Java is an object-oriented programming language and it has four major pillars that are abstraction, encapsulation, inheritance, and polymorphism. In this article, we will discuss abstraction in java and abstraction in java with examples.
Here is the table content of the article will we will cover this topic.
1. What is abstraction?
2. What is an abstraction in Java?
3. Ways to achieve Abstraction in Java?
4. Abstract class in java?
5. Interface in java?
6. Difference between abstract class and concrete class?
7. Difference between abstract class and interface?
8. When to use abstract class and interface in java?
What is abstraction?
In simple terms, the meaning of Abstraction is to show the necessary things to the user and hide the details. In abstraction, the user will able to access only those parts of information that are necessary for them and known as data abstraction in java.
Real-life example: Suppose a person wants to drive a car. The person only needs to know about some basic things that are necessary for him. The necessary things to drive a car accelerator, clutch, and brakes. He doesn’t need to know about the inner mechanism of the car. This is the best example of abstraction.
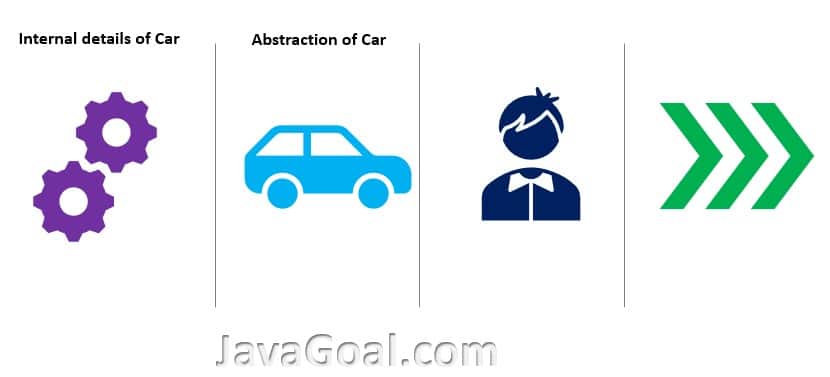
What is an abstraction in Java?
In Java, abstraction is a process of hiding the implementation details from the user and only provides the functionality to the user. The user will focus on the functionality rather than how it works.
An object contains a large amount of information. By use of Abstraction, we can show only the relevant information of the object to the user. It helps to reduce programming complexity and effort. It is one of the most important concepts of OOPs.
Let’s discuss the abstraction oops and abstraction in oops with examples. Let’s see the example of a banking application. In the banking application, A customer can see his/her account balance, mini statement, change password option, etc. So Banking software hides the fetch and updates operation from the customer. Because this will not create any value for the customer. Customer doesn’t care about it they just need to use the application functionality.
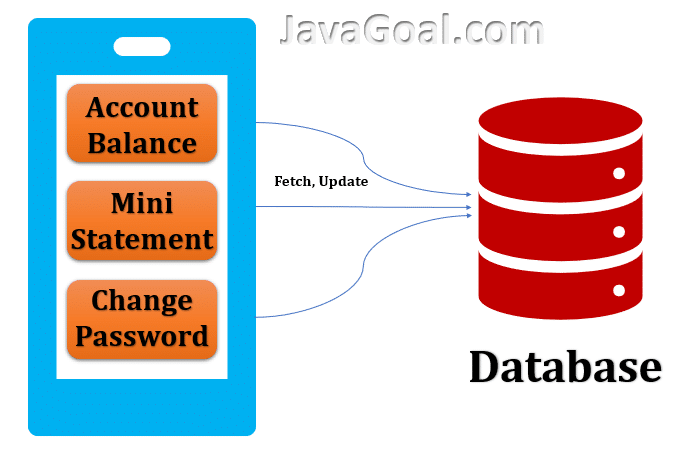
In Java, abstract class and interface used to achieve abstraction. The abstract class provides 1 to 100% of abstraction but by use of the interface, we can achieve 100% abstraction. We will discuss it later in detail.
Ways to achieve Abstraction in Java
1. Abstract class: When we declare a class with an abstract keyword is known as an abstract class. An abstract class can have abstract methods (methods without the body) and concrete/non-abstract methods (Methods with the body) also. A normal class can’t have any abstract method. In this article, we will discuss the abstract class in java, what are the properties of the abstract class, why we use an abstract class.
The abstract class provides the abstraction level from 1 to 100%. The level of abstraction is totally depending upon the abstract methods. If an abstract class has the abstract method and non-abstract method, then the abstraction level lies between 1 to 100. But if an abstract class contains only abstract methods then it provides 100% abstraction. We can use any number of abstract methods in the abstract class as per the use. For more detail.
