In this article, we will discuss the If statement in Java and also perform some practical examples. We categorize this article into small chunks so that we can understand the use of each part.
Here is the table content of this article we will cover all the parts of this topic.
1. if statement in java
2. nested if statement in java
3. if-else statement in java
4. if-else if statement in java/ladder if statements in java
5. Best practices for if statements
Practics programs for if statement
1. Practics Programs for if statement
2. Practics Programs for if else statement
3. Practics Programs for if else ladder statement
if statement in java
The if statement in Java is a simple decision-making statement. It uses to decide whether the statement or block of statements should execute or not. A block of statements executes only if the given condition returns true otherwise the block of the statement skips.
Youtube video available in the Hindi language
if(condition) { // If condition is true then block of statements will be executed }
Here, the condition will be always in boolean form. It can be either true or false. The if statement accepts only the boolean value in the condition. If a user doesn’t provide curly braces (‘{‘ ‘}’ ) then only one statement consider inside the if block. The rest of the statements don’t consider the body of the if statement block.
if(condition) //This statement will be consider in if block it will be execute if condition is true statement 1; //This statement will not be consider in if block it doesn’t depend on condition. statement 2;
Flowchart of if statement

class ExampleIfStatement { public static void main(String args[]) { int i = 8; if (i > 5) // Statement will be executed if value of “i” is greater than 5 { System.out.println("Number is greater than 5"); } System.out.println("Statement after the if block"); } }
Output:
Number is greater than 5
Statement after the if block
What type of condition can have in the if statement in Java?
The condition of an if statement can be an expression or any boolean variable.
First, we will understand it with an expression that will return a boolean value. We can use any expression as a condition that gives results in a boolean.
Example of expression:
5 > 3, It returns true because 5 is greater than 3.
5 > (3+3), It returns false because 5 is less than 6.
int a = 10;
int b = 15;
int c = 12;
a > b, It returns false because a(10) is less than b(15).
(a+b) > c, It returns true because a+b(25) is greater than c(12).
Let’s create an example, In which the condition can take the expression of different types.
class ExampleIfStatement { public static void main(String args[]) { if (5 > 3) // Statement will be executed because 5 is greater than 3 { System.out.println("The Number 5 greater than 3"); } int a = 10; int b = 12; int c = 15; if (a < b) // Statement will be executed because b is greater than a { System.out.println("The value of b is greater than a"); } if ((a+b) > c) // Statement will be executed because a+b is greater than c { System.out.println("The value of a+b is greater than c"); } } }
Output: The Number 5 greater than 3
The value of b is greater than a
The value of a+b is greater than c
We can directly use a boolean value as a condition in the if statement. Let’s create an example with a boolean value.
Example of expression:
true,
false
class ExampleIfStatement { public static void main(String args[]) { if(true) // Statement will be executed because we are using true { System.out.println("The statement executes because the value is true."); } if(!false) // Statement will be executed because we are inverting the false { System.out.println("The statement executes because the value is inverting of false."); } } }
Output: The statement executes because the value is true.
The statement executes because the value is inverting of false.
Let’s discuss an example of an if statement with an explanation
public class IfStatement { public static void main(String arg[]) { // Here conditon is false, so it will never execute if(false) { System.out.println("1: False if statement"); } // Here conditon is true, so it will execute if(true) { System.out.println("2: True if statement"); } // Here conditon is true, because 0 == 0 always true. so it will execute if(0 == 0) { System.out.println("3: True if statement"); } int age = 20; int a = 5; int b = 4; // Here age is 20 so it will execute if(age > 18) { System.out.println("Eligible for Vote"); } // Here a is 5 and it is less than 8 so it will execute if(a < 8) { System.out.println("4: True if statement"); } // Here a is 5 and it is not less than 5 so it will execute if(a < 5) { System.out.println("5: True If statement"); } // Here a is 5 and b is 4 so it will execute if(a < 8 && b < 5) { System.out.println("6: True if statement"); } // Here a is 5 so it will execute if(a < (5+3)) { System.out.println("7: True if statement"); } System.out.println("8: Statement above if statement"); if(a < 10) { System.out.println("9: True if statement"); System.out.println("10: Value of a is less than 10"); } System.out.println("11: Statement below if statement"); if(a < 10) System.out.println("12: Single statement in if statement"); System.out.println("13: Outside statement"); if(a > 10) System.out.println("13: Single statement in if statement"); System.out.println("14: Outside statement"); } }
Output: 2: True if statement
3: True if statement
Eligible for Vote
4: True if statement
6: True if statement
7: True if statement
8: Statement above if statement
9: True if statement
10: Value of a is less than 10
11: Statement below if statement
12: Single statement in if statement
13: Outside statement
14: Outside statement
Nested if statement in Java
We have discussed the if statement in the above section. Now, Let’s how can we use the nested if statement in java and nested if in java. There are a number of situations when we want to execute the code based on conditions. The nested if statement in java means an if statement inside another if statement. A statement inside the if statement is known as the inner if statement and another is known as the outer if statement. The inner block of the if statement executes only if the outer block condition is true. In Java, We can use nested if statements, It means if statements within if statements. i.e, we can place any number of if statements inside another if statement. But it may increase the complexity of your program.
