We have learned, how to insert objects in HashMap and get the element from HashMap. In this post, we will learn how to remove object from HashMap by use of hashmap remove() method. We can remove the entry from the HashMap by value or key (java map remove by value or java hashmap remove).
Here is the table content of the article will we will cover this topic.
1. remove(Object key) method
2. clear() method
remove(Object key) method
This method is used to remove an entry from the HashMap for the specified key. It removes the entry(key-value), if there is mapping with of key with any pair otherwise it remains the same.
It returns the object after removal if entry is presented otherwise it returns null.
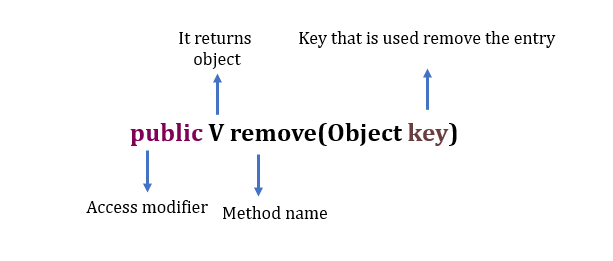
Where, the key is the key that you want to remove from the HashMap.
return type: Its return type is V. It can return an object or null.
import java.util.HashMap; public class ExampleOfHashMap { public static void main(String[] args) { HashMap<Integer, String> hashMap = new HashMap<Integer, String>(); // Adding objects in HashMap hashMap.put(1, "JavaGoal.com"); hashMap.put(2, "Learning"); hashMap.put(3, "Website"); // Removing the object associated with key System.out.println("Removed object: "+hashMap.remove(2)); // Removing the object associated with key System.out.println("Removed object: "+hashMap.remove(5)); // After removal of object System.out.println("After removal of object : "+ hashMap); } }
Output: Removed object: Learning
Removed object: null
After removal of object : {1=JavaGoal.com, 3=Website}
To delete all pairs of keys and values in a given hashmap
We can delete all the pairs of keys and values from a HashMap by use of the clear() method. The HashMap will be empty after the clear() method.
clear() method
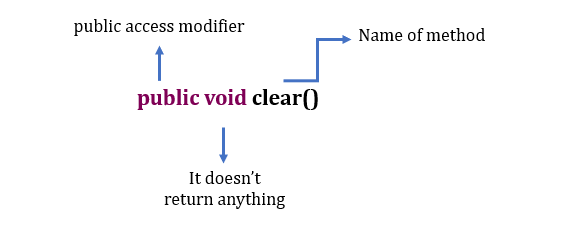
- This method doesn’t take any parameter.
- It doesn’t return anything.
- It is a public method so can be accessed from any class.
import java.util.HashMap; public class ExampleOfHashMap { public static void main(String[] args) { HashMap<Integer, String> hashMap = new HashMap<Integer, String>(); // Adding objects in HashMap hashMap.put(1, "JavaGoal.com"); hashMap.put(2, "Learning"); hashMap.put(3, "Website"); // Removing the all pairs hashMap.clear(); // After removal all entries System.out.println("After removal all entries: "+ hashMap); } }
Output: After removal all entries: {}