A program is a list of instructions or blocks of instructions. Java provides Control structures that can change the path of execution and control the execution of instructions. In this post, we will discuss the Control structures in programming language.
Here is the table content of the article.
Three kinds of control structures in Java?
1. Control statements/Conditional Branches?
i ) if statement in java
ii ) nested if statement in java
iii ) if-else statement in java
iv ) if-else if statement/ ladder if statements in java
v ) switch statement in java
2. Loops in Java?
3. Branching Statements in Java?
Three kinds of control structures in Java
1. Control statements in java / Conditional Branches in java
Java control statements use to choose the path for execution. There are some types of control statements:
if statement
It is a simple decision-making statement. It uses to decide whether the statement or block of statements should be executed or not. A Block of statements executes only if the given condition returns true otherwise the block of the statement skips. The if statement always accepts the boolean value and performs the action accordingly. Here we will see the simplest example of an if statement. We will discuss it in detail in a separate post. Visit here for more details.
public class IfStatementExample { public static void main(String arg[]) { int a = 5; int b = 4; // Evaluating the expression that will return true or false if (a > b) { System.out.println("a is greater than b"); } } }
Output: a is greater than b
nested if statement
The nested if statements mean an if statement inside another if statement. The inner block of the if statement executes only if the outer block condition is true. This statement is useful when you want to test a dependent condition. Here we will see the example of nested if with a common example and see how it works. Visit here for more details.
public class NestedIfStatementExample { public static void main(String arg[]) { int age = 20; boolean hasVoterCard = true; // Evaluating the expression that will return true or false if (age > 18) { // If outer condition is true then this condition will be check if (hasVoterCard) { System.out.println("You are Eligible"); } } } }
Output: You are Eligible
if-else statement
In the if statement, the block of statements executes when the given condition returns true otherwise block of the statement skips. Suppose when we want to execute the same block of code if the condition is false. Here we come on the if-else statement. In an if-else statement, there are two blocks one is an if block and another is an else block. If a certain condition is true, then if block executes otherwise else block executes. Let’s see the example and understand it. Visit here for more details.
public class IfElseStatementExample { public static void main(String arg[]) { int a = 10; int b = 50; // Evaluating the expression that will return true or false if (a > b) { System.out.println("a is greater than b"); } else { System.out.println("b is greater than a"); } } }
Output: b is greater than a
if-else if statement/ ladder if statements
If we want to execute the different codes based on different conditions then we can use if-else-if. It is known as if-else if ladder. This statement executes from the top down. During the execution of conditions if any condition is found true, then the statement associated with that if statement is executed, and the rest of the code will be skipped. If none of the conditions returns true, then the final else statement executes. Let’s see the example of the ladder if and you can visit here for more details.
public class IfElseIfStatementExample { public static void main(String arg[]) { int a = 10; // Evaluating the expression that will return true or false if (a == 1) { System.out.println("Value of a: "+a); } // Evaluating the expression that will return true or false else if(a == 5) { System.out.println("Value of a: "+a); } // Evaluating the expression that will return true or false else if(a == 10) { System.out.println("Value of a: "+a); } else { System.out.println("else block"); } } }
Output: Value of a: 10
Switch statement
The switch statement in java is like the if-else-if ladder statement. To reduce the code complexity of the if-else-if ladder switch statement comes.
In a switch, the statement executes one from multiple statements based on condition. In the switch statements, we have a number of choices and we can perform a different task for each choice. You can read it in detail, for more details.
public class SwitchStatementExample { public static void main(String arg[]) { int a = 10; // Evaluating the expression that will return true or false switch(a) { case 1: System.out.println("Value of a: 1"); break; case 5: System.out.println("Value of a: 5"); break; case 10: System.out.println("Value of a: 10"); break; default: System.out.println("else block"); break; } } }
Output: Value of a: 10
2. Loops in Java / Looping statements in java
The loop in java uses to iterate the instruction multiple times. When we want to execute the statement a number of times then we use loops in java.
for loop
A for loop executes the block of code several times. It means the number of iterations is fixed. JAVA provides a concise way of writing the loop structure. When we want to execute the block of code serval times, let’s see the example and visit here for more details.
public class ForLoopExample { public static void main(String arg[]) { // Creating a for loop for(int i = 1; i <= 3; i++) { System.out.println("Iteration number: "+i); } } }
Output: Iteration number: 1
Iteration number: 2
Iteration number: 3
while loop
A while loop allows code to execute repeatedly until the given condition returns true. If the number of iterations doesn’t fix, it recommends using a while loop. It is also known as an entry control loop. For more details.
public class WhileLoopExample { public static void main(String arg[]) { // Creating a while loop int i = 1; while(i <= 3) { System.out.println("Iteration number: "+i); i++; } } }
Output: Iteration number: 1
Iteration number: 2
Iteration number: 3
do-while loop
It is like a while loop with the only difference being that it checks for the condition after the execution of the body of the loop. It is also known as Exit Control Loop. For more details.
public class DoWhileLoopExample { public static void main(String arg[]) { // Creating a while loop int i = 1; do { System.out.println("Iteration number: "+i); i++; } while(i <= 3); } }
Output: Iteration number: 1
Iteration number: 2
Iteration number: 3
3. Branching Statements in Java
These use to alter the flow of control in loops.
If a break statement encounters inside a loop, the loop immediately terminates, and the control of the program goes to the next statement following the loop. It is used along with the if statement in loops. If the condition is true, then the break statement terminates the loop immediately.
public class BreakStatementExample { public static void main(String arg[]) { for(int i = 0; i <=3; i++) { System.out.println("Iteration number:" + i); if(i == 2) break; } } }
Output: Iteration number:0
Iteration number:1
Iteration number:2
continue
The continue statement uses in a loop control structure. If you want to skip some code and need to jump to the next iteration of the loop immediately. Then you can use a continue statement. It can be used in for loop or a while loop. Read it in detail
public class ContinueStatementExample { public static void main(String arg[]) { for(int i = 0; i <=3; i++) { if(i == 1) continue; System.out.println("Iteration number:" + i); } } }
Output: Iteration number:0
Iteration number:2
Iteration number:3
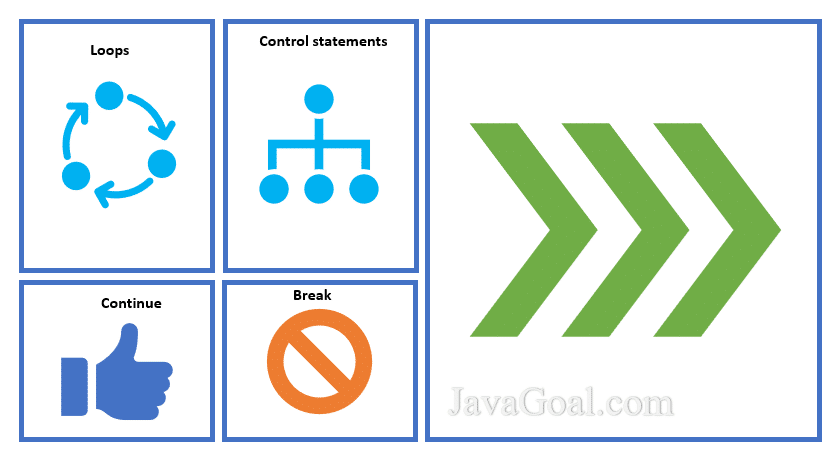