String in Java is one of the most important topics of java. Java string can be created in a number of ways. In this tutorial, we will see how to create a java substring. Here we will see what substring in java is and how to get substring java
Here is the table content of the article will we will cover this topic.
1. What is a substring in Java?
2. public String substring(int startIndex)
3. How does Substring in Java work in memory?
4. public String substring(int startIndex, int endIndex)
5. Important points about substring index java
6. String palindrome program in java
7. Find a substring in a string by char
1. What is a substring in Java?
A string is a group of characters or an array of characters. In java substring can be part of a String or a whole string as well. On other hand, we can say substring is a subset of String. Java provides some methods to find substring in string java.
Suppose we have a string “JavaGoal”, it has a number of substrings like “Java”, “Goal”, “va”, “ja”, “JavaGoal” etc.
To find substring in string java provides substring() method, which is overloaded.
String substring(int begIndex) String substring(int beginIndex, int endIndex)
We have two variants of the substring() method, the first method accepts one parameter, just begin index, and the second method accepts two parameters, begin index and end index. Both methods work based on the index. Let’s see the substring index java:
2. public String substring(int startIndex)
This method returns the substring of the string. It takes an integer value as a parameter which is called the index of the string. It will return a substring starting from the specified index i.e startIndex and extends to the character present at the end of the string.
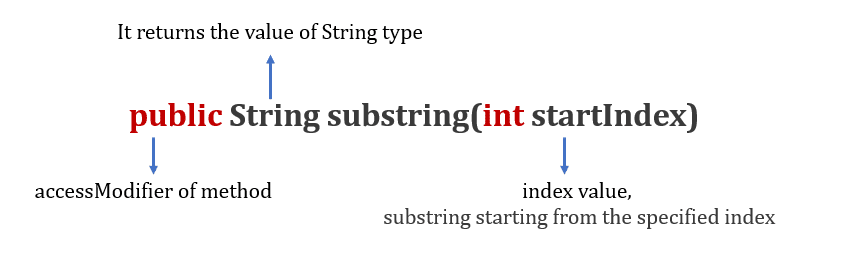
public: The access modifier of the method is public so it can be accessed from everywhere.
returnType: It returns a String type value.
startIndex: It accepts only integer value, But we can’t provide a negative value if we will provide it will throw StringIndexOutOfBoundsException. The startIndex value is inclusive in the substring.
exception: It throws StringIndexOutOfBoundsException if the index is greater than the length or less than 0.
String subStringName = stringName.subString(startIndex)
class ExampleOfSubString { public static void main(String args[]) { String nameOfUniv = "KUK University"; String subStringOfUnivName = nameOfUniv.substring(1); System.out.println(subStringOfUnivName); } }
Output: UK University
As you know, strings are stored like an array. In the above example, we have a string nameOfUniv that contains the value “KUK University”. So, we are getting a substring from index 1 and it is returning the substring which is “UK University”. It started with index 1 and extends to the character present at the end of the string.
3. How does Substring in Java work in memory?
The string always stores in the form of an array. It started with index 0 and ends with length-1. Here we have the string “KUK University” and its length is 14. So, it started with an index 0 and ends with 13.
K | U | K | U | n | i | v | e | r | s | i | t | y | |
0 | 1 | 2 | 3 | 4 | 5 | 6 | 7 | 8 | 9 | 10 | 11 | 12 | 13 |
When substring() method with startIndex 1 then it directly jumps to index 1 and returns the string.
public class SubStringExample { public static void main(String arg[]) { String str = "JavaGoal.com"; // Substring starts from index 0 System.out.println("Substring start from index 0 : "+str.substring(0)); // Substring start from index 2 System.out.println("Substring start from index 2 : "+str.substring(2)); // Substring start from index 4 System.out.println("Substring start from index 4 : "+str.substring(4)); // Getting last character int legnth = str.length(); System.out.println("Substring from last character : "+str.substring(legnth-1)); } }
Output: Substring start from index 0 : JavaGoal.com
Substring start from index 2 : vaGoal.com
Substring start from index 4 : Goal.com
Substring from last character : m
4. public String substring(int startIndex, int endIndex)
This method returns the substring of the string. It takes two integer parameters the first parameter is called startindex of the string and the second is endIndex of the string.
This method returns a substring starting from startIndex and extending to the character at endIndex. It will return the substring from startIndex to endIndex.
