Every programming language has a loop structure. A programming language is incomplete without the loop structure and it is most common and widely used in code. The basic concept of language starts from the loop. So in this article, we will see loops in java.
- What is the loop in java?
- What are the types of Loops in java?
- What is for loop in java?
- What is nested for loop in java?
- Print the right triangle
- Program to print the downward right triangle
- How to print left triangle by use of for loop
- Print: Pyramid pattern by the use of for loop
- Print reverse Pyramid pattern by the use of for loop
- Print: Diamond pattern by the use of for loop
- What is the while loop in java?
- What is the do while loop in java?
- Performance comparison of for loop vs while loop in java?
What is the loop in java?
Loops in java are used to execute the block of statement repeatedly until the condition is true. Using the loop, we can execute the code several times or until it satisfies the condition.
Loops are very useful when a programmer wants to execute a statement or block of statements multiple times. In this article, we will discuss all the Loops in Java. Basically, loops are part of the Control structure of Java. By the use of loops in java we can control the execution of code and decide how many times code should be executed.
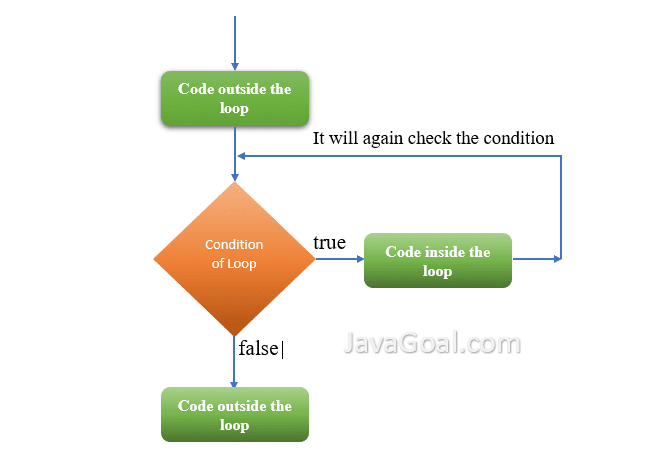
What are the types of Loops in java?
for loop in java
The for loop in java is a part of Control statements. If a user wants to execute the block of code several times. It means the number of iterations is fixed. Then you should use the for loop java. JAVA provides a concise way of writing the loop structure. It consists of four parts:
1. Initialization
2. Condition
3. Body of for loop
4. Increment/decrement
for(initialization; condition; increment/decrement) { // Body of for loop }
1. Initialization: Initialization is the first part of the loop and it happens only one time. It marks the starting point of the loop. Here, you can declare and initialize the variable, or you can use any already initialized variable.
2. Condition: Condition is the second part of the loop and we must provide/write a condition that must return a boolean value either true or false. It is executed each time to test the condition of the loop. It continues execution until the condition is false.
3. Body of for loop: It contains a block of code that executes each time after evaluating the condition true.
4. Increment/decrement: It is used to update the index value of the variable.
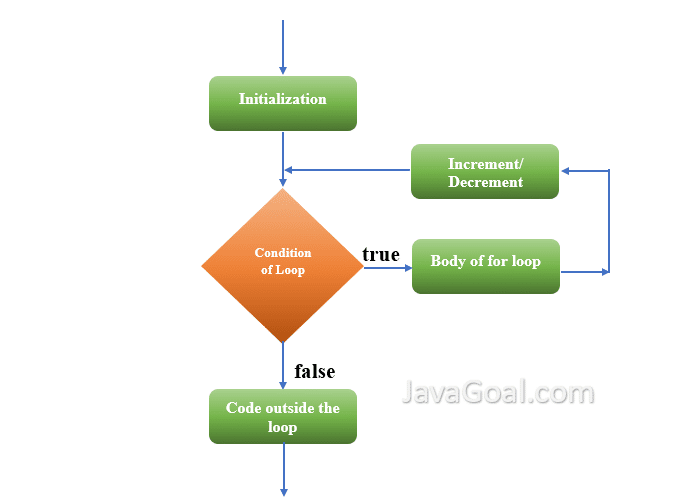
public class MyExample { public static void main(String[] args) { for(int i = 1; i <= 10 ; i++) { System.out.println(i); } } }
Explanation of the above example
In this example, we want to print the table of 1 so we used the for loop. Here, we declared a variable:
int i = 1;
1. Initialization: It is the initialization part of the loop. It is the starting point of the loop to print the count, so we initialized it with 1.
i <= 10;
2. Condition: It is the condition of for loop which will be tested/evaluated each time before execution of statements of body.