Syntax of nested if statement
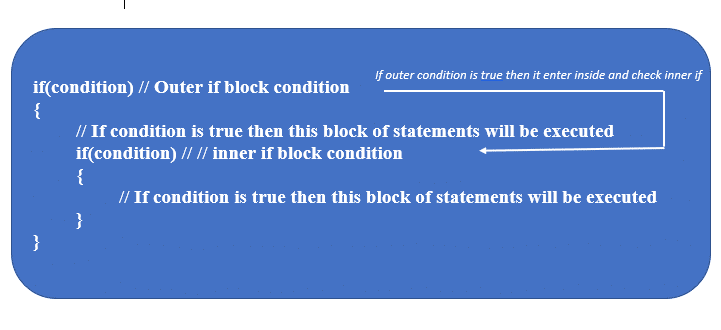
Flowchart
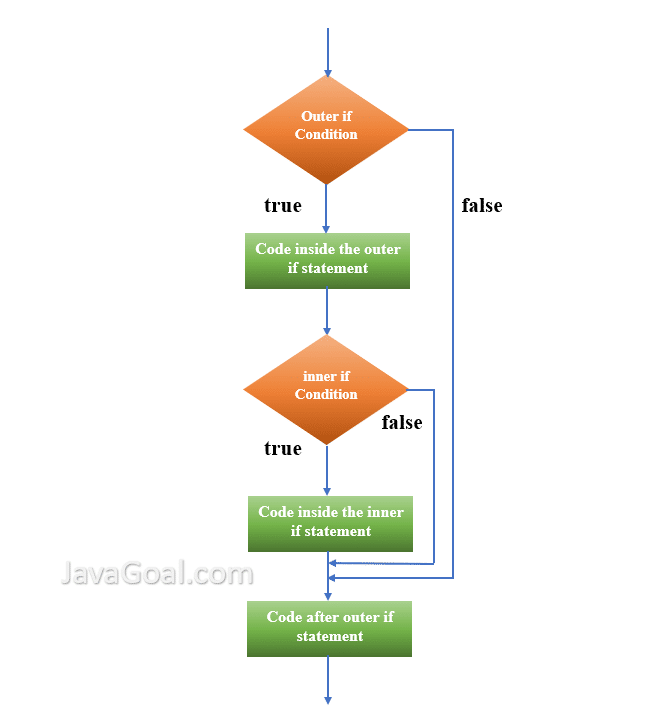
class ExampleNestedIfStatement { public static void main(String args[]) { int age = 20; boolean hasVoterCard = true; if (age > 18) // if age is greater than 18 { // It will be print when outer if block condition is true. // It doesn't depends on the inner if block. System.out.println("The age of person is : " + age); if(hasVoterCard) // If person has voter card { // It will be print only if outer and inner block condition both are true System.out.println("The person has voter card also"); } // inner if block is closed // It will be print when outer if block condition is true. // It doesn't depends on the inner if block . System.out.println("Statement after the inner if block"); } // outer if block is closed // It will print always because it outside the both if blocks. System.out.println("Statement after the outer if block"); } }
Output:
The age of person is : 20
The person has voter card also
Statement after the inner if block
Statement after the outer if block
Another example of Nest if with different scenario:
class ExampleNestedIfStatement { public static void main(String args[]) { int age = 20; boolean hasVoterCard = false; if (age > 18) // if age is greater than 18 { // It will be print when outer if block condition is true. // It doesn't depends on the inner if block System.out.println("The age of person is : " + age); if(hasVoterCard) // If person has voter card { // It will be print only if outer and inner block condition both are true System.out.println("The person has voter card also"); } // inner if block closed // It will be print when outer if block condition is true. // It doesn't depends on the inner if block. System.out.println("Statement after the inner if block"); } // outer if block closed // It will print always because it outside the both if blocks. System.out.println("Statement after the outer if block"); } }
Output: The age of person is : 20
Statement after the inner if block
Statement after the outer if block
In the above example, A person is eligible to vote only if he/she is above 18 and has a voter card. So, first of all, we need to check the age of the person and after that, we will verify whether the person has a voter card or not.
