Immutable objects are those objects that can’t change after creation. We can’t modify the state of an immutable object and if we try to modify it then it creates a new object. In Java, all the wrapper classes (like Integer, Boolean, Byte, and Short) and the String class are immutable. In this post, we will learn what is immutable class and how to create immutable class in java.
Here is the table content of the article we will cover this topic.
1. Immutable class in java?
2. benefits of immutable class?
3. How to create immutable class?
4. Immutable class in java example?
5. Immutable class with mutable object in java?
6. Why Deep Copy is important for immutability?
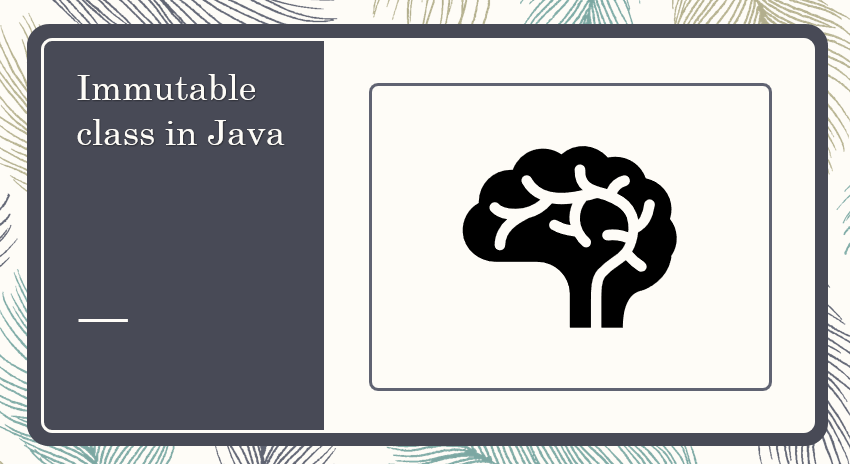
Immutable class in java?
A class is considered an immutable class if the object of the class is immutable. It means we can’t change the state of the object after construction. The Immutable class doesn’t provide any way for other objects to modify the state of the java immutable object.
For example, the String class is an immutable class, and we can’t modify the value of the string after initialization. You can see why String is an immutable class.
benefits of immutable class?
1. A developer can be sure that no one is able to change the state of the object. Sometimes it helps to maintain security.
2. The immutable object can’t be modified by multiple threads at the same because it creates a new object for each modification. So, we don’t need to care about synchronization.
3. The objects of the immutable class can be easily cached by VM cache or custom implementation. Because we are sure the values can’t be changed.
How to create an immutable class?
1. The class must be final so that no other classes can extend it.
2. All the data members (Variables) must be declared as final, so that, they can’t change the value of it after object creation.
3. No setter method should be there.
4. Create a getter method for each data member.
5. A parametrized constructor that will assign the value during the creation of the object.
6. If the class holds the reference of another class(mutable object):
The constructor should not assign real instances to mutable objects. It must create a clone copy of the passed argument and then use the clone copy. Make sure to always return a clone copy of the field and never return the real object instance.
Immutable class in java example
Let’s create an immutable class with the final keyword and final data members. Here we are creating an ImmutableStudent class, which is an immutable class.
final class ImmutableStudent { private final int id; private final String name; public ImmutableStudent(int id, String name) { this.name = name; this.id = id; } public int getId() { return id; } public String getName() { return name; } }
Immutable class with the mutable object in java
As we have seen custom immutable class in java in the above section. But An immutable class can contain a mutable object. Let’s see how a mutable object works in an immutable class.
final class ImmutableStudent { private final int id; private final String name; private final Address address; public ImmutableStudent(int id, String name, Address address) { this.name = name; this.id = id; this.address = address; } public int getId() { return id; } public String getName() { return name; } public Address getAddress() { return address; } } class Address { private String city; private String State; private int code; public String getCity() { return city; } public void setCity(String city) { this.city = city; } public String getState() { return State; } public void setState(String state) { State = state; } public int getCode() { return code; } public void setCode(int code) { this.code = code; } } public class ImmutableExample { public static void main(String arg[]) { Address address = new Address(); address.setCity("Chandigarh"); address.setState("Chandigarh"); address.setCode(12345); ImmutableStudent student = new ImmutableStudent(1, "Ram", address); System.out.println("Before modification: "+ student.getAddress().getCode()); student.getAddress().setCode(55555); System.out.println("After modification: "+ student.getAddress().getCode()); } }
Output: Before modification: 12345
After modification: 55555
In the above example, we are able to modify the address of the Student because the address is a mutable object. So, it is breaking the concept of immutable class. Because we are directly assigning the reference of address in the constructor, whenever the referenced address is modified outside the class, the change is reflected directly. We can fix this problem by the use of the clone instance. We will see this in the next section.
Why Deep Copy is important for immutability?
We can make an immutable class that contains a mutable object. We have to do deep cloning. You can read deep cloning from a separate post.
final class ImmutableStudent { private final int id; private final String name; Address address = new Address(); public ImmutableStudent(int id, String name, Address address) { this.name = name; this.id = id; this.address = address; } public int getId() { return id; } public String getName() { return name; } public Address getAddress() throws CloneNotSupportedException { return (Address) address.clone(); } } class Address implements Cloneable { private String city; private String State; private int code; public String getCity() { return city; } public void setCity(String city) { this.city = city; } public String getState() { return State; } public void setState(String state) { State = state; } public int getCode() { return code; } public void setCode(int code) { this.code = code; } public Object clone() throws CloneNotSupportedException { return super.clone(); } } public class ImmutableExample { public static void main(String arg[]) throws CloneNotSupportedException { Address address = new Address(); address.setCity("Chandigarh"); address.setState("Chandigarh"); address.setCode(12345); ImmutableStudent student = new ImmutableStudent(1, "Ram", address); System.out.println("Before modification: "+ student.getAddress().getCode()); student.getAddress().setCode(55555); System.out.println("After modification: "+ student.getAddress().getCode()); } }
Output: Before modification: 12345
After modification: 12345
What if you don’t support clone method , How can we solve immutable now.
Do we need to deep copy
Cloneable of Address class is not supported, Think we have any arrayList of number with in immutable class .
You have to use deep copy if you have reference variables. Please visit here once : https://javagoal.com/cloning-in-java/#8