2. Interface: The interface is a way to achieve 100% abstraction in JAVA. An interface is a blueprint of a class. An interface can have methods and variables like class, but the methods of an interface are by default abstract. It means the interface can contain only an abstract method(Method without body). But after java 7, we can create default methods in the interface. You can read that method here. For more detail.
Difference between abstract class and concrete class
1. Abstract class: A class that is declared with an abstract keyword is known as an abstract class. An abstract class can have abstract methods (methods without the body) and concrete/non-abstract methods (Methods with the body) also. The abstract class must have at least one abstract method(Method without body). A normal class can’t have any abstract method.

2. It is declared with only an abstract keyword. You can’t declare an abstract class without a special keyword. The abstract keyword indicates the JVM to make it an abstract class so that it can have an abstract method.
3. It can have abstract methods and concrete(non-abstract) methods. If we are declaring an abstract method in any class then it must be an abstract class otherwise it gives a compilation error. Because this is the general rule of the abstract class.
4. An abstract class may or may not have abstract methods.
5. An Abstract class can’t be declared as a final class because the final and abstract are opposite terms in JAVA.
Reason: An abstract class must be inherited by any derived class because a derived class is responsible to provide the implementation of abstract methods of an abstract class. But on another hand, if a class is a final class, then it can’t be extended(inherited). So both concepts are opposite to each other.
6. We can’t create an object of an abstract class. If java allows the creation of an object of the abstract class, then it will lead to two problems:
I). The first problem occurs because the abstract method has nobody. If someone calls the
abstract method using that object, then What would happen?
ii). Abstract classes are only like a template, to create an object of class the class should be
concrete. because an object is a concrete. An abstract class has no use without a concrete class. because you can use an abstract class only when it is extended by a concrete class.
1. Concrete class: In Java, A simple class(Without abstract keyword) is considered a concrete class. A concrete class implements all the abstract methods of its parent abstract class. The concrete class provides the implementations of all methods of interfaces it implements.
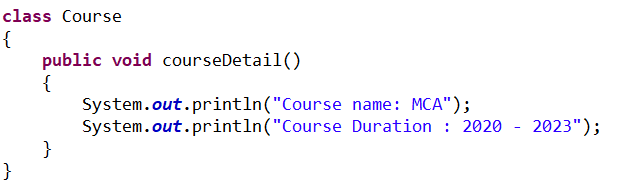
2. It is declared simply as a Java class (Without abstract keyword). By means of the concrete class are a complete class. As you know abstract classes are incomplete classes because they have an abstract method. But Concrete classes are considered a complete class.
3. It can’t have any abstract methods. Concrete classes can’t contain any abstract method if we are declaring an abstract method in any class then it must be an abstract class otherwise it gives a compilation error. Because this is the general rule of the abstract class.
4. It can’t contain any abstract methods.
5. A concrete class can be declared as a final class. We can make any concrete class as final according to use. A final class can’t be extended by any class.
6. We can create an object of the concrete class. The concrete class can work without abstract class. Because they are complete classes and independent.
Difference between abstract class and interface
In java, Abstract class and interface are very popular concepts. Both use to achieve abstraction in java. Here, we will see the difference between the abstract class and interface. There is a lot of difference between abstract class and interface in java.
Declaration and use
An interface declares by the interface keyword. Subclasses use implements keyword to implement interfaces
An abstract class is declared by the abstract keyword. Subclasses use extends keyword to extend an abstract class.
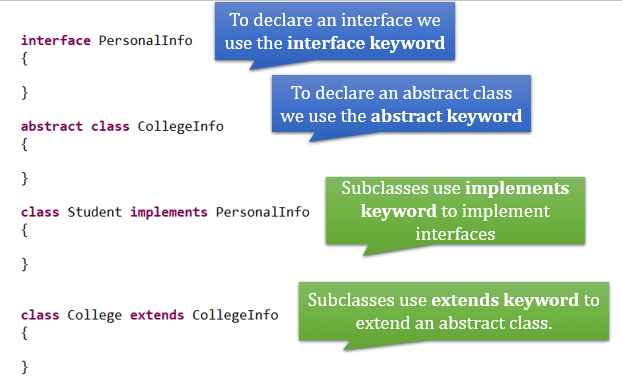
Variable
An interface has all the variables by default static and final. So, you must initialize them otherwise the compiler will throw an exception at compile time.
An abstract class can have final, non-final, static, and non-static variables. But if you declare a final variable then you must initialize it otherwise the compiler will throw an exception at compile time.