System.out.println(i);
3. Body of for loop: It is the body of the loop which will execute only if the condition of the loop returns true.
i++;
4. Increment/decrement: It is an increment/decrement part in a loop. In this example, we used the unary operator to change the index value of the variable. It will increment the value of “i” each time.
NOTE: After incrementing the value of “i” the compiler again test the condition of for loop.

Video Available Hindi – Java Programming Goal
Nested for loop in java
We can use one for loop inside the other for loop and this is called a nested for loop. The JVM can enter in the inner for loop only if the condition Outer for loop is true. otherwise, it will skip the inner for loop. The nested for loop is used to work with two dimensions. The outer loop is used for Row and the inner loop is used for the Column. The inner loop restarted each time when the outer loop changes the iteration. We will see its step-by-step execution.
for(initialization; condition; increment/decrement) { // Body of outer loop for(initialization; condition; increment/decrement) { // Body of inner loop } }
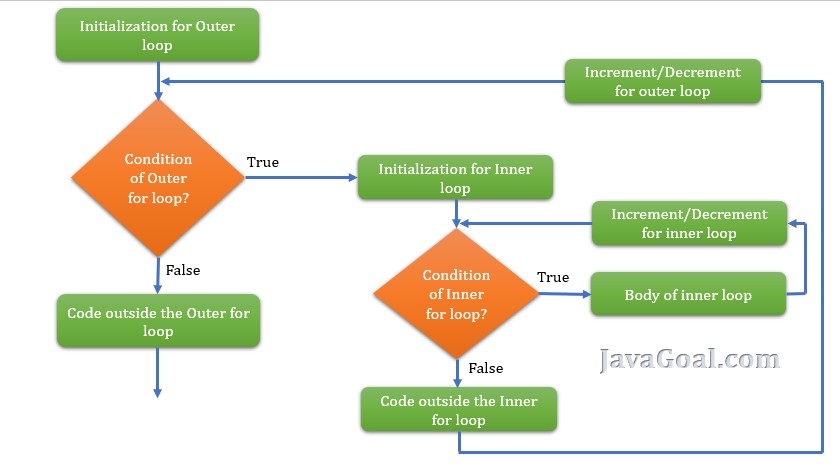
Explanation of nested for loop
Step 1: First of all, the compiler initializes the variable for the outer loop, and then it checks the condition of the outer loop.
- If the given condition returns True, then the compiler enters inside the outer for loop. Now Go to, Step 2.
- If the given condition returns False, then the compiler doesn’t enter inside the outer for loop and exit from the Outer for Loop.
Step 2: After entering the outer for loop, the compiler starts the execution of the statement. When it finds the inner for loop then it checks the condition of the inner for loop.
- If the given condition of the inner for loop returns True, then the compiler enters inside the inner for loop and executes the statement.
- If the given condition returns False, then the compiler doesn’t enter the inner loop and exit from the inner For Loop.
Step 3: After exit from the inner for loop, the compiler executes the rest of the statements that are outside the inner for loop but part of the outer for loop.