So Here are using nested if statements.
The outer if statement condition is checking, whether the person is above 18 or not.
The inner if statement condition is checking, whether the person has a voter card or not.
The inner if statement is totally dependent on the outer if statement. If a person’s age is less than 18 then not need to check the voter card.
Let’s take another example of a nested if statement with an explanation.
public class NestedIfStatement { public static void main(String arg[]) { int a = 5; int b = 4; int age = 20; boolean hasVoterCard = false; // Here value of age is 20 so outer if condition will be true if (age > 18) { // hasVoterCard variable has false value if (hasVoterCard) System.out.println("You are eligible"); System.out.println("Hello"); } // Here value of a is 5, and it is less than 8. So it will be executed if(a < 8) { // This statement will execute as it is body part of outer loop System.out.println("1: True in if statement"); // Here value of b is 4, and it's less than 5. So it will be executed if(b < 5) // This statement is body part of inner loop System.out.println("2: True in nested if statement"); // This statement will not be considered as part of inner nested if System.out.println("3: Outside nested if statement"); System.out.println("4: Inside if statement"); } // Here value of a is 5, and it is less than 8. So it will be executed if(a < 6) { System.out.println("5: True inside if statement"); // Here value of b is 4, and it is less than 8. So it will be executed if(b < 8) { System.out.println("6: True inside nested if statement"); // This statement will execute as condition is always true. if(true) System.out.println("7: True inside nested if statement"); System.out.println("8: Outside nested if statement"); } } } }
Output: Hello
1: True in if statement
2: True in nested if statement
3: Outside nested if statement
4: Inside if statement
5: True inside if statement
6: True inside nested if statement
7: True inside nested if statement
8: Outside nested if statement
If else statement in Java
In the if statement the block of statements executes only if the given condition is true otherwise block of the statements skips. But there may be a situation when we want to execute some other block of code if the condition is false. In this scenario, we can use the if else statement in Java. There are two blocks one block is the if block and another is the else block. If a certain condition returns true, then if block executes otherwise else block executes.
Syntax
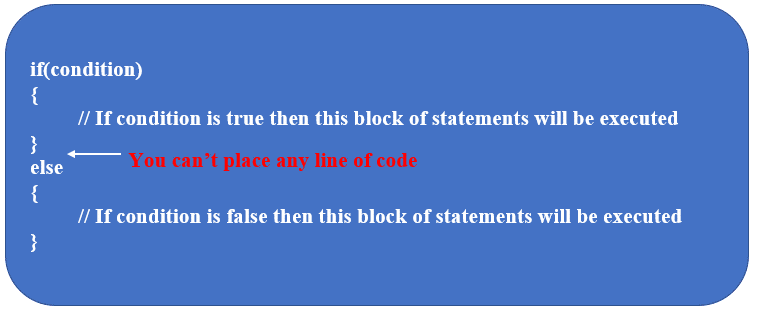
In Java, an if statement has an optional block and this block is known as the else block. You must think about why we are saying else block is optional. Because if block can be used without else block but else block exists only with if block. The else block only gets executed if the condition of the if the statement becomes false. There are a number of situations when we want to run the code based on different situations. Suppose we want to perform a task named TASK1 when the code satisfies the condition of the if statement otherwise you want to perform different tasks.
RULE: You can place any code in between closing braces of if (‘}’) and else.
