In this post, we will read the most important part of java which is the java collection framework, and see all the collection in java. In the java collections tutorial, we will cover the collection hierarchy in java and collection in java with examples.
Here is the table content of the article will we will cover this topic.
1. What is the Collection framework?
2. Why collection framework introduced in java?
3. How does the Java Collections Framework work?
4. Java iterable interface?
5. Java collection interface?
6. AbstractCollection class?
7. Java List Interface in java?
8. AbstractList class in java?
9. Java Set Interface in java?
10. AbstractSet class in java?
11. Map Interface in java?
12. Entry Interface in java?
What is the java collection framework?
In java, the Collection framework provides an architecture that uses to store and manipulate the group of objects. To operate the group of objects we need to understand the Collection framework. By use of the Collection framework, a programmer can manage/operate the group of objects. The Collection framework can perform searching, sorting, insertion, manipulation, and deletion operations as you perform on data. The group of objects behaves like a single unit in the Collection framework.
A Collection framework provides some interfaces known as collection interfaces in java and some classes known as collection classes in java. We will discuss all the classes and interfaces of the java collection framework in-depth and also try different scenarios of java collections programs.

Why collection framework introduced in java?
Before moving each interface and class of collection framework we should know what is the need for a framework. Before JDK 1.2, Java supported the group of objects by using Dictionary, Properties, Stack, and Vector classes. These classes were used for manipulating and performing an operation on the group of objects. But there were some problems in classes like lacking a central, unifying theme. Each class had a different way to operate the objects. So, the programmer was facing lots of problems managing the group of objects.
To resolve these problems the collection framework was designed for data collection organization and management. The collection framework is a robust, reliable, and high-performance mechanism. It allows various collections to operate and manage. In collection has many interfaces and classes.
Each data collection implements an algorithm such as a linked list, hash set, and tree set. The algorithm uses in the framework basis of each data collection and can be inherited by the child class.
The collection framework has interfaces and classes:
- Collection Interfaces in java : The collection framework has many interfaces. The interfaces allow for the manipulation of collections that are independent of their representation details.
- Collection Classes in java: The collection framework has many classes that provide concrete implementations to the collection interfaces.
How does the Java Collections Framework work?
The collection framework, each interface, and class are based on an algorithm. Actually, the algorithm is nothing, it is just the way data is organized in the collection object. The algorithm supports the objects to perform basic operations like sorting, searching, etc.
These algorithms have methods that provide the same output in different implementations. In the collection, interfaces have some abstract methods whenever any class implements an interface then implementing a class automatically provides the functionality. Because all structure is based on a common algorithm. The collection framework divides into two components:
1. collection interfaces: The framework has a lot of interfaces that provide just the declaration of methods. We will cover each interface in a separate topic. The framework has the following interfaces:
- Iterator Interface
- Collection Interface
- Set Interface
- SortedSet Interface
- List Interface
- Queue Interface
- Deque Interface
2. Collection classes: All the interfaces of the collection are implemented by classes and these classes provide a body to the method of interfaces. We will cover each class with a separate topic. The framework has the following classes:
- AbstractCollection class
- AbstractSet class
- HashSet class
- ImmutableSet class
- CopyOnWriteArraySet class
- LinkedHashSet class
- TreeSet class
- AbstractList class
- ArrayList class
- ImmutableList class
- CopyOnWriteArrayList class
- LinkedList class
- Vector class
- Stack class
- AbstractQueue class
- PriorityQueue class
- ArrayDeque class
Note: The Map is not part of the collection framework. The Map was introduced after the version of JAVA 1.2, but the collection framework also supports Map interfaces and classes.
Java iterable interface
The java iterable interface exists in java.lang package. It is the topmost interface in the collection framework. Each sub-interface extends it directly or indirectly and each class implements it. The iterable interface has some methods we will discuss them. It uses the generic type:
public interface Iterable<T>
where T represents the type of elements returned by the iterator.
As we said the java Iterable interface is the super interface in the collection framework. It is the root interface for all the collection classes. The Collection interface extends the Iterable interface.
