In this post we see comparator vs comparable a d when we should you them. How Comparator useful over comparable, Let’s discuss them with different examples.
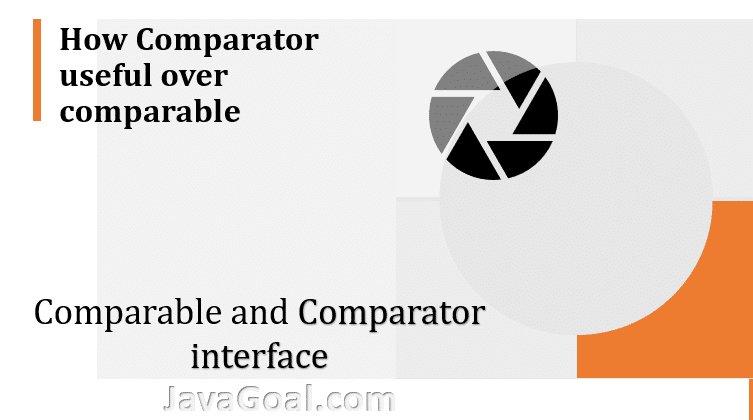
comparator vs comparable
Changes in Main Class: As you know Comparable must be implement it in your class for which you want to perform Sorting. So, you need to change your class.
public class Student implements Comparable { // Body of Class }
For more details of example please click here.
But to use Comparator interface you do not need to change the original class.You can create a separate class for sorting. It provides ease to programmer.For more details of example please click here.
Single Vs Multiple Sorting: If you have only single sorting criteria then you should go with Comparable. Here mean of single sort is, you want to Sort your data only base of single data member. Let’s say you want to sort Student data based on roll number only. For more details of example please click here.
But if you have more than one sorting criteria then you should go with Comparator. Here mean of multiple sort is, you want to Sort your data base of multiple data member. Let’s say you want to sort Student data based on roll number, Age, rank etc. So, you can perform multiple Sorting based on requirements. For more details of example please click here .
Flexibility: As you know Comparable implemented only in main class. So, you can’t add more criteria later. If want to add any criteria later then you have made changes in existing class.
But by use of you can add sorting criteria later easily because you do not need to modify the existing ones. You have to make another class for new criteria. So, Comparator provide the flexibility.
User choice: By use of Comparable you can sort elements to their natural order.
But by use of Comparator you can sort the order in different from natural order. Here is example how you can change order.
import java.util.ArrayList; import java.util.Collections; import java.util.Comparator; class Student { int rollNo; String name; int age; Student(int rollno,String name,int age) { this.rollNo=rollno; this.name=name; this.age=age; } } class RollNoComparator implements Comparator<Student> { @Override public int compare(Student student1, Student student2) { if(student1.rollNo==student2.rollNo) return 0; else if(student1.rollNo>student2.rollNo) return 1; else return -1; } } public class ExampleOfComparable { public static void main(String args[]) { ArrayList<Student> listOfStudent = new ArrayList<Student>(); listOfStudent.add(new Student(1,"Ravi",26)); listOfStudent.add(new Student(2,"kant",27)); listOfStudent.add(new Student(3,"kamboj",20)); // It Sorts all the objects based on RollNo Collections.sort(listOfStudent, new RollNoComparator().reversed()); for(Student student:listOfStudent) { System.out.println("RollNo of Student = "+student.rollNo); System.out.println("Age of Student = "+student.age); System.out.println("Name of Student = "+student.name); } } }
Output: RollNo of Student = 3
Age of Student = 20
Name of Student = kamboj
RollNo of Student = 2
Age of Student = 27
Name of Student = kant
RollNo of Student = 1
Age of Student = 26
Name of Student = Ravi
Since Comparator is a functional interface with only one method compare(). We can also use lambda expression instead of creating a class and implementing Comparator.
public void
sortingWithLamba () {
List humans = Lists.newArrayList(
new Human(“Sarah”, 10),
new Human(“Jack”, 12)
);
//We can do this
humans.sort((h1, h2) -> h1.getName().compareTo(h2.getName()));
//Instead of this
class HumanComparator implements Comparator {
public int compare(Human h1,Human h2){
h1.getName().compareTo(h2.getName());
}
Thankyou satyam 🙂