In this post, we will see how we can add elements in the ArrayList in java. There are two methods arraylist add() and ArrayList addAll() but both are overloaded. So let’s see in java add to list different elements and collections.
1. add(E e)
2. add(int index, E e)
3. add(Collection c)
4. add(int index, Collection c)
Java ArrayList add(E e)
In ArrayList add() method is used to add the given element in ArrayList. This method appends the specified element (e) to the end of the ArrayList. It returns a boolean value, It returns true if the given element added successfully else false.
boolean add(E e);
Where E represents the type of elements in the ArrayList.
e is the element that you want to add in the ArrayList.
return type: Its return type is boolean.
import java.util.ArrayList; public class ArraylistExample { public static void main(String[] args) { ArrayList<String> listOfNames = new ArrayList<String>(); listOfNames.add("JAVA"); listOfNames.add("GOAL"); listOfNames.add("Website"); // Adding Same element again boolean isSuccessFullyAdded = listOfNames.add("GOAL"); System.out.println("Element added again = "+ isSuccessFullyAdded); for(String name : listOfNames) { System.out.println("Names from list = "+name); } } }
Output: Element added again = true
Names from list = JAVA
Names from list = GOAL
Names from list = Website
Names from list = GOAL
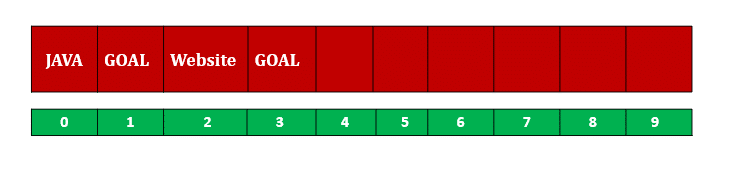
Now you can see the reason for duplicate elements in ArrayList. Each element is stored in different index value, so it doesn’t care whether the element already exists or not?
add(int index, E e)
When we want to add the specified element at the specified position in ArrayList. Suppose we have an ArrayList that containing 4 elements, now we want to add a new element at position 2. Its return type is void. When it inserts the element at the specified position, it shifts the element which presents at the current position.
boolean add(int index, E e);
Where index, index at which the specified element is to be inserted.
E, represents the type of elements in ArrayList.
e, is the element which you want to add in ArrayList.
return type: Its return type is void.
throw, IndexOutOfBoundsException if index is invalid.
import java.util.ArrayList; public class ArraylistExample { public static void main(String[] args) { ArrayList<String> listOfNames = new ArrayList<String>(); listOfNames.add("JAVA"); listOfNames.add("GOAL"); listOfNames.add("Website"); // Adding element at specified position listOfNames.add(1, "Learning"); for(String name : listOfNames) { System.out.println("Names from list = "+name); } } }
Output: Names from list = JAVA
Names from list = Learning
Names from list = GOAL
Names from list = Website
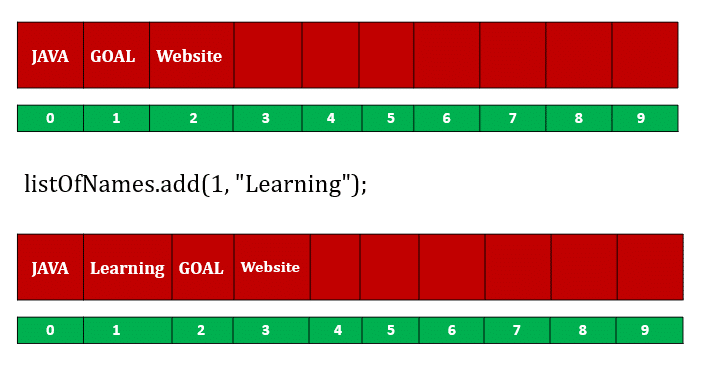
ArrayList addAll(Collection c)
In Java, ArrayList addAll() is used to appends all the elements of the specified collection to the end of the ArrayList. It returns boolean value. It returns true, after adding the specified collection. It throws the NullPointerException if the specified collection is null.
boolean addAll(Collection c);
Where, c collection containing elements to be added to this list.
throws NullPointerException, if the specified collection is null.
return type, Its return type is boolean.
import java.util.ArrayList; public class ArrayListExample { public static void main(String[] args) { ArrayList<String> listOfNames = new ArrayList<String>(); listOfNames.add("JAVA"); listOfNames.add("GOAL"); listOfNames.add("Website"); ArrayList<String> listOfNames2 = new ArrayList<String>(); listOfNames2.add("Learning"); listOfNames2.add("Concepts"); listOfNames.addAll(listOfNames2); for(String name : listOfNames) { System.out.println("Names from list = "+name); } } }
Output: Names from list = JAVA
Names from list = GOAL
Names from list = Website
Names from list = Learning
Names from list = Concepts

ArrayList addAll(int index, Collection c)
The ArrayList addAll(int index, Collection c) method is used to appends all the elements of the specified collection at a specified position in ArrayList. Its return type is boolean. It inserts all the elements in the specified collection into the ArrayList, starting at the specified position and Shifts the elements present at the current position.
boolean addAll(int index, Collection c);
Where index, index at which the specified element is to be inserted.
c, collection containing elements to be added to this list.
throws NullPointerException, if the specified collection is null.
return type, it’s return type is boolean.
package package1; import java.util.ArrayList; public class ArrayListExample { public static void main(String[] args) { ArrayList<String> listOfNames = new ArrayList<String>(); listOfNames.add("JAVA"); listOfNames.add("GOAL"); listOfNames.add("Website"); ArrayList<String> listOfNames2 = new ArrayList<String>(); listOfNames2.add("Learning"); listOfNames2.add("Concepts"); listOfNames.addAll(1, listOfNames2); for(String name : listOfNames) { System.out.println("Names from list = "+name); } } }
Output: Names from list = JAVA
Names from list = Learning
Names from list = Concepts
Names from list = GOAL
Names from list = Website
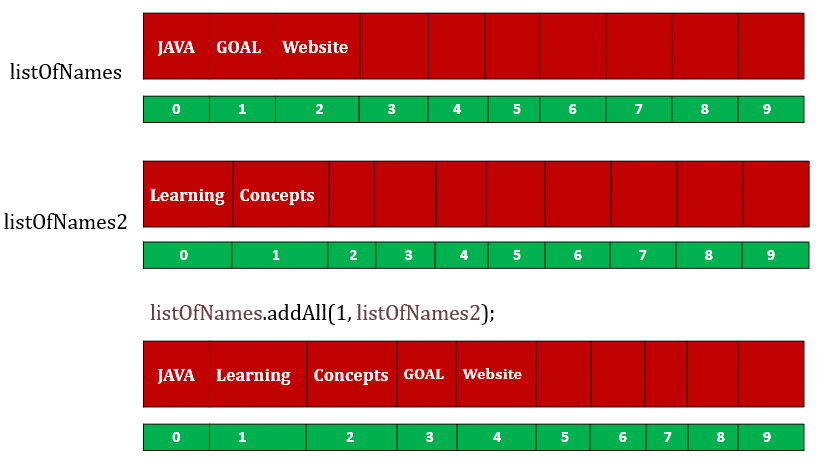