You must hear about finalize() method and Garbage collection in Java. Lots of questions in programmers’ minds and viewpoints on it. We will try to explain it in detail and will cover some most important points like – When is it needed, how often should it run, how to know when it is not operating as expected, and so on.
Here is the table content of the article will we will cover this topic.
1. What is Garbage Collection in Java?
2. Important points about Garbage Collection?
3. When an object becomes eligible for garbage collection?
4. What is Garbage Collector?
5. How does Garbage Collector work?
6. How Garbage Collector Works in Heap memory internally?
7. Ways for requesting JVM to run Garbage Collector?
8. Types of the garbage collector?
What is Garbage Collection in Java?
In simpler term Garbage means waste. But in terms of Java, Garbage means unreferenced objects. By means of unreferenced objects that are in heap memory, but they are useless. They are not in use in application anywhere and they should be destroyed. The process of removing unused objects from heap memory is known as Garbage collection
1. Garbage Collection is a process that is used to destroy the unused object and claiming the runtime unused memory automatically.
2. The garbage collection process is handled by Garbage Collector and it performs an operation for automatic memory management.
3. Unlike C and C++ language, In java, it is performed automatically. In C and C++, the programmer is responsible for both the creation and destruction of objects. But in java Garbage Collector takes care of destroying the objects those are not in use.
class Eaxmple { public static void main(String arg[]) { System.out.println("Creating new Object of String type in Heap memory"); String s = "Hello"; System.out.println("Creating new Object of Integer type in Heap memory"); Integer a = new Integer(123); System.out.println("The refernce points to NULL, iligible for Garbage Colledction"); s = null; } }
Output: Creating new Object of String type in Heap memory
Creating new Object of Integer type in Heap memory
The refernce points to NULL, iligible for Garbage Colledction
As you can see in the above example, we are creating two objects.
String s = "Hello"; Integer a = new Integer(123);
The JVM creates the objects in the heap memory but after that, we are reassigning the value to reference s.
s = null;
The object “Hello” still presented in heap memory but it is not referred by any referenced. Because the reference s is no pointing to NULL and now “Hello” is eligible for garbage collection. Let’s try to understand it with example.
In this diagram, you will see the object is presented in Heap memory and referenced by reference variables.
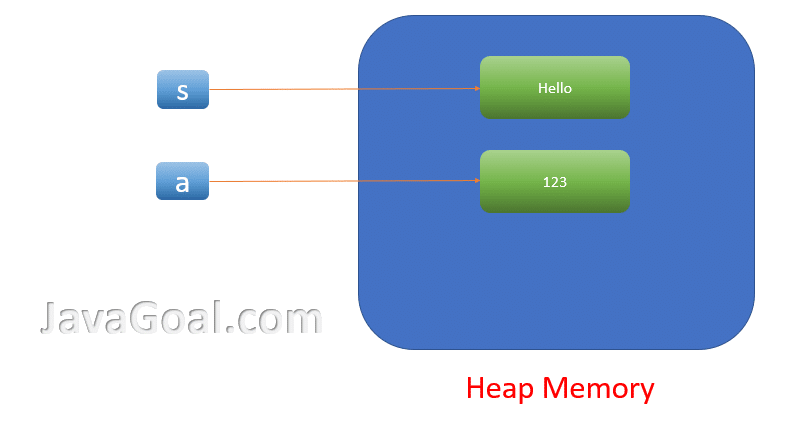
As you can see in the below diagram the “Hello” object is not referencing by any variable. Because s referred to NULL(s = null). The garbage collector collects this object remove from memory.

Important points about Garbage Collection
1. It is a process handled by JVM(Java Virtual Machine) to reclaim heap space from objects which are not in use and eligible for Garbage collection.
2. It is an automatic process that is handled by JVM, So the programmer doesn’t need to take responsibility for memory management.
3. The garbage collector is a daemon thread that always runs in the background and takes care of Garbage Collection.
4. The garbage collector always invokes the finalize() method before removing an object from memory. It gives an opportunity to perform cleanup required.
