In this article, We will learn what is java array and how to work with it. We will learn array declaration in java, java initialize an array, and access array elements with the help of examples, and flowcharts.
Here is the table content of this article we will cover all the parts of this topic.
1. What is an Array in Java?
2. Important things about Array?
3. Types of Array
4. One-dimensional array in Java?
5. Two-dimensional array in java?
7. Array of objects in java?
8. Arrayindexoutofboundsexception in java?
9. Jagged array in java?
10. Array default value in java?
11. Array interview program in java?
What is an Array in Java?
An array is a container that can hold multiple values of the same data type. One array object can’t hold values of different data types. In other words, we can say the array is a collection of similar types of elements that have a contiguous memory location.
For example: If you want to hold 50 integer values, then you can create an array that can hold 50 values of int type. Instead of declaring individual variables, such as a1, a2, …, and a49, you declare one array variable that holds all the 50 values and you can access the values of an array by using a[0], a[1], and …, a[49].
Real-time Example: Let’s say, you want to store the ID proof of 10 students. Then you can declare an array that can hold int-type values. You don’t need to declare 10 individual variables of int type.
public class JavaArray { public static void main(String args[]) { //Declaring 10 variables to store the 10 values int idProof1 = 11; int idProof2 = 2; int idProof3 = 123; int idProof4 = 042; int idProof5 = 015; int idProof6 = 026; int idProof7 = 037; int idProof8 = 034; int idProof9 = 01; int idProof10 = 9; System.out.print("Values from variables:"); System.out.print(idProof1 + " "); System.out.print(idProof2 + " "); System.out.print(idProof3 + " "); System.out.print(idProof4 + " "); System.out.print(idProof5 + " "); System.out.print(idProof6 + " "); System.out.print(idProof7 + " "); System.out.print(idProof8 + " "); System.out.print(idProof9 + " "); System.out.print(idProof10 + " "); System.out.println(); System.out.print("Values from variables:"); // Declaring an array to store the 10 values int idProofs[] = { 11, 2, 123, 042, 015, 026, 037, 034, 01, 9}; for(int i = 0; i < idProofs.length; i++) System.out.print(idProofs[i] + " "); } }
Output: Values from variables:11 2 123 34 13 22 31 28 1 9
Values from variables:11 2 123 34 13 22 31 28 1 9
Important things about Java Array
- An array can hold the value of the same data type. It never allows keeping values of a different datatype. If you are declaring an array of int type it means it can hold only its values. It can’t take a value of String type etc.
- An array is stored in a contiguous memory allocation. All the values are stored in order and each value is placed on an index that starts from 0.
- You can declare an Array as local if you are declaring it in the method.
- The size of the array is specified only by int value. You can’t use any other data type to specify the size of the array.
- Like other classes, Array also extends its superclass Object.
- Every array type implements the interfaces Cloneable and java.io.Serializable.
- An array can store primitive data types and Non-primitive data types.
Video in Hindi – Java Programming Goal
Types of Array in java
- One-dimensional array in java or a single-dimensional array
- Two-dimensional array in java or 2d array in java
One-dimensional array in java
Here is the table content for the one-dimensional array
i. Declaration of One-dimensional array in java?
ii. Construction of a One-dimensional array in java?
iii. Memory Representation After Construction?
iv. Initialization of One-dimensional array in java?
v. Memory Representation after Initialization?
vi. Example of an Array
vii. How to get elements from a One-dimensional array in java
In java, the One Dimensional Array always uses only one subscript([]). A one-dimensional array in java behaves like a list of variables. You can access the variables of an array by using an index in square brackets preceded by the name of that array. The index value should be an integer.
Steps:
- Declaration of Array
- Construction of Array
- Initialization of Array
Declaration of One-dimensional array in java
Before using the array, we must declare it. Like normal variables, we must provide the data type of array and the name of an array. Data type means the type of elements that we want to store in the Array. So, we must specify the data type of array according to our needs. We also need to specify the name of an array so that we can use it later by name.
datatype[] arrayName; Or datatype arrayName[]; Or datatype []arrayName;
- the datatype can be a primitive data type(int, char, Double, byte, etc.)or Non-primitive data (Objects).
- the arrayName is the name of an array
- [] is called a subscript.
int[] number; Or int number[]; or int []number;
Construction of One-dimensional array in java
For the creation of an array, a new keyword is used with a data type of array. You must specify the size of the array. The size should be an integer value or a variable that contains an integer value. How many elements you can store in an array directly depends upon the size of an array.
arrayName = new DataType[size];
new Datatype[size]: It creates an array in heap memory. Because an array is an object, it is stored in heap memory.
arrayName: The assignment operator assigns the newly created array to the reference of variable arrayName. We can access the elements of an array by use of array names.
number = new int[10]; // allocating memory to array
In this example new int[10] creates a new array of int types in heap memory. The assignment operator assigns the array object to the reference variable number. Now you can access the array by use of the number. You can access each value from the array by using numbers with subscript([]). We have to use only one subscript([]) for the one-dimensional array in java.