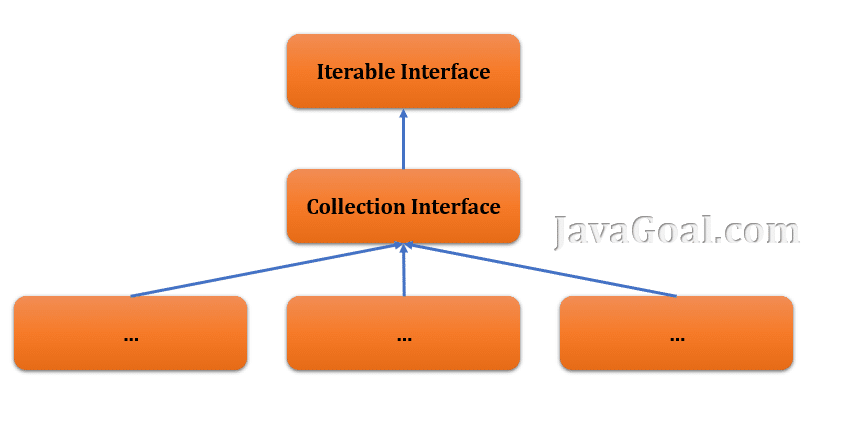
The iterable interface has three methods one abstract method and two default methods. These methods exist after java 8 (Introduced in java 8). Each interface and class inherit/override these methods and provides implementation accordingly.
java iterator method
This method uses to iterate the collection in java. In the iterable interface, it is an abstract method.
Iterator<T> iterator();
To use an iterator in your own class we need to provide its body. We can take an example from the ArrayList class, the ArrayList class provides the body to the iterator() method.
public Iterator<E> iterator() { return new Itr(); }
Earlier, there were two ways to iterate the collection first one is for(;;) loop and by use of an Iterator. The Iterator can get by invoking a collection object’s iterator() method.
import java.util.ArrayList; import java.util.Iterator; import java.util.List; public class ExampleOfForEach { public static void main(String[] args) { List<String> listOfNames = new ArrayList<>(); listOfNames.add("JAVA"); listOfNames.add("GOAL"); listOfNames.add("SITE"); System.out.println("Using for loop"); // First approch for (int i = 0; i < listOfNames.size(); i++) { System.out.println("Name = " +listOfNames.get(i)); } System.out.println("Using for Iterator"); // Second approch Iterator<String> iterator = listOfNames.iterator(); while (iterator.hasNext()) { System.out.println("Name = "+iterator.next()); } } }
Output: Using for loop
Name = JAVA
Name = GOAL
Name = SITE
Using for Iterator
Name = JAVA
Name = GOAL
Name = SITE
forEach(Consumer action) method
This method exists after java 8. It accepts the parameter of consumer type. If you are a beginner then don’t focus on it as of now. You have to read it Consumer interface in java and also need to see the details working forEach() method in java 8.
spliterator() method
It returns an object of Spliterator class. It uses to iterate the collection. We will discuss it later.
default Spliterator<T> spliterator() { return Spliterators.spliteratorUnknownSize(iterator(), 0); }
Java collection interface
The Java collection interface exists in java.lang package. It extends the Iterable interface.
public interface Collection<E> extends Iterator<T>
where E represents the type of elements in the collection.
The Collection framework builds upon the java collection interface because it declares some core methods that provide a common structure to all collection classes. It means the Collection interface is the interface that is implemented by all the classes in the collection framework.
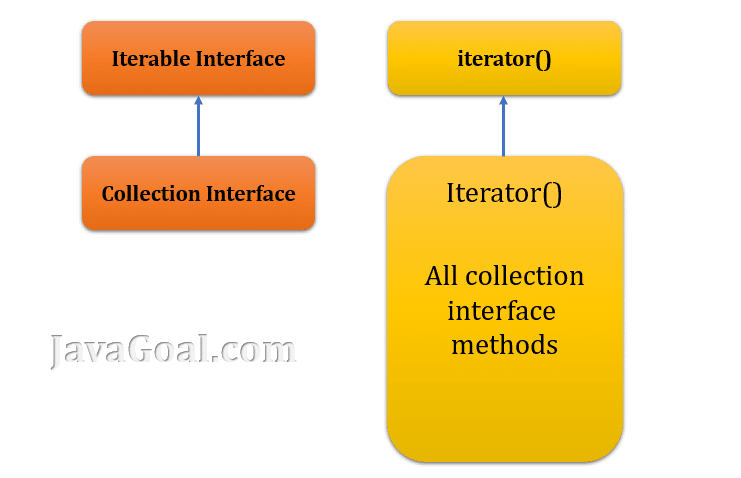
Collection interface methods
The collection interface has 14 methods and it inherits one method from the Iterable interface. All the methods of collection interface have different purposes and perform different types of operations. We categorized these methods according to their use and purpose:
- Basic operations
- Bulk operations
- Comparison operations
- Array operations
- Aggregate operations
Basic operations
The collection interface declared some methods to perform basic operations like add, remove, size, etc. These operations are very useful for the programmer in the case of collection.
