The final variable is the most important concept in java. Most programmers may know about the final variable. Here we will discuss, What is final variable and final reference variable. How many ways to initialize it?
Here is the table content of the article we will cover this topic.
1. What is the final variable in Java?
2. How to declare a final variable?
3. Initialization of the final variable?
4. Re-assign value to a final variable?
5. Local Java final variable?
6. final with a reference variable?
7. final with an array variable?
8. What is the blank final variable in java?
9. RULES to initialize the blank final variable in the constructor?
10. Real-life example?
11. Why we use the blank final variable?
12. What is the static final variable in java?
13. How to declare a static final variable?
14. Initialization of static final variable?
15. What is the static blank final variable in java?
16. RULES to initialize the static blank final variable?
17. What is the final reference variable?
What is the final variable in Java?
Whenever we use the final keyword with a variable it means we are declaring a constant. In java, final variables are just like constants. If you want to make any constant variable, then use the final keyword with the variable.
1. If you are using a final keyword with primitive types of the variable (int, float, char, etc) then you can’t change the value of a final variable once it is initialized. So, we should initialize it.
2. If you are using a final keyword with non-primitive variables (By means non-primitive variables are always references to objects in Java), the member of the object can be changed. It means we can change the properties of the object, but we can’t change it to refer to any other object. We will discuss it separately in a final reference variable.
Firstly we will see how to use the final keyword with the primitive data type. You can’t change the value of the final variable after initialization. If you try to change the value of the final variable then the compiler will throw an exception at compile time.
How to declare a final variable
To declare a final variable we need to take care of two things, First one is the use of the final keyword and another is the initialization of the variable. It is not mandatory to initialize a final variable at the time of declaration. We can initialize it by constructor we will discuss it separately in detail. If you are not initializing it at the time of declaration, it’s called a blank final variable.
final dataType variableName = value;
Here, the final is a keyword that indicates the JVM to treat this variable as the final variable.
dataType is the type of variable which you want to create.
varaibleName is the name of the variable which you want to create.
final int a = 5;
class FinalVariable { public static void main(String args[]) { final int a = 10; a = 10+5; System.out.println(a); } }
Output: Exception in thread “main” java.lang.Error: Unresolved compilation problem:
The final local variable a cannot be assigned. It must be blank and not using a compound assignment at FinalVariable.main(FinalVariable.java:8)
Initialization of final variable
In Java, the JVM especially takes care of the initialization of the final variable. We must initialize the final variable with a value, otherwise, the compiler throws a compile-time error. But as we have seen in the above example a final variable can only be initialized once.
Java provides three ways to initialize a final variable :
i) Initialization while the declaration
ii) Initialization with the constructor
iii) Initialization with a static block
i. Initialization while the declaration
We can initialize a final variable at the time of the declaration. This approach is common for initialization. If final variables are not initialized at the time of declaration, then it is called a blank final variable. But always remember if the final variable is not initialized at the time of declaration then it must be initialized in another way, otherwise, JVM throws the exception at compile time. We have already seen it in the above example.
ii. Initialization with the constructor
By the use of a constructor, we can initialize the blank final variable. We can initialize a final variable by the constructor. Some programmers misunderstood this concept and think a constructor can initialize the final variable multiple times which is not true. Whenever a constructor invokes by JVM it will create a new object and initialize the value of the new object.
class BlankFinalWithConstructor { // It is a blank final variable final int a; BlankFinalWithConstructor() { // If we remove this line, we get compiler error. a = 2; } BlankFinalWithConstructor(int x) { // If we remove this line, we get compiler error. this.a = x; } } class MainClass { public static void main(String args[]) { BlankFinalWithConstructor object = new BlankFinalWithConstructor(); System.out.println("Value of final variable a = "+ object.a); BlankFinalWithConstructor object1 = new BlankFinalWithConstructor(5); System.out.println("Value of final variable a = "+ object1.a); BlankFinalWithConstructor object2 = new BlankFinalWithConstructor(10); System.out.println("Value of final variable a = "+ object2.a); } }
Output:
Value of final variable a = 2
Value of final variable a = 5
Value of final variable a = 10
iii. Initialization with a static block
You can initialize a final variable in a static block, but it should be static also. But static final variables can’t be assigned value in the constructor. So, they must be assigned a value with their declaration.
class BlankFinalWithConstructor { // It is a blank final variable static final int a; static int b; static { // assigning value to final variable a = 5; b = 8; } } class MainClass { public static void main(String args[]) { System.out.println("Value of final variable a = "+ BlankFinalWithConstructor.a); System.out.println("Value of final variable b = "+ BlankFinalWithConstructor.b); } }
Java final variable: A normal variable can re-assign the value, but the final variables can’t change the value once assigned it. It will remain constant throughout the execution of the program. Hence final variables must be used only for the values that we want to remain constant.
