Exception in java is one of the important topics because every programmer uses this concept to control the execution of the program if anything unexcepted happens. In this post, we will understand the concept of exception handling in java and see what is the meaning of exception handling.
Here is the table content of the article will we will cover this topic.
1 What is Exception in java?
2. Exception Handling in Java?
3. Exception Hierarchy in java?
4. Checked Exceptions in java?
5. Unchecked exception in java?
6. How To handle Exceptions in java?
7. How to handle null pointer exception in java?
8. How an Exception Handled by JVM?
What is Exception in java?
During the execution of a program if any unwanted or unexpected error comes that is called an exception. It means if anything happens wrong during the execution of a program and disrupts the normal flow of the program’s instructions is called an exception. Exceptions may occur for different reasons:
- If the user has entered the wrong data. Like the user is trying to divide any value by 0 e.g. 5/0.
- If the user tries to open a file with an invalid name.
- If a user tries to access an array with a negative index value.
- If a user tries to print a page but the printer doesn’t find it.
Real-life example: Let’s take an example of a courier company. If I am sending a gift to my friend but due to some reason the courier company is failed to deliver. That is called an exception because this is an unexpected thing that happened during the normal delivery of our product. If a company handle these types of problem and send a notification to me about why they were not able to deliver it on time. This handling is called exceptional handling.

In Java, if any program is disrupted by an exception during execution. Then exception handling is used to handle exceptions.
Example of exception:
class FirstExampleForException { public static void main(String args[]) { String str = null; System.out.println(str.length()); } }
Output : Exception in thread “main” java.lang.NullPointerException at FirstExampleForException.main(FirstExampleForException.java:4)
Explanation of example: When users try to run this program then NullPointerException will be thrown. Because str is NULL.
Exception Handling in Java
It is a mechanism to handle exceptions during the execution of the program and maintain the normal flow of execution. In other words, we can say it is a way to handle runtime errors. Java provides many keywords to handle the exceptions.
There are two types of Exceptions, Checked exception in java and unchecked exception in java. We will cover them one by one. Java provides some keywords to handle an exception, the try catch finally java is the keyword that is used to control the exception.
1. try keyword: The try keyword is used on the block of statements where we are excepting an exception. If any exception occurs in the try block then the control jumps to the next block which will be the catch block or finally block. But it will handle the situation and the execution of the program will not be stopped. You can read it in detail.
2. catch keyword: The catch keyword is used with a block that is known as a catch block. The catch block catches the exception that is through by try block. We can catch the exception and print the reason of the exception. You can read it in detail.
3. finally keyword: This keyword is also used with a block but it is a special block because it always executes whether any error occurs or not. We can perform some important operations like closing the DB connection. You can read it in detail.
public class ExceptionHandling { public static void main(String[] args) { try { System.out.println("Before exception"); int a = 5/0; System.out.println("After exception"); } catch(Exception e) { System.out.println("From catch block"); } finally { System.out.println("From finally block"); } } }
Output: Before exception
From catch block
From finally block
Exception hierarchy in java
We learned about Exception handling in java. The exception hierarchy in java is the most essential part to handle the exception. In Java, there is a lot of exception but there is some rule to handle them so we need to take care of the exception class hierarchy in java. As of now, you might not be able to understand the benefit of the hierarchy of exceptions but when you will work on a big project you have to use the particular exception that occurs at a time. Let’s discuss with an example:
Suppose you are writing a program of String concatenation and you want to join the string. But there is one object of String that is null. It will throw NullPointerException. According to best practices, you should place NullPointerException between the braces of the catch. Even you can place an Exception between the braces of the catch.
public class ExceptionHandling { public static void main(String[] args) { try { String str = null; str.concat("Hi"); } catch(NullPointerException e) { e.printStackTrace(); } } }
Output: java.lang.NullPointerException
at ExceptionHandling.main(ExceptionHandling.java:8)
The most common question of the interview which is the class at the top of exception class hierarchy is? The answer is simple, Throwable is a superclass of all exception classes. All the classes which are used to handle exceptions and errors are subclasses of Throwable. It is subdivided into two branches one branch is Exception and another is Error.
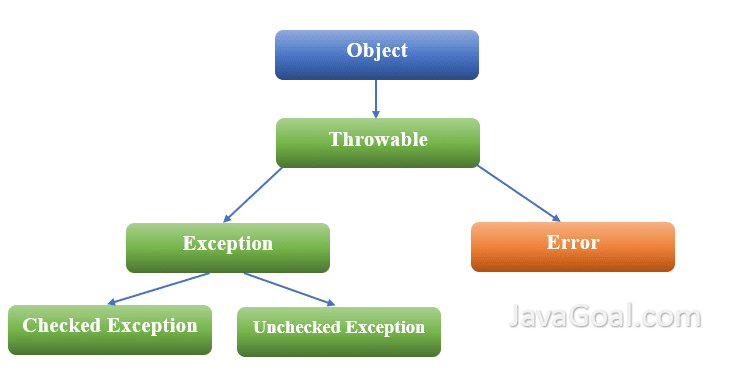
java.lang.Exception
All exception classes are a subclass of the java.lang.Exception class. If a user doesn’t know about the exception, then he/she can provide the Exception class in the catch block. But it doesn’t consider as good programming.