- add() method: This method is used to add a new element to the collection.
- remove() method: This method is used to remove a particular element for collection.
- size() method: This method is used to get the size of the collection. It returns the count of elements present in the collection.
- isEmpty() method: This method is used to check whether the collection is empty or not. It returns true if no element present in the collection else false.
- contains() method: This method is used to check whether the given elements exist in the collection or not. It returns true if the element is present in the collection else false.
Bulk operations
It declared some methods to operate in bulk. You can perform operations on a group of objects.
- addAll() method: This method is used to add all elements of a collection to another collection.
- removeAll() method: This method is used to remove all of an element from another collection.
- containsAll() method: This method is used to check whether all elements of a collection are present in another collection.
Comparison operations
We can compare two collections by use of methods. We can use comparison operations to compare two collections for equality.
- equals() method: This method is used to compare the specified object with other objects.
- hashCode() method: This method returns the hash code value of the collection.
Array operations
It provides some methods that can convert a collection into an array. Each class that implements the collection interface must provide the implementation of the method. Here we have two methods:
- toArray() method: This method returns the array of Objects. It can convert the collection to an array.
- toArray(T[] a) method: This method returns the array of T type. It can convert the collection to a T typed array.
Aggregate Operations
By use of some method, you can perform aggregation in collections. The Collection interface supports streams that help to perform the aggregation. A stream is a sequence of elements that supports sequential and parallel aggregate operations. We can create a Stream object from a collection using the following methods from the Collection interface:
- stream() method: This method returns a stream with the collection as the source of elements. It returns a sequential Stream with this collection as its source.
- parallelStream() method: This method returns a possibly parallel Stream with this collection as its source. It is allowable for this method to return a sequential stream.
AbstractCollection class
AbstractCollection class is a part of the Collection Framework and implements the Collection interface.
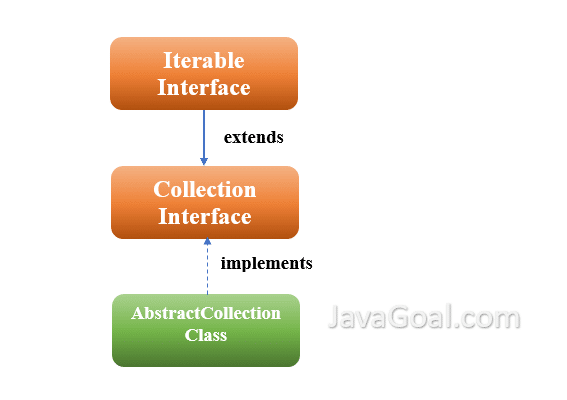
The AbstractCollection java class implements some methods of the Collection interface and provides a skeletal implementation of the Collection interface. This class uses to minimize the effort required to implement a collection interface.
public abstract class AbstractCollection<E> implements Collection<E>
where E denotes the type of element maintained by this collection.
How AbstractCollection class is useful?
1. Unmodifiable collection: Those collections that can’t be modified (like ImmutableList) are known as unmodified collections. To implement an unmodified collection the programmer needs only to extend AbstractCollection class and provide implementations for the iterator() and size() methods.
2. Modifiable collection: Those collections that can be modified (like ArrayList) are known as modified collections. To implement a modified collection the programmer must additionally override the add() method. If the programmer doesn’t override the add() method, it throws an
UnsupportedOperationException.
Constructor: The default constructor, but being protected, it doesn’t allow the creation of an AbstractCollection object.
Java List Interface
In java List interface extends the collection interface. It is one of the most important interfaces of the collection framework. Basically, the java List interface is implemented by different classes, that can store the collection of elements and each element is stored on the basis of an index. It can contain duplicate elements as well. The List interface inherits the methods from the Collection interface. The List interface has its implementation in various classes like ArrayList, LinkedList, Vector and Stack. We will discuss each class in a separate post.
