Here is the table content of the article will we will cover this topic.
1. What is the default method in interface?
2. The need of Default Method?
3. How to achieve multiple inheritances by default methods?
4. Static method in Interface?
Till java 7, Interfaces could have only abstract methods. We have learned a lot of things about interfaces. As we already know a separate class is used to provide the implementation of abstract methods. If the programmer adds a new method to an interface, then the implementation of a new abstract method must be provided by the class which is implementing the interface. To resolve the issue, Java 8 introduced default methods and static methods in interfaces. We will discuss it in detail.
Onward Java 8, You can declare the default methods and static methods in the interfaces. Now the developer can add new functionality to the interface by use of default method without breaking their existing implementation.
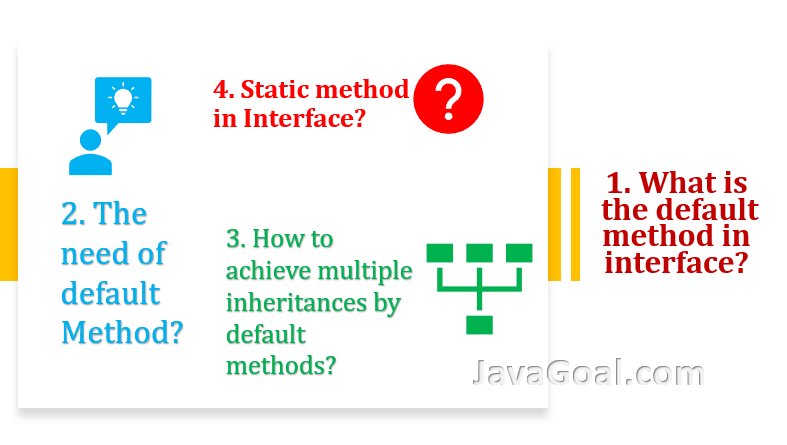
default method in Interface
1. The default methods in the interface are defined with the default keyword.
2. You can call a default method of the interface from the class that provides the
implementation of the interface.
Syntax: To define a default method in the interface, we must use the “default” keyword with the method signature.
interface InterfaceName { default void methodName() { // Body of default method } }
interface InterfaceWithDefaultMethod { default void show() { System.out.println("This is default method"); } void printData(); } public class MainClass implements InterfaceWithDefaultMethod { public void printData() { System.out.println("Implementing abstract method in seprate class"); } public static void main(String arg[]) { MainClass obj = new MainClass(); // Calling default method obj.show(); // Calling implemented method of same class obj.printData(); } }
Output: This is default method
Implementing abstract method in seprate class
In InterfaceWithDefaultMethod interface we are defining a default method show(). As you can see it is not an abstract method, we are providing the implementation to it. So, when we MainClass implements this interface, then there is no need to provide the implementation to show() method.
The need of Default Method?
The default method provides the flexibility to add the default method in the interface without hampering the existing functionality.
1. The default method plays the main role to enable the functionality of lambda expression in java. As you know Lambda expression always uses the functional interface. To provide the support of lambda expressions in all core classes must be modified.
2. But In java, a lot of core classes are written in other languages. We can’t modify them.
3. The JDK framework is very complex if we introduced a new method in the interface. That could break millions of lines of code.
Let’s discuss it with example
As you know forEach() method is introduced in Java 8. To provide the support of forEach() in Collection framework. The forEach() method is defined in the Iterable interface as a default method. So that all those classes that use the Iterable interface can use the forEach() method.
default void forEach(Consumer<? super T> action) { Objects.requireNonNull(action); for (T t : this) { action.accept(t); } }.
Important points:
- The default methods help to extend the functionality of interfaces without breaking the implementation of existing classes.
- By the use of default methods in interfaces, the Collections API was enhanced in Java 8 to support lambda expressions.
How do achieve multiple inheritances by default methods?
Now we have basic knowledge of the default method. Let’s discuss some complex things about the default method.
A class can extend only one class in java but can implement N number of interfaces. Let’s say we have two interfaces having the same default method which are implemented by a single class. The class will get confused which default method to call.
interface Draw { default void show() { System.out.println("This is default method of Draw Interface"); } void DrawData(); } interface Print { default void show() { System.out.println("This is default method of Print Interface"); } void PrintData(); } class ManageRecord implements Draw, Print { public void PrintData() { System.out.println("PrintData method Implemented by ManageRecord class"); } public void DrawData() { System.out.println("DrawData method Implemented by ManageRecord class"); } @Override public void show() { Draw.super.show(); Print.super.show(); } } public class MainClass { public static void main(String arg[]) { ManageRecord obj = new ManageRecord(); obj.DrawData(); obj.PrintData(); obj.show(); } }
Output: DrawData method Implemented by ManageRecord class
PrintData method Implemented by ManageRecord class
This is default method of Draw Interface
This is default method of Print Interface
Static method in Interface
Static method in Interface
1. The static methods in Interface are defined with the static keyword.
2. You can define static default methods in the interface. The static method will be available for all instances of the class that implements the interface.
Syntax: To define a static default method in the interface, we must use the “static” keyword with the method signature.
interface InterfaceName { static void methodName() { // Body of default method } }
interface InterfaceWithStaticMethod { static void show() { System.out.println("This is static default method"); } void printData(); } public class MainClass implements InterfaceWithStaticMethod { public void printData() { System.out.println("Implementing abstract method in separate class"); } public static void main(String arg[]) { MainClass obj = new MainClass(); // Calling static default method InterfaceWithStaticMethod.show(); // Calling implemented method of same class obj.printData(); } }
Output:This is static default method
Implementing abstract method in separate class
separate
I do not even know how I ended up here, but I thought this post was great.
I do not know who you are but definitely you’re going to
a famous blogger if you aren’t already 😉 Cheers!
Hi antwan.
I’m very much agree to your words.
He is definitely a great blogger…
Thanks you.
Very good detailed information. Thank you