Java has a lot of keywords that are used for different purposes. In this post, we will discuss, what is the difference between final, finally and finalize keyword in java and when we should use them. It is the most common and tricky question for the interview. I have seen many times many programmers get confused in the final, finally and finalize keywords.
In java, we have final, finally and finalize keywords that look similar but the working of these keywords is totally different. In this post, we will not find one to one difference between final finally finalize in java. We will discuss the working and use of each keyword. After that, you can find the difference and use of each keyword.
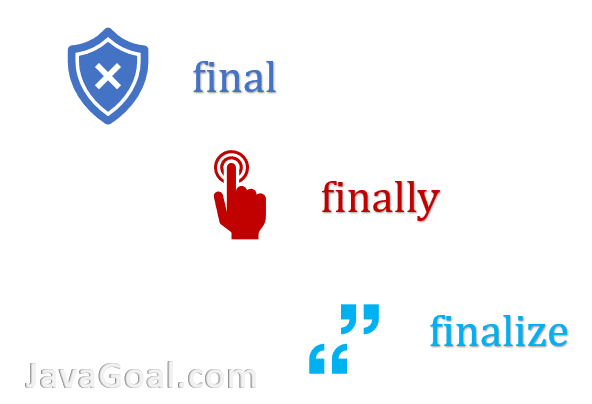
1. final keyword
The final keyword in Java is a very important keyword that is used to apply some restrictions to the user. We can use it with class, method, and variable in Java.
The final is a reserved word or keyword in Java that can be used with a variable to make a constant, used with a method to restrict the method overriding, used with a class to restrict the inheritance of class.
i) final variable
ii) final method
iii) final class
i) final variable
The final variables are just like constants. To make a constant variable, you can use the final keyword with the variable. You can’t change the value of a final variable once it is initialized. So, we should initialize it.
Note: It is not mandatory to initialize a final variable at the time of declaration. You can initialize it by constructor you can read it in detail. If you are not initializing it at the time of declaration, it’s called a blank final variable.
It is good practice to represent final variables in all uppercase, using an underscore to separate words. You can read it in detail here.
class FinalVariable { public static void main(String args[]) { final int a = 10; a = 10+5; System.out.println(a); } }
Output: Exception in thread “main” java.lang.Error: Unresolved compilation problem: The final local variable cannot be assigned. It must be blank and not using a compound assignment at FinalVariable.main(FinalVariable.java:8)
ii) final keyword
A final keyword can be applied to a variable, method, or class. I have seen in the previous post how the final variable is used in java and applied some restrictions to the user. When we use the final keyword with the method, JVM applies restriction to the method.
We can declare a method in a class with the final keyword. A method declared with the final keyword is called the final method. A final method can’t be overridden in subclasses. Whenever we define a final keyword with a method, the final keyword indicates to the JVM that the method should not be overridden by subclasses.
In Java, there are many classes that have final methods and they are not overridden in subclasses. In java, Object class have notify() and wait() method are final methods. The final method enforces the subclasses to follow the same implementation throughout all the subclasses.
NOTE: The final method always binds at compile time in java. It is much faster than non-final methods because they are not required to be resolved during run-time.
class ParentClass { final void showData() { System.out.println("This is a final method of Parent class"); } } class ChildClass extends ParentClass { // It will throw compliation error final void showData() { System.out.println("This is a final method of Child class"); } } class MainClass { public static void main(String arg[]) { ParentClass obj = new ChildClass(); obj.showData(); } }
Output: Compilation error
iii) final class
A class that is declared with the final keyword is known as the final class. A final class can’t be inherited by subclasses. By use of the final class, we can restrict the inheritance of the class. We can create a class as a final class only if it is complete in nature it means it must not be an abstract class. In java, all the wrapper classes are final class like String, Integer, etc.
If we try to inherit a final class, then the compiler throws an error at compilation time. We can’t create a class as immutable without the final class.
final class ParentClass { void showData() { System.out.println("This is a method of final Parent class"); } } //It will throw compilation error class ChildClass extends ParentClass { void showData() { System.out.println("This is a method of Child class"); } } class MainClass { public static void main(String arg[]) { ParentClass obj = new ChildClass(); obj.showData(); } }
Output: Exception in thread “main” java.lang.Error: Unresolved compilation problem: Type mismatch: cannot convert from ChildClass to ParentClass at MainClass.main(MainClass.java:21)
2. finally
If you haven’t read the exception handling yet then you should read the exception handling first.
In java, finally is a keyword is used to execute a set of statements or blocks. Generally, the finally keyword is known as the finally block. A finally block executes after the execution of the try block. A finally block contains all statements that must be executed whether an exception occurs or not. If you want to perform any necessary operation in your program whether an exception occurs or not, then you can use finally block.
There are a lot of scenarios of finally block in java. You can read them as well. Here we are going to demonstrate with one scenario.
public class ExampleFinallyWithoutCatchBlock { public static void main(String args[]) { try { System.out.println("This code is inside in the try block"); String s = "Ravikant"; System.out.println("result of calculation = "+ s); System.out.println("Rest code inside the try block"); } finally { System.out.println("This is finally block"); } //rest code of the program System.out.println("This code is outside from the try block"); } }
Output: This code is inside in the try block result of calculation = Ravikant Rest code inside the try block This is finally block This code is outside from the try block
3. finalize
It is not a keyword in java, it is a method that is known as finilize() method. The finalize() method is defined in Object class which is the super most class in Java. This method is called by Garbage collector just before they destroy the object from memory. The Garbage collector is used to destroy the object which is eligible for garbage collection.
public class Data { public static void main(String[] args) { String s = "Hello"; s = null; System.gc(); System.out.println("Garbage collector"); } @Override protected void finalize() { System.out.println("finilize() method called"); } }
Output: Garbage collector