In Java, Comparable and Comparator interfaces are useful for sorting the collection of objects. Java has some methods that are useful for sorting primitive types array or Wrapper classes array or list. So, first of all, we will see how we can sort our Primitive data type array and wrapper class array:
import java.util.ArrayList; import java.util.Arrays; import java.util.Collections; import java.util.List; public class SortingOfDifferentObjects { public static void main(String[] args) { // int array i.e Primitive data types int[] intArray = {2,9,11,1}; // String array i.e Wrapper class String stringArray[] = {"BB", "DD", "RR", "LL"}; // Integer array i.e Wrapper class Integer integerArray[] = {3,8,10,2}; // ArrayList containing wrapper class String List<String> listOfString = new ArrayList<String>(); listOfString.add("A"); listOfString.add("E"); listOfString.add("L"); listOfString.add("B"); // Sorting of int array i.e Primitive data types Arrays.sort(intArray); System.out.println(Arrays.toString(intArray)); // Sorting of String array i.e Wrapper class Arrays.sort(stringArray); System.out.println(Arrays.toString(stringArray)); // Sorting of Integer array i.e Wrapper class Arrays.sort(integerArray); System.out.println(Arrays.toString(integerArray)); // Sorting of ArrayList containing wrapper class String Collections.sort(listOfString); System.out.println(listOfString.toString()); } }
Output: [1, 2, 9, 11]
[BB, DD, LL, RR]
[2, 3, 8, 10]
[A, B, E, L]
In the above example, you can see how we are sorting the Array of different types. To provide the Sorting functionality for Custom classes Java provides two interfaces
Java.lang.Comparable and java.util.Comparator
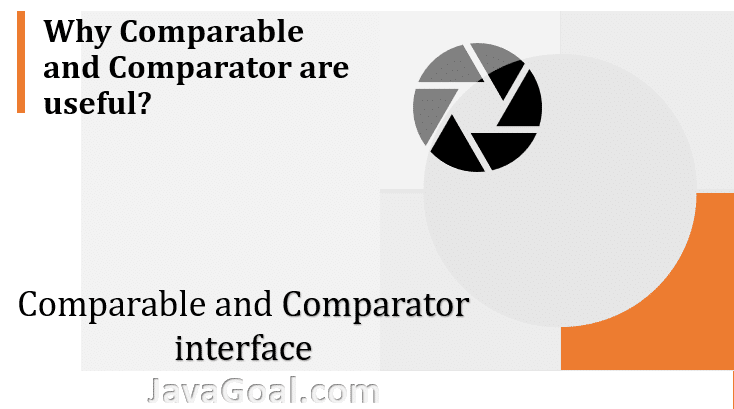
Let’s take an example, suppose you are maintaining the data of the College. There is the number of Students and you want to collect all the data in ascending order of rollNo. So now you need to Sort them. But you can achieve this task by using a comparable and comparator interface. You can see the Sorting of custom classes in detail.