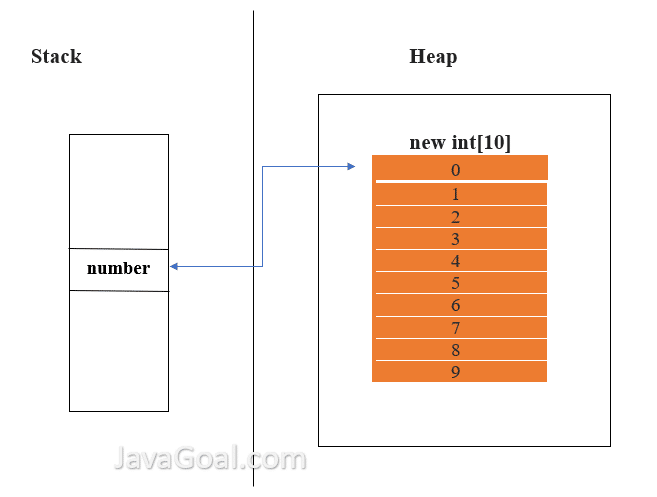
Note: When we are creating a new array the elements in the array will automatically be initialized by their default values. For e.g. : zero (int types), false (boolean), or null (for object types).
Memory Representation after Construction
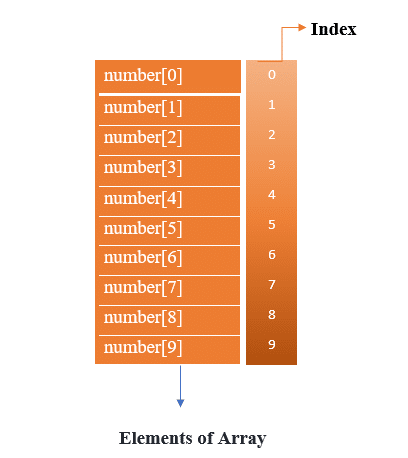
The index of an array starts from 0 and ends with length-1. The first element of an array is number[0], the second is number[1], and so on. If the length of an array is n, the last element will be arrayName[length-1]. Since the length of the number array is 10, the last element of the array is number[9].
Initialization of One-dimensional array in java
To initialize the Array we have to put the values at each index of the array. In the above section, we have created an Array with the size of 10. So now we will see how we can add values in Array.
public class MyExample { public static void main(String[] args) { // Declaration of Array int[] number; // Construction of Array with given size // Here we are giving size 10 it mean it can hold 10 values of int type number = new int[10]; // Initialization of Array number[0] = 11; number[1] = 22; number[2] = 33; number[3] = 44; number[4] = 55; number[5] = 66; number[6] = 77; number[7] = 88; number[8] = 99; number[9] = 100; //Print the values from Array for(int i = 0; i < number.length; i++) System.out.print(number[i] + " "); } }
Output: 11 22 33 44 55 66 77 88 99 100
You can also construct an array by initializing the array at declaration time. If you are initializing the array it is another way to construct an array.
dataType arrayName[] = {value1, value2, …valueN}
Some points about Initialization:
- If you choose the datatype of an array as int then values should be int type. It means a type of value is totally dependent on the datatype of an array.
- Value1 will be stored at 0 indexes, value2 in 1 index, and so on.
- The size of the array depends upon how many values you are providing at the time of initialization.
int number[] = {11, 22, 33 44, 55, 66, 77, 88, 99, 100}
It’s created an array of int types as you see we are initializing it during declaration.
Memory Representation after Initialization

Example of an Array
Here we will see different examples of Java Array and How to use array in java. We will also see how to initialize an array with values java (java initialize array with values).
So Let’s pick the first example, Here we will show how we can create the array and initialize the values at the same time. Here we will do two operations in single-step i.e. array declaration and initialization in java.
public class MyExample { public static void main(String[] args) { // Creating a int Array with with values int[] number = new int[] { 11, 22, 33, 44, 55, 66, 77, 88, 99}; System.out.println("Print int Array:"); for(int i = 0; i < number.length; i++) { System.out.println("Values on index "+ i + ": " + number[i]); } // Creating a String Array with with values String[] names = new String[] { "Java", "Goal", "Learning", "Website", "for", "Java", "Concepts"}; System.out.println("Print String Array:"); for(int i = 0; i < names.length; i++) { System.out.println("Values on index "+ i + ": " + names[i]); } } }
Output: Print int Array:
int Array values on index 0: 11
int Array values on index 1: 22
int Array values on index 2: 33
int Array values on index 3: 44
int Array values on index 4: 55
int Array values on index 5: 66
int Array values on index 6: 77
int Array values on index 7: 88
int Array values on index 8: 99
Print String Array:
String Array values on index 0: Java
String Array values on index 1: Goal
String Array values on index 2: Learning
String Array values on index 3: Website
String Array values on index 4: for
String Array values on index 5: Java
String Array values on index 6: Concepts
Here we are creating an array and initializing the values. The size of the array depends on the number of elements. The JVM allocates the size to the array is equal to the number of elements presented in braces. The first element stores at index 0 and the last element stores at length – 1.