Let’s take a simple example of nested for loop and see how it works. In the first example, we will try to print the iterations of the outer loop and inner loop. Let’s see the nested loop example
public class MyExample { public static void main(String[] args) { // Outer for loop for(int i = 1; i <= 3 ; i++) { // Inner for loop for(int j = 1; j <= 3 ; j++) { System.out.println("Iteration of outer loop i: "+ i +" Iteration of inner loop: "+ j ); } } } }
Output: Iteration of outer loop i: 1 Iteration of inner loop: 1
Iteration of outer loop i: 1 Iteration of inner loop: 2
Iteration of outer loop i: 1 Iteration of inner loop: 3
Iteration of outer loop i: 2 Iteration of inner loop: 1
Iteration of outer loop i: 2 Iteration of inner loop: 2
Iteration of outer loop i: 2 Iteration of inner loop: 3
Iteration of outer loop i: 3 Iteration of inner loop: 1
Iteration of outer loop i: 3 Iteration of inner loop: 2
Iteration of outer loop i: 3 Iteration of inner loop: 3
Explanation of for loop example
In this example, We are using the nested for-loop concept because we have two for-loops, one is an outer loop and another is an inner loop.
The Outer for loop runs 1 time and performs 3 iterations but the inner for loop runs 3 times and performs 9 iterations. The inner for loops restarted its execution when the outer for loop increment itself.
Infinite for loop: In an infinite for loop the condition always returns true. Sometimes we write the condition that makes a for loop infinite.
public class ExampleOfInfiniteForLoop { public static void main(String[] args) { for( int i = 0; i >= 0 ; i++) { System.out.println(i); } } }
You can see the importance of Boolean expression and increment/decrement operation coordination. The initialization part set the value of variable i = 0, the Boolean expression always returns true because i is incrementing by 1. This would eventually lead to the infinite loop condition.
for( ; ; ) { // Body of for loop }
Write a Program to print the right triangle by use of for loop
import java.util.Scanner; public class RightTriangleByForLoop { public static void main(String arg[]) { System.out.print("Enter number of rows:"); Scanner scanner = new Scanner(System.in); int rows = scanner.nextInt(); // Outer loop for moving row for(int i = 1; i <= rows; i++) { // Inner loop for moving column for(int j = 1; j <= i; j++) System.out.print("* "); System.out.println(""); } } }
Output: Enter number of rows:5
*
* *
* * *
* * * *
* * * * *
Print the downward right triangle by for loop
import java.util.Scanner; public class DownwardRightTriangleByForLoop { public static void main(String arg[]) { System.out.print("Enter number of rows:"); Scanner scanner = new Scanner(System.in); int rows = scanner.nextInt(); // Outer loop for moving row for (int i = rows; i >= 1 ; i--) { // Inner loop for moving column for (int j = 1; j <= i; j++) { //prints star and space System.out.print("* "); } //Moving the cursor in the next line after printing each line System.out.println(); } } }
