Here is the table content of the article will we will cover this topic.
1. What is Constructor in java?
2. Properties of Constructor?
3. Defining a Constructor in Java?
4. How does the constructor work?
5. How does the constructor work in heap memory?
6. Important points about Constructor?
7. Rules for the constructor in java?
8. Two types of constructors in Java?
9. Default constructor(without arguments/parameters)?
10. The reason behind the use of a default constructor?
11. Parameterized constructor?
12. Constructor overloading in java?
13. Constructor chaining in java?
What is Constructor in java?
A constructor in java is like a method (but not actually a method). The constructor has the same name as the class, and it is invoked automatically when an object is instantiated. In other words, when you use the new keyword. A constructor does not return any value. Whenever we are creating an object by use of a new keyword then it invokes the constructor and allocates some memory for the object in heap memory.
Properties of Constructor
1. A constructor must have the same name as that of the class.
2. It doesn’t return any type of value.
3. A constructor is used to create and initialize the object. Whenever we create an object by new keyword then JVM internally invokes the constructor.
4. Every time an object is created using the new keyword, at least one constructor is called.
5. Every class has a default constructor by default that provides by the java compiler.
6. Constructors are automatically chained by using this keyword and the super keyword.
7. Like a method, you can also overload constructors in Java. We will discuss it later.
Defining a Constructor in Java
Before defining the constructor let’s have a look at the syntax of the constructor.
class class_Name { accessModifier constructor_Name(Parameters) { // Body of constructor } }
Access modifies(accessModifier): The access modifier defines the accessibility of the constructor. It has the same meanings as for methods and fields. Also, you can use the access modifiers with the constructor and set the access of the constructor. For more details on the access modifier of the constructor, you read the article in detail here.
Constructor name(constructor_Name): The name of the constructor should be exactly the same as the name of the class. Another, The constructor has no return type, like other methods.
Parameters: Like a method, You can declare a list of parameters in between the parentheses( ). If you are not providing any parameters then the compiler considered it the default constructor automatically.
Body: The body of the constructor is defined inside the curly brackets { } after the parameter list.
Example:
class Demo { public Demo() { // Body of constructors } }
How does the constructor work?
In Java, each class has at least one constructor that is provided by the compiler. Whenever an object is created by using a new keyword then at least one constructor is invoked and allocates some memory to the object.
Similarly, to understand the working of the constructor more closely we will discuss it with examples.
Suppose we have a class Student and we create an object of Student by using the new keyword. Then JVM internally invokes the constructor of Student class. In this class, we are creating a default constructor(No- argument constructor) and print some statements within the constructor. Therefore, Let’s see how it invokes the constructor.
public class Student { int roll; String className; Student() { System.out.println("Body of default constructor"); } public static void main(String arg[]) { System.out.println("Object is going to create"); Student s1 = new Student(); } }
Output: Object is going to create
Body of default constructor
The new keyword here creates the object of the class Student and invokes the constructor to that defined in the class. The constructor initializes the object in memory and also prints the statements. So, It initializes the object in heap memory.

How does the constructor work in heap memory?
When the constructor gets the call, it does check the initial value of variables. Even the developer can provide the initial value to variables. In the above example developer is not providing any initial value to variables. So, the default constructor provides the default values to a variable and initializes them in heap memory.
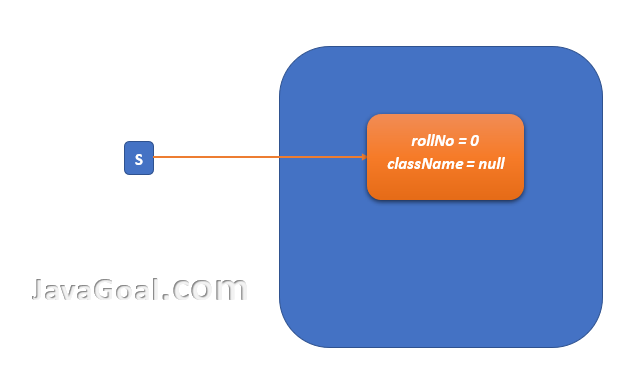
Important points about Constructor
1. The name of the constructor must be exactly the same as the class name. If you are providing any other name, then the compiler will show an error.
2. A class can have any number of constructors as methods. Hence, It means we can overload the constructors.
3. A constructor doesn’t have a return type. The constructor looks like a method, but it is different than methods and doesn’t return anything. Java Constructor is by default of type void.
4. You can use a return statement inside the constructor. But you can’t return any value. Because Constructors are by default of type void. Using a return statement, you can return the control flow from the constructor.
5. Every Class contains a default constructor by default. This constructor is provided by the compiler.
6. If the programmer doesn’t declare any parameterized constructor then the compiler automatically inserts a default constructor at the back end.
7. If the Programmer provides any parameterized constructor then the compiler doesn’t provide the default constructor. It is the responsibility of the programmer to provide the default constructor. Otherwise, the compiler throws the exception.
8. By use of this keyword and super keyword, you can call one constructor to another constructor. This property is known as constructor chaining.
