In a recent post, we have seen what is a string in java and how many ways to create a string. When we create a string by use of the String class, the JVM always creates an immutable string object, which means the string is immutable in java. But what is the meaning of an immutable string object? How it differs from other objects? In this post, we will see all the details of the java string immutable objects and how does it work in memory? After that, we will read Why string is immutable in java?
Here is the table content of the article we will cover these topics.
1. What is an immutable string?
2. Why string is immutable in java?
What is an immutable string?
Immutable means unchanging over time or unable to be changed. Java defines some objects as immutable objects; developers can’t modify those objects once they are created. The meaning of immutable is unchangeable or unmodifiable. It means after the creation of the string we can’t change the value in the same object. But if you try to change in value, The JVM creates a new String object with the new value.
Whenever we create a string in java either as a string literal or by use of the new keyword. The JVM creates a string object in memory. The string object created in memory is immutable. We can’t change the value of the string in the same object. If you are making any change in a string, then the compiler will create a new string instead of a modification in the string.
class ExampleStringImmutable { public static void main(String args[]) { String studentName = "Ravi"; studentName.concat("kant"); System.out.println("My name is = "+ studentName); } }
Output: My name is = Ravi
Memory representation: In the above example, we created a string studentName with value “Ravi”.
String studentName = “Ravi”;
After the execution of the above statement, the compiler will create a string in String constant pool. String constant pool is part of heap memory.

After the creation of the string, we are concatenating some other string.
studentName.concat(“kant”);
After the execution of the above statement, the compiler will create a new object of string. but this object doesn’t have any reference variable.
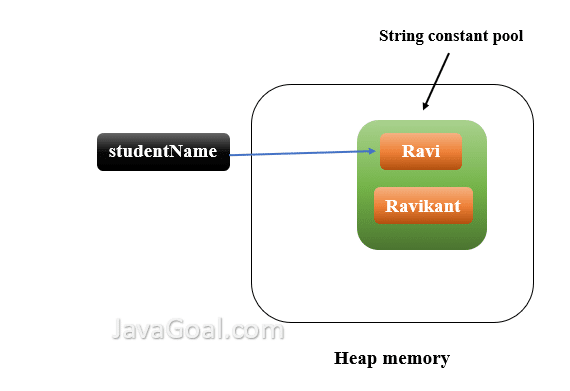
As you see in the above example the reference variable studentName is still referred to as “Ravi”. You can explicitly assign the string to the reference variable.
class ExampleStringImmutable { public static void main(String args[]) { String studentName = "Ravi"; studentName = studentName.concat("kant"); System.out.println("My name is = "+ studentName); } }
Output: My name is = Ravikant

Why string is immutable in java?
The most programmer knows string is immutable in java and we can’t change its value within the same object. If we change its value, the JVM creates a new object of string. We have discussed it in a recent post that is the immutable string in java. But still, there is a popular question of why string is immutable in java. In java there are multiple reasons to design a string is immutable in java.
Why string is immutable in java?
String in java is a final class and immutable class, so it can’t be inherited, and we can’t alter the object. There are a number of factors that were considered when the string was introduced in java. We will discuss them one by one and need the concept of memory, synchronization, data structures, etc.
1. Requirement of String Pool
In Java, the String is the most widely used data structure. The string constant pool is special storage in heap memory that Caching the String literals and reuses them. Whenever we create a string, the JVM checks whether the string already exists in the string constant pool or not. If the string is already presented the reference of the existing string will be returned, instead of creating a new object. Let’s see how to string constant pool works in memory. Here we will create three strings with the same and different reference variables.
String str1 = "JavaGoal"; String str2 = "JavaGoal"; String str3 = "JavaGoal";

Suppose the string is not immutable and here three reference variables pointing to the same object. If we change the string with one reference will lead to the wrong value for the other references. It creates problems and affects all other references.
2. Cache of Hashcode
As we know string objects have a large use in java. The hashcode() implementation of the string is widely used in java like HashMap, HashTable, HashSet, etc. The hashcode() method returns the value of a particular string object. As we know strings are very popular as HashMap keys, The immutable string gives guarantees that its value will not be changed. It means, there is no need to calculate the hashcode every time it is used.
3. Security
In java, security parameters are also represented as String like in network connections, opening files, database connection URLs, usernames, and passwords, etc. Suppose the string is mutable then any hacker could change the referenced value to break the security.
4. Synchronized
Due to the immutable nature of string, it is thread-safe. Suppose multiple threads are running but they can’t be changed because if a thread changes the value, then instead of modifying the same, a new String would be created in the String pool. This eliminates the requirements of doing synchronization.
5. Performance
As we have seen the string constant pool enhances memory storage and performance. Since String is the most widely used data structure, improving the performance
6. Classloader
The String object is also used as an argument for class loading. Due to the immutability of the string the correct class is getting loaded by Classloader.
After reassigning the studentName variable. whatever output you printing is not correct.
Here:
studentName = studentName.concat(“kant”);
System.out.println(“My name is = “+ studentName);
Please modify the output as “Ravikant”
Thankyou Narendra Kumar
I have corrected my typo mistake. 🙂
Agree
my question is – Now u concat the ravikanth, assign to studentName . then the value is changed now . and refer to studentName .
Q1- how to get the old value – ravi
Q2 – how it is achieve the it is immutable , any one can modify and refer the actual reference (studentName). when ever we wang print raviKant will display .
Can you little clarify me .
How
String s=”sushil”;
s=”Ravi”;
s=”sushil”;
String s1=new String (“Sushil”);
s1=s;
How may object will create in heap and string constant pool . Can you please clarify me memory point of view .
Here is your answer: https://javagoal.com/string-class-in-java/
If you will need any assistance the text me through live chat option or you can comment also.