In this article, we will discuss how to use the Optional class in java 8? What are the benefits of optional class? We will also discuss the methods of Optional class.
Here is the table content of the article will we will cover this topic.
1. What is the Optional class in Java 8?
2. How to use the Optional class?
3. Methods in the Optional class?
What is the Optional class in Java 8?
In Java 8, a new class was introduced which is an Optional class. The optional class is the final class that is present in java.util package. This class helps the programmer to write better code and avoid the use of null checks.
Let’s understand it in brief:
Every programmer knows about the NullPointerException in Java. Many of us must have encountered it. The NullPointerException exception occurs only when the programmer tries to use an object reference that doesn’t point to any instance. There is the various reason if the object doesn’t point to any instance, maybe it is not initialized, or it initialized with null. It is not an easy job to avoid null without using too many null checks.
The solution is given by Java 8:
To resolve this problem Java 8, introduce a new class Optional class. The Optional class provides various methods to check the value of the object. The programmer can specify the alternate value to return. It makes the code in readable form and the programmer doesn’t need to specify the null check.
First of all, we will see without the use of the Optional class.
public class WithoutOptional { public static void main(String[] args) { Integer[] intArray = new Integer[5]; for(int i = 0; i < intArray.length; i++) { String word = intArray[i].toString(); System.out.print(word); } } }
Output: Exception in thread “main” java.lang.NullPointerException at First.WithoutOptional.main(WithoutOptional.java:10)
Now, we will see how you can use the Optional class:
import java.util.Optional; public class WithoutOptional { public static void main(String[] args) { Integer[] intArray = new Integer[5]; for(int i = 0; i < intArray.length; i++) { Optional<Integer> checkNull = Optional.ofNullable(intArray[i]); if(checkNull.isPresent()) { String word = intArray[i].toString(); System.out.print(word); } else System.out.println("String is NULL"); } } }
Output: String is NULL
String is NULL
String is NULL
String is NULL
String is NULL
How to use the Optional class?
public final class Optional<T> { /** * Common instance for {@code empty()} }
Optional is a generic class in java, so you must specify the type of data that can it hold. The Optional class provides a way, to replace the null reference of T Type with a non-null value. You can directly check whether the reference is present or absent. Because the Optional class either contains any non-null value or nothing.
import java.util.Optional; public class WithOptional { public static void main(String[] args) { String s = ""; String s1 = "Hello"; Optional<String> objOfOptional = Optional.of(s); // Return true if it contain non-null value else false System.out.println(objOfOptional.isPresent()); Optional<String> objOfOptional1 = Optional.of(s1); // Return true if it contain non-null value else false System.out.println(objOfOptional1.isPresent()); } }
Output: true
true
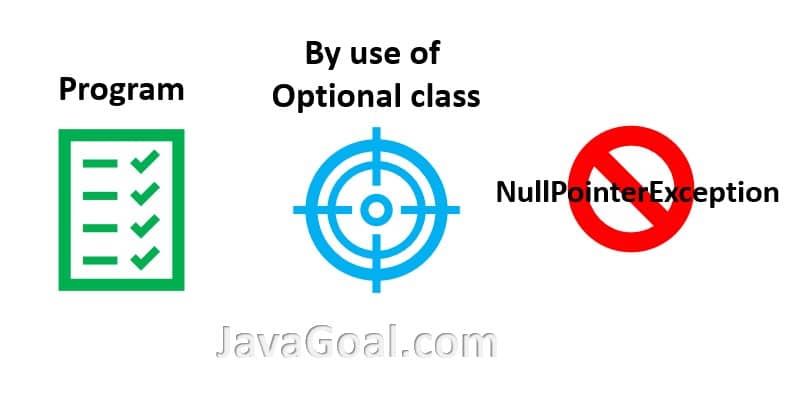
Methods in the Optional class
The optional class has various methods that are used to perform the operation on the object optional. It contains static or non-static methods.