How to get elements from a One-dimensional array in java
You can access an array’s element with the name of the array variable, followed by brackets([]). You must specify an integer value in brackets([]) called the index of an array.
arrayName[subscript];
class AccessOfArray { public static void main(String[] args) { int[] number= {11, 22, 33, 44, 55, 66, 77, 88, 99, 100}; int length = number.length; // get size of array for (int i = 0; i < length; i++) { System.out.println("Index of element = " + i +" & Element is = " +number[i]); } } }
Output:
Index of element = 0 & Element is = 11
Index of element = 1 & Element is = 22
Index of element = 2 & Element is = 33
Index of element = 3 & Element is = 44
Index of element = 4 & Element is = 55
Index of element = 5 & Element is = 66
Index of element = 6 & Element is = 77
Index of element = 7 & Element is = 88
Index of element = 8 & Element is = 99
Index of element = 9 & Element is = 100
Accessing array elements in java
- To access any element from the array you must use an array name with a subscript and the subscript takes an index. Remember one thing here, an index should be always in an integer value.
- An array index value always starts from 0 and ends with the array’s length-1. So, when you are accessing an array you must take the length of an array. If you are trying to access an element, then the index value should be less than the length of an array.
- All the elements can access via loops in Java.
Changing elements of Array: You can assign a new value and replace the old value by using a subscript with an array name. To assign a value at the index, you can assign value by using an assignment statement:
arrayName[subscript] = value;
class ArrayClassExample { public static void main(String[] args) { // Initiation an array at declaration time String[] names = { "Ravi", "Ram"}; int length = names.length; // Print all names before changes for (int i = 0; i < length; i++) System.out.println("Names of Students = " + names[i]); // Changing value of elements names[0] = "Radhe"; // Print all names before changes for (int i = 0; i < length; i++) System.out.println("After changes names of Students = "+ names[i]); } }
Output:
Names of Students = Ravi
Names of Students = Ram
After changes names of Students = Radhe
After changes names of Students = Ram
Video in Hindi – Java Programming Goal
Two-dimensional array in java
Here is the table content for the two-dimensional array
i. Declaration of Two-dimensional array in java?
ii. Construction of Two-dimensional array in java?
iii. Memory Representation After Construction?
iv. Initialization of One-dimensional array in java?
v. Memory Representation after Initialization?
vi. How to get elements from a Two-dimensional array in java
A two-dimensional array(2-D) is the simplest form of a multidimensional array. A two-dimensional array is an array of arrays because they store in tabular form. Like a single-dimensional array, we must declare the two-dimensional array variable. We must specify the array name followed by two square brackets called subscript([][]).
A two-dimensional array is stored in the tabular form of the row-column matrix. The two-dimensional array uses two subscripts([][]) where the first index behaves like a row and the second index column. The size of the matrix depends upon the size of subscripts.
Steps:
- Declaration of Array
- Construction of Array
- Initialization of Array
Declaration of two-dimensional array Java
Like the one–dimension array, in two dimensional we provide the data type of array and name of the array for the declaration. Data type means which type of elements we want to store in the Array. In the two-dimensional array, we use the two subscripts for the declaration of the array. First subscript is used for ROW and the second for COLUMN.
datatype[][] arrayName; Or datatype arrayName[][]; Or datatype [][]arrayName;
int[][] number; Or int number[][]; Or int [][]number;
Here int is datatype and number is the name of the two-dimensional array. Two subscripts [][] are used to define a two-dimensional array.
Construction of two-dimensional array Java
Like a one-dimensional array, for the creation of a two-dimensional array, the new keyword is used with the data type of array. There are two subscripts for the size of an array, which is the size of the matrix. The first subscript is used to define the number of rows in the matrix and the second one is for the number of columns. You must specify the size of the array because how many number elements you can store in an array directly depends upon the size of the array.
arrayName = new DataType[size][size];
new Datatype[size][size] : It creates an array in heap memory. Because an array is an object, it is stored in heap memory. The first subscript size will define the number of rows and the second subscript used for the number of columns.
arrayName: Assignment operator assigns the newly created array to the reference of variable arrayName.
number = new int[3][3]; // allocating memory to array
Memory Representation after Construction
You can see in the example, the first and second subscript size is 3 which means it will create a 3X3 matrix.
Column 1 | Column 2 | Column 3 | |
Row 1 | number[0][0] | number[0][1] | number[0][2] |
Row 2 | number[1][0] | number[1][1] | number[1][2] |
Row 3 | number[2][0] | number[2][1] | number[2][2] |
The first element of array is number[0][0], second is number[0][1] and so on.
Initialization of two-dimensional array Java
You can provide value to an array at declaration time. Here is the syntax of the initialization of a two-dimensional array it differs from the one-dimensional array.
dataType arrayName[][] = { {value1, value2, …valueN}, {value1, value2, …valueN}, {value1, value2, …valueN}, };
It’s created an array and initializes it during declaration.
int[][] number = { {11, 22, 33}, {44, 55, 66}, {77, 88, 99}, };
Memory Representation after Initialization
In this example here, we are initializing a two-dimensional array. This array is holding the 9 elements of type int.
