As we know, A HashMap stores the data in form of key-value(Pair). Each pair is known as an entry in HashMap. HashMap stores all the value with its corresponding keys. We can get all the keys from HashMap by use of the keySet() method. This method returns all the keys as a Set. Let’s see the HashMap keySet() method.
keySet() method
This method is used to fetch the Set view of all keys. It returns all the keys from HashMap as a set. This Set worked on backed of HashMap, if any change makes in HashMap also reflects in this Set and vice versa.
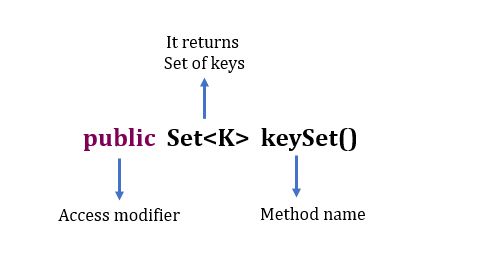
Where, K represents the type keys in HashMap.
import java.util.HashMap; public class ExampleOfHashMap { public static void main(String[] args) { HashMap<Integer, String> hashMap = new HashMap<Integer, String>(); // Adding objects in HashMap hashMap.put(1, "JavaGoal.com"); hashMap.put(2, "Learning"); hashMap.put(3, "Website"); System.out.println("Get all keys: "+ hashMap.keySet()); } }
Output: Get all keys: [1, 2, 3]
Here we have a HashMap that stores the Integer keys and String values. So we can get all the keys by use of keySet() method.
import java.util.HashMap; public class ExampleOfHashMap { public static void main(String[] args) { HashMap<String, String> hashMap = new HashMap<String, String>(); // Adding objects in HashMap hashMap.put("Hi", "JavaGoal.com"); hashMap.put("Hello", "Learning"); hashMap.put("Good", "Website"); System.out.println("Get all keys: "+ hashMap.keySet()); } }
Output: Get all keys: [Hi, Hello, Good]