Firstly, we will discuss some methods that are used to create an object of the Optional class:
1. static <T> Optional<T> empty(): It is used to create an empty optional. It means there is no value present.
import java.util.Optional; public class WithOptional { public static void main(String[] args) { Optional<String> objOfOptional = Optional.empty(); // Return true if it contain non-null value else false System.out.println("Is value Present = "+objOfOptional.isPresent()); } }
Output: Is value Present = false
2. static <T> Optional<T> of(T value): This method is used to create an object of optional that contains a non-null value. If you try to pass any null value it will throw NullPointerException.
import java.util.Optional; public class WithOptional { public static void main(String[] args) { Optional<String> objOfOptional = Optional.of("Hello"); // Return true if it contain non-null value else false System.out.println("Is value Present = "+objOfOptional.isPresent()); } }
Output: Is value Present = true
3. static <T> Optional<T> ofNullable(T value): This method is used to create an object of optional that may contain a null value.
import java.util.Optional; public class WithOptional { public static void main(String[] args) { Optional<String> objOfOptional = Optional.ofNullable("Hello"); // Return true if it contain non-null value else false System.out.println("Is value Present = "+objOfOptional.isPresent()); Optional<String> objOfOptional1 = Optional.ofNullable(null); // Return true if it contain non-null value else false System.out.println("Is value Present = "+objOfOptional1.isPresent()); } }
Output: Is value Present = true
Is value Present = false
How to check whether the value exists/presented or not?
4. boolean isPresent(): This method is used to check whether the optional is contain any value or not. It returns true if the value is present otherwise false.
import java.util.Optional; public class WithOptional { public static void main(String[] args) { Optional<Integer> objOfOptional = Optional.of(1); // Return true if it contain non-null value else false System.out.println("Is value Present = "+objOfOptional.isPresent()); Optional<String> objOfOptional1 = Optional.ofNullable(null); // Return true if it contain non-null value else false System.out.println("Is value Present = "+objOfOptional1.isPresent()); } }
Output: Is value Present = true
Is value Present = false
5. void ifPresent(Consumer<? super T> action): This method is used to perform given action on a given value if the value is present in optional, otherwise it doesn’t perform any operation.
import java.util.Optional; import java.util.function.Consumer; public class WithOptional { public static void main(String[] args) { Optional<String> objOfOptional = Optional.of("Hi"); objOfOptional.ifPresent((a) -> System.out.println("The optional contain value = "+a)); Optional<String> objOfOptional1 = Optional.ofNullable(null); objOfOptional1.ifPresent((a) -> System.out.println("The optional contain value")); } }
Output: The optional contain value = Hi
6. void ifPresentOrElse (Consumer<? super T> action, Runnable emptyAction): This method is used to perform a given action on a given value if the value is present in optional, else it performs the emptyAction operation. You can specify the action which you want to perform if the value id not present.
import java.util.Optional; import java.util.function.Consumer; class check implements Runnable { @Override public void run() { // TODO Auto-generated method stub } } public class WithOptional { public static void main(String[] args) { Optional<String> objOfOptional = Optional.of("Hi"); Optional<String> objOfOptional1 = Optional.ofNullable(null); Consumer<String> consumer = (a) -> System.out.println("The optional contain value = "+a); Runnable emptyAction = () -> System.out.println("This is else part"); objOfOptional.ifPresentOrElse(consumer, emptyAction); objOfOptional1.ifPresentOrElse(consumer, emptyAction); } }
Output: The optional contain value = Hi
This is else part
7. T get(): This method is used to get the value from optional. It returns the value, If the value is present in this Optional, otherwise throws NoSuchElementException.
import java.util.Optional; public class WithOptional { public static void main(String[] args) { Optional<String> objOfOptional = Optional.of("Hi"); System.out.println(objOfOptional.get()); } }
Output: Hi
8. T orElse(T other): This method is used to get the value from optional. It returns the value, If the value is present in this Optional, otherwise return the specified value provided as the parameter.
import java.util.Optional; public class WithOptional { public static void main(String[] args) { Optional<String> obj = Optional.of("Hi"); System.out.println("Value from Optional = "+obj.orElse("Hello")); Optional<String> obj1 = Optional.ofNullable(null); System.out.println("Value from specifeid parameter = "+obj1.orElse("Hello")); } }
Output: Value from Optional = Hi
Value from specifeid parameter = Hello
9. Optional<T> filter(Predicate<? super T> predicate): This method returns an object of Optional. If the value is present and matched with the given Predicate. Otherwise, it returns an empty Optional.
import java.util.Optional; public class WithOptional { public static void main(String[] args) { Optional<String> obj = Optional.of("Hello"); Optional<String> objByFilter = obj.filter(a -> a.equals("Hello")); System.out.println("Is this empty optional = "+ objByFilter.isPresent()); Optional<String> objByFilter1 = obj.filter(a -> a.equals("Hi")); System.out.println("Is this empty optional = "+ objByFilter1.isPresent()); } }
Output: Is this empty optional = true
Is this empty optional = false