Flowchart of if-else statement
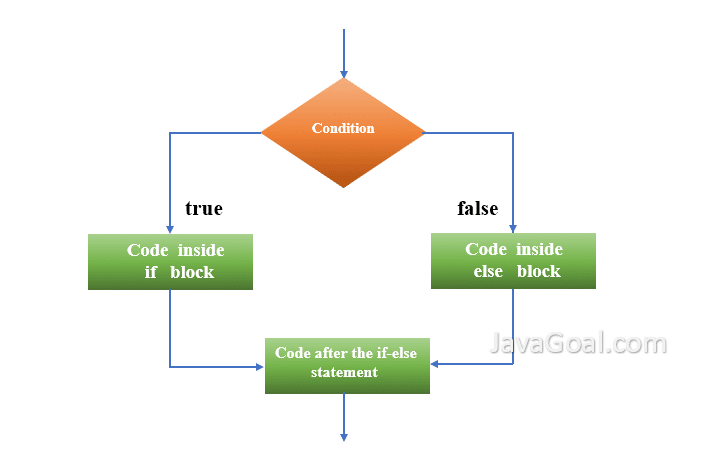
if else program in java
class ExampleIfElseStatement { public static void main(String args[]) { int age = 15; if (age > 18) // if age is greater than 18 { // It will be print if block condition is true. System.out.println("The age of person is : " + age + "He/she is eligible for voting"); } else { // It will be print if block condition is false. System.out.println("He/she is not eligible for voting"); } } }
Output: He/she is not eligible for voting
In the above example, A person is eligible to vote only if he/she is above 18 otherwise he/she is not eligible for voting. Here we want to show the message to the person about whether he/she is able to vote or not. First of all, we need to check the age of the person.
So Here are using the if-else statement.
The if block condition checks, whether the person is above 18 or not.
The else block will be executed only when the evaluation of the condition (if statement) is false.
If a person is greater than 18 then if block is getting executed otherwise else block.
Let’s take an example of an if else statement in java with the explanation.
public class IfElseStatement { public static void main(String arg[]) { int a = 10; int b = 5; int age = 20; boolean hasVoterCard = false; // Here age is 20 which is greater than 18 but user doesn't voter card // So hasVoterCard variable is false. So this condition has become false // Compiler will not execute the body of if statement if (age > 18 && hasVoterCard) { System.out.println("You are eligible"); } // As if statement doesn't satisfy the condition so else block // will be executed else { System.out.println("You are not eligible"); } // Here value of a is 5, and it is not greater than 15 if (a > 15) { System.out.println("5: a is greater than 15"); System.out.println("6: If statement"); } // As if statement doesn't satisfy the condition so else block // will be executed else { System.out.println("7: a is less than 15"); System.out.println("8: else statement"); // Value of a is 15 and it satisfies the condition // But it will be executed only if outer block will be executed. if(a == 15) System.out.println("9: a is equal to 15"); } // Here value of b is 5 and which is greater than 1. // So condition of if block will return true, and it will // get executed if (b > 1) { System.out.println("10: b is greater than 1"); // Value of b is 5, and it satisfies the condition // But it will be executed only if, outer if statement block will be executed. if(b == 5) System.out.println("11: If statement"); else System.out.println("12: else statement"); } // If b is not greater than 1 else { System.out.println("13: b is less than 1"); System.out.println("14: else statement"); if(b == 5) System.out.println("15: b is equal to 5"); } } }
Output: You are not eligible
7: a is less than 15
8: else statement
10: b is greater than 1
11: If statement
if else ladder in java
We have discussed the if else statement and also discussed its use. Let’s discuss the if else if ladder and also see the flowchart of the ladder if statement.
Point 1: In the if else statement, the if block of statements executes only if the given condition is true, otherwise compiler executes the else block of the statement.
Point 2: In the if statement the block of statements executes only if the given condition returns true otherwise block of the statement skips.
The scenario of the ladder if statement: If we want to execute the different code based on different conditions then we can use the if-else-if statement. It is also known as if else if ladder statement in java. This statement executes from the top down. During the execution of conditions if any condition is founds true, then the statement associated with that block will be executed, and the rest of the code will be skipped. If none of the conditions is true, then the final else statement will be executed.
