Java 9 modules are a major change in the structure of java, and it is the biggest feature of Java 9. You must think about why we are saying changes in the structure of java because in this feature java collects packages and code into a single unit that is known as java modules. In this post, we will see what is the module in java and what is the need for the Java 9 module. It is also known as Project jigsaw. In java, Project Jigsaw started in 2005 and it was planned for Java 7 but due to huge changes, it was delayed and released in Java SE 9. It took almost 10 years to finish the Java modules in a proper way.


What is Module in Java 9?
A module is a Set of related Java packages, types with code & data and resources. It is a mechanism to collect your Java application and Java packages into a module. Each module can have a number of packages and specify which package should be visible to other Java modules. A Java module also specifies which module is necessary to do its job. This is the most popular feature of Java 9 and is known as the Java Platform Module System (JPMS). Sometimes it is referred to as Java Jigsaw or Project Jigsaw. Each Module has a set of related codes and data to support the Single Responsibility Principle (SRP).
In Java 9, the Module System has 95 modules. Oracle separated JDK jar and Java SE specifications. All JDK modules start with “JDK.*” and all Java SE Specifications Modules start with “java.*”
There are two types of Package in the module
1. Exported Packages: These packages can be used from outside of the module, which means any program residing in any other module can use these packages.
2. Concealed Packages: These packages can be used only inside the module and they are internal to the module.
Before Java 9, all the packages of Java are not dived into modules and the size of the application increased in every release. After the release of Java 8, rt.jar file size is around 64MB. To resolve this problem, Java 9 restructured JDK into a set of modules.

What is the need for Java modules?
In the above section, we read about the module system. Here we will discuss “Why we need Java SE 9 Module System” and what is the problems of the Current Java System.
- JDK is too big and it increases on every release. So, it creates problems for small devices. Over time java packages increased due to different functionality. Suppose you are working on a project and using different packages then it is very difficult to understand which classes are using the different packages. So, there is a needs to organize the packages when we need to use several of them in our code.
- The rt.jar file was too big to use in small devices and applications. It creates performance issues.
- As we know public access modifier is too open because everyone can access it. Everyone can access critical API. This creates problems some and it is a weak Encapsulation in the current Java System.
- On every new release of Java, they have to test the whole JDK and JRE. Due to the big size, it is very hard to Test and Maintain applications.
How to Write Modular Code
We have learned a lot of things about java modules, now we will see how to write modular code in java or how to create a module in java. To create a module in java we have to use a file that is module-info.java. This file is also known as module descriptor and defines the following:
- Module name: A name of a Java module must be a unique name like package, class, variable etc.
- What does it export? : We can specify those packages, that we want to export in other modules. It means these packages will be visible in other modules.
- What does it require? : If a module requires another module to do its work, that other module must be specified in the module descriptor too.
Java 9 Module – Create and use modules in Eclipse IDE
Let’s create a module and use that module in another module. Here we will create a module in eclipse and use it in another module.
Step1: Create a Java project
Let’s create a Java project by using the name JavaGoal.calculator. Right-click on Project explorer and select a new Project > Java project > Enter name > Finish. Here we are using javaGoal.calculator as a project name.
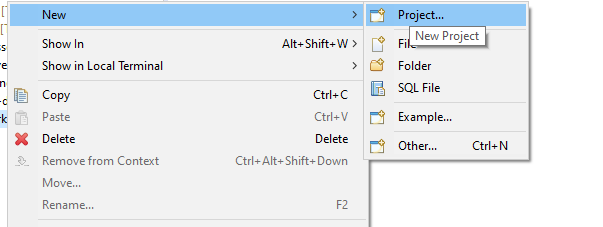
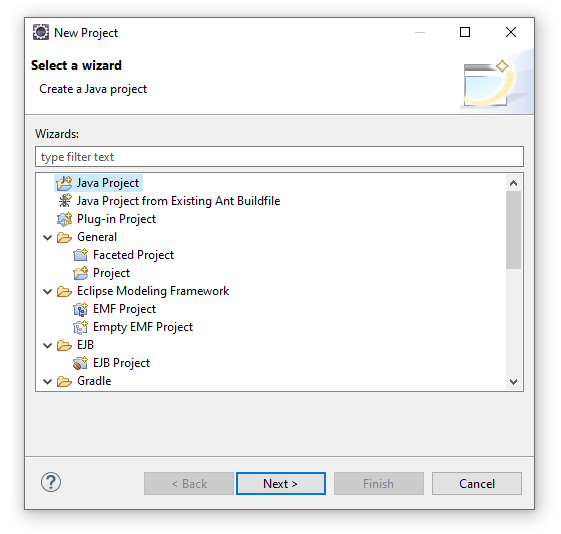
Step2: Create module-info.java file
After the creation of a java project, right-click on the project name and go to the Configure option and click create module-info.java option. Now type the module name for example javaGoal.calculator.

