You have read the HashMap in Java and now we will see how to insert objects in HashMap. The HashMap class provides some methods like Java HashMap put() method, putAll() method(java map put all). Here we will discuss HashMap put example.
Here is the table content of the article will we will cover this topic.
1. put(K key, V value) method
2. putAll(Map map) method
3. putIfAbsent(K key, V value) method
put(K key, V value) method
This method is used to set the value with the corresponding key in HashMap. It inserts the entry(Key-Value) in the hashmap. If the HashMap already contained the key, then the old value is replaced by a new Value.
It returns null if there is no value presented in the hashmap for the given key. But it returns the old object if there is any value presented with the given key.
You can read the internal working of put() method
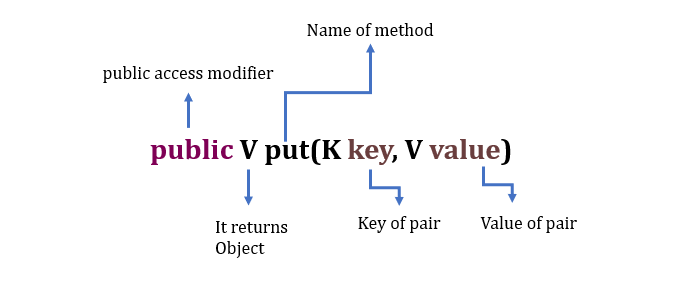
Where K is the key with which the specified value(V) is to be associated in HashMap.
V is the value to be associated with the specified key(K) in HashMap.
return NULL, if there was no mapping for key. It returns the previous value if the associated key already present.
import java.util.HashMap; public class ExampleOfHashMap { public static void main(String[] args) { HashMap<Integer, String> hashMap = new HashMap<Integer, String>(); // Adding element in HashMap // The put method returns the old object after performing insertion // It will return null if there is no mapping for the given key String previousObject = hashMap.put(1, "JavaGoal"); System.out.println("Previous object: "+ previousObject); // It will return the previous object and replace with a new object for given key previousObject = hashMap.put(1, "JavaGoal.com"); System.out.println("Previous object: "+ previousObject); hashMap.put(2, "Learning"); hashMap.put(3, "Website"); System.out.println("All Pair of HashMap: "+hashMap); } }
Output: Previous object: null
Previous object: JavaGoal
All Pair of HashMap: {1=JavaGoal.com, 2=Learning, 3=Website}
putAll(Map map) method
This method is used to copy all elements of the specified map in the current HashMap. It copies all the mapping(Key and value pairs) of the specified Map to the current HashMap. It returns NullPointerException if the given map is null.
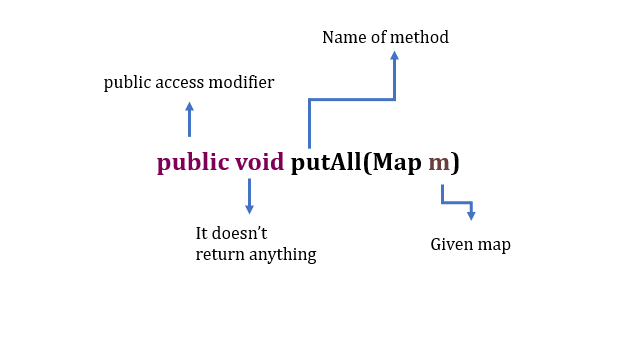
Where, map is specified Map which you want to copy.
return Void, it doesn’t return anything.
import java.util.HashMap; public class ExampleOfHashMap { public static void main(String[] args) { HashMap<Integer, String> hashMap = new HashMap<Integer, String>(); hashMap.put(1, "JavaGoal.com"); hashMap.put(2, "Learning"); hashMap.put(3, "Website"); HashMap<Integer, String> newHashMap = new HashMap<Integer, String>(); newHashMap.putAll(hashMap); System.out.println("All Pair of HashMap: "+newHashMap); } }
Output: All Pair of HashMap: {1=JavaGoal.com, 2=Learning, 3=Website}
putIfAbsent(K key, V value) method
This method is used to put the given value with the corresponding key in HashMap. If the specified key is not already present in HashMap. It works similarly like put() method except the if condition.
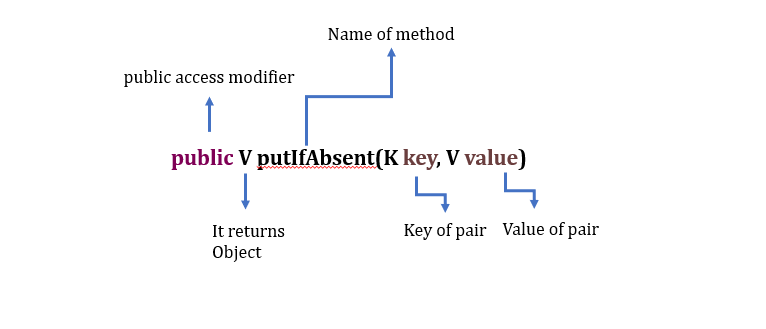
Where K is the key with which the specified value(V) is to be associated with HashMap.
V is the value to be associated with the specified key(K) in HashMap.
return NULL, if there was no mapping for key. It returns the previous value if the associated key already present.
import java.util.HashMap; public class ExampleOfHashMap { public static void main(String[] args) { HashMap<Integer, String> hashMap = new HashMap<Integer, String>(); // Adding element in HashMap String previousObject = hashMap.putIfAbsent(1, "JavaGoal.com"); System.out.println("Previous object: "+ previousObject); hashMap.put(2, "Learning"); hashMap.put(3, "Website"); System.out.println("All Pair of HashMap: "+hashMap); } }
Output: Previous object: null
All Pair of HashMap: {1=JavaGoal.com, 2=Learning, 3=Website}