5. We can’t force the JVM to clean or remove some objects from memory. It always depends on JVM. Most probably the JVM calls Garbage Collector if there is less memory available in the Heap area or when the memory is low.
6. Whenever System.gc() is called it makes a request to JVM to execute the Garbage Collector. The JVM decides when to call Garbage Collector.
7. If most of the objects are alive in memory and heap haven’t memory space for creating a new object. Then JVM throws OutOfMemoryError or java.lang.OutOfMemoryError heap space
8. System.gc() and Runtime.gc() are hooks to request the JVM to initiate the garbage collection process.
When an object becomes eligible for garbage collection?
As we know Garbage collection removes the objects from memory that is not in use. But here a question comes in mind, how garbage collection checks whether the object is eligible for garbage collection?
An object can’t be eligible for Garbage collection until all references of the object are discarded. Let’s try to understand the reference of the object. Whenever a new object is created by the use of a new operator then JVM allocates memory for an object and returns a reference to it. The returned reference is used to operate the object.
Person p = new Person();
Here p is the reference of the object (new Person). This object exists in heap memory.
Let’s discuss it with some scenarios in those cases object is eligible for garbage collection.
1. The object created in limited scope
In java, when a method is get called it popped from the stack and all its members are destroyed. If we have any object that is created inside the method, then after the execution of the method object becomes unreachable or anonymous.
class Data { //Body of class } class Example { public static void main(String arg[]) { // This object will destroy when class will destroy Data data1 = new Data(); check(); } private static void check() { // This object is created in method // After execution of method object will be destroy Data data2 = new Data(); } }
If you want to verify it then you can use the finalize() method. Because this method is called by Garbage collector just before the destruction of the object from memory. Here we are discussing it in a separate post ( finalize() method ).
2. Reassigning the reference variable
Let’s say we have two objects with references.
Data data1 = new Data(); Data data2 = new Data();
As of now, we are creating two objects and JVM assigns some memory to them as shown below. But If the reference of one object (data2) is assigned to the reference of another object (data1).
Then the previous object (which referred by data1) has no longer reference to it and becomes unreachable and thus becomes eligible for garbage collection.
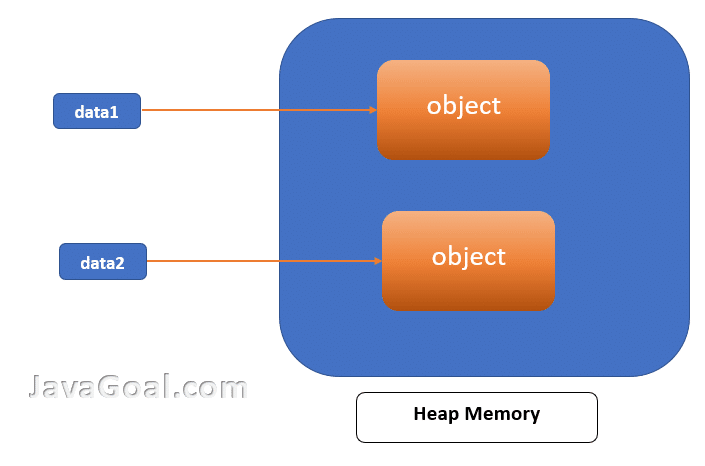
data1 = data2;
After the execution of the above statement, both data1 and data2 are referring to one object.

3. Reference variable point to NULL
If any reference variable of an object is changed to NULL during the execution of the program then becomes eligible for garbage collection.
class Example { public static void main(String arg[]) { String s = "Hi"; int a = 5; if(a > 3) { //Eligible for Garbage collection s = null; } } }
4. Anonymous object
If we are creating any object and the reference object is not storing anywhere. It means it is an anonymous object and eligible for garbage collection.
class Example { public static void main(String arg[]) { // These are anonymous objects new String(); new Integer(1); new String("Hello"); } }