Column 1 | Column 2 | Column 3 | |
Row 1 | number[0][0]=11 | number[0][1]=22 | number[0][2]=33 |
Row 2 | number[1][0]=44 | number[1][1]=55 | number[1][2]=66 |
Row 3 | number[2][0]=77 | number[2][1]=88 | number[2][2]=99 |
A two-dimensional array is an array of array. You can see the structure of the array:
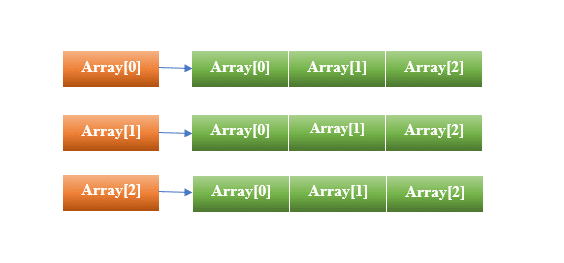
Let’s take an example of a two-dimensional array. In which we will declare and initialize the array.
public class ExampleOfTwoDimentionalArray { public static void main(String[] args) { // Creation of Array with initilization int[][] number = { {1, 2, 3}, {4, 5, 6}, {7, 8, 9}, }; String[][] names = { {"Hello1", "Hello2", "Hello3"}, {"Hello4","Hello5", "Hello6"}, {"Hello7", "Hello8", "Hello9"}, }; // Accessing of Array for(int i = 0; i < 3; i++) { for(int j = 0; j < 3; j++) { System.out.println(number[i][j]); System.out.println(names[i][j]); } } } }
Output: 1
Hello1
2
Hello2
3
Hello3
4
Hello4
5
Hello5
6
Hello6
7
Hello7
8
Hello8
9
Hello9
Let’s take another example of a one-dimensional array. Firstly we are declaring Array and after that initialize the array.
public class ExampleOfOneDimentionalArray { public static void main(String[] args) { // Creation of Array int[][] number; String[][] names; // Construction of Array number = new int[3][3]; names = new String[3][3]; // initilization of Array for(int i = 0; i < 3; i++) { for(int j = 0; j < 3; j++) { number[i][j] = i+j; names[i][j] = "Hello"+i+j; } } // Accessing of Array for(int i = 0; i < 3; i++) { for(int j = 0; j < 3; j++) { System.out.println(number[i][j]); System.out.println(names[i][j]); } } } }
Output:0
Hello00
1
Hello01
2
Hello02
1
Hello10
2
Hello11
3
Hello12
2
Hello20
3
Hello21
4
Hello22
How to get elements from a Two-dimensional array in java
In Java two – dimensional arrays are arrays of arrays, so arrayName[x][y] is an array x(size) of rows in arrays and y(size) of columns in the array. So, if you want to access any value from the array then you must specify the index of the row and column. These are also known as multidimensional array java.
class ArrayExample { public static void main(String args[]) { //declaration two dimensional array with initialization int number [][]={ {11,22,33}, {44,55,66}, {77,88,99} }; //print the value of two dimensional array for(int i = 0; i < 3; i++) { for(int j=0;j<3;j++) { System.out.println("Index of number is i = "+i+ " j = "+ j+ " and Element is = "+ number [i][j]+" "); } System.out.println(); } } }
Output:
Index of number is i = 0 j = 0 and Element is = 11
Index of number is i = 0 j = 1 and Element is = 22
Index of number is i = 0 j = 2 and Element is = 33
Index of number is i = 1 j = 0 and Element is = 44
Index of number is i = 1 j = 1 and Element is = 55
Index of number is i = 1 j = 2 and Element is = 66
Index of number is i = 2 j = 0 and Element is = 77
Index of number is i = 2 j = 1 and Element is = 88
Index of number is i = 2 j = 2 and Element is = 99
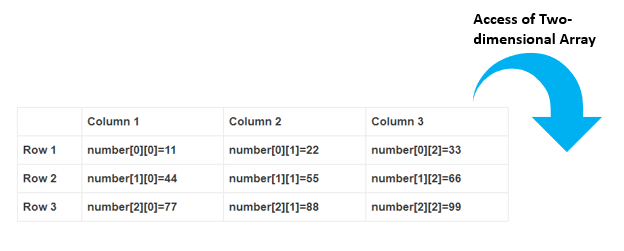
An array of objects in java
An array can store primitive or non-primitive data types. In the above example, we used the int (Primitive data type) type of array.
Now we will discuss the array of objects in java(non-primitive data types). Let’s say you want to store a record of students. Each Student has some information like rollNo, name, className, etc. So can store objects of Student in an Array. That’s why we call this Array of objects. In an Array of objects, each index value can store an object.
For example, we will create a class Student that holds information about a student. We want to hold a number of student records so we will use an array of objects in java.
public class Student { int rollNo; String name; String className; public int getRollNo() { return rollNo; } public void setRollNo(int rollNo) { this.rollNo = rollNo; } public String getName() { return name; } public void setName(String name) { this.name = name; } public String getClassName() { return className; } public void setClassName(String className) { this.className = className; } }
class StudentRecord { public static void main(String[] args) { Student[] recordOfStudent = new Student[3]; // First student record Student firstStudent = new Student(); firstStudent.setRollNo(1); firstStudent.setName("Ravi kant"); firstStudent.setClassName("MCA"); recordOfStudent[0] = firstStudent; // Second student record Student secondStudent = new Student(); secondStudent.setRollNo(2); secondStudent.setName("Rama"); secondStudent.setClassName("MCA"); recordOfStudent[1] = secondStudent; // Third student record Student thirdStudent = new Student(); thirdStudent.setRollNo(3); thirdStudent.setName("Radhe"); thirdStudent.setClassName("MCA"); recordOfStudent[2] = thirdStudent; // print record of all student displayStduentRecord(recordOfStudent); } public static void displayStduentRecord(Student[] recordOfStudent) { for(int i = 0; i < recordOfStudent.length; i++) { Student stduent = recordOfStudent[i]; System.out.println("Student rollNo = "+stduent.getRollNo()); System.out.println("Student Name = "+stduent.getName()); System.out.println("Student class = "+stduent.getClassName()); } } }
Output:
Student rollNo = 1
Student Name = Ravi kant
Student class = MCA
Student rollNo = 2
Student Name = Rama
Student class = MCA
Student rollNo = 3
Student Name = Radhe
Student class = MCA
As you can see in the above example, We want to store records of the number of students. So we created objects for each student and stored them in Array. We created an Array of objects
Student[] recordOfStudent = new Student[3];
It is storing three objects for students. You can change the size of the Array according to your requirements. After the creation of an array of objects, we initialized the value of each index.