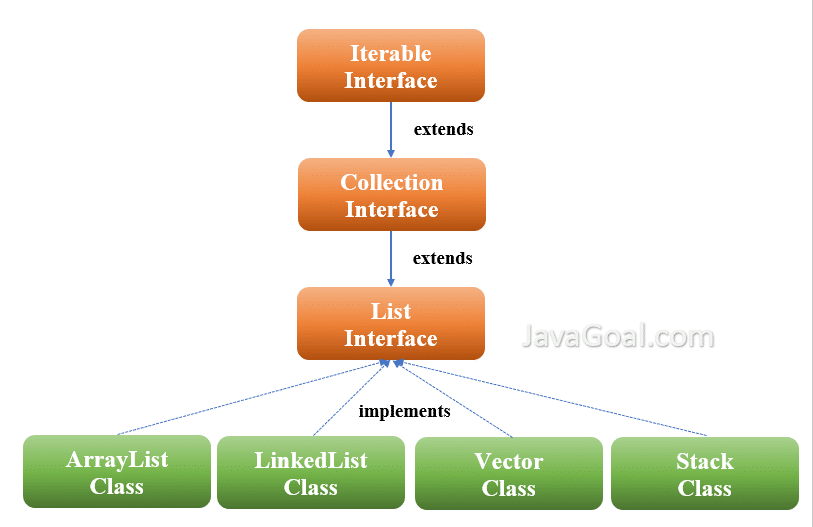
List interface properties
The List interface has some properties that use to handle the collections of objects. Whoever class implements the List interface, gets all the properties automatically.
1. Java List interface provides the functionality to add duplicate elements to the list. In the collection framework ArrayList, LinkedList, Vector, and Stack implement it, It means they all can store the duplicate elements.
2. It allows having ‘null’ elements in the Java list.
3. Since Java 8, the List interface provides some default methods.
4. We can make a list as Generic in java to avoid ClassCastException at runtime.
Important Methods
It has a lot of methods, some methods inherit from the collection interface and some are their own. We will discuss the most common and useful method of list.
1. size(): It returns the size of the list. The size of the list means how many elements are present in the list.
2. isEmpty(): To find whether the list is empty or not. If the list is empty it returns true otherwise false.
3. contains(Object o): To find whether the given Object o exists or not. It returns true if the list contains the given element.
4. iterator(): To get an iterator so that you can iterate over the elements in the list in the proper sequence.
5. toArray(): It returns an array that contains all the elements of the list in proper sequence
6. add(E e): The add() method uses to append given element e to the end of this list.
7. remove(Object o): The remove() method is uses to remove the first occurrence of the given element from the list.
8. retainAll(Collection c): This method uses to retain the elements in the list, those contained in the given collection c.
9. clear(): To remove all the elements from the list.
10. get(int index): To get the element from the specified position in the list.
11. set(int index, E element): This method uses to add the element at a specific position. If any element is already there, then it Replaces the element at the specified position.
12. listIterator(): It returns a list iterator over the elements in the list.
13. suList(int fromIndex, int toIndex): By use of this method we can get a sublist from the list. It means to get a portion of the list between the specified fromIndex, inclusive, and toIndex, exclusive.
Implementation of the List Interface
ArrayList, LinkedList, Vector, and Stack classes implement the list interface. Here we will discuss the most popular classes that are ArrayList and LinkedList.
Let’s see how ArrayList implements the List interface and use it.
public class ArrayList<E> extends AbstractList<E> implements List<E>, RandomAccess, Cloneable, java.io.Serializable
package package1; import java.util.ArrayList; import java.util.List; class ArrayListExample { public static void main(String[] args) { // Creating list using the ArrayList class List<String> names = new ArrayList<String>(); // Add elements to the list names.add("Hi"); names.add("JavaGoal"); names.add("Website"); System.out.println("List: " + names); // Access element from the list String name = names.get(1); System.out.println("Accessed Element: " + name); // Remove element from the list names.remove("Hi"); System.out.println("After Removed name: " + names); } }
Output: List: [Hi, JavaGoal, Website]
Accessed Element: JavaGoal
After Removed name: [JavaGoal, Website]
Let’s see the how LinkedList implements List interface and use it.
public class LinkedList<E> extends AbstractSequentialList<E> implements List<E>, Deque<E>, Cloneable, java.io.Serializable
package package1; import java.util.LinkedList; import java.util.List; class LinkedListExample { public static void main(String[] args) { // Creating list using the LinkedList class List<String> names = new LinkedList<String>(); // Add elements to the list names.add("Hi"); names.add("JavaGoal"); names.add("Website"); System.out.println("List: " + names); // Access element from the list String name = names.get(1); System.out.println("Accessed Element: " + name); // Remove element from the list names.remove("Hi"); System.out.println("After Removed name: " + names); } }
Output: List: [Hi, JavaGoal, Website]
Accessed Element: JavaGoal
After Removed name: [JavaGoal, Website]
AbstractList class in java
The AbstractList class is a part of the Java Collection Framework which implements the List interface and extends the AbstractCollection class.
