In the last post, we discussed the static keyword in java. We can use a static keyword with a variable, block, method, and class. In this post, we will discuss the static variable in java. Most of us already knew some things about static, but to get more clarity we will discuss it in detail.
Here is the table content of the article will we will cover this topic.
1. What is the static variable?
2. Properties of static variable?
3. How to declare a static variable?
4. How to access the static variable?
5. How to use a static variable with example?
6. How does the static variable work?
What is a static variable?
A variable declared with a static keyword is known as a static variable in java. If we declare a variable with a static keyword the JVM loads it during the class loading. A static variable is known as a class variable. Because the static variable belongs to the class instead of the instance/objects. It means a static variable is common to all the objects of the class.
I know you must hear this line thousands of times, but I will tell you what the meaning of the above line is.
Each non-static variable belongs to an object. Whenever we create an object, a copy of non-static members store in memory and associated with the object. It means each object has its own non-static variables. That’s why we called a variable belonging to the object.
But a static variable doesn’t belong to an object. It means when we create an object, the static variable doesn’t store with object memory and it stores in a static pool. The static variables are associated with class, which means all objects share the same variable from a single memory location.
Properties of static variable
A static variable in java has some unique proprieties that make it different from another variable. A static variable totally differs from other variables. To get more clarity we need to understand all the properties of the static variables.
1. Static variable memory
A static variable always stores in the static pool. It is the memory area that stores the static variables, static class metadata, etc.
2. Initialization of static variable
The Initialization of the static field totally different from other fields. When JVM loads a class it loads the static variables and initializes them. The lifetime of the static variable exists until JVM destroys the class. Initialization of Static Variable is not Mandatory. Its default value is depending on the data type of variable.
3. static variable belongs to the class
The static variable link with class rather than instance/object. A static member doesn’t create a separate copy for each instance. As it stores in a static pool which is common for each instance.
4. Common for all objects
As you know static variables stores in the static pool and the static pool are accessible by each object. A static variables are common for all the objects, it doesn’t associate with objects. Unlike the instance variable, it doesn’t create a copy for each object. If any object makes a change in the value of a static variable, then it will reflect for all objects
5. Scope
You can’t declare a static variable within the method, a block of code, constructor. because the static variable always binds with the class. We can only declare it at the class level because JVM binds it at the time of class loading.
6. Access to the static variable
A static variable is accessible by the class name. Unlike instance variables, we don’t need to create an object to access them. If we access the static variable through an object the compiler will show the warning message. The compiler will replace the object name with the class name automatically.
How to declare a static variable in java?
We can declare a static variable by use of the static keyword. The static keyword indicates the JVM to load it when the class gets load. You can initialize it at the time of declaration but if you don’t initialize it the JVM provides its default value according to the data type.
static dataType variableName = value;
static is a reserved word or keyword in java.
datatype, is the type of data.
variableName is the name of the variable.
Value you can provide the value to a static variable.
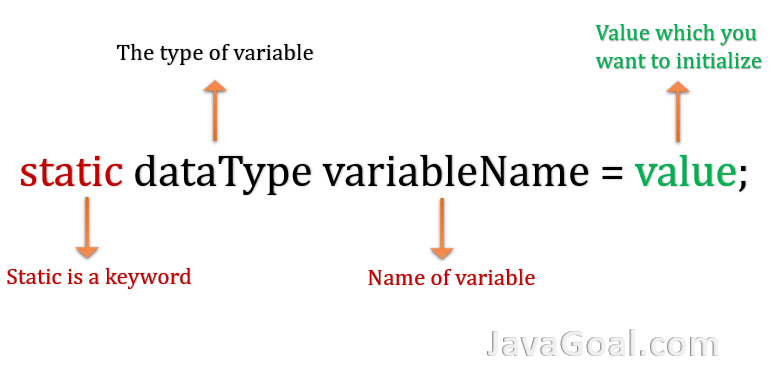
How to access the static variable in java?
To access a static variable we don’t need to create an object. The static variable can directly access the class name.
ClassName.variableName
ClassName, is the name of the class
.(dot), uses to access to a variable through the class name.
variableName, the name of the static variable which you want to access.