As of now, the file is empty but we will add data later.
3. Create a package and a class together
Here we will create a class and that class will be part of the module. Here we will create a class Calculator and we will use it in other modules. We will create a package at the same time. It means a module will hold the package and the package will hold the class.
Let’s create a package with javaGoal.calculator and also create a class Calculator.
package javaGoal.calculator; public class Calculator { public static void sum(int a, int b) { System.out.println(a+b); } public static void sub(int a, int b) { System.out.println(a-b); } public static void multiple(int a, int b) { System.out.println(a*b); } public static void divide(int a, int b) { System.out.println(a/b); } }
We have created a Calculator class in JavaGoal.calculator module. Here Calculator class acts as a utility class. Suppose we want to use the Calculator class in another module then we have to specify it in module-info.java. In the next step, we will see how we can specify it.
4. Export the package that we have created
As we are planning to use the Calculator class in another module, So this package must be used with an exported keyword in module-info.java. To do this, write this code in module-info.java file:

5. Let’s create another module
We have to repeat all the above steps except the 4th step. Firstly, we will create a java project with the name javaGoal.user. then we will create a module-info.java file. After that, we will create a module and package. Here we will create a class with the name User. Here we will use the class Calculator that exists in javaGoal.calculator module. The module-info.java file of this project has this code:
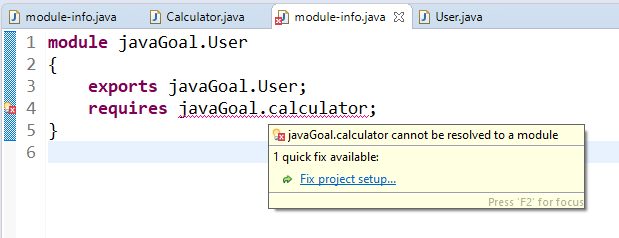
As you can see it is showing an error because the other module is not in the build path. To resolve this error, we have to build the path.
Right-click on the project > go to build path > configure build path. as shown in the following screenshot:
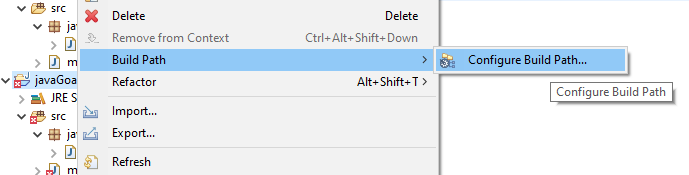
Now click on the Projects tab, click on Modulepath then click on Add button on the right side and add the javaGoal.calculator project and you will find an error has been resolved.
