The Static method in Java is an important concept. But many programmers misunderstood and misused it. In this post, we will discuss the static method in java with examples so that you can get it easily. Almost all programmer knows that static methods belong to the class and non-static methods belong to the objects of the class. but only a few of them understand what it means and when to use the static method and non-static method.
Here is the table content of the article we will cover this topic.
1. What is the static method in Java?
2. How to create a static method in java?
3. How to use a static method in java?
4. Scenario 1: When static variables and static methods are in the same class?
5. Scenario 2: When the static variable and static methods are in different classes?
6. Properties of Static Methods in Java?
7. When to use static methods in java?
What is the static method?
A method declared with a static keyword is known as a static method. The static method belongs to a class instead of an instance/object. A static method is part of a class definition instead of part of the object.
What is the mean of the static method as part of the class definition?
We can call a static method without the creation of an object. It means we don’t need to create an object to invoke any static method. It means it’s not dependent upon the object state. On the other hand, the non-static is invoked only through the objects. We can call them only when they are part of an object. That’s why the static method is part of the class definition.
How to create a static method?
To create a static method, we must use the static keyword with the method. Whenever we use the static keyword during the method declaration, the JVM binds that method with the class definition.
AccessModifier static returnType methodName(parameters) { //Body of static method }

Here accessModifier specifies the scope of the method. You can read about access modifiers.
static is a keyword that is used to make it a static method.
returnType is the type of data that you want to return from the method.
methodName is the name of the method.
parameters, You can define any number of parameters.
How to use a static method?
We have read, how can we create a static method. Now we will see how we can use it. As you know static methods bind with the class instead of the object. To use a static method, we don’t need to create an object we can access it by the class name in which it is created.
className.methodName()
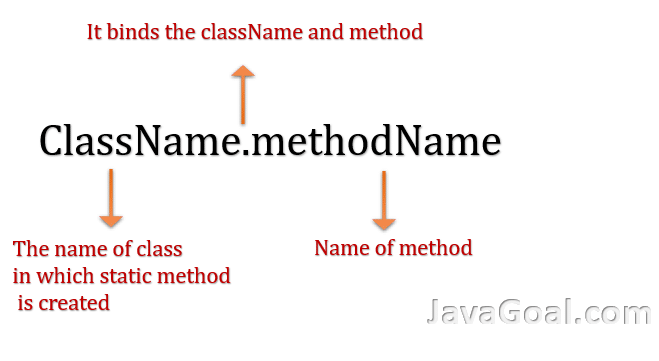
Let’s see with examples, how we can create and use it. We will discuss it in a different scenario that will clear some doubts.
Scenario 1: When static variables and static methods are in the same class?
Suppose we are working in a single class, here we have static variables and static methods. So, let us see how it will work in a single class.
A static method can access any static variable or static method without any object creation. As we have read already in the above section, to access a static variable or static method, we can use class names directly. But if the static variable and static method exist in the same class then we do not need to use the class name, Because of its presence in the same class. But to access a non-static variable or method we need to use the object.
class StaticMethodExample { int a = 0; static int b = 0; void showData() { System.out.println("Value of a : "+ a); System.out.println("Value of b : "+ b); } static void displayData() { StaticMethodExample obj = new StaticMethodExample(); System.out.println("Value of a : "+ obj.a); System.out.println("Value of b : "+ b); } public static void main(String arg[]) { displayData(); StaticMethodExample obj = new StaticMethodExample(); obj.showData(); } }
Output: Value of a : 0
Value of b : 0
Value of a : 0
Value of b : 0
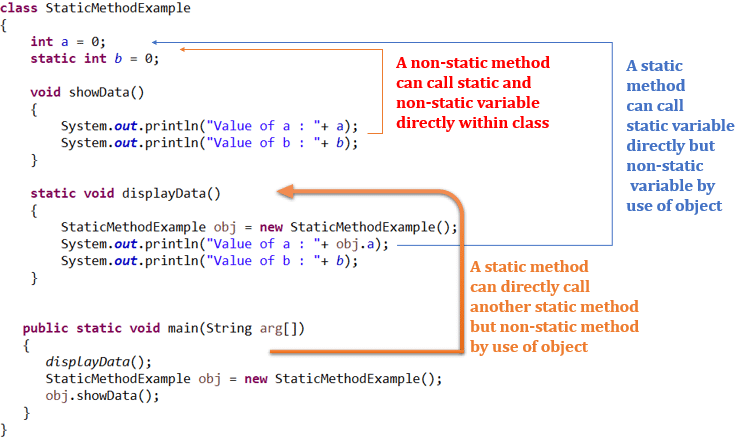
Scenario 2: When the static variable and static methods are in different classes?