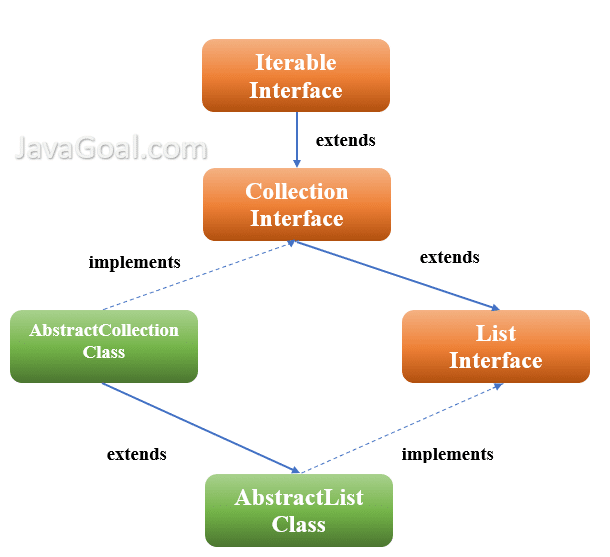
public abstract class AbstractListt<E> extends AbstractCollection<E> implements List<E>
The AbstractList class implements some methods of the List interface and provides a skeletal implementation of the List interface. This class uses to minimize the effort required to implement the List interface. This class provides a skeletal implementation for “random access” data store (such as ArrayList), and for “sequential access” data (such as a linked list).
Java Set Interface in java
In Java Set interface extends the Collection interface and provides the skeletal for the classes that implement it. The Set in Java ensures the uniqueness of its element but it doesn’t maintain the order of collection. There are some classes that implement the Set interface and hold unique elements only. The Set interface in java inherits the methods from Collection interface also. HashSet, EnumSet, LinkedHashSet, and TreeSet classes implemets the Set interface. We will discuss each class in a separate post.

Properties of Java Set interface
Set interface in java has some properties that extend by many classes and used to handle the collections of objects.
1. If any class implements the Set interface then it can’t contain duplicate elements. It means a collection of the set can contain only unique values.
2. A set doesn’t maintain the order of collection objects. We can’t find the order of elements.
3. Since Java 8, the Set interface has some default methods, those uses by derived classes through inheritance.
4. It supports generic concepts, we can make a set as class specific.
Important Methods
Let’s discuss the methods of the Set interface. There are a number of set methods but some methods inherited from the Collection interface and some are it’s own. Let’s discuss the most common and useful set methods.
1. size(): This method returns an int value. It returns the size of the set and tells how many objects presents in Set.
2. isEmpty(): This method returns a boolean value and we can find whether the Set is empty or not.
3. contains(Object o): This method returns a boolean value and uses to find whether the given Object o exists or not.
4. containsAll(Collection c): This method returns a boolean value and uses to find whether the given Collection c exists or not.
5. iterator(): This method returns an object of the Iterator. We can iterate over the objects by use of iterator.
6. toArray(): This method returns an Array of Objects. The array contains all the objects of Set.
7. add(E e): This method uses to add the given object in Set. If an object already exists it doesn’t add it.
8. addAll(Collection c): This method adds all the objects from given collection c to Set.
9. remove(Object o): The method uses to remove the given object from Set.
10. retainAll(Collection c): This method uses to retain the elements from the Set only those are common in given Collection c and Set.
11. removeAll(Collection c): This method uses to remove all objects from the Set that are common in Set and collection c. It returns a boolean value.
12. clear(): To remove all the elements from the Set.
Java set interface implementation classes in java
The HashSet, EnumSet, LinkedHashSet, and TreeSet classes implements the Set interface. We will discuss each implementation in a separate post.
Let’s see how HashSet implements the Set interface and use it.
import java.util.HashSet; import java.util.Set; public class HashSetExample { public static void main(String[] args) { Set<Integer> integerHashSet = new HashSet<Integer>(); integerHashSet.add(1); integerHashSet.add(2); integerHashSet.add(3); integerHashSet.add(4); integerHashSet.add(5); System.out.println("HashSet of Integer: "+ integerHashSet); Set<String> stringHashSet = new HashSet<String>(); stringHashSet.add("Hi"); stringHashSet.add("Java"); stringHashSet.add("Goal"); stringHashSet.add("Learning"); stringHashSet.add("Website"); System.out.println("HashSet of String: "+ stringHashSet); } }
Output: HashSet of Integer: [1, 2, 3, 4, 5]
HashSet of String: [Learning, Hi, Java, Goal, Website]
Let’s see how LinkedHashSet implements the Set interface and use it.