if(condition) { // If condition is true then this block of statements will be executed } else if(condition) { // If condition is true then this block of statements will be executed } . . . else { // If none of condition is true, then this block of statements will be executed }
Flowchart of if else if ladder statement in java
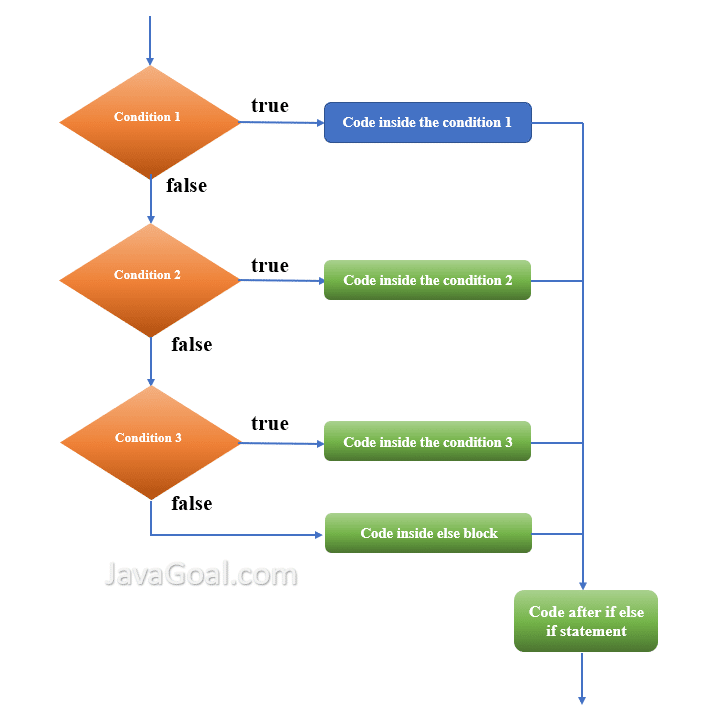
class ExampleIfElseIfStatement { public static void main(String args[]) { int age = 15; if(age < 18) { System.out.println("The age of person is : " + age + " He/she is a child."); } else if(age > 18 && age < 40) { System.out.println("The age of person is : " + age + " He/she is Younger."); } else { System.out.println("The age of person is : " + age + " He/she is old person."); } System.out.println("This statement is outside of the if-else-if"); } }
Output: The age of person is : 15 He/she is a child.
This statement is outside of the if-else-if
Important point
1. The if block can be followed by either zero or one else block or if block.
2. If any of else if block succeeds, none of the remaining will be tested.
public class IfElseIfStatement { public static void main(String arg[]) { int a = 10; // Evaluating the expression that will return true or false // Value of a is not equal to 1, so it will return false if (a == 1) { System.out.println("Value of a: "+a); } // Evaluating the expression that will return true or false // Value of a is not equal to 5, so it will return false else if(a == 5) { System.out.println("Value of a: "+a); } // Evaluating the expression that will return true or false // Value of a is not equal to 11, so it will return false else if(a == 11) { System.out.println("Value of a: "+a); } // This block will be executed only if all the above condition returns false. else { System.out.println("else block"); } } }
Output: else block
if statement programs for practices
1. Write a program to check if a number is positive or negative
import java.util.Scanner; public class CheckSign { public static void main(String[] args) { // Create a Scanner object to read input from the user Scanner input = new Scanner(System.in); // Prompt the user to enter a number System.out.print("Enter a number: "); // Read the number from the user int num = input.nextInt(); // Check if the number is greater than zero if (num > 0) { // If the number is positive, print a message System.out.println(num + " is positive."); } } }
2. Write a program to check if a year is a leap year
import java.util.Scanner; public class CheckLeapYear { public static void main(String[] args) { // Create a Scanner object to read input from the user Scanner input = new Scanner(System.in); // Prompt the user to enter a year System.out.print("Enter a year: "); // Read the year from the user int year = input.nextInt(); // Check if the year is a leap year if ((year % 4 == 0) && (year % 100 != 0)) { // If the year is a leap year, print a message System.out.println(year + " is a leap year."); } } }
3. Write a program to find the largest of three numbers
import java.util.Scanner; public class LargestOfThree { public static void main(String[] args) { Scanner sc = new Scanner(System.in); System.out.print("Enter first number: "); int num1 = sc.nextInt(); System.out.print("Enter second number: "); int num2 = sc.nextInt(); System.out.print("Enter third number: "); int num3 = sc.nextInt(); int largest = num1; // initialize largest with first number // check if second number is greater than largest if (num2 > largest) { largest = num2; } // check if third number is greater than largest if (num3 > largest) { largest = num3; } System.out.println("Largest of three numbers is: " + largest); sc.close(); } }
4. Write a program to check if a character is a vowel or not
import java.util.Scanner; public class VowelCheck { public static void main(String[] args) { Scanner sc = new Scanner(System.in); System.out.print("Enter a character: "); char ch = sc.next().charAt(0); // check if the character is 'a', 'e', 'i', 'o', or 'u' (case-insensitive) if (ch == 'a' || ch == 'A' || ch == 'e' || ch == 'E' || ch == 'i' || ch == 'I' || ch == 'o' || ch == 'O' || ch == 'u' || ch == 'U') { System.out.println(ch + " is a vowel."); } else { System.out.println(ch + " is not a vowel."); } sc.close(); } }
5. Write a Program to check if a number is even