Suppose we are working with multiple classes. We have static variables and static methods in different classes. So, let us see how we can access them.
A static method can access any static variable or static method without any object creation. We can access them by use of the class names. But to access a non-static variable or method we need to use the object.
class StaticExample { int a = 0; static int b = 0; static String displayData() { return "Static method"; } } public class MainClass { public static void main(String arg[]) { System.out.println("Value of b :"+ StaticExample.b); System.out.println(StaticExample.displayData()); } }
Output: Value of b :0
Static method
Properties of Static Methods in Java
1. Restriction on this keyword
We can’t use this keyword within the static method because this keyword is a reference variable that is used to refer to the current object. We can’t use it in static methods because static methods belong to a class instead of an object If you try to use this keyword inside a static method then the compiler will show a compile-time error in Java.
class StaticExample { int a = 0; static int b = 0; static void initlize(int a, int b) { this.a = a; this.b = b; } void showData() { System.out.println("Values : "+ a + ", "+ b); } public static void main(String arg[]) { initlize(5, 6); StaticExample obj = new StaticExample(); obj.showData(); System.out.println(); } }
Output: Exception in thread “main” java.lang.Error: Unresolved compilation problems: Cannot use this in a static context Cannot use this in a static context at StaticExample.initlize(StaticExample.java:9) at StaticExample.main(StaticExample.java:20)
2. Restriction on super keyword
We can’t use the super keyword within the static method because the super keyword is a reference variable that is used to refer to the current object. We can’t use it in static methods because static methods belong to a class instead of an object If you try to use the super keyword inside a static method then the compiler will show a compile-time error in Java.
class ParentClass { public void ParentData() { System.out.println("Method of Parent class"); } } class ChildClass extends ParentClass { public static void showData() { super.ParentData(); System.out.println("Method of Child class"); } public static void main(String arg[]) { showData(); } }
Output: Exception in thread “main” java.lang.Error: Unresolved compilation problem: Cannot use super in a static context at ChildClass.showData(ChildClass.java:14) at ChildClass.main(ChildClass.java:20)
3. Can’t directly use the non-static variables in the static method
We can’t directly use any non-static variable inside a static method. Because the non-static variable exists only when an object is created, and they don’t exist when the static method gets called. The static methods are not associated with any instance. In short, We use an object to access the non-static members inside your static methods in Java.
4. static method can’t be overridden
In Java, we can declare a static method in the child class with the same signatures as in the Parent class. But it will be considered the method overriding. The static method of the child class will hide the implementation of the method of the Parent class. It is known as the method hiding. Because the compiler decides which method to execute at the run-time, not at the compile time. As you know the static method can be accessed by class name rather than the object. When the compiler invokes the static method then it accesses the static method of the child class. You can read “Can we override the static method in java?”
5. static method can be overloaded
Yes, we can overload the static method. A class can have more than one static method with the same name, but different in input parameters. You can overload the static method by changing the number of arguments or by changing the type of arguments. There are many ways to overload a method in Java. You can read “Can we overload static method in java?“
6. static methods are faster than non-static
Yes, the invocation of static methods is more efficient than non-static methods. When the compiler invokes a non-static method, it needs to pass the current reference, but a static method can invoke without any reference.
7. Static methods as Utility methods
The static method is useful to create Utility methods. Because utility methods are used to perform common and re-used tasks. So, we should make them static so that they can easily access by class name.
8. static method uses the static binding
In java static method bonds during the compile time. That is the main reason Java doesn’t support the overriding of the static method. Because the compiler decides which method to execute at the compile time, not at the runtime. As you know the static method can be accessed by class name rather than the object. When the compiler invokes the static method then it accesses the static method of the child class. You can read “Static polymorphism or compile-time polymorphism?“
9. static method can access by class name
It is the most powerful feature of the static method. We can call a static method by use of the class name and we don’t need to create an instance of that class. The best example is the main method of the class. You know the main method is a static method so when JVM calls the main method, it doesn’t need to create an object of the class to call the main method.