Let’s take an example to explain the use of the Exception class: In this example, the user doesn’t know what type of exception was thrown by the system. If you don’t know anything about exceptions you can directly use the Exception class because it will automatically handle all the subclasses.
public class ExampleOfException { public static void main(String[] args) { int a = 25; try { int b = a/0; //it will throw exception } catch(Exception e) { System.out.println(e); } } }
Error
some types of errors may be coming at runtime and this type of error is indicated by JVM. For example, hardware failure or out-of-memory error. These types of situations are not handled by any programmer because they are unpredictable.
Checked exceptions in java
Checked exceptions in java are also known as compile-time exceptions because these exceptions are checked by the compiler at compile-time. If any method throws a checked exception, then it is the programmer’s responsibility either handle the exception or throw the exception by using the throws keyword. We can’t ignore these exceptions at compile time. The JVM checks these exceptions at the time of compilation time.
checked exception example
Lets us take an example of checked exceptions in java or compile-time exception. In this example, we are trying to read data from a file that is on our PC. So, we are creating a class CheckedExceptionExample and reading a file text with the help of FileReader. So firstly, we need to specify the address of the file which we want to read. There are two possibilities that the database can contain that file or maybe not.

import java.io.BufferedReader; import java.io.FileReader; class CheckedExceptionExample { public static void main(String[] args) { FileReader fileReader = new FileReader("D:\\abc.txt"); BufferedReader bufferReader = new BufferedReader(fileReader); // We trying to print first line only so we counter should be less than 1 for (int counter = 0; counter < 1; counter++) System.out.println(bufferReader.readLine()); bufferReader.close(); } }
Output: Exception in thread “main” java.lang.Error: Unresolved compilation problems: Unhandled exception type FileNotFoundException Unhandled exception type IOException Unhandled exception type IOException at CheckedExceptionExample.main(CheckedExceptionExample.java:6)
Explanation of example: This exception is thrown by the compiler at compile time when if the file is not found at the specified location. When we are trying to read any file in our program then the compiler tells the programmer to handle the FileNotFoundException and IOException exception.
We can resolve this problem we have two solutions:
- By using throws (Discuss it later)
- By using a try-catch block.
We can resolve this problem by using a try-catch block. As we discuss in the above example it is a programmer’s responsibility to handle Checked exceptions. So, we need to put the code in a try block and catch the exception in the catch block. FileNotFoundException is a subclass of IOException so we can use the superclass to handle both types of exceptions.
import java.io.BufferedReader; import java.io.FileReader; import java.io.IOException; class CheckedExceptionExample { public static void main(String[] args) { try { FileReader fileReader = new FileReader("D:\\abc.txt"); BufferedReader bufferReader = new BufferedReader(fileReader); for (int counter = 0; counter < 1; counter++) System.out.println(bufferReader.readLine()); bufferReader.close(); } catch(IOException e) { e.printStackTrace(); } } }
Output: It will print first line of your specified file
Unchecked exception in java
An unchecked exception in java is an exception that is not checked at the compiled time. The compiler is not able to recognize these exceptions at compile time. An unchecked exception in java occurs at the time of execution, so they are also called Runtime Exceptions. For example NullPointerException, ArithmeticException, ArrayIndexOutOfBound, logic error, etc.
unchecked exception example
Lets us take an example of an unchecked exception or runtime exception. In this example, we are trying to print some strings and do some operations on them. So, we are creating a class UncheckedExceptionExample and after that, we are printing some names and append the strings.
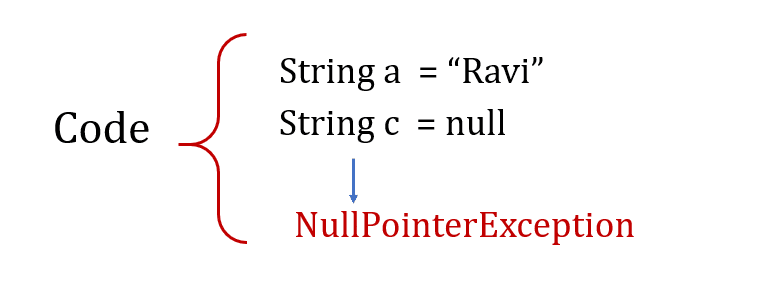
class UncheckedExceptionExample { public static void main(String[] args) { String a = "Ravi"; String b = "Ram"; String c = null; // Now print all the strings System.out.println("First name = " +a); System.out.println("Second name = " +b); System.out.println("Third name = " +c); // concatenating string System.out.println("Concatenation of String = " + c.concat(a)); } }
Output: First name = Ravi Second name = Ram Third name = null Exception in thread “main” java.lang.NullPointerException at UncheckedExceptionExample.main(UncheckedExceptionExample.java:14)
Explanation of example: NullPointerException is thrown by the compiler at runtime time. Because we declare a String reference variable that was pointing to null. We can’t call any method by use of null. So, this program throws NullPointerException.