import java.util.LinkedHashSet; import java.util.Set; public class LinkedHashSetExample { public static void main(String[] args) { Set<Integer> integerLinkedHashSet = new LinkedHashSet<Integer>(); integerLinkedHashSet.add(1); integerLinkedHashSet.add(2); integerLinkedHashSet.add(3); integerLinkedHashSet.add(4); integerLinkedHashSet.add(5); System.out.println("LinkedHashSet of Integer: "+ integerLinkedHashSet); Set<String> stringLinkedHashSet = new LinkedHashSet<String>(); stringLinkedHashSet.add("Hi"); stringLinkedHashSet.add("Java"); stringLinkedHashSet.add("Goal"); stringLinkedHashSet.add("Learning"); stringLinkedHashSet.add("Website"); System.out.println("LinkedHashSet of String: "+ stringLinkedHashSet); } }
Output: LinkedHashSet of Integer: [1, 2, 3, 4, 5]
LinkedHashSet of String: [Hi, Java, Goal, Learning, Website]
Let’s see how TreeSet implements the Set interface and use it.
import java.util.Set; import java.util.TreeSet; public class TreeSetExample { public static void main(String[] args) { Set<Integer> integerTreeSet = new TreeSet<Integer>(); integerTreeSet.add(1); integerTreeSet.add(2); integerTreeSet.add(3); integerTreeSet.add(4); integerTreeSet.add(5); System.out.println("TreeSet of Integer: "+ integerTreeSet); Set<String> stringTreeSet = new TreeSet<String>(); stringTreeSet.add("Hi"); stringTreeSet.add("Java"); stringTreeSet.add("Goal"); stringTreeSet.add("Learning"); stringTreeSet.add("Website"); System.out.println("TreeSet of String: "+ stringTreeSet); } }
Output: TreeSet of Integer: [1, 2, 3, 4, 5]
TreeSet of String: [Goal, Hi, Java, Learning, Website]
AbstractSet class in java
AbstractSet class is part of the collection framework since JDK 1.2. If you are not familiar with the Collection framework and Set interface then you should read the first. The Abstract Set class provides a skeleton to the Set interface. In this section, we will see the use of AbstractSet class add why AbstractSet class was introduced in JDK.
AbstractSet class in java
The AbstractSet class implements the Set interface and extends the AbstractCollection class. It provides implementations to some methods of the Set interface. So that it can minimize the efforts required to implement the Set interface. Let’s see the hierarchy of Abstract Set class.
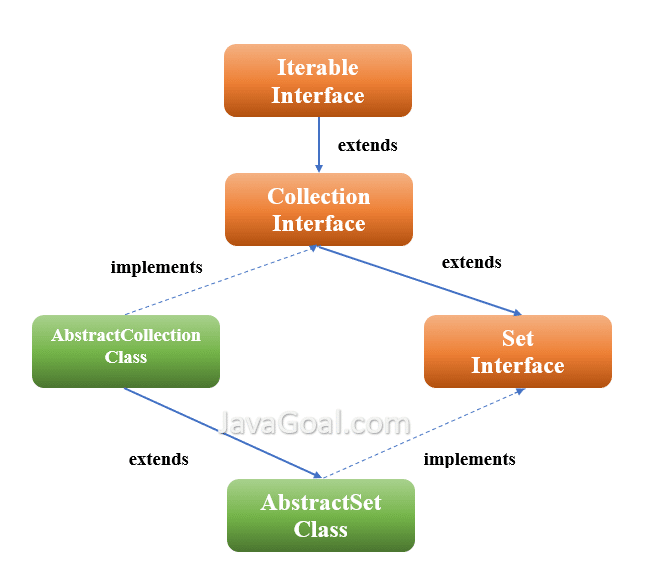
public abstract class AbstractSet<E> extends AbstractCollection<E> implements Set<E>
The AbstractSet class implements some methods of the Set interface and provides a skeletal implementation of the Set interface. It doesn’t override the functionality of the Abstract collection class. Also, It provides a body to three methods of the Set interface. In conclusion, It adds the implementation of equal(), hashCode(), and removeAll() methods.
The equals() and hashCode() method overrides and provides the implementation to check the equality of objects. If you want to know why and when we should override them. You can read it here.
equals() method in java
hashCode() method in java
equals() method
The equals method Compares the specified object with this set for equality.
