Java optional class was introduced in java 8 to avoid the NullPointerException exception. As we know, every developer faces the NullPointerException in daily coding. Before java 8, developers place the null checks on every place, but since Java 8, providing the optional class to remove it. Java 9 introduced some new methods to Optional Class Improvements the optional class. In this post, we will see what is the Optional Class Improvements. Before moving forward, if you are not familiar with the Optional class read it first.
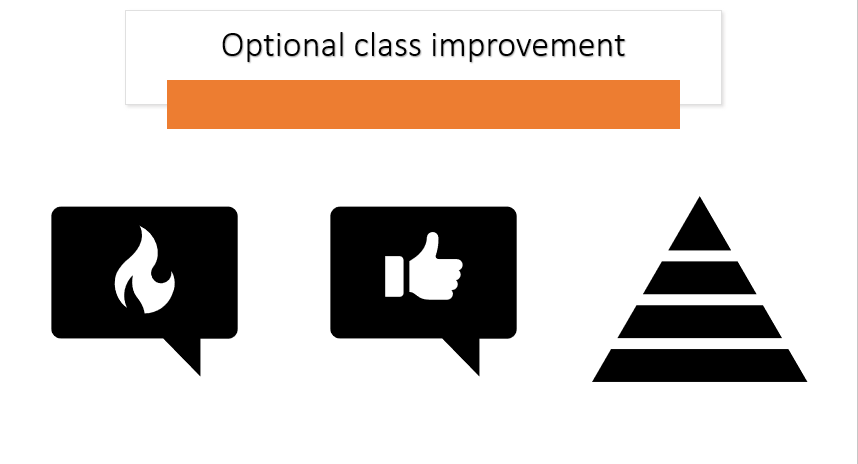
Java 9 introduced three new methods in optional class . Let’s discuss all the methods one by one.
- ifPresentOrElse()
- stream()
- or()
1. ifPresentOrElse() method
Before moving the working of ifPresentOrElse() method we should know why java 9 introduced it. To understand the concept, we will discuss it with a java 8 optional class example. We will use isPresent() method to check whether the value is present or not.
import java.util.Optional; public class Java8OptionalExample { public static void main(String arg[]) { Optional<String> obj = Optional.ofNullable("JavaGoal.com"); if(obj.isPresent()) System.out.println("Value is presented: "+obj.get()); else System.out.println("Value is not presented"); } }
Output: Value is presented: JavaGoal.com
We can write it in a more readable form by the use of consumers. We have another ifPresent(Consumer) method that accepts the parameter of consumer type. It means we can define the action as a consumer but the ifPresented() method does work only if optional has value otherwise consumer action will be skipped. But we can’t write the else logic.
import java.util.Optional; public class Java8OptionalExample { public static void main(String arg[]) { Optional<String> obj = Optional.ofNullable("JavaGoal.com"); obj.ifPresent(value -> System.out.println("Hello " + value)); } }
Output: Hello JavaGoal.com
Let’s see the how does ifPresentOrElse() method resolve the problem.
This method accepts two parameters one is type Consumer and another is Runnable. This method performs an action if an Optional value is present, or performs a different action if the value is absent.
import java.util.Optional; public class Java8OptionalExample { public static void main(String arg[]) { Optional<String> obj = Optional.ofNullable("JavaGoal.com"); obj.ifPresentOrElse(value -> System.out.println("Presented Value: " + value), () -> System.out.println("Not Presented")); } }
Output: Hello JavaGoal.com
2. stream() method
This method is used to get the stream from the optional. You can transfer the optional value to stream. If the value is present in optional then it returns a stream that contains a value. But if a value is not present then it returns an empty stream.
But a question comes to mind, how it can be helpful because streams are useful to manipulate collections of data. But here optional contains one only value that is converted in the stream.
The answer is here when you have a collection of optional objects and you want to filter the optional objects. Suppose you want to retain only those optional objects, that have some value.
import java.util.List; import java.util.Optional; public class Java8OptionalExample { public static void main(String arg[]) { List<Optional<String>> data = List.of(Optional.of("ABC"), Optional.empty(), Optional.of("DEF"), Optional.of("GHI"), Optional.empty()); data.stream().flatMap(Optional::stream).forEach(System.out::println); } }
Output: ABC DEF GHI
3. or() method
Java 9 introduced or() method that takes a supplier as an argument. This method is used to get the object optional that contains a value. If the value is present in optional then it returns it otherwise an optional produced by the provided supplier function is returned.
You may be thinking Optional class already has two methods orElse()
and orElseGet()
to return a default value when an Optional is empty. Then why is java 9 introduced or() method?
import java.util.Optional; public class Java8OptionalExample { public static void main(String arg[]) { Optional<String> dataWithValue = Optional.of("JavaGoal"); String str = dataWithValue.orElse("Website"); Optional<String> dataEmptyValue = Optional.ofNullable(null); String str1 = dataEmptyValue.orElseGet(() -> "Website"); System.out.println("String str1: "+str); System.out.println("String str2: "+str1); } }
Output: String str1: JavaGoal
String str2: Website
The orElse()
and orElseGet()
method returns a default value when the Optional is empty or returns the value inside the Optional if the optional is not empty. But suppose, you want the final returned value to be an Optional instead of a simple type then or() method is useful.
import java.util.Optional; public class Java8OptionalExample { public static void main(String arg[]) { Optional<String> dataWithValue = Optional.of("JavaGoal"); Optional<String> optionalStr = dataWithValue.or(() -> Optional.of("Website")); Optional<String> dataEmptyValue = Optional.ofNullable(null); Optional<String> optionalStr1 = dataEmptyValue.or(() -> Optional.of("Website")); System.out.println("String str1: "+optionalStr); System.out.println("String str2: "+optionalStr1); } }
Output: String str1: Optional[JavaGoal]
String str2: Optional[Website]
I really like your writing style, great info , thanks for posting : D. Letta Trev Cornish
Thank you ever so for you article. Really thank you! Really Great. Dyanna Baudoin Duwalt
Having read this I believed it was really informative. I appreciate you taking the time and effort to put this article together. I once again find myself spending way too much time both reading and leaving comments. But so what, it was still worth it! Laure Alexandr Peirce