Re-assign value to a final variable
A final variable cannot be reassigned, doing it will throw a compile-time error.
class MainClass { // It is a blank final variable final int a = 5; public void setValue() { a = 10; } public static void main(String args[]) { MainClass obj = new MainClass(); obj.setValue(); System.out.println("Value of a = "+ obj.a); } }
Output: Exception in thread “main” java.lang.Error: Unresolved compilation problem: The final field MainClass.a cannot be assigned at MainClass.setValue(MainClass.java:9) at MainClass.main(MainClass.java:14)
Local Java final variable
You can create a final variable inside a method/constructor/block. It must initialize once where it is created.
class MainClass { public static void main(String args[]) { // It is a local final variable final int a = 5; System.out.println("Value of a = "+ a); } }
Output: Value of a = 5
final with a reference variable
If a reference variable of an object is final, then this final variable is called the reference final variable. As you know that a final variable cannot be re-assign. In referenced final variable you can changes/reassign the value of its variables. But you can re-assign the reference final variable.
class FinalWithReference { int a = 5; } class MainClass { public static void main(String args[]) { final FinalWithReference obj = new FinalWithReference(); obj.a = 10; System.out.println("Value of a = "+ obj.a); // You can't re-assign it. it will throw compilation error //obj = new FinalWithReference(); } }
Output: Value of a = 10
final with array variable
We can create an array variable as the final. We can’t re-assign the array variable reference. But we can change the elements of the array.
Example:
class MainClass { public static void main(String args[]) { final int arr[] = {1, 2, 3}; arr[0] = 10; arr[1] = 20; arr[2] = 30; for(int i = 0; i < 3 ; i++ ) { System.out.println(arr[i]); } // You can't re-assign it. it will throw compilation error // arr = new int[5]; } }
Output:
10
20
30
What is the Blank final variable in java?
As you already know, a final variable can be initialized only one time. You can’t initialize a final variable more than one time. Java provides three ways to initialize it. But some time programmer doesn’t want to initialize a final variable at declaration time. So the Blank final variable is a final variable that is not initialized during declaration. It initializes by the constructor.
A Blank Final variable can be initialized only in the constructor. Java doesn’t provide any other way to initialize a blank final variable.
RULES to initialize the Blank final variable in the constructor
1. If you are not initializing a final variable during the declaration (Blank final variable), then it must be initialized in the constructor otherwise compiler throws an exception at compile time.
2. If you have more than one constructor, then every constructor must be initialized with the Blank final variable.
3. A static final blank variable can’t be initialized in the constructor. It must be initialized in a static block.
Real-life example
Suppose we want to maintain the data of persons. Each person has a name, address, PAN, etc. But a PAN can register for the only single person we don’t want to change during the execution of the program. So, we will create a Blank final variable so that value could be provided during the Construction of the Object. In this example, we will create a final variable PAN so that it will remain the same during the program execution.
public class Person { String name; String address; final int PAN; public String getName() { return name; } public void setName(String name) { this.name = name; } public String getAddress() { return address; } public void setAddress(String address) { this.address = address; } Person(int pan) { this.PAN = pan; } void showData(Person person) { System.out.println("Name of Person : " + person.getName()); System.out.println("Address of person : " + person.getAddress()); System.out.println("PAN of person : "+ person.PAN); } public static void main(String arg[]) { Person person1 = new Person(123456); person1.setName("Ram"); person1.setAddress("India"); person1.showData(person1); Person person2 = new Person(654321); person2.setName("John"); person2.setAddress("USA"); person2.showData(person2); } }
Output: Name of Person: Ram
Address of person: India
PAN of person: 123456
Name of Person: John
Address of person: USA
PAN of person: 654321
In the above example, we are creating a Blank final variable in the Person class. So whenever we create an object of a person, we can provide different values to the Final variable for each person. We are declaring final int PAN. For best practices, we should use the UPPERCASE letters for the final variables.
Why do we use the Blank final variable?
Sometimes we don’t know the value of the final variable during the creation of the class. The value could be decided during the construction time of the object.
If we want to create a constant value that will be common for all the objects, then we should initialize the final variable during the declaration.
But there may be a situation when we want to create a constant value that will not be common for all objects. We want to make it constant only within the object. When a constructor will initialize the Blank final variable? Then it will create a new copy for each object.