recordOfStudent[0] = firstStudent; recordOfStudent[1] = firstStudent; recordOfStudent[2] = firstStudent;
ArrayIndexOutOfBoundsException in java
As you know Array has a fixed length. You can’t change it after the declaration of the array. It consists of a fixed number of elements. You can access the element from an array by using the index. The index of an array is always an integer value and it starts from 0 and ends with the array’s length-1. If users trying to access an element by either negative or greater than or equal to the size of the array then the system will throw an exception called ArrayIndexOutOfBoundsException. It is a run-time exception. In this article, we will discuss all the scenarios of arrayindexoutofboundsexception in java or java arrayindexoutofboundsexception.
class ArrayIndexOutOfBoundsExceptionExample { public static void main(String[] args) { String[] names = { "Ravi", "Rama", "Radhe"}; for (int i = 0; i <= 3; i++) System.out.println("Names of Students = "+names[i]); } }
Output: Names of Students = Ravi
Names of Students = Rama
Names of Students = Radhe Exception in thread “main” java.lang.ArrayIndexOutOfBoundsException: 3 at ArrayIndexOutOfBoundsExceptionExample.main(ArrayIndexOutOfBoundsExceptionExample.java:6)
Explanation:
In this example compiler simply prints the names of the student until i(index) is less than 3. Complier throwing an exception when i(index) becomes equal to 3 because we are trying to get an element by index(i) which is equal to the size of the array. We should always remember one thing the index of an array starts from 0 and you can traverse an array’s length – 1. As you can see in below image. The name at index 3 (i = 3) doesn’t exists.
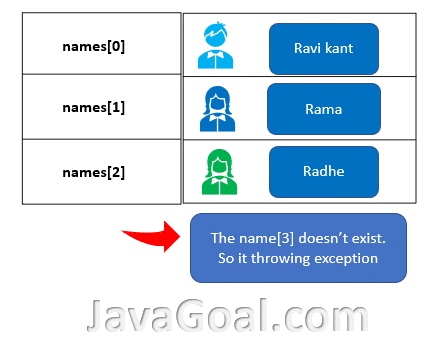
Jagged array in java
In a two-dimensional array, we can also choose different sizes for rows and columns. It means we can make a matrix of any size. These types of arrays are also known as Jagged arrays in java. A jagged array is an array of arrays such that member arrays can be of different sizes.
dataType nameOfArray = new Datatype[size][size];
dataType nameOfArray = new datatype[2][3];
Memory Representation after Construction
Column 1 | Column 2 | Column 3 | |
Row 1 | number[0][0] | number[0][1] | number[0][2] |
Row 2 | number[1][0] | number[1][1] | number[1][2] |
You can see in the example we define the size of the array. The first subscript size is 2 and the second subscript size is 3 which means it will create a 2X3 matrix.
Initialization of Jagged array in java
You can provide value to an array at declaration time. Here is the syntax of the initialization of the Jagged array is similar to the two-dimensional array.
dataType arrayName[][] = { {value1, value2, …valueN}, {value1, value2, …valueN}, {value1, value2, …valueN}, };
Here is the syntax to create an array with the initialization of values.
int[][] number = { {11, 22, 33}, {44, 55, 66}, };
Memory Representation after Initialization
In this example here, we are initializing a Jagged array. This array is holding the 6 elements of type int.
Column 1 | Column 2 | Column 3 | |
Row 1 | number[0][0]=11 | number[0][1]=22 | number[0][2]=33 |
Row 2 | number[1][0]=44 | number[1][1]=55 | number[1][2]=66 |
A jagged array is an array of arrays. You can see the structure of the array:

Let’s take an example of a Jagged array. In which we are declaring and initializing the array.
public class ExampleOfJaggedArray { public static void main(String[] args) { // Creation of Array int[][] number; String[][] names; // Construction of Array number = new int[2][3]; names = new String[3][3]; // initilization of Array for(int i = 0; i < 2; i++) { for(int j = 0; j < 3; j++) { number[i][j] = i+j; names[i][j] = "Hello"+i+j; } } // Accessing of Array for(int i = 0; i < 2; i++) { for(int j = 0; j < 3; j++) { System.out.println(number[i][j]); System.out.println(names[i][j]); } } } }
Output: 0
Hello00
1
Hello01
2
Hello02
1
Hello10
2
Hello11
3
Hello12
The array default value in java
When we are creating a new array, the elements of the array automatically be initialized by their default values. After the construction of the array, a new array of in heap memory according to the type of array. In this article, we will discuss what is Array default value in java.