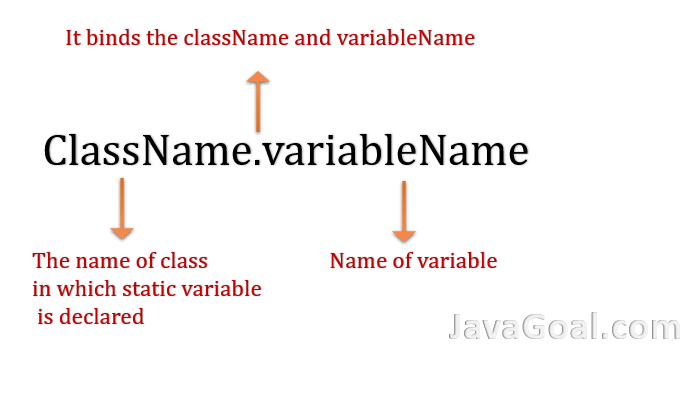
How to use a static variable with an example
Let’s see how we can declare a static variable and how we can access it. We will declare some static variables.
public class StaticVariableExample { // Declaring static variable static int a; static int b = 1; static String s; static String s1 = "Hi"; public static void main(String[] args) { // Access of static variable by class name System.out.println("Value of a : "+ StaticVariableExample.a); System.out.println("Value of b : "+StaticVariableExample.b); System.out.println("Value of s : "+StaticVariableExample.s); System.out.println("Value of s1 : "+StaticVariableExample.s1); } }
How does the static variable work?
A static variable in Java is common to all the objects of the class. Because the static variable link with a class. When a static variable loads in memory (static pool) it creates only a single copy of the static variable and shares it among all the objects of the class.
If we make any change in the value through any object, then it reflects in other objects also. We will discuss this by scenario.
Example: Let’s take an example. The user wants to count how many numbers of objects are calling the same function.
Firstly, we will discuss without static
class ExampleWithoutStatic { int numberOfClick = 0; public void incrementCountAndPrint() { numberOfClick++; System.out.println("Value of count = " +numberOfClick ); } public static void main(String args[]) { ExampleWithoutStatic object1 = new ExampleWithoutStatic(); object1.incrementCountAndPrint(); ExampleWithoutStatic object2 = new ExampleWithoutStatic(); object2.incrementCountAndPrint(); ExampleWithoutStatic object3 = new ExampleWithoutStatic(); object3.incrementCountAndPrint(); } }
Output:
Value of count = 1
Value of count = 1
Value of count = 1
Memory presentation:
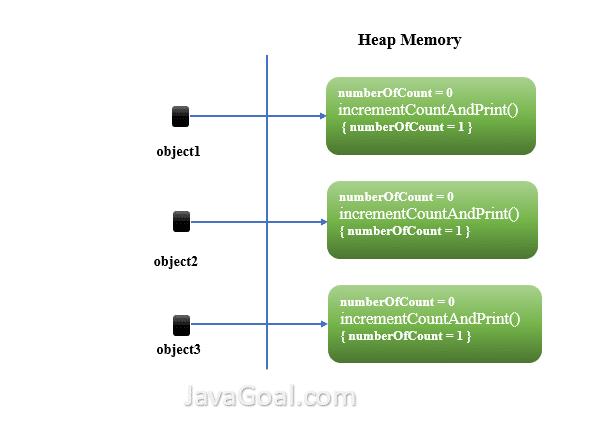
We declared an instance/class variable count. Each object creates(Objects always created in heap memory) its own copy in memory. When we called the incrementCountAndPrint() method by object1 then it increments the value of count. But it doesn’t make any change in other objects’ values. So, when we called the same method by object2 it makes changes to its own memory.