Output: Enter number of rows: 5
* * * * *
* * * *
* * *
* *
*
How to print left triangle by use of for loop
import java.util.Scanner; public class LeftTriangleByForLoop { public static void main(String arg[]) { System.out.print("Enter number of rows:"); Scanner scanner = new Scanner(System.in); int rows = scanner.nextInt(); int j; // Outer loop for moving row for (int i = rows; i >= 1 ; i--) { // Inner loop for moving column for (j = 1; j <= i-1; j++) { //prints star and space System.out.print(" "); } for ( ; j <= rows; j++) { System.out.print("* "); } //Moving the cursor in the next line after printing each line System.out.println(); } } }
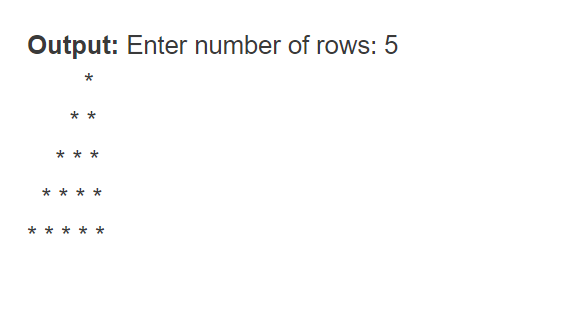
Printing Pyramid pattern by the use of for loop
import java.util.Scanner; public class PyramidPattern { public static void main(String arg[]) { System.out.print("Enter number of rows:"); Scanner scanner = new Scanner(System.in); int rows = scanner.nextInt(); //Outer loop work for rows for (int i = 1; i <= rows; i++) { //inner loop creating space for (int j = rows-i; j >= 1; j--) { //prints space between two stars System.out.print(" "); } //inner loop for columns for (int j = 1; j <= i; j++ ) { //prints star System.out.print("* "); } //Moving the cursor in a new line after printing each line System.out.println(); } } }
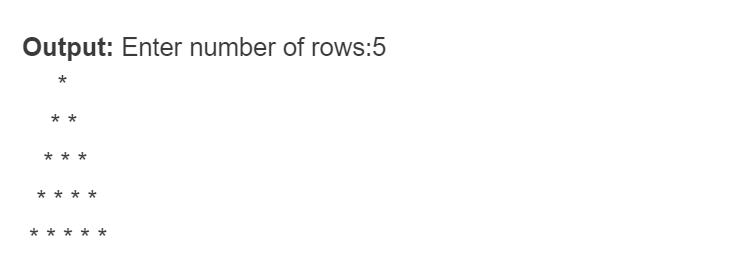
Print reverse Pyramid pattern by the use of for loop
import java.util.Scanner; public class ReversePyramidPatten { public static void main(String arg[]) { System.out.print("Enter number of rows:"); Scanner scanner = new Scanner(System.in); int rows = scanner.nextInt(); for (int i = 1; i <= rows; i++) { for (int j = 1; j <= i; j++) { System.out.print(" "); } for (int k = 1; k <= rows-i; k++) { System.out.print("* "); } System.out.println(); } } }
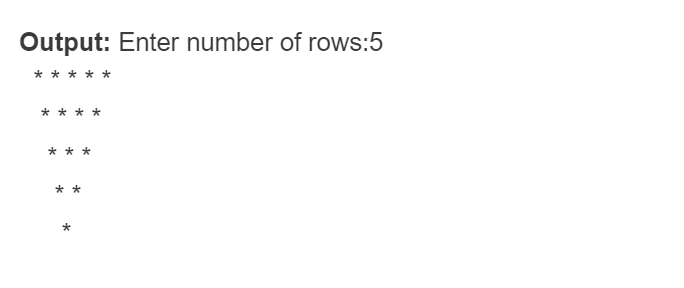
Print Diamond pattern by the use of for loop
mport java.util.Scanner; public class DiamondPattern { public static void main(String arg[]) { System.out.print("Enter number of rows:"); Scanner scanner = new Scanner(System.in); int rows = scanner.nextInt(); //Outer loop work for rows for (int i = 0; i < rows; i++) { //inner loop creating space for (int j = rows-i; j > 1; j--) { //prints space between two stars System.out.print(" "); } //inner loop for columns for (int j = 0; j <= i; j++ ) { //prints star System.out.print("* "); } //Moving the cursor in a new line after printing each line System.out.println(); } for (int i = 1; i <= rows; i++) { for (int j = 1; j <= i; j++) { System.out.print(" "); } for (int k = 1; k <= rows-i; k++) { System.out.print("* "); } System.out.println(); } } }
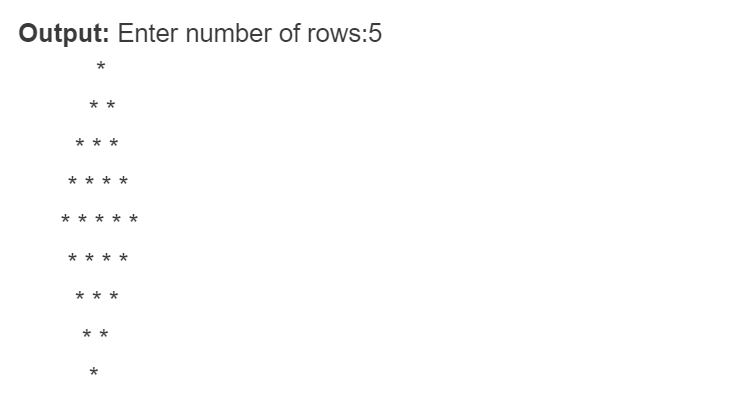
while loop in java
We will read the while loop in java and java while loop example. As you can see the name of the loop already says, the code will be executed repeatedly while the condition is true.