Below are the default assigned values.
- boolean: false
- int : 0
- double : 0.0
- String: null
- User-Defined Type: null
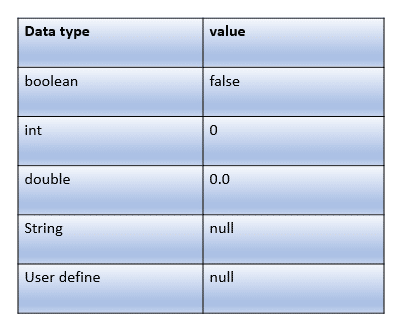
class ClassOne { public static void main(String[] args) { // Declaring an int type array int intArray[] = new int[2]; // Print all elements of intArray for (int i = 0; i < intArray.length; i++) System.out.println("Elements of intArray = " + intArray[i]); // Declaring an boolean type array boolean[] booleanArray = new boolean[2]; // Print all elements of booleanArray for (int i = 0; i < booleanArray.length; i++) System.out.println("Elements of booleanArray = " + booleanArray[i]); // Declaring an String type array String[] stringArray = new String[2]; // Print all elements of stringArray for (int i = 0; i < stringArray.length; i++) System.out.println("Elements of stringArray = " + stringArray[i]); } }
Output: Elements of intArray = 0
Elements of intArray = 0
Elements of booleanArray = false
Elements of booleanArray = false
Elements of stringArray = null
Elements of stringArray = null
Video in Hindi – Java Programming Goal
How to take input in One-dimensional array from user input
import java.util.Scanner; public class OneDimensionalNumberArrayWithUserInput { public static void main(String arg[]) { Scanner scanner = new Scanner(System.in); System.out.print("Enter the size of Array: "); int size = scanner.nextInt(); int numbers[] = new int[size]; System.out.print("Enter the numbers:"); for (int i = 0; i < size; i++ ) { numbers[i] = scanner.nextInt(); } System.out.println("You have entered all the numbers"); System.out.print("All numbers from array: "); for (int i = 0; i < size; i++) { System.out.print(numbers[i] + " "); } } }
Output: Enter the size of Array: 5
Enter the numbers:1
3
5
7
9
You have entered all the numbers
All numbers from array: 1 3 5 7 9
import java.util.Scanner; public class OneDimensionalStringArrayWithUserInput { public static void main(String arg[]) { Scanner scanner = new Scanner(System.in); System.out.print("Enter the size of Array: "); int size = scanner.nextInt(); String names[] = new String[size]; System.out.print("Enter the names:"); for (int i = 0; i < size; i++ ) { names[i] = scanner.next(); } System.out.println("You have entered all the names"); System.out.print("All names from array: "); for (int i = 0; i < size; i++) { System.out.print(names[i] + " "); } } }
Output: Enter the size of Array: 5
Enter the names:KKR
YNR
DEL
NCR
CHD
You have entered all the names
All names from array: KKR YNR DEL NCR CHD
How to take user input for two dimensional (2D) array
import java.util.Scanner; public class TwoDimensionalNumberArrayWithUserInput { public static void main(String arg[]) { Scanner scanner = new Scanner(System.in); System.out.print("Enter the size of rows: "); int rows = scanner.nextInt(); System.out.print("Enter the size of columns: "); int columns = scanner.nextInt(); int numbers[][] = new int[rows][columns]; for (int i = 0; i < rows; i++ ) { System.out.print("Enter the number for row:"); for (int j = 0; j < columns; j++) numbers[i][j] = scanner.nextInt(); } System.out.println("You have entered all the numbers"); System.out.println("All numbers from array: "); for (int i = 0; i < rows; i++ ) { for (int j = 0; j < columns; j++) { System.out.print(numbers[i][j] + " "); } System.out.println(); } } }
Output: Enter the size of rows: 3
Enter the size of columns: 3
Enter the number for row:11
22
33
Enter the number for row:44
55
66
Enter the number for row:77
88
99
You have entered all the numbers
All numbers from array:
11 22 33
44 55 66
77 88 99
import java.util.Scanner; public class TwoDimensionalStringArrayWithUserInput { public static void main(String arg[]) { Scanner scanner = new Scanner(System.in); System.out.print("Enter the size of rows: "); int rows = scanner.nextInt(); System.out.print("Enter the size of columns: "); int columns = scanner.nextInt(); String names[][] = new String[rows][columns]; for (int i = 0; i < rows; i++ ) { System.out.print("Enter the names for row: "); for (int j = 0; j < columns; j++) names[i][j] = scanner.next(); } System.out.println("You have entered all the names"); System.out.println("All names from array: "); for (int i = 0; i < rows; i++ ) { for (int j = 0; j < columns; j++) { System.out.print(names[i][j] + " "); } System.out.println(); } } }
Output: Enter the size of rows: 3
Enter the size of columns: 3
Enter the names for row: ABC
DEF
GHI
Enter the names for row: JKL
MNO
PQR
Enter the names for row: STU
UXY
Z
You have entered all the names
All names from array:
ABC DEF GHI
JKL MNO PQR
STU UXY Z
In java matrix addition program