import java.util.Scanner; public class EvenNumber { public static void main(String[] args) { Scanner sc = new Scanner(System.in); System.out.print("Enter a number: "); int num = sc.nextInt(); // check if the number is divisible by 2 if (num % 2 == 0) { System.out.println(num + " is an even number."); } else { System.out.println(num + " is an odd number."); } sc.close(); } }
6. Write a program to check if a number is prime or not
import java.util.Scanner; public class PrimeNumber { public static void main(String[] args) { Scanner sc = new Scanner(System.in); System.out.print("Enter a number: "); int num = sc.nextInt(); boolean isPrime = true; // flag to store if the number is prime or not // loop to check if the number is divisible by any number from 2 to num-1 for (int i = 2; i < num; i++) { if (num % i == 0) { isPrime = false; // if the number is divisible, set the flag to false break; } } // check if the flag is still true, i.e. the number is not divisible by any number if (isPrime) { System.out.println(num + " is a prime number."); } else { System.out.println(num + " is not a prime number."); } sc.close(); } }
7. Write a java program that finds the maximum of two numbers using an if
statement
import java.util.Scanner; public class MaxOfTwoNumbers { public static void main(String[] args) { Scanner sc = new Scanner(System.in); System.out.print("Enter first number: "); int num1 = sc.nextInt(); System.out.print("Enter second number: "); int num2 = sc.nextInt(); // compare the two numbers and store the larger number in a variable int max; if (num1 > num2) { max = num1; } else { max = num2; } System.out.println("The maximum of " + num1 + " and " + num2 + " is " + max + "."); sc.close(); } }
Comment on page if you like the content “if statement programs for practices”.
2. Practics Programs for if else statement
1. Write a java program to check if a number is positive or negative, by use of an if else statement in java
import java.util.Scanner; public class PositiveNegativeCheck { public static void main(String[] args) { Scanner sc = new Scanner(System.in); System.out.print("Enter a number: "); int number = sc.nextInt(); sc.close(); // Check if the number is positive or negative if (number > 0) { System.out.println(number + " is positive"); } else { System.out.println(number + " is negative"); } } }
2. Write a java program to find the largest of two numbers, by use of an if else statement in java
import java.util.Scanner; public class LargestNumber { public static void main(String[] args) { Scanner sc = new Scanner(System.in); System.out.print("Enter first number: "); int num1 = sc.nextInt(); System.out.print("Enter second number: "); int num2 = sc.nextInt(); sc.close(); // Check which number is larger if (num1 > num2) { System.out.println(num1 + " is larger"); } else { System.out.println(num2 + " is larger"); } } }
3. Write a java program to check if a character is a vowel or consonant, by use of an if else statement in java
public class VowelOrConsonant { public static void main(String[] args) { char c = 'a'; // Check if the input character is a vowel or consonant if (c == 'a' || c == 'e' || c == 'i' || c == 'o' || c == 'u' || c == 'A' || c == 'E' || c == 'I' || c == 'O' || c == 'U') { System.out.println(c + " is a vowel"); } else { System.out.println(c + " is a consonant"); } } }
4. Write a java program to check if a year is a leap year or not, by use of an if else statement in java
public class LeapYear { public static void main(String[] args) { int year = 2000; // Check if the year is a leap year if ((year % 400 == 0) || ((year % 4 == 0) && (year % 100 != 0))) { System.out.println(year + " is a leap year"); } else { System.out.println(year + " is not a leap year"); } } }
5. Write a java Program to find the largest of three numbers, by use of an if else statement in java
import java.util.Scanner; public class LargestOfThree { public static void main(String[] args) { Scanner input = new Scanner(System.in); System.out.print("Enter the first number: "); int num1 = input.nextInt(); System.out.print("Enter the second number: "); int num2 = input.nextInt(); System.out.print("Enter the third number: "); int num3 = input.nextInt(); // Check if num1 is the largest among num1, num2 and num3 if (num1 > num2 && num1 > num3) { System.out.println(num1 + " is the largest number"); } // Check if num2 is the largest among num1, num2 and num3 else if (num2 > num1 && num2 > num3) { System.out.println(num2 + " is the largest number"); } // If neither num1 nor num2 is the largest, num3 must be the largest else { System.out.println(num3 + " is the largest number"); } } }