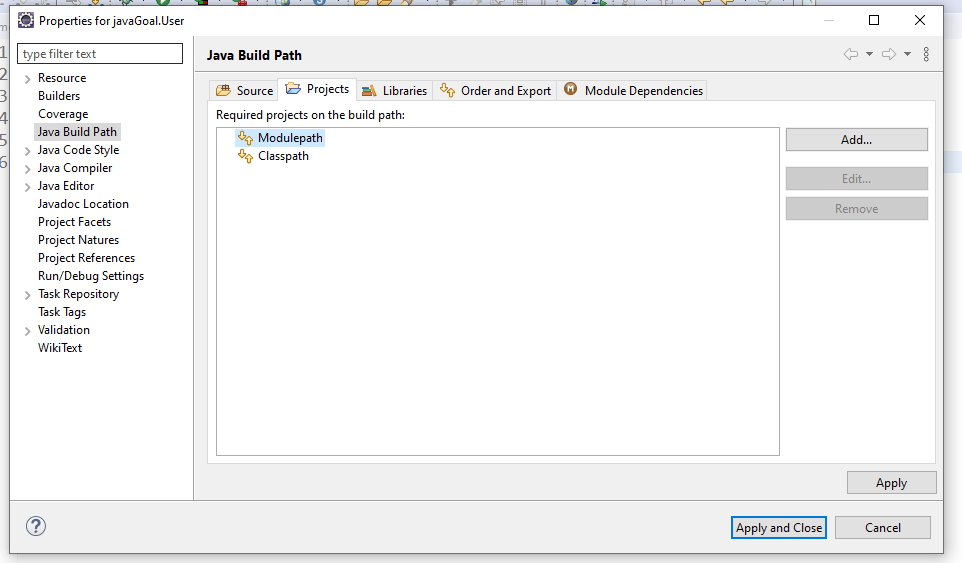
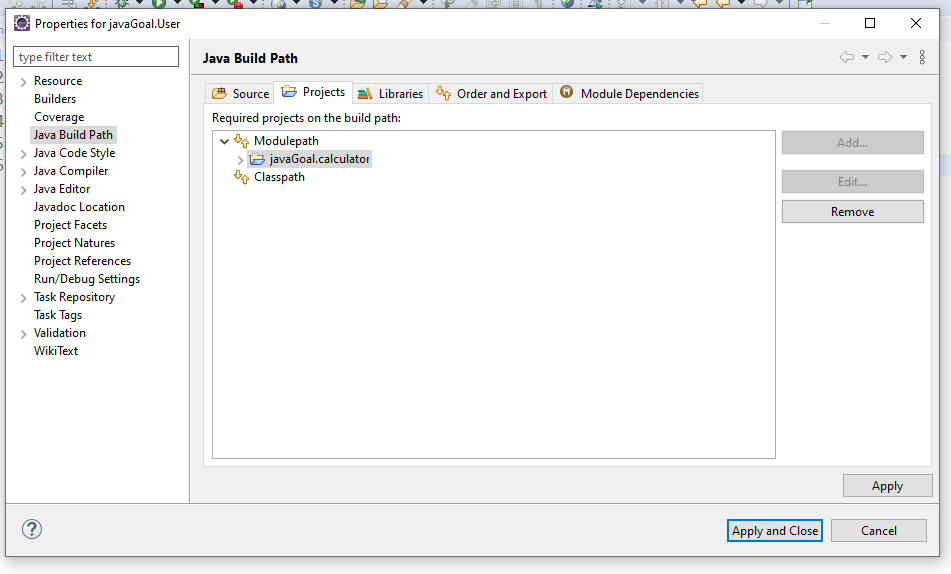
6. Let’s create a class in the second module
Let’s create a User class in JavaGoal.user module.
package javaGoal.User; import javaGoal.calculator.Calculator; public class User { public static void main(String[] args) { Calculator.sum(1, 2); Calculator.sub(5, 2); Calculator.divide(4, 2); Calculator.multiple(5, 5); } }
Output: 3
3
2
25
Here you can see, that we are using the Calculator class that exists in different modules.
7. Let’s see the structure of both modules.
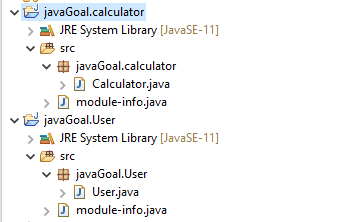
Excellent article. I am dealing with a few of these issues as well.. Ashli Ricky Rosenthal
Awesome write-up. I am a regular visitor of your web site and appreciate you taking the time to maintain the excellent site. I will be a frequent visitor for a really long time. Lina Nero Makell