As per our recent discussion on Consumer, Now you have some knowledge of the Consumer interface. In this article, we will read Why consumers in java 8 and a real-time java consumer example?
As you already know Consumer interface consumes the object and doesn’t return the result. In the Consumer interface we have an abstract method accept(T t). Whenever you are using a Consumer interface then you must have to provide the body to accept(T t) method. You can implement it by using lambda expression or by using implements keyword. By using the Consumer interface the lambda expression provides optimized code. You can perform any operation on the object without returning the values. In Java 8, Streams have forEach loop that expects a Consumer as input so that it can perform the operation on it. Let’s see a java consumer example:
void forEach(Consumer<? super T> action);
It accepts the Consumer as input, and You can perform the operation on the object.
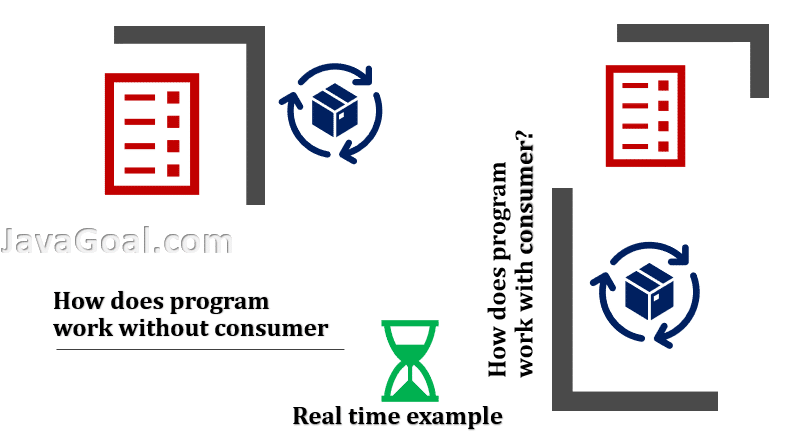
Use of Consumer in Real-life example
Let’s say you have a record of the library and want to print the name of the Books in capital letters. So, you must have to perform some operations on the name of each Book. So, we will do this task with two approaches. The first approach goes without Consumer and the second with Consumer.
Here is the first approach without the Consumer
public class Book { int bookId; String name; String authorName; public Book(int bookId, String name, String authorName) { this.bookId = bookId; this.name = name; this.authorName = authorName; } public int getBookId() { return bookId; } public void setBookId(int bookId) { this.bookId = bookId; } public String getName() { return name; } public void setName(String name) { this.name = name; } public String getAuthorName() { return authorName; } public void setAuthorName(String authorName) { this.authorName = authorName; } }
import java.util.ArrayList; import java.util.List; public class ExampleWithoutConsumer { public static void main(String[] args) { Book javaBook = new Book(123, "java begin", "RKK"); Book digitalBook = new Book(432, "Digital logics", "KG"); Book mathematicsBook = new Book(412, "mathmaticsc", "DL saini"); List<Book> books = new ArrayList<Book>(); books.add(javaBook); books.add(digitalBook); books.add(mathematicsBook); // print all the name of books System.out.println("Printng by use of loop"); for(Book book : books) { System.out.println("Book Name: "+ book.getName().toUpperCase()); } } }
Output: Printing by use of loop
Book Name: JAVA BEGIN
Book Name: DIGITAL LOGICS
Book Name: MATHMATICSC
In the above example, we are printing the name of books and performing one operation on the book’s name.
Here is the second approach with the Consumer:
public class Book { int bookId; String name; String authorName; public Book(int bookId, String name, String authorName) { this.bookId = bookId; this.name = name; this.authorName = authorName; } public int getBookId() { return bookId; } public void setBookId(int bookId) { this.bookId = bookId; } public String getName() { return name; } public void setName(String name) { this.name = name; } public String getAuthorName() { return authorName; } public void setAuthorName(String authorName) { this.authorName = authorName; } }
import java.util.ArrayList; import java.util.List; public class ExampleWithConsumer { public static void main(String[] args) { Book javaBook = new Book(123, "java begin", "RKK"); Book digitalBook = new Book(432, "Digital logics", "KG"); Book mathematicsBook = new Book(412, "mathmaticsc", "DL saini"); List<Book> books = new ArrayList<Book>(); books.add(javaBook); books.add(digitalBook); books.add(mathematicsBook); // print all the name of books System.out.println("Printng by use of loop"); books.forEach(book -> System.out.println("Book Name: "+ book.getName().toUpperCase())); } }
Output: Printing by use of loop
Book Name: JAVA BEGIN
Book Name: DIGITAL LOGICS
Book Name: MATHMATICSC
In this example printing the book names by use of forEach. In JAVA, Streams have forEach method that takes Consumer as input.
So now you can see we are printing all the data in one line. So, it makes code more readable and optimized.