Method keywords
An Interface has all methods that are public and abstract by default. You can declare a method with the public, private, abstract, default, static and strictfp are permitted. If you try to use a protected modifier then it will throw an exception at compile time because it is not permitted the protected modifier. Since Java 8 we can define the default method and static method in the interface. In Java 9, we can define private methods also.
An abstract class is more flexible than the interface in declaring the methods. You can define abstract methods with protected modifier also. In the interface, the static method and default method are supported since Java 8 but in the abstract class, you could define the static and default method before java 8.
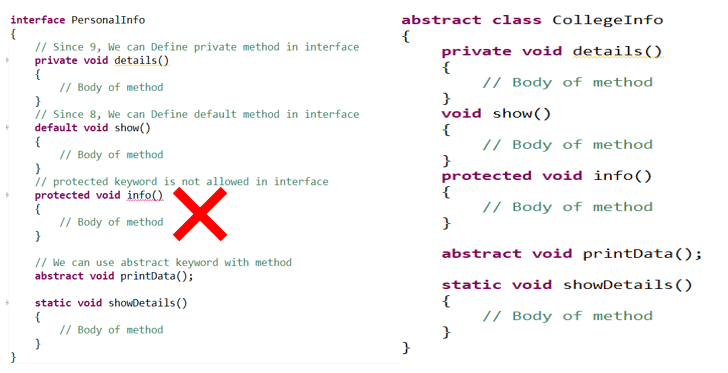
Inheritance
An interface can extend more than one interface. An Interfaces can extend other interfaces but it can’t not any class (abstract or concrete).
An abstract class can implement multiple interfaces. But it can’t extend more than one class.

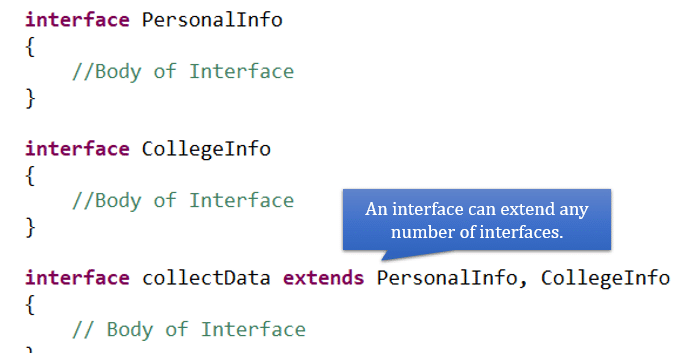
Support multiple inheritances
As you know a class can implement multiple interfaces. So, it does support multiple inheritances because a class can inherit properties from different interfaces. In Java 8, default methods are also used to support multiple inheritances.
A class can extend only one class whether it is the concrete class or abstract class. So, it doesn’t support multiple inheritances.
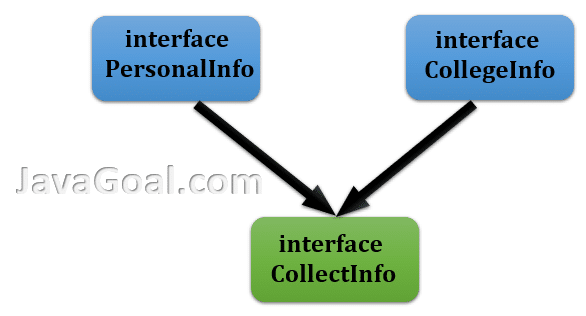
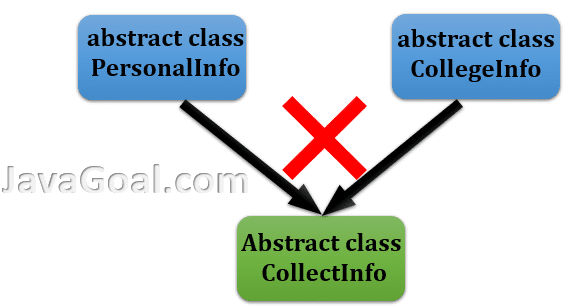
When to use
An interface would be a good choice when you want to achieve 100% abstraction. It means that multiple classes want to provide different implementations to the only method signature.
An abstract class would be a good choice when you want to achieve 1% to 100% abstraction. It should be used when multiple classes want to provide different implementations and also want to share some common implementations.
Constructor
An interface doesn’t contain any constructor. Because we can’t create an object of an interface. In an abstract class, the constructor is used to initialize the variable of an abstract class. but the interface doesn’t contain any variable, It just contains the final variable.
