In java, Interface is part of abstraction in java and it is one of the strong pillars of OOP. We have already covered a lot of things about Interface in a recent post. Java 8 allows defining default and static methods in the interface. If you want to know, why the default method was introduced in java you can read it here. In this post, we will how java 9 allows us to define the private method and what is the need for the private methods in the interface.
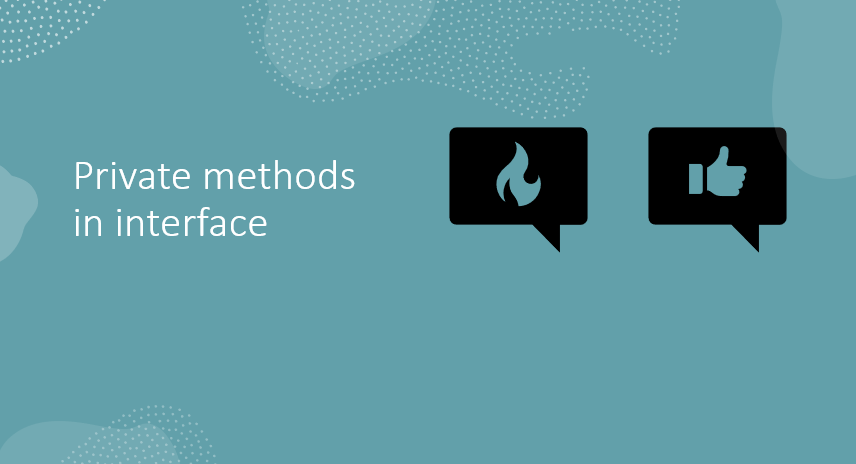
Before moving further let’s see the evolution of the Java interface.
Java 7 and earlier version: Java 7 and before java 7, we can have only two things in the interface i.e. Constants and Abstract methods. All the abstract methods of interface MUST be implemented by classes that choose to implement the interface.
- Constant variables
- Abstract methods
Java 8: Java 8 allows us to define default and static methods in the interface. But default method was introduced for a special purpose. The purpose was to handle the existing implantation of the JDK class. You can read what is needed for default methods.
- Constant variables
- Abstract methods
- Default methods
- Static methods
Java 9: Java 9 allows us to define private methods in the interface. It means now encapsulation is possible in interface also. But we should know what the need for private methods in the interface is and when we should use them. Let’s take an example:
- Constant variables
- Abstract methods
- Default methods
- Static methods
- Private methods
- Private Static methods
Suppose we have a record of Student. Here we have two default methods in the interface.
interface Student { default void college() { System.out.println("Student class"); System.out.println("Student Rollno"); System.out.println("Student College name"); } default void game() { System.out.println("Student class"); System.out.println("Student Rollno"); System.out.println("Student game name"); } public void show(); } public class StudentRecord implements Student { public static void main(String args[]) { StudentRecord obj = new StudentRecord(); obj.college(); obj.game(); obj.show(); } @Override public void show() { System.out.println("All information"); } }
Output: Student class
Student Rollno
Student College name
Student class
Student Rollno
Student game name
All information
Here you can see both methods have some common code. We can place the common code in one method that will be a private method.
Example in Java 9 – Default methods sharing common code using private methods
Let’s see how we will introduce a private method to share the common code.
interface Student { private void classDetails() { System.out.println("Student class"); System.out.println("Student Rollno"); } default void college() { classDetails(); System.out.println("Student College name"); } default void game() { classDetails(); System.out.println("Student game name"); } public void show(); } public class StudentRecord implements Student { public static void main(String args[]) { StudentRecord obj = new StudentRecord(); obj.college(); obj.game(); obj.show(); } @Override public void show() { System.out.println("All information"); } }
Output: Student class
Student Rollno
Student College name
Student class
Student Rollno
Student game name
All information
Rules for using the private method in interface
- We can’t make the Private method an abstract method. It means we can’t use abstract keywords with a private method.
- The private method can be a static method as well. It means a private method can be static and non-static.
- We should use a private modifier to define these methods and have no lesser accessibility than private modifiers.