Suppose you are providing login functionality on your website. A user can set the username only when he/she is creating the account on the website. After that, it should not be changed. Here you can create a blank final variable of username.
Each user will provide the username during the creation of the account.
public class Login { final String USERNAME; String address; String name; public String getName() { return name; } public void setName(String name) { this.name = name; } public String getAddress() { return address; } public void setAddress(String address) { this.address = address; } Login(String userName) { this.USERNAME = userName; } void showData(Login login) { System.out.println("Name of Person : " + login.getName()); System.out.println("Address of person : " + login.getAddress()); System.out.println("PAN of person : "+ login.USERNAME); } public static void main(String arg[]) { Login user1 = new Login("abc123"); user1.setName("Ram"); user1.setAddress("India"); user1.showData(user1); Login user2 = new Login("123abc"); user2.setName("John"); user2.setAddress("USA"); user2.showData(user2); } }
Output: Name of Person: Ram
Address of person: India
PAN of person: abc123
Name of Person: John
Address of person: USA
PAN of person: 123abc
What is the static final variable in java?
Most of us already know a static variable is declared on the class level. It means a static variable is shared with all the objects of the class. If we make any change in a static variable that reflects the other objects also. A static variable is always an instance variable of the class. You can’t declare a static variable within the method, block, or constructor. So, if we won’t initialize a static variable, then JVM will initialize it and provides the default value.
But if we are declaring a static variable with the final keyword then JVM does not provide the default value. We must need to initialize a static final variable because JVM will not provide a default value to it. A static final variable is a compile-time constant because it loads in memory when a class loads to memory.
How to declare a static final variable?
To declare a static final variable, we need to take care of two things, Use the final keyword and initialize the variable. It is not mandatory to initialize a static final variable at the time of declaration. We can initialize it in a static block also. If you are not initializing it at the time of declaration, it’s called a blank static final variable.
static final dataType variableName = value;
Here, static is a keyword that indicates the JVM, the variable will be shared for all objects.
final is a keyword that indicates the JVM to treat this variable as the final variable.
dataType is a type of variable which you want to create.
varaibleName is the name of the variable which you want to create.
class StaticFinalVariable { static final int a = 5; public void sum() { a = a + 10; } } public class MainClass { public static void main(String args[]) { StaticFinalVariable object = new StaticFinalVariable(); System.out.println("Value of static final variable = "+ StaticFinalVariable.a); } }
Output: Exception at compile time
Initialization of static final variable
In Java, the JVM especially takes care of the initialization of the static final variable. We must initialize the static final variable with a value, otherwise, the compiler throws a compile-time error. But as we have seen in the above example a static final variable can only be initialized once.
i) initialization during the declaration
We can initialize a static final variable during the declaration of the variable. As you know static variables are read before the class loading.
class StaticFinalVariable { static final int a = 5; } public class MainClass { public static void main(String args[]) { StaticFinalVariable object = new StaticFinalVariable(); System.out.println("Value of static final variable = "+ StaticFinalVariable.a); } }
Output: Value of static final variable = 5
ii) initialization inside a static block
You can initialize a final variable in a static block, but it should be static also. But static final variables can’t be assigned value in the constructor. So, they must be assigned a value with their declaration.
class StaticFinalVariable { static final int a; static { a = 5; } } public class MainClass { public static void main(String args[]) { StaticFinalVariable object = new StaticFinalVariable(); System.out.println("Value of static final variable = "+ StaticFinalVariable.a); } }
Output: Value of static final variable = 5
What is the static blank final variable in java?
As you already know a static final variable can be initialized only one time. You can’t initialize a static final variable more than one time. The blank static final variable is a final variable that is not initialized during declaration. It can be initialized in static block only.
A blank final variable can be initialized only in the static block. Java doesn’t provide any other way to initialize a blank static final variable.
RULES to initialize the static blank final variable
1. If you are not initializing a static final variable during the declaration(blank static final variable) then it must be initialized in a static block otherwise compiler throws an exception at compile time.