It is a part of Control statements. A while loop allows code to be executed repeatedly until a condition is satisfied. If the number of iterations is not fixed, it is recommended to use a while loop. It is also known as an entry control loop. In this article, we will discuss how we can use while loop java.
while(condition) { // Body of while loop }
Firstly, the condition will be evaluated:
- If it returns true, then the body of the while loop will be executed. In the body of the while loop, the statements contain an update value for the variable being processed for the next iteration.
- If it returns false, then the loop terminates which marks the end of its life cycle.
Youtube video available in the Hindi language
Flowchart of while loop Java
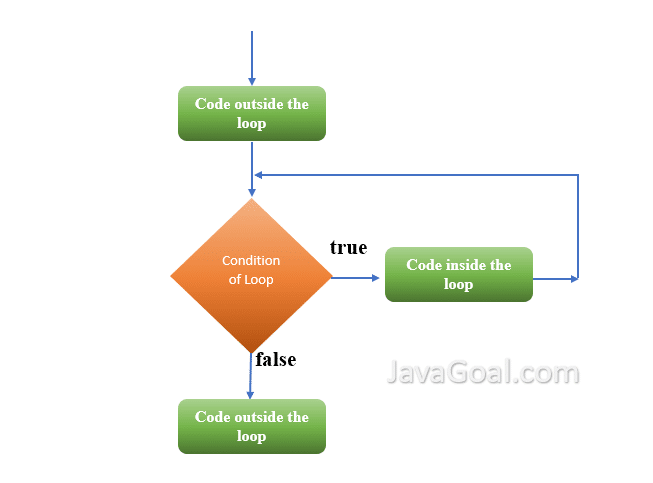
public class ExampleOfwhileLoop { public static void main(String[] args) { int i = 1; while(i <= 10) { System.out.print(i + " "); i++; } } }
Output: 1 2 3 4 5 6 7 8 9 10
Java Infinitive while Loop
If the condition of the while loop will never false. Then it’s an infinite while loop. Here, below is an example of the infinite while loop.
public class ExampleOfInfinitewhileLoop { public static void main(String[] args) { int i = 1; while(i > 0) { System.out.println(i); i++; } } }
It is an infinite loop. Because this is because the condition is i > 0 which would always be true as we are incrementing the value of i inside the while loop.
public class ExampleOfInfinitewhileLoop { public static void main(String[] args) { while(true) { System.out.println(i); } } }
If we pass true in the while loop condition. Then it will be an infinite loop.
do while loop in Java
Now we will discuss the do while loop in Java, the do while java example, and also see the syntax of the do while loop in java. Most of us already know about the do while loop, here we will see the execution of the example of the do while loop in java.
It is like a while loop, It also allows code to be executed repeatedly until a condition is satisfied. The only difference is that it checks for the condition after the execution of the body of the loop. It is also known as Exit Control Loop. Here we will discuss how to use the do while loop in Java.
do { // Body of loop } While(condition);
Youtube video available in the Hindi language
Working of do while loop in Java?
First, the statements inside the loop execute and then the condition gets evaluated.
If the condition returns true then the control gets transferred to the “do” and it again executes the statement that is presented in the loop body.
If the condition returns false then the control flow directly jumps out of the loop and it executes the following statement after the loop.
NOTE: The do-while loop executes the code at least one time because it checks the condition after the execution of the body.
