As you already know lambda expression is used only to implement the Functional interfaces. Whenever you are using a lambda expression then a reference of the interface will be created. Here we will see how lambda expression works in java.
Here is the table content of the article will we will cover this topic.
1. First Approach without Lambda expression?
2. Second Approach with Lambda?
3. How can use multiple parameters in lambda expression?
4. How can use if you have multiple statements in lambda expression?
5. Why does Lambda expression use functional interface only?
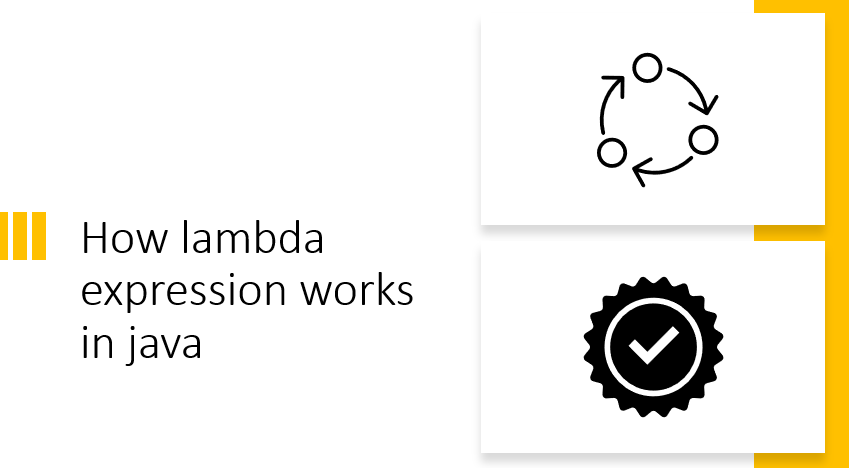
InterfaceName reference = (parameters) () -> { Statements; }
Firstly, the compiler will execute the right-hand side and after that assign it to reference.
The above statement is the implementation of the abstract method of functional interface. This function is anonymous because it is without a name. The anonymous function can create only whenever it needs.
To understand the working of lambda expression and how the anonymous function works? We will discuss it with two approaches. The first approach goes without lambda and the second with lambda.
First Approach without Lambda expression
public interface Records { public void displayAllRecords(); }
public class ExampleWithoutLambdaExpression { public static void main(String[] args) { Records records = new Records() { @Override public void displayAllRecords() { System.out.println("This method showing all the records of data"); } }; records.displayAllRecords(); } }
Output: This method showing all the records of data
In the above example, we are providing body to abstract method displayAllRecords() and a reference(records) of Record Interface has been created. Now by using the reference variable we are calling the method displayAllRecords().
So here we did two things the first one created a reference for Records Interface and the second provide implementation to the method by use of the anonymous class. If you don’t know anonymous implementation, please click here.
Second Approach with Lambda
public interface Records { public void displayAllRecords(); }
public class ExampleWithLambdaExpression { public static void main(String[] args) { Records records = () -> System.out.println("This method showing all the records of data"); records.displayAllRecords(); } }
Output: This method showing all the records of data
In the above example, we are using a lambda expression. We are providing the implementation of the abstract method. Whenever a lambda expression is used it means you are providing body to the abstract method of function interface.
Records records = () -> System.out.println("This method showing all the records of data");
1. By use of lambda expression, you don’t need to specify the method name again. It
is automatically understood because the Function interface can have only one
abstract method.
2. As you can see, we provided the body with the method without any function name.
So, it’s called an anonymous function.
3. A reference(records) of the Record Interface has been created. Now by using reference
variable, we are calling method displayAllRecords().
Let’s discuss some more examples of how lambda expression works in java:
1. How can use multiple parameters in lambda expression
interface Records { public void displayRecords(int a, int b); }
public class ExampleWithLambdaExpression { public static void main(String[] args) { Records records = (int c, int d) -> System.out.println("First Record:"+c+" Second Record:"+d); records.displayRecords(5, 10); } }
Output: First Record:5 Second Record:10
2. How can use it if you have multiple statements in the lambda expression
interface Records { public void displayRecords(int a, int b); }
public class ExampleWithLambdaExpression { public static void main(String[] args) { Records records = (int c, int d) -> { System.out.println("First Record:"+c); System.out.println("Second Record:"+d); }; records.displayRecords(5, 10); } }
Output: First Record:5
Second Record:10
Why does Lambda expression use functional interface only?
As you know functional programming is supported in JAVA 8 with the help of Lambda expression. The Lambda expression supported the Functional interface. These interfaces also are known as the Single Abstract method(SAM). The Lambda expressions support only those interfaces which have only one abstract method. It provides a body to the abstract method and is assigned as the reference of the interface.
Whenever you are using the lambda expression for the Function interface, the compiler strictly ensures the interface has only one abstract method. If the interface contains more than one abstract method the program shows an error. Because the lambda expression provides body to only a single abstract method. When you write lambda expression the compiler assumes the statements of lambda expression will be the body of the abstract method.
public interface Display { public void show(); }
public class ExampleWithLambda { public static void main(String[] args) { Display display = () -> { System.out.println("In Show interface we have only Single abstract method"); System.out.println("This is the body of abstract method"); }; display.show(); } }
Output: In Show interface we have only Single abstract method
This is the body of abstract method
So, let’s add another abstract method to the interface and try to implement it with Lambda expression.
public interface Display { public void show(); public void data(); }
public class ExampleWithLambda { public static void main(String[] args) { Display display = () -> { System.out.println("In Show interface we have only Single abstract method"); System.out.println("This is the body of abstract method"); }; display.show(); } }
Output: Exception in thread “main” java.lang.Error: Unresolved compilation problem: The target type of this expression must be a functional interface at java_8.ExampleWithLambda.main(ExampleWithLambda.java:7)
It breaks at the compile time when you try to refer to the interface. To stop these things, you could add an annotation FunctionalInterface explicitly.