6. Write a java program to check if a number is divisible by 5 and 11 or not, by use of an if else statement in java
import java.util.Scanner; public class DivisibleBy5And11 { public static void main(String[] args) { Scanner sc = new Scanner(System.in); // Taking input from the user System.out.print("Enter a number: "); int num = sc.nextInt(); // Check if the number is divisible by 5 and 11 if (num % 5 == 0 && num % 11 == 0) { System.out.println(num + " is divisible by 5 and 11"); } else { System.out.println(num + " is not divisible by 5 and 11"); } } }
7. Write a java program to check if a number is prime or not, by use of an if else statement in java
import java.util.Scanner; public class PrimeNumberCheck { public static void main(String[] args) { Scanner sc = new Scanner(System.in); // Taking input from the user System.out.print("Enter a number: "); int num = sc.nextInt(); boolean isPrime = true; // Check if the number is prime or not for (int i = 2; i <= num / 2; i++) { if (num % i == 0) { isPrime = false; break; } } // Output the result if (isPrime) { System.out.println(num + " is a prime number"); } else { System.out.println(num + " is not a prime number"); } } }
8. Write a java Program to check if a number is even or odd, by use of an if else statement in java
import java.util.Scanner; public class EvenOddCheck { public static void main(String[] args) { Scanner input = new Scanner(System.in); System.out.println("Enter a number to check if it is even or odd:"); int number = input.nextInt(); // Check if the number is divisible by 2 if (number % 2 == 0) { System.out.println(number + " is an even number."); } else { System.out.println(number + " is an odd number."); } } }
3. Practics Programs for if else ladder statement
1. Write a java program to find if a number is positive or negative by the if else statement.
import java.util.Scanner; public class PositiveNegative { public static void main(String[] args) { Scanner input = new Scanner(System.in); System.out.println("Enter a number: "); int number = input.nextInt(); if(number > 0) { System.out.println(number + " is positive."); } else if(number < 0) { System.out.println(number + " is negative."); } else { System.out.println(number + " is neither positive nor negative."); } } }
2. Write a java program to Find the largest of three numbers by if else statement.
import java.util.Scanner; public class LargestNumber { public static void main(String[] args) { Scanner input = new Scanner(System.in); System.out.println("Enter three numbers: "); int num1 = input.nextInt(); int num2 = input.nextInt(); int num3 = input.nextInt(); if(num1 >= num2 && num1 >= num3) { System.out.println(num1 + " is the largest number."); } else if(num2 >= num1 && num2 >= num3) { System.out.println(num2 + " is the largest number."); } else { System.out.println(num3 + " is the largest number."); } } }
3. Write a java program to Check if a year is a leap year by if else statement.
import java.util.Scanner; public class LeapYear { public static void main(String[] args) { Scanner input = new Scanner(System.in); System.out.println("Enter a year: "); int year = input.nextInt(); if(year % 4 == 0) { if(year % 100 == 0) { if(year % 400 == 0) { System.out.println(year + " is a leap year."); } else { System.out.println(year + " is not a leap year."); } } else { System.out.println(year + " is a leap year."); } } else { System.out.println(year + " is not a leap year."); } } }
4. Write a java program to Check if a character is a vowel or consonant by an if else statement.
import java.util.Scanner; public class VowelConsonant { public static void main(String[] args) { Scanner input = new Scanner(System.in); System.out.println("Enter a character: "); char ch = input.next().charAt(0); if(ch == 'a' || ch == 'e' || ch == 'i' || ch == 'o' || ch == 'u' || ch == 'A' || ch == 'E' || ch == 'I' || ch == 'O' || ch == 'U') { System.out.println(ch + " is a vowel."); } else { System.out.println(ch + " is a consonant."); } } }
1. Quiz, Read the below code and do answer.
Click on anyone to know the answer.