In java ArrayList and array, both are famous data structure. In this post, we will discuss the difference between ArrayList and array. Before moving further, you should read the Array in java and ArrayList in java.
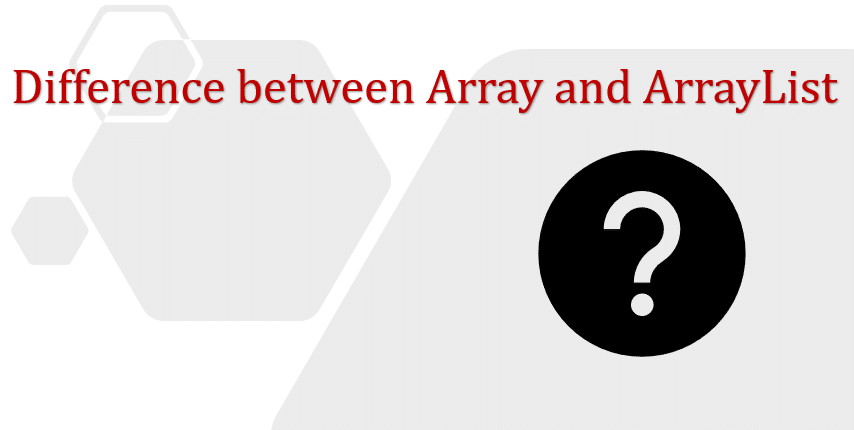
1. Size
The most popular difference between ArrayList and Array is size.
An Array has fixed length and we can’t change the length after the creation of Array object. If we try to add more elements than the size of the Array. It will throw ArrayIndexOutOfBoundException.
An ArrayList has the dynamic size, it’s a growable array. When we create an ArrayList it has default size i.e. 10. It automatically grows or shrinks when we add or remove elements.
2. Generic
An Array can’t be generic, it can hold a different type of element that checks on run time. If you add any incompatible element into an array, the compiler throws ArrayStoreException at run time. But we should try to get errors at compile time.
ArrayList supports the generic concept, unlike Array. By use of the generic concept, JVM ensures the type safety at compile time. So ArrayList restrict the different type of data in generic ArrayList.
3. Initialization
An Array should be initialized by some value. It is mandatory to provide the size of the Array.
An ArrayList is automatically initialized by default size 10. So, it is not mandatory to initlize it.
4. Primitive and non-primitive
An Array can hold primitive(int, float, double) and non-primitive(String or User-defined class) data type.
But some developers misunderstood it and think ArrayList can take primitive data type also. Let’s take an example.
ArrayList<Integer> list = new ArrayList<Integer>(); list.add(1);
Here ArrayList is can take only Integer type of data. But we are adding element of int type. Here JVM converts the int to Integer type.
list.add(new Integer(1));
5. Iterate
We can Iterate the Array by use of for loop, forEach loop.
To iterate an ArrayList we can use for loop, forEach loop, Iterator, List Iterator.
6. Add element
In Array we add the element by use assignment operator (=), But in ArrayList, we add the element by use of add() method.
7. Multidimensional
We can make an Array as multi-dimensional but an ArrayList is always single dimensional.
8. Method
To get the size of Array we use the length() method but in ArrayList we use the size() method to find the size of ArrayList.