2. A static final blank variable can’t be initialized in the constructor. It must be initialized in a static block.
Real-life example
Suppose we want to maintain data of persons. Each person has a name, address, country name, etc. But we want to provide a common(static) and constant(final) country name to all persons. Here we are creating a static final variable and we will be initialized it in a static block. In this example, we will create a static final variable COUNTRYNAME so that it will remain the same during the execution of the program.
public class Person { String name; String address; static final String COUNTRYNAME; static { COUNTRYNAME = "India"; } public String getName() { return name; } public void setName(String name) { this.name = name; } public String getAddress() { return address; } public void setAddress(String address) { this.address = address; } void showData(Person person) { System.out.println("Name of Person : " + person.getName()); System.out.println("Address of person : " + person.getAddress()); System.out.println("Country of person : "+ person.COUNTRYNAME); } public static void main(String arg[]) { Person person1 = new Person(); person1.setName("Ram"); person1.setAddress("India"); person1.showData(person1); Person person2 = new Person(); person2.setName("John"); person2.setAddress("USA"); person2.showData(person2); } }
Output: Name of Person : Ram
Address of person : India
Country of person : India
Name of Person : John
Address of person : USA
Country of person : India
final reference variable
A final variable can’t change the value during the execution of the program. We have seen how the final variable works with primitive data types. In this article, we will see how the reference variable works with the final keyword.
A reference variable stores the reference of an object. For example
ArrayList<String> list = new ArrayList<String>();
Here the list is the reference variable for the object of ArrayList. A reference variable is only way to access an object.
What is the final reference variable?
When we use the final keyword with a reference variable it means we can’t reassign it to another reference or object. But you can change the value of members by using the setter methods. When we create a reference variable with the final keyword it means we can’t use the “=” operator to reassign it. If you try to reassign the value it will throw a compilation error.
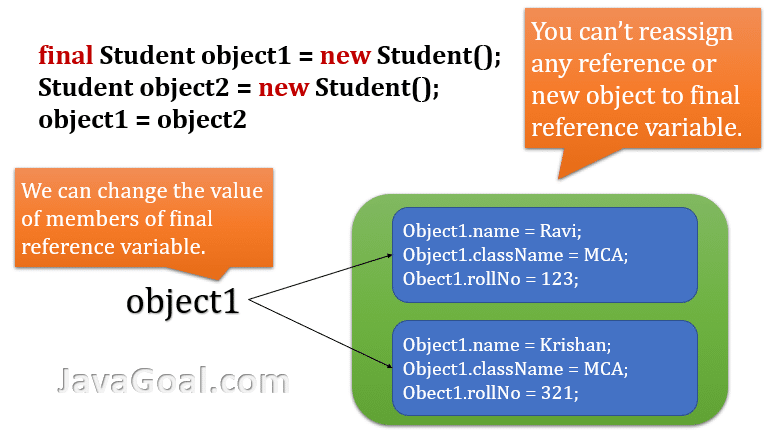
Let us discuss it with an example. We will create a class Student having some member variable.
public class Student { int rollNo; String className; String name; public int getRollNo() { return rollNo; } public void setRollNo(int rollNo) { this.rollNo = rollNo; } public String getClassName() { return className; } public void setClassName(String className) { this.className = className; } public String getName() { return name; } public void setName(String name) { this.name = name; } public static void main(String args[]) { final Student object = new Student(); object.setName("Ram"); object.setRollNo(123); object.setClassName("MCA"); Student object1 = new Student(); object1.setName("Krishan"); object1.setRollNo(456); object1.setClassName("MCA"); // object is a reference variable with final keyword so we can't assign it object = object1; } }
Output: Exception in thread “main” java.lang.Error: Unresolved compilation problem: The final local variable object cannot be assigned. It must be blank and not using a compound assignment at ok.Student.main(Student.java:38)
In the above example, we are creating a reference with the final keyword of the Student class. In the next line, we are trying to reassign it with another reference which is not possible. Because Java doesn’t permit reassigning the reference to the reference with the final keyword. But we can change the value of members by use of the setter method.
public class Student { int rollNo; String className; String name; public int getRollNo() { return rollNo; } public void setRollNo(int rollNo) { this.rollNo = rollNo; } public String getClassName() { return className; } public void setClassName(String className) { this.className = className; } public String getName() { return name; } public void setName(String name) { this.name = name; } public static void main(String args[]) { final Student object = new Student(); object.setName("Ram"); object.setRollNo(123); object.setClassName("MCA"); // object is a reference variable with final keyword // so we can change only its members value object.setName("Krishan"); object.setRollNo(222); object.setClassName("MCA"); System.out.println("Name :" +object.getName()); System.out.println("Class name :" +object.getClassName()); System.out.println("RollNo :" +object.getRollNo()); } }
Output: Name :Krishan
Class name :MCA
RollNo :222
In the above example, we have created a reference variable with the final keyword. Although we know we can’t reassign it with any reference of the object but we can change the value of variables of a final reference. We can the properties of an object but can change the reference value.
I think this is one of the most vital info for me. And i’m
glad reading your article. But wanna remark on few general things, The web site style is ideal, the articles is really nice : D.
Good job, cheers
Appreciate the recommendation. Will try it out.