As we know ArrayList in java works based on the index and each value stores in a unique index. There may be a situation when we want to find the index of an object. Then we can use the indexOf(Object o) and lastIndexOf(Object o). Lets see how to use java arraylist indexof() method.
Here is the table content of the article will we will cover this topic.
1. index(object o) method
2. lastIndexOf(Object o) method
index(object o) method
The index(Object o) method is used to get the index of the specified element. Suppose if you have duplicate elements in ArrayList then it returns the index value of first occurrence of the element. It returns the int value and if the element doesn’t exist in the ArrayList it returns -1.
Sntytax of java arraylist indexof() method
Where, Object represents the type of elements in ArrayList .
o, is the element which you want to find in ArrayList .
import java.util.ArrayList; import java.util.Iterator; public class ArrayListExample { public static void main(String[] args) { ArrayList<Integer> listOfNumbers = new ArrayList<Integer>(); listOfNumbers.add(111); listOfNumbers.add(222); listOfNumbers.add(333); listOfNumbers.add(444); listOfNumbers.add(555); System.out.println("Index value of 111: "+ listOfNumbers.indexOf(111)); System.out.println("Index value of 555: "+ listOfNumbers.indexOf(555)); System.out.println("Index value of 123: "+ listOfNumbers.indexOf(123)); } }
Output:Index value of 111: 0
Index value of 555: 4
Index value of 123: -1
The index value of ArrayList start from 0 and ends to length-1. Here we have 5 elements in ArrayList from index 0 to 4. So when we are getting first element 111 then it returning index value 0 and for 555 it returning 4.
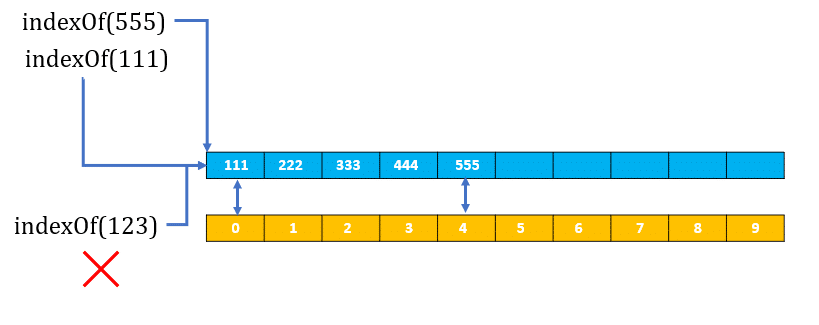
lastIndexOf(Object o) method
The lastIndexOf(Object o) method is also used to find the index of element but it returns the last index of the specified element. It returns the index value of the last occurrence of the element. It returns the int value. If the element doesn’t exist in ArrayList it returns -1.
int lastIndexOf(Object o)
Where, Object represents the type of elements in ArrayList.
o, is the element which you want to find in ArrayList.
package package1; import java.util.ArrayList; import java.util.Iterator; public class ArrayListExample { public static void main(String[] args) { ArrayList<Integer> listOfNumbers = new ArrayList<Integer>(); listOfNumbers.add(111); listOfNumbers.add(222); listOfNumbers.add(333); listOfNumbers.add(444); listOfNumbers.add(555); listOfNumbers.add(111); listOfNumbers.add(444); listOfNumbers.add(111); System.out.println("Last Index value of 111: "+ listOfNumbers.lastIndexOf(111)); System.out.println("Last Index value of 444: "+ listOfNumbers.lastIndexOf(444)); System.out.println("Last Index value of 123: "+ listOfNumbers.lastIndexOf(123)); } }
Output: Last Index value of 111: 7
Last Index value of 444: 6
Last Index value of 123: -1
As you can see the element 111 is presented at three positions 0, 5, 7. But this method returns only last position of the element.