import java.util.Scanner; public class MatrixAdditionExample { public static void main(String arg[]) { Scanner scanner = new Scanner(System.in); System.out.println("Enter the details of first matrix"); System.out.print("Enter the size of rows: "); int rows = scanner.nextInt(); System.out.print("Enter the size of columns: "); int columns = scanner.nextInt(); int firstMatrix[][] = new int[rows][columns]; for (int i = 0; i < rows; i++ ) { System.out.print("Enter the number for row:"); for (int j = 0; j < columns; j++) firstMatrix[i][j] = scanner.nextInt(); } System.out.println("You have entered all the numbers"); System.out.println("All numbers from array: "); for (int i = 0; i < rows; i++ ) { for (int j = 0; j < columns; j++) { System.out.print(firstMatrix[i][j] + " "); } System.out.println(); } System.out.println("Enter the details of second matrix "); System.out.print("Enter the size of rows: "); int secondRows = scanner.nextInt(); System.out.print("Enter the size of columns: "); int secondColumns = scanner.nextInt(); int secondMatrix[][] = new int[secondRows][secondColumns]; for (int i = 0; i < secondRows; i++ ) { System.out.print("Enter the number for row:"); for (int j = 0; j < secondColumns; j++) secondMatrix[i][j] = scanner.nextInt(); } System.out.println("You have entered all the numbers"); System.out.println("All numbers from array: "); for (int i = 0; i < secondRows; i++ ) { for (int j = 0; j < secondColumns; j++) { System.out.print(secondMatrix[i][j] + " "); } System.out.println(); } System.out.println("After addition of matrix"); for (int i = 0; i < rows; i++ ) { for (int j = 0; j < columns; j++) { System.out.print(firstMatrix[i][j] + secondMatrix[i][j] + " "); } System.out.println(); } } }
Output: Enter the details of first matrix
Enter the size of rows: 3
Enter the size of columns: 3
Enter the number for row:11
22
33
Enter the number for row:44
55
66
Enter the number for row:77
88
99
You have entered all the numbers
All numbers from array:
11 22 33
44 55 66
77 88 99
Enter the details of second matrix
Enter the size of rows: 3
Enter the size of columns: 3
Enter the number for row:1
2
3
Enter the number for row:4
5
6
Enter the number for row:7
8
9
You have entered all the numbers
All numbers from array:
1 2 3
4 5 6
7 8 9
After addition of matrix
12 24 36
48 60 72
84 96 108
Program to perform matrix subtraction in java
import java.util.Scanner; public class MatrixSubtractionExample { public static void main(String arg[]) { Scanner scanner = new Scanner(System.in); System.out.println("Enter the details of first matrix"); System.out.print("Enter the size of rows: "); int rows = scanner.nextInt(); System.out.print("Enter the size of columns: "); int columns = scanner.nextInt(); int firstMatrix[][] = new int[rows][columns]; for (int i = 0; i < rows; i++ ) { System.out.print("Enter the number for row:"); for (int j = 0; j < columns; j++) firstMatrix[i][j] = scanner.nextInt(); } System.out.println("You have entered all the numbers"); System.out.println("All numbers from array: "); for (int i = 0; i < rows; i++ ) { for (int j = 0; j < columns; j++) { System.out.print(firstMatrix[i][j] + " "); } System.out.println(); } System.out.println("Enter the details of second matrix "); System.out.print("Enter the size of rows: "); int secondRows = scanner.nextInt(); System.out.print("Enter the size of columns: "); int secondColumns = scanner.nextInt(); int secondMatrix[][] = new int[secondRows][secondColumns]; for (int i = 0; i < secondRows; i++ ) { System.out.print("Enter the number for row:"); for (int j = 0; j < secondColumns; j++) secondMatrix[i][j] = scanner.nextInt(); } System.out.println("You have entered all the numbers"); System.out.println("All numbers from array: "); for (int i = 0; i < secondRows; i++ ) { for (int j = 0; j < secondColumns; j++) { System.out.print(secondMatrix[i][j] + " "); } System.out.println(); } System.out.println("After Subtraction of matrix"); for (int i = 0; i < rows; i++ ) { for (int j = 0; j < columns; j++) { System.out.print(firstMatrix[i][j] - secondMatrix[i][j] + " "); } System.out.println(); } } }
Output: Enter the details of first matrix
Enter the size of rows: 3
Enter the size of columns: 3
Enter the number for row:11
22
33
Enter the number for row:44
55
66
Enter the number for row:77
88
99
You have entered all the numbers
All numbers from array:
11 22 33
44 55 66
77 88 99
Enter the details of second matrix
Enter the size of rows: 3
Enter the size of columns: 3
Enter the number for row:1
2
3
Enter the number for row:4
5
6
Enter the number for row:7
8
9
You have entered all the numbers
All numbers from array:
1 2 3
4 5 6
7 8 9
After Subtraction of matrix
10 20 30
40 50 60
70 80 90
How to calculate Matrix multiplication program in java