We can resolve this problem we have two solutions:
- By using throws (Discuss it later)
- By using a try-catch block.
We can resolve this problem by using a try-catch block. So, we need to put the code in a try block and catch the exception in the catch block.
class UncheckedExceptionExample { public static void main(String[] args) { String a = "Ravi"; String b = "Ram"; String c = null; try { // Now print all the strings System.out.println("First name = " +a); System.out.println("Second name = " +b); System.out.println("Third name = " +c); // concating string System.out.println("Concatination of String = " + c.concat(a)); } catch(NullPointerException e) { System.out.print("NullPointerException exception is occured"); } } }
Output: First name = Ravi
Second name = Ram
Third name = null
NullPointerException exception is occured
How to Handle Exception in Java
An Exception is an event that occurs during the exception of the program and disrupts the normal executions. In recent posts, we have seen what can be the causes of exception and how it breaks the normal flow of executions. In this post, we will see How to handle exception in java.
There are a few keywords that are used in exception handling in Java. These keywords will you help to understand How to handle exceptions in java?
1. try
2. catch
3. finally
4. throw
5. throws
Let’s start with the basic example and then move to advance topics. The try block and catch block is the first step to handle any type of exception. In the try block, we put the code that can be the reason for the exception.
Suppose we are dividing numbers then we should put the code in a try block because there may be a chance the divisor can be 0. If any exception occurs in the try block, the try block takes control and through the control flow to the catch block.
try { // block of code that can throw exceptions } catch (Exception ex) { // Exception handler }
We can’t use try block without catch or finally block.
When a try block has thrown the exception, now catch block comes in the role. The catch block catches the exception and takes the appropriate action on the exception. We can have any number of catch blocks.
try { // block of code that can throw exceptions } catch (ExceptionType1 ex1) { // exception handler for ExceptionType1 } catch (ExceptionType2 ex2) { // Exception handler for ExceptionType2 }
The code that we want to execute irrespective of the occurrence of an exception is put in a finally block. It means the Finally block always executes whether the exception occurs or not. In other words, a finally block executes in all circumstances. This block is used to perform mandatory operations like closing the connection.
try { // block of code that can throw exceptions } catch (ExceptionType1 ex1) { // exception handler for ExceptionType1 } finally { //Mandatory code }
How to handle null pointer exception in java
Whenever we perform any operation on null, the compiler throws the NullPointerException exception. Suppose you have a two-string and you want to check the equality of two strings. But there is one string object that is null and another holding value.
public class ExceptionHandling { public static void main(String[] args) { String str1 = null; String str2 = "Hello"; boolean isEqual = str1.equals(str2); System.out.println("Are they equals: "+isEqual); System.out.println("Bye"); } }
Output: Exception in thread “main” java.lang.NullPointerException
at ExceptionHandling.main(ExceptionHandling.java:7)
Here you can see the program is showing NullpointerException. It is not showing any output when it encountered null and breaks the flow of execution. Let’s see how we can handle it.
We can pull this code try block and handle o flow of execution.
public class ExceptionHandling { public static void main(String[] args) { String str1 = null; String str2 = "Hello"; boolean isEqual = false; try { isEqual = str1.equals(str2); } catch(NullPointerException e) { System.out.println("There is null string"); } System.out.println("Are they equals: "+isEqual); System.out.println("Bye"); } }
Output: There is null string
Are they equals: false
Bye
How an Exception Handled by JVM
Default Exception Handling: If any exception has occurred in the method then the method creates an object known as an exception Object and this object will be handled by JVM. When a method creates an exception object and hands over it to JVM it’s called throwing an Exception. This exception object holds all the details about exceptions like the name, and description of the exception, and retains the state of the program where an exception occurred. There may be a chance when multiple exceptions occurred in different methods. This ordered list of methods is called Call Stack.
Now here is the procedure that will happen.
- First, the run-time system finds the method in the call stack that has an Exception handler. Through exception handler, if any method contains a block of code that can handle the occurred exception.
- The run-time system starts searching which methods were called. It means the run-time system tries to find the caller.
- If it finds any handler for a thrown exception(a type of exception object thrown matches the type of the exception used in the handler).
- If the run-time system(JVM) doesn’t find the appropriate handler then the default exception handler will handle the exception, which is part of the run-time system. It prints the exception and terminates the program abnormally.