10. static method supported in the interface
Since java 8, we can declare static methods in the interface. You can read “static method in interface“
11. Invokes another static method and non-static method
The static methods can invoke another static method by the use of a class name. But to invoke the non-static you have to create an object then you can invoke them.
When to use static methods in java
We have read what is a static method in java. You must see there “how to create and use it in different scenarios”. A static method has some unique properties. In this post, we will see “when to use static methods in java? or what is the need for static method? ”. To move further and for a deep dive you must know about the “Important properties of the static method”.
Let’s find out the answer to the question “when to use static methods in java ?“. Making a method as the static method is an important part of your program or project. You need to take care of the properties of the static method also. Because a static method has some unique properties, if you want to use them you need to think about every perspective. Suppose you want to create an object to call a method, you want to restrict the overriding concept of the method. Here we have the number of scenarios we will discuss them one by one. That will help you to decide when to use static methods in java.
Independent Code
The code which is written is a static method that is not dependent on object creation. As you know static methods do not bind with the object, they are directly binding with the class. So, the code is considered independent of object creation.
Suppose you have a record of School and you want to maintain all the Student’s data. Each student has some data like class name, roll no, section, etc. that information exists based on per student in school. But the data of the school like school name, location, etc. always exists and is not dependent on students.
If we talk about it in java, those method provides student information are only exists when an object for a will be created. But the information about the school is not dependent on object creation because it exists already.
class Student { String name; String className; int rollNo; public String getName() { return name; } public void setName(String name) { this.name = name; } public String getClassName() { return className; } public void setClassName(String className) { this.className = className; } public int getRollNo() { return rollNo; } public void setRollNo(int rollNo) { this.rollNo = rollNo; } public Student(String name, String className, int rollNo) { this.name = name; this.className = className; this.rollNo = rollNo; } } class Record { public void StudentInfo(Student student) { System.out.println("Name : "+ student.getName()); System.out.println("Class Name : "+ student.getClassName()); System.out.println("RollNo : "+ student.getRollNo()); } static void SchoolInfo() { System.out.println("School Name : ABCD"); System.out.println("School Location : XYZ"); } } public class MainClass { public static void main(String args[]) { Record record = new Record(); // To get information of student must create an object record.StudentInfo(new Student("Ram", "MCA", 123)); // To get information of school non need to create an object Record.SchoolInfo(); } }
Output: Name : Ram
Class Name : MCA
RollNo : 123
School Name : ABCD
School Location: XYZ
Invoke by Class Name
Many people have a doubt about why we use the static method even though we have a class with getters, setter and we can create any number of non-static methods. Why we should create a static method though we can invoke any non-static method by the object of the class?
The answer is simple, sometimes we want to use the functionality of the method without the creation of objects. So, the method must be static.
Let’s take a real-time example. Each application has a class that contains the main method.
public static void main(String args[]) { // Body of method }
You have noticed the main method is always a static method. Actually, the main method is the entry point of the execution of the class. Whenever we run any application, the JVM finds the main method and invokes it by use of the class name. Suppose the main method will not be static then how JVM will create an object of class? Where it can store the created object? It creates a headache.
To write utility methods
If you want to create a utility method, then it would be great if you make them a static method. Utility methods are those methods that perform common and used functions. You must think about why we should declare them as static.
The utility method provides common functionality that you can use anywhere. If we make it a non-static method, then you must create an object to use it. Even if you don’t want to construct the object of the class.
In our JDK there is a number of classes that have a utility method. Here we have a class Math that has a number of static methods, the multiplyExact(int x, int y) is one of them.
To use the functionality of this method you just need to call it by class name because it is static.
Prevent the overriding of the method
A static method can’t be overridden in child classes. So the time we don’t want to modify the implementation in child classes. In Java, we can declare a static method in the child class with the same signatures as in the Parent class. But it will not be considered the method overriding. The static method of the child class will hide the implementation of the method of the Parent class. It is known as method hiding. Because the compiler decides which method to execute at the compile time, not at the runtime. As you know the static method can be accessed by class name rather than the object. When the compiler invokes the static method then it accesses the static method of the child class. You can read the article in detail “Can we override the static method in java”.
By keeping all the scenarios in mind, You can decide when to make a method static in Java and when to use them.