import java.util.Scanner; public class MatrixMultiplicationExample { public static void main(String arg[]) { Scanner scanner = new Scanner(System.in); System.out.println("Enter the details of first matrix"); System.out.print("Enter the size of rows: "); int rows = scanner.nextInt(); System.out.print("Enter the size of columns: "); int columns = scanner.nextInt(); int firstMatrix[][] = new int[rows][columns]; for (int i = 0; i < rows; i++ ) { System.out.print("Enter the number for row:"); for (int j = 0; j < columns; j++) firstMatrix[i][j] = scanner.nextInt(); } System.out.println("You have entered all the numbers"); System.out.println("All numbers from array: "); for (int i = 0; i < rows; i++ ) { for (int j = 0; j < columns; j++) { System.out.print(firstMatrix[i][j] + " "); } System.out.println(); } System.out.println("Enter the details of second matrix "); System.out.print("Enter the size of rows: "); int secondRows = scanner.nextInt(); System.out.print("Enter the size of columns: "); int secondColumns = scanner.nextInt(); int secondMatrix[][] = new int[secondRows][secondColumns]; for (int i = 0; i < secondRows; i++ ) { System.out.print("Enter the number for row:"); for (int j = 0; j < secondColumns; j++) secondMatrix[i][j] = scanner.nextInt(); } System.out.println("You have entered all the numbers"); System.out.println("All numbers from array: "); for (int i = 0; i < secondRows; i++ ) { for (int j = 0; j < secondColumns; j++) { System.out.print(secondMatrix[i][j] + " "); } System.out.println(); } System.out.println("After addition of matrix"); int multiplication[][] = new int[rows][columns]; for (int i = 0; i < rows; i++ ) { for (int j = 0; j < columns; j++) { multiplication[i][j] = 0; for(int k = 0; k < rows; k++) { multiplication[i][j] += firstMatrix[i][k] * secondMatrix[k][j]; } System.out.print( multiplication[i][j]+ " " ); } System.out.println(); } } }
Output: Enter the details of first matrix
Enter the size of rows: 3
Enter the size of columns: 3
Enter the number for row:1
2
3
Enter the number for row:4
5
6
Enter the number for row:7
8
9
You have entered all the numbers
All numbers from array:
1 2 3
4 5 6
7 8 9
Enter the details of second matrix
Enter the size of rows: 3
Enter the size of columns: 3
Enter the number for row:1
2
3
Enter the number for row:4
5
6
Enter the number for row:7
8
9
You have entered all the numbers
All numbers from array:
1 2 3
4 5 6
7 8 9
After addition of matrix
30 36 42
66 81 96
102 126 150
How to perform the sum of diagonal elements of a matrix
import java.util.Scanner; public class SumOfLeftDiagonal { public static void main(String arg[]) { Scanner scanner = new Scanner(System.in); System.out.print("Enter the size of rows: "); int rows = scanner.nextInt(); System.out.print("Enter the size of columns: "); int columns = scanner.nextInt(); int numbers[][] = new int[rows][columns]; for (int i = 0; i < rows; i++ ) { System.out.print("Enter the number for row:"); for (int j = 0; j < columns; j++) numbers[i][j] = scanner.nextInt(); } System.out.println("You have entered all the numbers"); System.out.println("All numbers from array: "); for (int i = 0; i < rows; i++ ) { for (int j = 0; j < columns; j++) { System.out.print(numbers[i][j] + " "); } System.out.println(); } System.out.print("Sum of left diagonal: "); int sumOfDiagonal = 0; for (int i = 0; i < rows; i++ ) { for (int j = 0; j < columns; j++) { if(i == j) sumOfDiagonal += numbers[i][j]; } } System.out.print("Sum of left diagonal: "+ sumOfDiagonal); } }
Output: Enter the size of rows: 3
Enter the size of columns: 3
Enter the number for row:1
3
5
Enter the number for row:7
9
11
Enter the number for row:13
15
17
You have entered all the numbers
All numbers from array:
1 3 5
7 9 11
13 15 17
Sum of left diagonal: Sum of left diagonal: 27
import java.util.Scanner; public class SumOfRightDiagonal { public static void main(String arg[]) { Scanner scanner = new Scanner(System.in); System.out.print("Enter the size of rows: "); int rows = scanner.nextInt(); System.out.print("Enter the size of columns: "); int columns = scanner.nextInt(); int numbers[][] = new int[rows][columns]; for (int i = 0; i < rows; i++ ) { System.out.print("Enter the number for row:"); for (int j = 0; j < columns; j++) numbers[i][j] = scanner.nextInt(); } System.out.println("You have entered all the numbers"); System.out.println("All numbers from array: "); for (int i = 0; i < rows; i++ ) { for (int j = 0; j < columns; j++) { System.out.print(numbers[i][j] + " "); } System.out.println(); } System.out.print("Sum of Right diagonal: "); int sumOfDiagonal = 0; for (int i = 0; i < rows; i++ ) { for (int j = 0; j < columns; j++) { if((i + j) == (rows - 1)) sumOfDiagonal += numbers[i][j]; } } System.out.print("Sum of Right diagonal: "+ sumOfDiagonal); } }
Output: Enter the size of rows: 3
Enter the size of columns: 3
Enter the number for row:1
3
5
Enter the number for row:7
9
11
Enter the number for row:13
15
17
You have entered all the numbers
All numbers from array:
1 3 5
7 9 11
13 15 17
Sum of Right diagonal: Sum of Right diagonal: 27
arrray quiz