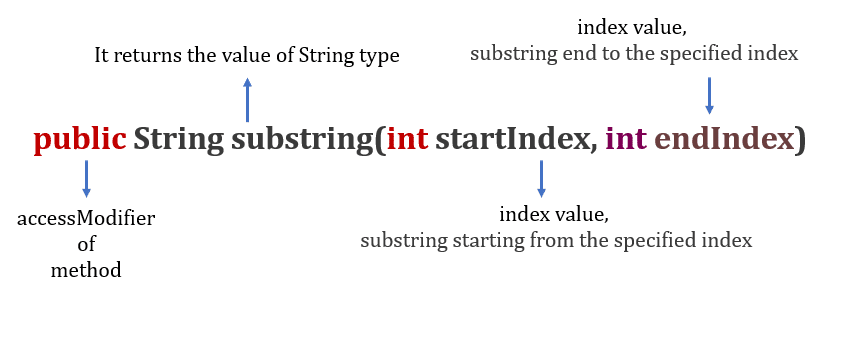
public: access modifier of the method is public so it can be accessed from everywhere.
returnType: It returns a String type value.
startIndex: It accepts only integer value, But we can’t provide a negative value if we will provide it will throw StringIndexOutOfBoundsException. The index value is inclusive in the substring.
endIndex: It also accepts only integer values, But we can’t provide a negative value or less than startIndex. The endIndex value is exclusive in the substring.
exception: It throws StringIndexOutOfBoundsException if the index is greater than the length or less than 0 or endIndex is less than startIndex.
String subStringName = stringName.subString(startIndex, endIndex)
class ExampleOfSubString { public static void main(String args[]) { String nameOfUniv = "KUK University"; String subStringOfUnivName = nameOfUniv.substring(1,10); System.out.println(subStringOfUnivName); } }
Output: UK Univer
public class SubStringExample { public static void main(String arg[]) { String str = "JavaGoal.com"; int length = str.length(); // Substring starts from index 0 and end to last character System.out.println("Substring index 0 to end : "+str.substring(0, length-1)); // Substring start from index 2 and end to 5 System.out.println("Substring start from index 2 to end : "+str.substring(2, 5)); // Substring start from index 4 and end to 7 System.out.println("Substring start from index 4 : "+str.substring(4, 7)); // Getting last character System.out.println("Substring : "+str.substring(length-2, length-1)); } }
Output: Substring start from index 0 to end : JavaGoal.co
Substring start from index 2 to end : vaG
Substring start from index 4 : Goa
Substring from last character : o
5. Important points about substring index java
1. The substring() method is overloaded in the String class. The first substring(int beginIndex) method accepts only the starting point of the substring and extends the substring to the end of the string.
Where the second substring(int beginIndex, int endIndex) method accepts both starting and endpoints of the substring.
public String substring(int beginIndex) public String substring(int beginIndex, int endIndex)
2. As we know string always stores as an array of characters. So, the first character is presented at index 0, and the last one is presented at length-1. If you want to get the last character of the string just use the substring(0, length-1) and it will return the last character of the string.
3. In the substring method, the beginIndex is inclusive but the endIndex is exclusive. So if you want to get a substring from the second character to the last character then you need to focus on the index value. The second character will present on index 1 and the last character present length-1(length of the string). So you can use substring(1, string.length()-1).
4. The substring() method will return an empty String if beginIndex= endIndex.
5. The substring() method will throw StringIndexOutOfBoundsException, If
beginIndex < 0 or beginIndex >= lengthOfString
endIndex <0 or endIndex < beginIndex or endIndex >= lengthOfString
We have discussed the java substring in recent topics. Java provides two methods to get the substring in java from the string. If you are not familiar with that method, please read them first.
In this post, we will see how to get a java substring in various ways. Actually, we will discuss some frequently asked questions and java substring example like palindrome in java or how to get a string palindrome program in java, find a substring in a string by char.
6. String palindrome program in java
A string is a palindrome if the reverse of string equals a string.
“AbccbA”, it a palindrome string because the reverse of a string is also the same.
“Java”, is not a palindrome string because the reverse of a string is not the same.
public class SubStringExample { public static void main(String[] args) { System.out.println(isPalindrome("abcba")); System.out.println(isPalindrome("Java")); System.out.println(isPalindrome("123321")); System.out.println(isPalindrome("CCCCC")); System.out.println(isPalindrome("AbcCDA")); } private static boolean isPalindrome(String str) { if (str == null) return false; if (str.length() <= 1) return true; int i = 0, j = str.length() - 1; while (i < j) { if (str.charAt(i) != str.charAt(j)) return false; i++; j--; } // Given string is a palindrome return true; } }
Output: true
false
true
true
false
7. Find a substring in a string by char
Suppose you want to find a substring that starts from a particular character. Then we need to use find the index of a particular character and we can use the indexOf() method.
public class SubStringExample { public static void main(String[] args) { String str1 = "JavaGoal"; String str2 = "Programming"; String str3 = "Website"; String str4 = "learning"; // Finding substring that start from char 'J' int indexOfChar = str1.indexOf('J'); System.out.println(str1.substring(indexOfChar)); // Finding substring that start from char 'g' indexOfChar = str2.indexOf('g'); int length = str2.length(); System.out.println(str2.substring(indexOfChar, length-1)); // Finding substring that start from char 'b' and ends with 't' System.out.println("Website"); int startIndex = str3.indexOf('b'); int endIndex = str3.indexOf('t'); System.out.println(str3.substring(startIndex, endIndex)); // Finding substring that start from char 'n' indexOfChar = str4.indexOf('n'); System.out.println(str4.substring(indexOfChar)); } }
Output: JavaGoal
grammin
Website
bsi
ning
Woww… Thats some pent up rage explosion i had yet to see