An abstract class can have a constructor. By default, JVM provides the default constructor, you can also provide the constructor explicitly. We can’t create the object of the abstract class. The constructor can only be called during constructor chaining and used to initialize common variables, which are declared inside an abstract class.
main method
Before java 8, An interface could not have the main method in the interface. But after Java 8, you can create the main method in the interface. because the main method is always using the static keyword. In Java 8, It allows declaring static methods also.
An abstract class can have the main method. Because in abstract class we can define abstract and non-abstract methods.
When to use abstract class and interface in java
When to use abstract class and interface in java is one of the most important topics in java. You can decide “when to use interface and abstract class” if you know the difference between abstract class and interface. If you are familiar with an abstract class and interface in java you can easily find the difference between them. But it’s not easy to decide when to use abstract class and interface in Java, because it depends upon the situation or how and what you want to do in your application.
1. Common functionality
If you want to provide some common or default functionality then the abstract class would be a better choice. Because an abstract class can have abstract methods and concrete methods. So concrete method can provide some default behavior to each subclass.
On another hand, an interface can contain only abstract methods. Since java 8 we can define default methods in the interface, but the scope of methods is limited because it uses the default keyword.
We can take an example from JDK, here we have an abstract class AbstractCollection. The AbstarctCollection class provides common behavior to the subclasses like ArrayList, LinkedList, HashSet, etc.

2. To make a contract
If you want to enforce some rule or want to make a contract of implementation the interface would be a better choice. An interface provides contracts only and it enforced the rule to subclass. So, it is the responsibility of subclasses to implement each and every single contract provided to them.
But an abstract class is not a good choice when you want to enforce some rule or want to make a contract of implementation. Let’s say you have two abstract classes with different contracts, but the concrete class can extend only one class. it can’t follow both the rule at the same time.
3. To support multiple inheritance
As you know we can’t achieve multiple inheritance by the use of classes. So, when you want to perform multiple inheritance then interface would be a good choice.
We can take the example of the creation of a thread. We have two ways to create a thread, those are by Extending the thread class and Implementing the Runnable interface.
If we are creating a thread by extending of thread class, then we can‘t extend any other class. But if we create an object by implement the interface then we can extend any other class also.


4. Access modifier
As know, we can declare the variable in the abstract class with different access modifiers. An abstract class doesn’t apply any restrictions. If you want to declare non- public variables while performing abstraction, then interface is a good choice. Because in the interface by default all variables are public.
In interface you can’t any variable other than public. All variables are by default public.
5. Related class or unrelated classes
Let’s say you want to use the abstraction, but you are getting confused about whether you should use the abstract class or interface. You can decide on the basis of its use if you need to provide functionality that is used by some type of class then use the Abstract class. We can take the example of the AbstractCollection class. AbstractCollection provides the functionality which is used by same type class like ArrayList.
But if functionality can be used by completely unrelated classes than using the interface. Let’s take the example of a Cloneable interface. This interface is unrelated for each class but you can implement it and you the functionality.
6. Variable or final variable(Constant)
Suppose you have a situation in which you want to perform abstraction. You can’t tell the exact implementation of the method. You can use the abstract class or interface. But if you want to declare non-static or non-final variables then it can be possible only by the use of the abstract class.
Because in an interface we can declare only static and final variables.

7. Without breaking existing code
An abstract class is a good choice when there is a chance to add more new behavior. Because you can still add behavior without breaking the existing code.
But in an interface, all the subclass must provide the implementation to the method.
Let’s say you had developed an application one year ago. You have been using the abstract class. If you want to provide some new behavior you can add it without breaking the existing code.
Since java 8, You can use the default method in interfaces to resolve this problem.
We recommend you, If you are designing small functionality, then interfaces are a good choice because can make changes after developed the functionality. But in large applications, it can create a problem.
8. Use abstract and interface together
We can use the abstract class and interface together. An interface can provide only a contract but by use of an abstract class, we can provide skeletal of that contract. We can take an example from the Collection framework. In the Collection framework, Collection is an interface that extends the Iterable interface and AbstractCollection is an abstract class that implements Collection interface. AbstractCollection provides a skeletal implementation of the Collection interface. By use of this approach, it minimizes the effort to implement the Collection interface by concrete class.
whoah this weblog is wonderful i love studying your articles.
Stay up the great work! You know, a lot of persons are looking round for this
information, you could aid them greatly.
Wow, that’s what I was looking for, what a stuff! present here at this website,
thanks admin of this web page.
This page is so amazing!
extremely good content