Here is the table content of the article will we will cover this topic.
1. What is User-defined exception or Custom Exception in Java?
2. What are the rules to create?
3. What is the need of custom exceptions?
What is User-defined exception or Custom Exception in Java?
We have seen what are the exception in java and how to handle the exception. Java allows to user to custom/user-defined exception. To create custom exceptions, you have to your own exception class and throws that exception using the ‘throw’ keyword.
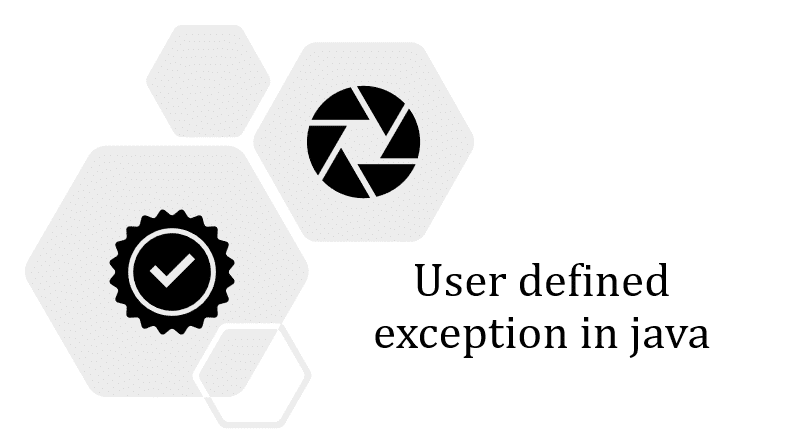
What are the rules to create?
- Naming Convention: All the exception classes provided by the JDK end with Exception. So, your custom exception should follow naming convention.
- Extends Exception class: If you are creating custom exception class then you must extend the Exception class.
- Constructor: Its not mandatory to create any constructor in custom exception class. But its good habit to provide a parameterized constructor in custom exception class. Because you can pass the essential information that you will need to analyze a production incident.
Class <CustomExceptionClass> extends Exception { // Body of class }
class LowBalanceException extends Exception { // creating a constructor to print the message LowBalanceException(String messageOfException) { super(messageOfException); // message passing to super class which is Exception } } class CashWithDraw { static void validateAmount(int amount) { if(amount < 1000) { try { throw new LowBalanceException("You can't withdraw your amount if amount is less than 1000"); } catch (LowBalanceException e) { e.printStackTrace(); } } else System.out.println("welcome to vote"); } public static void main(String args[]) { validateAmount(545); System.out.println("Thanks for using the ATM"); } }
Output: LowBalanceException: You can’t withdraw your amount if amount is less than 1000 at CashWithDraw.validateAmount(CashWithDraw.java:18 at CashWithDraw.main(CashWithDraw.java:31) Thanks for using the ATM
In this example, we are creating a constructor in LowBalanceException class and that constructor taking a string parameter which will be message of exception. The string is passed to parent class Exception’s constructor using super().
It’s not mandatory to create a constructor in Exception class because it all depends upon the programmer whether he/she wants to provide a message or not.
What is the need of custom exceptions?
In Java, almost all general exceptions are provided that are happen in programming. But someone user wants to create some own standards or rule, to provide this functionality custom exceptions were introduced. For e.g., If you want to create a class for any business and you want to provide some set of rules. If user requirements don’t match with rules than throw the custom exception.