Now, we will discuss it with a static variable
public class ExampleWithStatic { static int numberOfClick = 0; public void incrementCountAndPrint() { numberOfClick++; System.out.println("Value of count = " +numberOfClick ); } public static void main(String args[]) { ExampleWithStatic object1 = new ExampleWithStatic(); object1.incrementCountAndPrint(); ExampleWithStatic object2 = new ExampleWithStatic(); object2.incrementCountAndPrint(); ExampleWithStatic object3 = new ExampleWithStatic(); object3.incrementCountAndPrint(); } }
Output: Value of count = 1
Value of count = 2
Value of count = 3
Memory presentation:
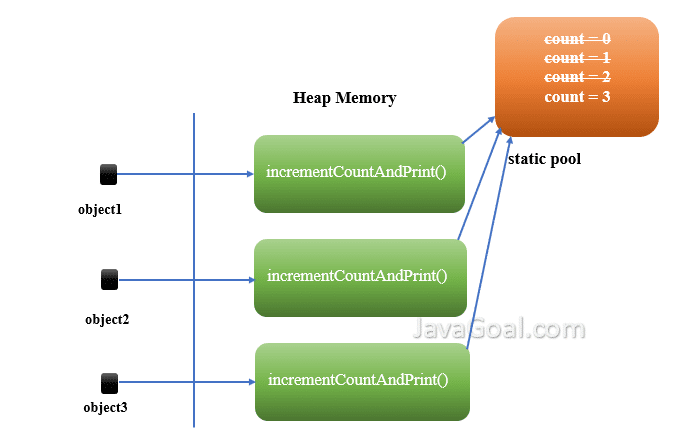
We declared a static variable count. The static variable always stores in a static pool. Each object shares the variable from a static pool. When we called the incrementCountAndPrint() method by object1 then it increments the value of the count and stores it in the static pool. Now, object2 will access the incremented value of the count. So, when we called the same method by object2 it will increment the value again.
static and non-static variables in java
The static and non static variable in java. Now we will see the difference between static and non static variable in java and what is the use of static variables and non-static variables.
Static variable
If we use a static keyword with a variable then it considers a static variable in java. It shares by all the objects of the class. A static variable doesn’t belong to an object. It means when we create an object, the static variable doesn’t store with object memory and it stores in a static pool. The static variables link with the class, which means each object shares the same variable from a single memory location.
Non-static variable
There are two types of non-static variables.
1. Local variables
2. Instance variables
1. local variables
A variable declared inside the body of a method or block or constructor has known as a local variable. You can’t access these variables outsides of the method. JVM provides memory to these variables when the function/block/constructor gets invoke and destroys them after the completion of the execution. We can access these variables only within that block because the scope of these variables exists only within the block. The initialization of the local variables is mandatory. If you don’t initialize the variable compiler will throw a run time exception. You can read it in a separate post.
2. Instance Variables
An instance variable always declares outside the method/block/constructor but inside the class. It doesn’t use the static keyword because instance variables are non-static variables. You can read it in a separate post.
- When an object of the class is created then the object also creates one copy of the instance variable and is destroyed when the object is destroyed.
- We can specify the access specifier for instance variables. By default, the default access specifier will be used.
- Initialization of Instance Variable is not Mandatory. Its default value is depending on the data type of variable.
- These variables can be accessed by creating objects.
An important point about instance variables: Every object has its own separate copy of the instance variables. If we created two objects of a class, then each object contains its own instance variables.
Static vs Non-static variable in java
1. A static variable is known as a class variable because it belongs to the class.
2. A static variable is common for each object of the class. It doesn’t create a separate copy in each object.
3. Static variable stores in the static pool. Which is shared memory for each object
4. A static member can’t declare within a method or constructor because it is a class variable that must be associated with the class.
5. All the static variable loads when JVM loads the class and are destroyed when JVM unloads the class.
6. A static variable can be accessed in a static method and a nonstatic method
7. A static variable can be accessed by class name.
1. A non-static variable doesn’t belong to the class. They are directly associated with objects
2. A non-static variable doesn’t belong to objects. They are directly associated with objects
3. A non-static variable doesn’t store in the static pool. They store in heap memory with objects.
4. A non-static variable can declare within a method or constructor. because it can be a local variable or instance variable.
5. The non-static variables always load in memory in memory after the static variables. Suppose a non-static variable declared in the method then must be loaded when the method will get a call. But if it is an instance variable then it will be loaded at the time when the class gets loaded.
6. A non-static method can’t be accessed in a static method. You can access it only in the nonstatic method.
7. A non-static variable can’t be accessed by the class name. You need to create an object to access them.
Thanks java Goal .I found the best website.I have cleared my all doubts .This website provide best content .
very impressive and you are doing great job.
Can u share me about accessibility modifiers in mail
I have shared it with you on Gmail.
One of the best site I ever see.. the way you explain everything is just superb
Greatly explained
Hi,
Can you share with me an example for the below point.
6. Access of static variable
A static variable can direct class by use of the class. Unlike instance variables, we don’t need to create an object to access them. If we access the static variable through an object the compiler will show the warning message. The compiler will replace the object name to the class name automatically.
Here is example : https://javagoal.com/static-variable-in-java-2/#4
why local variables are intialization mandatary?
Thanks you vary gooooood