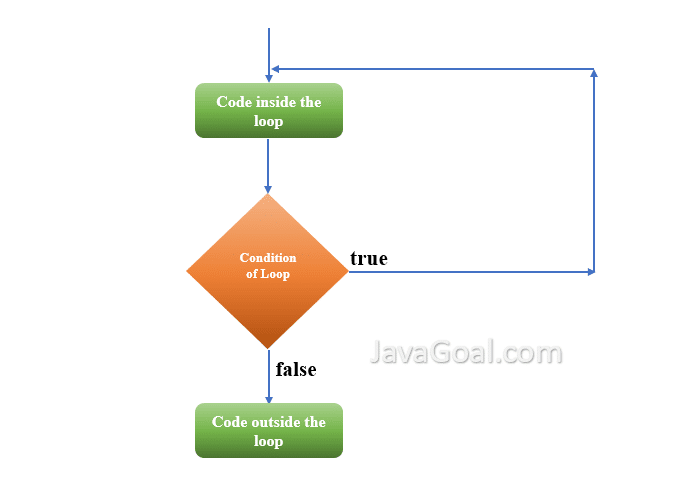
class ExampleDoWhileLoop { public static void main(String args[]) { int i=1; do { System.out.println(i + " "); i++; }while(i <=10); } }
Output:1 2 3 4 5 6 7 8 9 10
Infinitive do while in java
If the condition of the loop will never be false. Then it’s infinite do while loop.
public class ExampleOfInfiniteDOWhileLoop { public static void main(String[] args) { int i = 0; do { System.out.println(i); i++; } while(i > 0); } }
It is an infinite loop. Because this is because the condition is i > 0 which would always be true as we are incrementing the value of i inside body of the loop.
Another example:
public class ExampleOfInfiniteDoWhileLoop { public static void main(String[] args) { int i = 0; do { System.out.println(i); i++; } while(true); } }
Performance comparison of for loop vs while loop in java
In Java, the for loops and while loops are both used for iterating over a collection or repeating a block of code until a certain condition is met. Generally, the performance of for loops and while loops in Java is very similar, and the choice between the two is often a matter of personal preference or the specific requirements of the program.
That being said, there are a few situations where a for loop might be faster than a while loop in Java:
Iterating over an array: When iterating over an array in Java, a for loop can be faster than a while loop because it is designed for this specific purpose. The loop counter is typically initialized before the loop begins and incremented at each iteration, which can be more efficient than manually initializing and incrementing a loop counter with a while loop.
public class ForVsWhileExample { public static void main(String[] args) { int[] arr = {1, 2, 3, 4, 5, 6, 7, 8, 9, 10}; // Using a for loop to iterate over the array long startTimeFor = System.nanoTime(); // Get start time for (int i = 0; i < arr.length; i++) { System.out.print(arr[i] + " "); } long endTimeFor = System.nanoTime(); // Get end time long durationFor = endTimeFor - startTimeFor; // Calculate duration System.out.println(); // Using a while loop to iterate over the array int i = 0; // Initialize loop counter long startTimeWhile = System.nanoTime(); // Get start time while (i < arr.length) { System.out.print(arr[i] + " "); i++; // Increment loop counter } long endTimeWhile = System.nanoTime(); // Get end time long durationWhile = endTimeWhile - startTimeWhile; // Calculate duration System.out.println(); System.out.println("For loop duration: " + durationFor + " nanoseconds"); System.out.println("While loop duration: " + durationWhile + " nanoseconds"); if (durationFor < durationWhile) { System.out.println("For loop was faster."); } else if (durationWhile < durationFor) { System.out.println("While loop was faster."); } else { System.out.println("Both loops took the same amount of time."); } } }
Output:
1 2 3 4 5 6 7 8 9 10
NOTE: Output can be different
1 2 3 4 5 6 7 8 9 10
For loop duration: 275000 nanoseconds
While loop duration: 6978100nanoseconds
for loop was faster
Using a local variable: When using a local variable in a loop condition, a for loop can be faster than a while loop because the local variable is only in scope for the duration of the loop. With a while loop, the local variable must be declared and initialized outside of the loop and is in scope for the entire method.
// Using a for loop with a local variable for (int i = 0, max = arr.length; i < max; i++) { System.out.println(arr[i]); } // Using a while loop with a local variable int i = 0; int max = arr.length; while (i < max) { System.out.println(arr[i]); i++; }
In this case, the for loop is likely to be slightly faster because the local variable max
is only in scope for the duration of the loop.
It’s worth noting that the performance difference between for loops and while loops in Java is likely to be very small in most cases, and the choice between the two should be based on the specific requirements of the program and the readability of the code.