Therefore, It performs three operations in the equals() method.
- It returns true if the specified object and this are the same by comparing them by ==.
- Then it compares the size of both objects. If the size is the same then move to the third step.
- Every member of the given set is contained in this set
The Set interface has different implementations like HashSet, LinkedHashSet, TreeSet. So, It ensures the equals method works properly with these implementations.
Hence, Let’s see the code of removeAll(Collection c) method.
public boolean removeAll(Collection<?> c) { Objects.requireNonNull(c); boolean modified = false; if (size() > c.size()) { for (Object e : c) modified |= remove(e); } else { for (Iterator<?> i = iterator(); i.hasNext(); ) { if (c.contains(i.next())) { i.remove(); modified = true; } } } return modified; }
Let’s see the example of AbstractSet class.
import java.util.*; public class AbstractSetClassExample { public static void main(String[] args) { // Create a HashSet AbstractSet<String> names = new HashSet<String>(); names.add("Ram"); names.add("Sham"); names.add("Krisha"); names.add("Vindhu"); names.add("Ravi"); for ( String name: names) { System.out.println("Name from HashSet: "+name); } } }
Output: Name from HashSet: Ravi
Name from HashSet: Sham
Name from HashSet: Krisha
Name from HashSet: Vindhu
Name from HashSet: Ram
Java Map Interface in java
In Java Map Interface is one of the most important topics. The map interface considers a part of the Collection framework, but it doesn’t implement the Collection interface. In this section, we will read what is Java Map Interface and how it is implemented by other classes.
The Map interface exists in java.util package and it represents the mapping of key and value. A map maintains the data in two parts, value and key. Map stores each value with a unique key that is known as an entry. A Map doesn’t allow duplicate keys, but it can have duplicate values.
We can’t traverse a Map directly; we have to convert it into
Set using keySet() or entrySet() method. The Map interface has its implementation in various classes like HashMap, LinkedHashMap, and TreeMap.

Characteristics of Map Interface
1. It doesn’t implement the Collection interface. So it’s characteristics are different from the rest of the collection types.
2. The Map interface provides different views of the collection. It provides a view that contains a set of keys, a set of key-value mappings, and a collection of values.
3. It contains a pair of keys and values. It doesn’t contain duplicate keys, but it can contain duplicate values. Each value links with a unique key.
4. A Map interface doesn’t maintain the order of elements; it depends on the implementation. For example, HashMap doesn’t guarantee the order of mappings but TreeMap does.
Java map methods
- put(Object key, Object value): This method is used to insert an entry in this map. It takes two parameters one is key, and another is value.
- putAll(Map map): This method is used to copy all elements of the specified map in the current map.
- remove(Object key): This method is used to remove an entry for the specified key.
- get(Object key): This method is used to get the value for the specified key.
- containsKey(Object key): This method is used to check whether the specified key exists or not. Its return type is boolean.
- keySet(): This method is used to get the Set view containing all the keys. It returns all the key set.
- entrySet(): This method is used to get the Set view containing all the keys and values. It returns all the keys and values set.
- size(): It is used to check the number of key-value pairs present in Map.??
- isEmpty(): This method is used to check whether the map contains any key-value pair or not. Its return type is boolean.
- containsValue(Object value): This method is used to check whether the specified value exists in the map or not. Its return type is boolean.
- clear(): This method is used to remove all elements from the Map. It doesn’t return anything, so its return type is void.
- values(): This method is used to get all values of the Map. It returns the Collection type.
Implementations of Map
Several classes implement the Map interface but three major implementations are the most popular. Here we will discuss HashMap, LinkedHashMap, and TreeMap.
HashMap Class
The most important and common implementation of the Map interface is the HashMap class. A HashMap implements all the methods of the Map interface and provides the body to the methods. A HashMap doesn’t maintain any order because all values are associated with keys. It allows only null values and one null key. Let’s see the java HashMap example.
import java.util.HashMap; import java.util.Map; class HashMapExample { public static void main(String arg[]) { Map<Integer, String> hashMap = new HashMap<Integer, String>(); hashMap.put(1, "Hi"); hashMap.put(2, "Java"); hashMap.put(3, "Goal"); hashMap.put(4, "Learning"); hashMap.put(5, "Website"); System.out.println("Get all keys: "+ hashMap.keySet()); System.out.println("Get all values: "+ hashMap.values()); System.out.println("Get all entry set: "+ hashMap.entrySet()); } }
Output: Get all keys: [1, 2, 3, 4, 5]
Get all values: [Hi, Java, Goal, Learning, Website]
Get all entry set: [1=Hi, 2=Java, 3=Goal, 4=Learning, 5=Website]
LinkedHashMap class
Like HashMap, LinkedHashMapclass is also an implementation of Map. A LinkedHashMap maintains an order of elements inserted into it. Let’s see the LinkedHashMap example.