9. In java, the constructor is by default with a default access modifier. In the same way, you can use any access modifier with a Java constructor. Hence, you can make public, protected, or private constructors.
Rules for the constructor in java
1. The constructor name must be the same as its class name.
2. A constructor doesn’t have an explicit return type.
3. Java constructors cannot be abstract, static, final, and synchronized
4. We can use an access modifier while declaring a constructor. So, we have private,
protected, public, or, default constructors. Access modifier is used to control its access i.e.
which other classes can call the constructor.
Two types of constructors in Java
1. Default constructor(without arguments/parameters)
2. Parameterized constructor.
1. Default constructor(without arguments/parameters)
It is a constructor that has no parameter known as the default constructor. If you are not creating any constructor in a class, then the compiler creates a default constructor for the class. But if you are creating any constructor (with arguments or default) then the compiler does not create a default constructor. Then it is the responsibility of a programmer to create a constructor according to the use in an application.
class_Name() { // body of default constructor }
public class Student { int rollNo; String className; //default construcotr Student() { System.out.println("This is default constructor of class Student"); } public static void main (String arg[]) { // Creating a object that will call the default constructor Student newStudent = new Student(); } }
Output: This is the default constructor of class Student
The reason behind the use of a default constructor?
The default constructor is used to provide the default values to the variables, objects like 0, null, etc., depending on the type. So, Here I will show you the compiler provides automatically a default constructor that provides default values.
Example of default constructor with default values
public class Student { int rollNo; String className; boolean isFromCity; public void studentData() { System.out.println("This is default constructor of class provides default values"); System.out.println("Roll no = "+ rollNo); System.out.println("Class Name = "+ className); System.out.println("Is student belongs to city = "+ isFromCity); } public static void main (String arg[]) { // Creating a object that will call the default constructor Student newStudent = new Student(); newStudent.studentData(); } }
Output:
This is default constructor of the java class provides default values
Roll no = 0
Class Name = null
Is student belongs to city = false
Memory representation with default values:
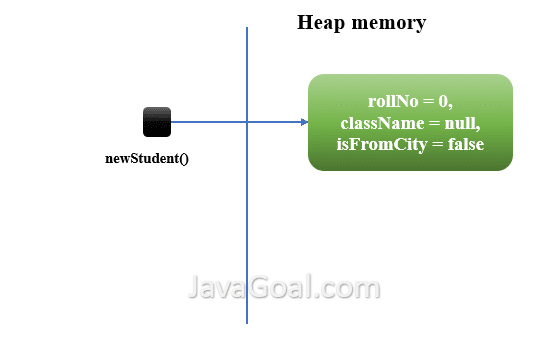
2. Parameterized constructor
A constructor that has a parameter is known as a parameterized constructor. By use of parameterized constructor, you can also initialize the values of fields. You can provide the values to the parameterized constructors when you are creating an object.
Let’s see Java program to print student details using the constructor:
class_Name(paramerters/agruments) { // body of constructor }
public class Student { String name; int rollNo; String className; boolean isFromCity; Student(String name, int rollNo, String className, boolean isFromCity) { this.name = name; this. rollNo = rollNo; this.className = className; this.isFromCity = isFromCity; } public void studentData() { System.out.println("Name of Student = " + name); System.out.println("Roll no = "+ rollNo); System.out.println("Class Name = "+ className); System.out.println("Is student belongs to city = "+ isFromCity); } public static void main (String arg[]) { // Creating object and providing some values by using parameterized constructor Student student1 = new Student("Ravi", 1, "MCA", false); student1.studentData(); Student student2 = new Student("Ram", 2, "MCA", true); student2.studentData(); } }
Output:
Name of Student = Ravi
Roll no = 1
Class Name = MCA
Is student belongs to city = false
Name of Student = Ram
Roll no = 2
Class Name = MCA
Is student belongs to city = true
Important point: In this example, we created only a parameterized constructor. If we create an object by using the default constructor, then it will throw a compilation error.
Student student3 = new Student(); // compilation error
The compiler always provides a default constructor to the class but if the programmer providing any parameterized constructor then it’s the programmer’s responsibility to provide the default constructor.
Saved as a favorite!, I really like your website!
Thanks Alden
I bookmarked this website in my laptop. Crystal clear explanation.
Thankyou Shabarish Kumar
I and my friends have already been reviewing the excellent information and facts located on the website and then quickly developed a horrible suspicion I had not thanked the web blog owner for them. My young boys had been as a consequence thrilled to read all of them and have now actually been using these things. Appreciate your actually being really accommodating and for figuring out this kind of marvelous information most people are really eager to know about. Our sincere apologies for not expressing gratitude to earlier.
เล่นสล็อตฟรี Thankyou 🙂
Clear Explanation with Proper Example….
Thankyou 🙂
Excellent explanation with perfect examples. And more I liked is the multiple-choice which you mentioned after each concept it really makes me stronger in my programming skills. Thank you so much for filling the confidence level in the JAVA programming language.
Good learning platform for everyone, its given all basics knowledge according .