The contains() method in java is declared in Collection interface. But if you are finding about java string contains method then you can click here. The contains method in java is used to check the given element is exist in collection or not. We will discuss it in detail. As we know collection interface is implemented by all the collections class like ArrayList. So this method exists in these classes also. Let’s understand the working of contains method with different collection classes.
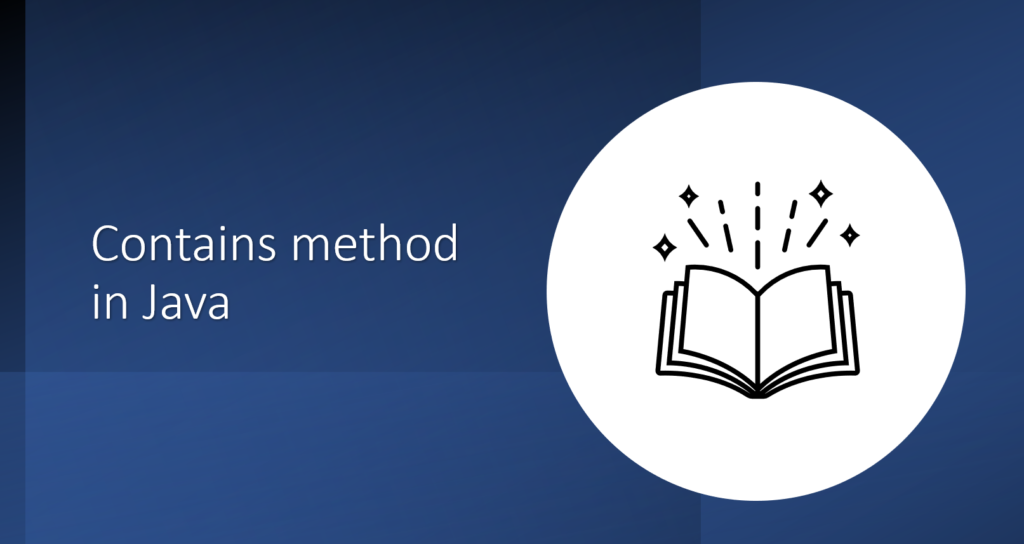
contains() method in java
Contains in Java method is used to check the given element exists in collection or not. It takes a parameter of Object type. It returns boolean value. If the given element exists in collection, then it returns true otherwise false.
boolean contains(Object obj);
Where, Object represents the type of parameter.
obj is the element which we want to check in the collection.
return type: Its return type is boolean. It can be either true or false.
Let’s understand it’s working with different classes ad how we can use it.
contains in ArrayList
The ArrayList class has Contains() method that checks the given object is present in ArrayList or not. In ArrayList contains() method internally finds the index of given method. If index of given object is greater than 0. It means it exists in ArrayList. Let’s see the example.
import java.util.ArrayList; public class ContainsExample { public static void main(String[] args) { ArrayList<String> listOfNames = new ArrayList<String>(); listOfNames.add("JAVA"); listOfNames.add("GOAL"); listOfNames.add("WEB"); listOfNames.add("SITE"); // Return, true because JAVA is exist System.out.println("Is 'JAVA' present in ArrayList = "+listOfNames.contains("JAVA")); // Return, true because JAVAGOAL.COM doesn't exist System.out.println("Is 'JAVAGOAL.COM' present in ArrayList = "+listOfNames.contains("JAVAGOAL.COM")); } }
Output: Is ‘JAVA’ present in ArrayList = true
Is ‘JAVAGOAL.COM’ present in ArrayList = false
Let’s take another example of ArrayList with User defined objects.
import java.util.ArrayList; public class ContainsExample { public static void main(String[] args) { ArrayList<Student> studentArrayList = new ArrayList<Student>(); Student student1 = new Student("Ram", "MCA", 101); Student student2 = new Student("Krishna", "MCA", 105); Student student3 = new Student("Sham", "MCA", 103); studentArrayList.add(student1); studentArrayList.add(student2); studentArrayList.add(student3); Student student4 = new Student("Ram", "MCA", 101); // Return, true because Student1 is exist System.out.println("Is 'Student1' present in ArrayList = "+studentArrayList.contains(student1)); // Return, true because Student4 doesn't exist System.out.println("Is 'Student4' present in ArrayList = "+studentArrayList.contains(student4)); } } class Student { private String name; private String className; private int rollNo; public Student(String name, String className, int rollNo) { this.name = name; this.className = className; this.rollNo = rollNo; } public String getName() { return name; } public void setName(String name) { this.name = name; } public int getRollNo() { return rollNo; } public void setRollNo(int rollNo) { this.rollNo = rollNo; } public String getClassName() { return className; } public void setClassName(String className) { this.className = className; } }
Output: Is ‘Student1’ present in ArrayList = true
Is ‘Student4’ present in ArrayList = false
Here you can see Student1 exists in ArrayList but Student4 doesn’t exits. Even studet1 and student4 has same data but both are different objects.
HashSet contains method
import java.util.ArrayList; import java.util.HashSet; public class ContainsExample { public static void main(String[] args) { HashSet<Integer> hashSetOfNumbers = new HashSet<Integer>(); hashSetOfNumbers.add(1); hashSetOfNumbers.add(2); hashSetOfNumbers.add(3); hashSetOfNumbers.add(4); // Return, true because 1 is exist System.out.println("Is '1' present in HashSet = "+hashSetOfNumbers.contains(1)); // Return, true because 5 doesn't exist System.out.println("Is '5' present in HashSet = "+hashSetOfNumbers.contains(5)); } }
Output: Is ‘1’ present in HashSet = true
Is ‘5’ present in HashSet = false