The Abstract Factory design pattern is also part of the Creational pattern like the Factory design pattern. If you are not familiar with the Factory design pattern? Then you should read it first because the Abstract Factory pattern is almost similar to the Factory Pattern, and it just adds an abstraction layer over the factory pattern. Abstract Factory patterns are also called the factory of factories.
Here is the table content of the article will we will cover this topic.
1. Abstract factory design pattern?
2. Why do we use abstract factory design patterns?
3. Steps to create abstract factory pattern in java?
4. Abstract factory design pattern real–time example?
5. Abstract Factory Pattern Benefits?
What is abstract factory design pattern?
An abstract factory pattern is also known called the Factory of Factories because it is a super factory that creates other factories. It provides an interface that is responsible for creating a factory of related objects without explicitly specifying their classes. The generated factory gives the objects related to the factory. It means the abstract factory pattern is an extended version of the factory pattern that adds one more layer.
The Abstract Factory pattern provides a structure so that we can remove the huge number of if-else blocks and have a factory class for each sub-class. The Abstract Factory class will return the objects from the sub-factory. Let’s see how we can implement it.
Why do we use abstract factory design patterns?
Suppose we have an interface Mobile. Here we can have android mobile and Apple mobile. Each has a different configuration according to the operating system. If we create the only factory that will produce both types of mobile with different models we have to put a lot of if-else or switch conditions like factory design pattern. In the future, if we get a new requirement, then we will put more checks in the code and make it complex.
Instead of this, we can use an Abstract design pattern and resolve the problem.
Steps to create abstract factory design in java
1. Create an Abstract class that will be extended by all sub-factories.
2. Each generated factory(Sub factory) creates the object of the Concrete class.
3. We will get the object of the concrete class by generated factories and each generated factory gets from the main factory.
Abstract factory design pattern real-time example
To resolve the above problem, we will use an abstract factory pattern. We will create two different factories that will produce different types of Mobile. One factory will be AndroidMobileFactory, and another is AppleMobileFactory.The FactoryProducer class will produce the factory based on input.
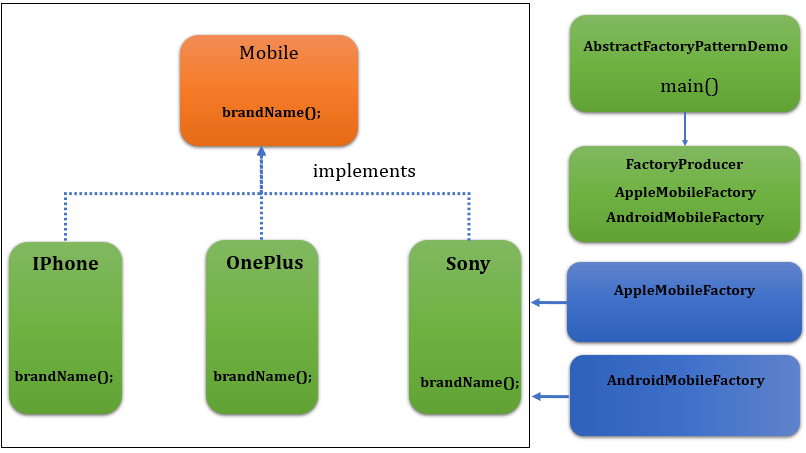
interface Mobile { String IPHONE = "IPhone"; String ONEPLUS = "OnePlus"; String SONY = "Sony"; void brandName(); } class Iphone implements Mobile { @Override public void brandName() { System.out.println("Iphone"); } } class OnePlus implements Mobile { @Override public void brandName() { System.out.println("OnePlus"); } } class Sony implements Mobile { @Override public void brandName() { System.out.println("Sony"); } } abstract class AbstractFactory { abstract Mobile getMobile(String model) ; } class AppleMobileFactory extends AbstractFactory { @Override public Mobile getMobile(String model){ if(model.equalsIgnoreCase("iphone")){ return new Iphone(); } return null; } } class AndroidMobileFactory extends AbstractFactory { @Override public Mobile getMobile(String brand) { if(brand.equalsIgnoreCase("Oneplus")) { return new OnePlus(); } else if(brand.equalsIgnoreCase("Sony")) { return new Sony(); } return null; } } class FactoryProducer { public static AbstractFactory getFactory(boolean isApple) { if(isApple) { return new AppleMobileFactory(); } else { return new AndroidMobileFactory(); } } } public class AbstractFactoryPatternDemo { public static void main(String[] args) { AbstractFactory mobileFactory1 = FactoryProducer.getFactory(false); Mobile oneplus = mobileFactory1.getMobile("Oneplus"); oneplus.brandName(); Mobile sony = mobileFactory1.getMobile("Sony"); sony.brandName(); AbstractFactory mobileFactory2 = FactoryProducer.getFactory(true); Mobile iphone = mobileFactory2.getMobile("iphone"); iphone.brandName(); } }
Abstract Factory Pattern Benefits
1. The Abstract Factory pattern provides the control to create an object of classes. Also, It takes responsibility and the process of creating product objects. Clients manipulate instances through their abstract interfaces. So, The concrete class’s name is isolated in the implementation of the concrete factory.
2. The concrete factory or generated factory uses once in an application to instantiate itself. That provides an ease to make any change in an application uses. Hence, It can use various product configurations simply by changing the concrete factory.
3. It provides flexibility because it is a “factory of factories” and can be easily extended according to requirements. We can add more concrete and factories later.
4. It avoids the tight coupling between concrete products and client code.
Some genuinely prize articles on this web site , saved to bookmarks . I found this similiar one here
I am going to go ahead and bookmark this article for my brother for their study project for school. This is a sweet internet site by the way. Where do you acquire the design for this web page?