import java.util.LinkedHashMap; import java.util.Map; class HashMapExample { public static void main(String arg[]) { Map<Integer, String> linkedHashMap = new LinkedHashMap<Integer, String>(); linkedHashMap.put(1, "Hi"); linkedHashMap.put(2, "Java"); linkedHashMap.put(3, "Goal"); linkedHashMap.put(4, "Learning"); linkedHashMap.put(5, "Website"); System.out.println("Get all keys: "+ linkedHashMap.keySet()); System.out.println("Get all values: "+ linkedHashMap.values()); System.out.println("Get all entry set: "+ linkedHashMap.entrySet()); } }
Output: Get all keys: [1, 2, 3, 4, 5]
Get all values: [Hi, Java, Goal, Learning, Website]
Get all entry set: [1=Hi, 2=Java, 3=Goal, 4=Learning, 5=Website]
TreeMap class
A TreeMap implements the Map interface like HashMap. It maintains the order of elements. It doesn’t allow the null key but we can add null as a value. Let’s see the TreeMap example.
import java.util.Map; import java.util.TreeMap; class HashMapExample { public static void main(String arg[]) { Map<Integer, String> linkedHashMap = new TreeMap<Integer, String>(); linkedHashMap.put(1, "Hi"); linkedHashMap.put(2, "Java"); linkedHashMap.put(3, "Goal"); linkedHashMap.put(4, "Learning"); linkedHashMap.put(5, "Website"); linkedHashMap.put(6, null); System.out.println("Get all keys: "+ linkedHashMap.keySet()); System.out.println("Get all values: "+ linkedHashMap.values()); System.out.println("Get all entry set: "+ linkedHashMap.entrySet()); } }
Output: Get all keys: [1, 2, 3, 4, 5, 6]
Get all values: [Hi, Java, Goal, Learning, Website, null]
Get all entry set: [1=Hi, 2=Java, 3=Goal, 4=Learning, 5=Website, 6=null]
Entry Interface in java
The Entry Interface is basically a sub-interface that exists in the Map interface. It internally maintains the key-value pair. If you are not familiar with Map ad it’s internal working then you should read it first from here.
The map interface contains the Entry interface. So, Entry is the subinterface of Map. So, we can access it by Map.Entry name. The Map.Entry interface enables you to work with a map entry. The Entry interface returns a collection view of the map. Entry has some methods to access the Map and manipulate it.
Methods of Entry Interface
1. getKey(): It returns the key from the Entry Interface entry Map.
2. getValue(): It returns the value from the entry Map.
3. setValue(V value): This method uses to set the value in Map. It will throw
ClassCastException, if the value doesn’t acceptable by map.
NullPointerException, null doesn’t support by map.
UnsupportedOperationException, if manipulation of the map doesn’t support by the map.
IllegalArgumetException, if there is some problem with the argument.
IllegalStateException, if the entry doesn’t exist in the map.
4. equal(): The equals() method declares in the Object class and It compares the specified object o with the entry for equality.
5. hashCode(): The hashCode() method declared in Object class. It returns the hash code value for the map entry.
import java.util.HashMap; import java.util.LinkedHashMap; import java.util.Map; import java.util.Set; public class MapInterfaceExample { public static void main(String[] args) { // Create a LinkedHashMap HashMap<String,Integer> hashMapOfNames = new HashMap<String, Integer>(); hashMapOfNames.put("Ram" , 25000); hashMapOfNames.put("Sham" , 50000); hashMapOfNames.put("Krishna" , 75000); hashMapOfNames.put("Ravi", 65000); // Let's use the entrySet() to get the entry's of the map Set<Map.Entry<String,Integer>> entryMapOfNames = hashMapOfNames.entrySet(); for (Map.Entry<String, Integer> name: entryMapOfNames) { System.out.println("Key and Value = " + name.getKey() + " " + name.getValue()); } } }
Output: Key and Value = Krishna 75000
Key and Value = Ravi 65000
Key and Value = Sham 50000
Key and Value = Ram 25000
Good work and will explained
Thankyou Ibra
Collection interface extends ‘Iterable’ and not `Iterator`.
Thanks… It was a mistake 🙂