should go with Comparator. Here mean of multiple sorts is, that you want to Sort your database of multiple data members. Let’s say you want to sort Student data based on roll number, Age, rank, etc. So, you can perform multiple Sorting based on requirements. For more details on the example please click here.
Flexibility: As you know Comparable is implemented only in the main class. So, you can’t add more criteria later. If want to add any criteria later then you have made changes to the existing class.
But by use, you can add sorting criteria later easily because you do not need to modify the existing ones. You have to take another class for new criteria. So, Comparator provides flexibility.
User choice: By use of Comparable you can sort elements to their natural order.
But by use of Comparator, you can sort the order in different from the natural order. Here is an example of how you can change order.
import java.util.ArrayList; import java.util.Collections; import java.util.Comparator; class Student { int rollNo; String name; int age; Student(int rollno,String name,int age) { this.rollNo=rollno; this.name=name; this.age=age; } } class RollNoComparator implements Comparator<Student> { @Override public int compare(Student student1, Student student2) { if(student1.rollNo==student2.rollNo) return 0; else if(student1.rollNo>student2.rollNo) return 1; else return -1; } } public class ExampleOfComparable { public static void main(String args[]) { ArrayList<Student> listOfStudent = new ArrayList<Student>(); listOfStudent.add(new Student(1,"Ravi",26)); listOfStudent.add(new Student(2,"kant",27)); listOfStudent.add(new Student(3,"kamboj",20)); // It Sorts all the objects based on RollNo Collections.sort(listOfStudent, new RollNoComparator().reversed()); for(Student student:listOfStudent) { System.out.println("RollNo of Student = "+student.rollNo); System.out.println("Age of Student = "+student.age); System.out.println("Name of Student = "+student.name); } } }
Output: RollNo of Student = 3
Age of Student = 20
Name of Student = kamboj
RollNo of Student = 2
Age of Student = 27
Name of Student = kant
RollNo of Student = 1
Age of Student = 26
Name of Student = Ravi
How comparable useful over Comparator?
What are the different scenarios in which we should use the comparable and comparator in java.
Usage of Java function Arays.sort() and Collection.sort(): If you are using Comparable for Sorting then you can use Arrays.sort() and Collections.sort() for sorting directly. You do not need to provide a comparator. You just need to call the method.
Provide ease for TreeMap and TreeSet: Here I want to suggest you please read TreeMap and TreeSet. Because TreeMap and TreeSet also use the Comparator and Comparable.
If your implementing a Comparable interface in your class, then objects of that class can be used as keys in a TreeMap or as elements in a TreeSet. But if you are using the Comparator interface then you have to write a separate Comparator and pass it in the constructor of TreeMap or TreeSet. For more details click here (TreeSet with Comparator and TreeMap with Comparator).
Now we will see how can use comparable with TreeSet:
import java.util.TreeSet; class SortedString implements Comparable<String> { String string1; SortedString(String s) { this.string1 = s; } @Override public int compareTo(String string2) { return string1.compareTo(string2); } } public class ExampleOfTreeSet { public static void main(String arg[]) { TreeSet<String> listOfNames = new TreeSet<String>(); listOfNames.add("RAVI"); listOfNames.add("JAVA"); listOfNames.add("GOAL"); listOfNames.add("SITE"); for(String name : listOfNames) { System.out.println(name); } } }
Output: GOAL
JAVA
RAVI
SITE
The number of classes: As you know to implement the Comparator interface you have to provide extra classes. There is some situation where you do not want to write the extra class you should go with a Comparable interface. Because Comparable does not require the creation of extra classes.
For more details of examples please here for Comparable and Comparator.