Java has two types of data types primitive data types and non-primitive data types. The Wrapper class is considered in non-primitive data types. But it was introduced to support the primitive data type. In this post, we will see what is wrapper class? and what is the use of wrapper class?
Here is the table content of the article we will cover this topic.
1. Wrapper class in java?
2. Why do we need wrapper classes in java? OR Use of wrapper class in java?
3. Wrapper class in java with example?
4. Autoboxing and unboxing in java?
5. Custom Wrapper class in Java?
Wrapper class in java
As we know Java is not a pure object-oriented programming language, because there are 8 primitive data types. An object-oriented programming language is all about objects. But the eight primitive data types byte, short, int, long, float, double, char, and boolean are not objects. To use these 8 primitive data types in the form of objects we use wrapper classes. It is used to convert a primitive data type to an object. When we create an object of the wrapper class, it contains the value of the primitive data type. The object of the wrapper class wraps the value of primitive data types.

Why do we need wrapper classes in java? OR Use of wrapper class in java?
1. As we know java supports call by value. Suppose we are passing a primitive data type in the method then it will not change in the original value. But the wrapper class resolves this problem and they convert primitive data types into objects.
2. All the classes in java.util package can handle only objects So there was only one choice to use the primitive data types to make their wrapper classes.
3. As we know in Serialization, we convert the objects into streams to perform the serialization. So, we can use the primitive values through the wrapper classes.
4. Collection framework is totally based on objects. We can’t create any collection by use of primitive data types. But we can use the Wrapper classes to use primitive data values.
Wrapper class in java with example
Let’s see all the wrapper classes in java with their example.
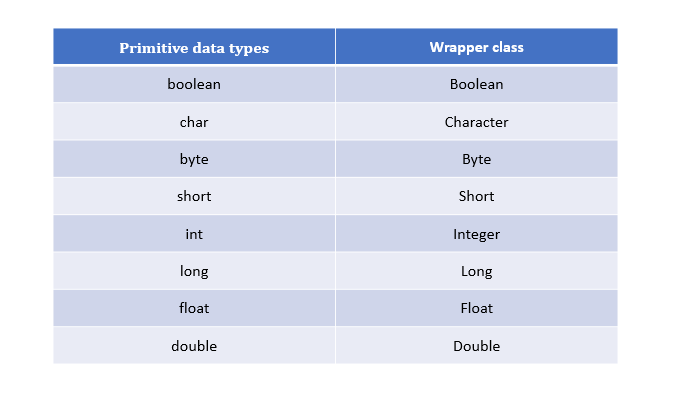
public class WrapperClassExample { public static void main(String arg[]) { boolean a = false; char b = 'b'; byte c = 1; short d = 2; int e = 3; long f = 4; float g = 5; double h = 6; System.out.println("Value of boolean: "+ a); System.out.println("Value of char: "+ b); System.out.println("Value of byte: "+ c); System.out.println("Value of short: "+ d); System.out.println("Value of int: "+ e); System.out.println("Value of long: "+ f); System.out.println("Value of float: "+ g); System.out.println("Value of double: "+ h); Boolean a1 = a; Character b1 = b; Byte c1 = c; Short d1 = d; Integer e1 = e; Long f1 = f; Float g1 = g; Double h1 = h; System.out.println("Value of boolean: "+ a1); System.out.println("Value of char: "+ b1); System.out.println("Value of byte: "+ c1); System.out.println("Value of short: "+ d1); System.out.println("Value of int: "+ e1); System.out.println("Value of long: "+ f1); System.out.println("Value of float: "+ g1); System.out.println("Value of double: "+ h1); } }
Output: Value of boolean: false
Value of char: b
Value of byte: 1
Value of short: 2
Value of int: 3
Value of long: 4
Value of float: 5.0
Value of double: 6.0
Value of boolean: false
Value of char: b
Value of byte: 1
Value of short: 2
Value of int: 3
Value of long: 4
Value of float: 5.0
Value of double: 6.0
Autoboxing and unboxing in java
Autoboxing and unboxing are used when we want to convert the primitive data type to the Wrapper class and the Wrapper class to the primitive data type. Java provides the automatic conversion of objects and primitive data types.
AutoBoxing: Autoboxing is a process in which JVM Automatically converts the primitive data type to the object of their corresponding wrapper class. For example, int to Integer, float to Float etc.
public class WrapperClassExample { public static void main(String arg[]) { // Autoboxing Boolean a = false; Character b = 'b'; Byte c = 1; Short d = 2; Integer e = 3; Long f = 4l; Float g = 5f; Double h = 6d; System.out.println("Value of boolean: "+ a); System.out.println("Value of char: "+ b); System.out.println("Value of byte: "+ c); System.out.println("Value of short: "+ d); System.out.println("Value of int: "+ e); System.out.println("Value of long: "+ f); System.out.println("Value of float: "+ g); System.out.println("Value of double: "+ h); } }
Output: Value of boolean: false
Value of char: b
Value of byte: 1
Value of short: 2
Value of int: 3
Value of long: 4
Value of float: 5.0
Value of double: 6.0
Unboxing: Unboxing is a process in which JVM automatically converts the object of the wrapper class to its corresponding primitive type. It is the reverse process of autoboxing. It internally invokes the valueOf() method of the wrapper class.
public class WrapperClassExample { public static void main(String arg[]) { boolean a = new Boolean(false); char b = new Character('b'); byte c = new Byte((byte) 1); short d = new Short((short) 2); int e = new Integer(3); long f = new Long(4); float g = new Float(5); Double h = new Double(6); System.out.println("Value of boolean: "+ a); System.out.println("Value of char: "+ b); System.out.println("Value of byte: "+ c); System.out.println("Value of short: "+ d); System.out.println("Value of int: "+ e); System.out.println("Value of long: "+ f); System.out.println("Value of float: "+ g); System.out.println("Value of double: "+ h); } }
Output: Value of boolean: false
Value of char: b
Value of byte: 1
Value of short: 2
Value of int: 3
Value of long: 4
Value of float: 5.0
Value of double: 6.0
Custom Wrapper class in Java
As we know java Wrapper classes are used to wrap the primitive data types. We can also create a class that wraps a primitive data type. So, let’s see how to define wrapper class in java
class Test { private int i; Test(){} Test(int i) { this.i=i; } public int getValue() { return i; } public void setValue(int i) { this.i=i; } @Override public String toString() { return Integer.toString(i); } } //Testing the custom wrapper class public class WrapperClassExample { public static void main(String[] args) { Test i = new Test(10); System.out.println(i); } }
Output: 10